akonadi
#include <contactstreemodel.h>
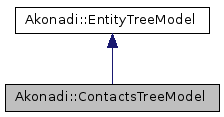
Public Member Functions | |
ContactsTreeModel (ChangeRecorder *monitor, QObject *parent=0) | |
virtual | ~ContactsTreeModel () |
Columns | columns () const |
void | setColumns (const Columns &columns) |
![]() | |
EntityTreeModel (ChangeRecorder *monitor, QObject *parent=0) | |
virtual | ~EntityTreeModel () |
virtual bool | canFetchMore (const QModelIndex &parent) const |
CollectionFetchStrategy | collectionFetchStrategy () const |
virtual int | columnCount (const QModelIndex &parent=QModelIndex()) const |
virtual QVariant | data (const QModelIndex &index, int role=Qt::DisplayRole) const |
virtual bool | dropMimeData (const QMimeData *data, Qt::DropAction action, int row, int column, const QModelIndex &parent) |
virtual void | fetchMore (const QModelIndex &parent) |
virtual Qt::ItemFlags | flags (const QModelIndex &index) const |
virtual bool | hasChildren (const QModelIndex &parent=QModelIndex()) const |
virtual QVariant | headerData (int section, Qt::Orientation orientation, int role=Qt::DisplayRole) const |
bool | includeRootCollection () const |
bool | includeUnsubscribed () const |
virtual QModelIndex | index (int row, int column, const QModelIndex &parent=QModelIndex()) const |
bool | isCollectionPopulated (Akonadi::Collection::Id) const |
bool | isCollectionTreeFetched () const |
ItemPopulationStrategy | itemPopulationStrategy () const |
virtual QModelIndexList | match (const QModelIndex &start, int role, const QVariant &value, int hits=1, Qt::MatchFlags flags=Qt::MatchFlags(Qt::MatchStartsWith|Qt::MatchWrap)) const |
virtual QMimeData * | mimeData (const QModelIndexList &indexes) const |
virtual QStringList | mimeTypes () const |
virtual QModelIndex | parent (const QModelIndex &index) const |
QString | rootCollectionDisplayName () const |
virtual int | rowCount (const QModelIndex &parent=QModelIndex()) const |
void | setCollectionFetchStrategy (CollectionFetchStrategy strategy) |
virtual bool | setData (const QModelIndex &index, const QVariant &value, int role=Qt::EditRole) |
void | setIncludeRootCollection (bool include) |
void | setIncludeUnsubscribed (bool show) |
void | setItemPopulationStrategy (ItemPopulationStrategy strategy) |
void | setRootCollectionDisplayName (const QString &name) |
void | setShowSystemEntities (bool show) |
virtual Qt::DropActions | supportedDropActions () const |
bool | systemEntitiesShown () const |
Additional Inherited Members | |
![]() | |
void | collectionFetched (int collectionId) |
void | collectionPopulated (Akonadi::Collection::Id collectionId) |
void | collectionTreeFetched (const Akonadi::Collection::List &collections) |
![]() | |
static QModelIndexList | modelIndexesForItem (const QAbstractItemModel *model, const Item &item) |
static QModelIndex | modelIndexForCollection (const QAbstractItemModel *model, const Collection &collection) |
![]() | |
void | clearAndReset () |
virtual int | entityColumnCount (HeaderGroup headerGroup) const |
virtual QVariant | entityData (const Item &item, int column, int role=Qt::DisplayRole) const |
virtual QVariant | entityData (const Collection &collection, int column, int role=Qt::DisplayRole) const |
virtual QVariant | entityHeaderData (int section, Qt::Orientation orientation, int role, HeaderGroup headerGroup) const |
virtual bool | entityMatch (const Item &item, const QVariant &value, Qt::MatchFlags flags) const |
virtual bool | entityMatch (const Collection &collection, const QVariant &value, Qt::MatchFlags flags) const |
Detailed Description
A model for contacts and contact groups as available in Akonadi.
This class provides a model for displaying the contacts and contact groups which are available from Akonadi.
Example:
- Since
- 4.5
Definition at line 78 of file contactstreemodel.h.
Member Typedef Documentation
typedef QList<Column> Akonadi::ContactsTreeModel::Columns |
Describes a list of columns of the contacts tree model.
Definition at line 156 of file contactstreemodel.h.
Member Enumeration Documentation
Describes the columns that can be shown by the model.
Definition at line 86 of file contactstreemodel.h.
Describes the role for contacts and contact groups.
Enumerator | |
---|---|
DateRole |
The QDate object for the current index. |
Definition at line 161 of file contactstreemodel.h.
Constructor & Destructor Documentation
|
explicit |
Creates a new contacts tree model.
- Parameters
-
monitor The ChangeRecorder whose entities should be represented in the model. parent The parent object.
Definition at line 48 of file contactstreemodel.cpp.
|
virtual |
Destroys the contacts tree model.
Definition at line 53 of file contactstreemodel.cpp.
Member Function Documentation
ContactsTreeModel::Columns ContactsTreeModel::columns | ( | ) | const |
Returns the columns that the model currently shows.
Definition at line 65 of file contactstreemodel.cpp.
void ContactsTreeModel::setColumns | ( | const Columns & | columns | ) |
Sets the columns
that the model should show.
Definition at line 58 of file contactstreemodel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:00:28 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.