kabc
#include <stdaddressbook.h>
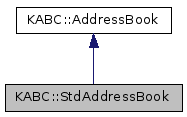
Public Member Functions | |
~StdAddressBook () | |
void | setWhoAmI (const Addressee &addr) |
Addressee | whoAmI () const |
![]() | |
AddressBook () | |
AddressBook (const QString &config) | |
virtual | ~AddressBook () |
bool | addCustomField (const QString &label, int category=Field::All, const QString &key=QString(), const QString &app=QString()) const |
bool | addResource (Resource *resource) |
Addressee::List | allAddressees () const |
QStringList | allDistributionListNames () const |
QList< DistributionList * > | allDistributionLists () |
bool | asyncLoad () |
bool | asyncSave (Ticket *ticket) |
ConstIterator | begin () const |
Iterator | begin () |
void | clear () |
ConstIterator | constBegin () const |
ConstIterator | constEnd () const |
DistributionList * | createDistributionList (const QString &name, Resource *resource=0) |
void | dump () const |
void | emitAddressBookChanged () |
void | emitAddressBookLocked () |
void | emitAddressBookUnlocked () |
ConstIterator | end () const |
Iterator | end () |
void | error (const QString &msg) |
Field::List | fields (int category=Field::All) const |
Iterator | find (const Addressee &addr) |
ConstIterator | find (const Addressee &addr) const |
Addressee::List | findByCategory (const QString &category) const |
Addressee::List | findByEmail (const QString &email) const |
Addressee::List | findByName (const QString &name) const |
Addressee | findByUid (const QString &uid) const |
DistributionList * | findDistributionListByIdentifier (const QString &identifier) |
DistributionList * | findDistributionListByName (const QString &name, Qt::CaseSensitivity caseSensitivity=Qt::CaseSensitive) |
virtual QString | identifier () const |
void | insertAddressee (const Addressee &addr) |
bool | load () |
bool | loadingHasFinished () const |
void | releaseSaveTicket (Ticket *ticket) |
void | removeAddressee (const Addressee &addr) |
void | removeAddressee (const Iterator &it) |
void | removeDistributionList (DistributionList *list) |
bool | removeResource (Resource *resource) |
Ticket * | requestSaveTicket (Resource *resource=0) |
QList< Resource * > | resources () const |
bool | save (Ticket *ticket) |
void | setErrorHandler (ErrorHandler *errorHandler) |
Static Public Member Functions | |
static bool | automaticSave () |
static void | close () |
static QString | directoryName () |
static QString | fileName () |
static KABC_DEPRECATED bool | save () |
static StdAddressBook * | self () |
static StdAddressBook * | self (bool asynchronous) |
static void | setAutomaticSave (bool state) |
Protected Member Functions | |
StdAddressBook (bool asynchronous) | |
![]() | |
KRES::Manager< Resource > * | resourceManager () |
void | setStandardResource (Resource *resource) |
Resource * | standardResource () |
Additional Inherited Members | |
![]() | |
void | addressBookChanged (AddressBook *addressBook) |
void | addressBookLocked (AddressBook *addressBook) |
void | addressBookUnlocked (AddressBook *addressBook) |
void | loadingFinished (Resource *resource) |
void | savingFinished (Resource *resource) |
![]() | |
void | resourceLoadingError (Resource *resource, const QString &errMsg) |
void | resourceLoadingFinished (Resource *resource) |
void | resourceSavingError (Resource *resource, const QString &errMsg) |
void | resourceSavingFinished (Resource *resource) |
Detailed Description
Standard KDE address book.
This class provides access to the standard KDE address book shared by all applications.
It's implemented as a singleton. Use self() to get the address book object. On the first self() call the address book also gets loaded.
Example:
- Deprecated:
- Port to libakonadi-kontact. For instance using Akonadi::ContactSearchJob. See http://techbase.kde.org/Development/AkonadiPorting/AddressBook for details.
Definition at line 59 of file stdaddressbook.h.
Constructor & Destructor Documentation
StdAddressBook::~StdAddressBook | ( | ) |
Destructor.
Definition at line 135 of file stdaddressbook.cpp.
Member Function Documentation
|
static |
Returns whether the address book is saved at destruction time.
See also setAutomaticSave().
Definition at line 235 of file stdaddressbook.cpp.
|
static |
Closes the address book.
Depending on automaticSave() it will save the address book first.
Definition at line 224 of file stdaddressbook.cpp.
|
static |
Returns the default directory name for vcard-based addressbook.
Definition at line 69 of file stdaddressbook.cpp.
|
static |
Returns the default file name for vcard-based addressbook.
Definition at line 64 of file stdaddressbook.cpp.
|
static |
Saves the standard address book to disk.
- Deprecated:
- Use AddressBook::save( Ticket* ) instead
Definition at line 213 of file stdaddressbook.cpp.
|
static |
Returns the standard addressbook object.
It also loads all resources of the users standard address book synchronously.
Definition at line 74 of file stdaddressbook.cpp.
|
static |
This is the same as above, but with specified behaviour of resource loading.
- Parameters
-
asynchronous When true, the resources are loaded asynchronous, that means you have the data foremost the addressBookChanged() signal has been emitted. So connect to this signal when using this method!
Definition at line 84 of file stdaddressbook.cpp.
|
static |
Sets the automatic save property of the address book.
- Parameters
-
state If true, the address book is saved automatically at destruction time, otherwise you have to call AddressBook::save( Ticket* ).
Definition at line 230 of file stdaddressbook.cpp.
void StdAddressBook::setWhoAmI | ( | const Addressee & | addr | ) |
Sets the users contact.
See whoAmI() for more information.
- Parameters
-
addr The users contact.
Definition at line 248 of file stdaddressbook.cpp.
Addressee StdAddressBook::whoAmI | ( | ) | const |
Returns the contact, that is associated with the owner of the address book.
This contact should be used by other programs to access user specific data.
Definition at line 240 of file stdaddressbook.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:01:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.