KCal Library
#include <incidencebase.h>
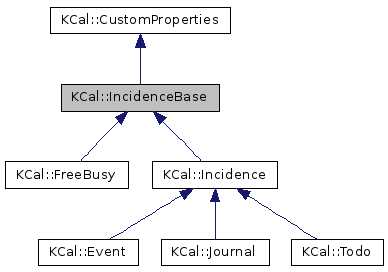
Classes | |
class | IncidenceObserver |
class | Visitor |
Public Member Functions | |
IncidenceBase () | |
IncidenceBase (const IncidenceBase &ib) | |
virtual | ~IncidenceBase () |
virtual bool | accept (Visitor &v) |
void | addAttendee (Attendee *attendee, bool doUpdate=true) |
void | addComment (const QString &comment) |
bool | allDay () const |
Attendee * | attendeeByMail (const QString &email) const |
Attendee * | attendeeByMails (const QStringList &emails, const QString &email=QString()) const |
Attendee * | attendeeByUid (const QString &uid) const |
int | attendeeCount () const |
const Attendee::List & | attendees () const |
void | clearAttendees () |
void | clearComments () |
QStringList | comments () const |
virtual KDateTime | dtStart () const |
virtual KCAL_DEPRECATED QString | dtStartDateStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
virtual KCAL_DEPRECATED QString | dtStartStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
virtual KCAL_DEPRECATED QString | dtStartTimeStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
Duration | duration () const |
void | endUpdates () |
bool | hasDuration () const |
bool | isReadOnly () const |
KDateTime | lastModified () const |
IncidenceBase & | operator= (const IncidenceBase &other) |
bool | operator== (const IncidenceBase &ib) const |
Person | organizer () const |
void | registerObserver (IncidenceObserver *observer) |
bool | removeComment (const QString &comment) |
void | setAllDay (bool allDay) |
virtual void | setDtStart (const KDateTime &dtStart) |
virtual void | setDuration (const Duration &duration) |
void | setHasDuration (bool hasDuration) |
void | setLastModified (const KDateTime &lm) |
void | setOrganizer (const Person &organizer) |
void | setOrganizer (const QString &organizer) |
virtual void | setReadOnly (bool readOnly) |
void | setUid (const QString &uid) |
virtual void | shiftTimes (const KDateTime::Spec &oldSpec, const KDateTime::Spec &newSpec) |
void | startUpdates () |
virtual QByteArray | type () const =0 |
QString | uid () const |
void | unRegisterObserver (IncidenceObserver *observer) |
void | updated () |
KUrl | uri () const |
![]() | |
CustomProperties () | |
CustomProperties (const CustomProperties &other) | |
virtual | ~CustomProperties () |
QMap< QByteArray, QString > | customProperties () const |
QString | customProperty (const QByteArray &app, const QByteArray &key) const |
QString | nonKDECustomProperty (const QByteArray &name) const |
CustomProperties & | operator= (const CustomProperties &other) |
bool | operator== (const CustomProperties &properties) const |
void | removeCustomProperty (const QByteArray &app, const QByteArray &key) |
void | removeNonKDECustomProperty (const QByteArray &name) |
void | setCustomProperties (const QMap< QByteArray, QString > &properties) |
void | setCustomProperty (const QByteArray &app, const QByteArray &key, const QString &value) |
void | setNonKDECustomProperty (const QByteArray &name, const QString &value) |
Protected Member Functions | |
virtual void | customPropertyUpdated () |
Protected Attributes | |
bool | mReadOnly |
Additional Inherited Members | |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
Detailed Description
An abstract class that provides a common base for all calendar incidence classes.
define: organizer (person) define: uid (same as the attendee uid?)
Several properties are not allowed for VFREEBUSY objects (see rfc:2445), so they are not in IncidenceBase. The hierarchy is:
So IncidenceBase contains all properties that are common to all classes, and Incidence contains all additional properties that are common to Events, Todos and Journals, but are not allowed for FreeBusy entries.
Definition at line 102 of file incidencebase.h.
Constructor & Destructor Documentation
IncidenceBase::IncidenceBase | ( | ) |
Constructs an empty IncidenceBase.
Private class that helps to provide binary compatibility between releases.
Definition at line 111 of file incidencebase.cpp.
IncidenceBase::IncidenceBase | ( | const IncidenceBase & | ib | ) |
Constructs an IncidenceBase as a copy of another IncidenceBase object.
- Parameters
-
ib is the IncidenceBase to copy.
Definition at line 119 of file incidencebase.cpp.
|
virtual |
Destroys the IncidenceBase.
Definition at line 126 of file incidencebase.cpp.
Member Function Documentation
|
inlinevirtual |
Accept IncidenceVisitor.
A class taking part in the visitor mechanism has to provide this implementation:
bool accept(Visitor &v) { return v.visit(this); }
- Parameters
-
v is a reference to a Visitor object.
Definition at line 228 of file incidencebase.h.
void IncidenceBase::addAttendee | ( | Attendee * | attendee, |
bool | doUpdate = true |
||
) |
Add Attendee to this incidence.
IncidenceBase takes ownership of the Attendee object.
- Parameters
-
attendee a pointer to the attendee to add doUpdate If true the Observers are notified, if false they are not.
Definition at line 362 of file incidencebase.cpp.
void IncidenceBase::addComment | ( | const QString & | comment | ) |
Adds a comment to thieincidence.
Does not add a linefeed character; simply appends the text as specified.
- Parameters
-
comment is the QString containing the comment to add.
- See also
- removeComment().
Definition at line 332 of file incidencebase.cpp.
bool IncidenceBase::allDay | ( | ) | const |
Returns true or false depending on whether the incidence is all-day.
i.e. has a date but no time attached to it.
- See also
- setAllDay()
Definition at line 310 of file incidencebase.cpp.
Attendee * IncidenceBase::attendeeByMail | ( | const QString & | ) | const |
Returns the attendee with the specified email address.
- Parameters
-
email is a QString containing an email address of the form "FirstName LastName <emailaddress>".
- See also
- attendeeByMails(), attendeesByUid().
Definition at line 397 of file incidencebase.cpp.
Attendee * IncidenceBase::attendeeByMails | ( | const QStringList & | emails, |
const QString & | email = QString() |
||
) | const |
Returns the first incidence attendee with one of the specified email addresses.
- Parameters
-
emails is a list of QStrings containing email addresses of the form "FirstName LastName <emailaddress>". email is a QString containing a single email address to search in addition to the list specified in emails
.
- See also
- attendeeByMail(), attendeesByUid().
Definition at line 409 of file incidencebase.cpp.
Attendee * IncidenceBase::attendeeByUid | ( | const QString & | uid | ) | const |
Returns the incidence attendee with the specified attendee UID.
- Parameters
-
uid is a QString containing an attendee UID.
- See also
- attendeeByMail(), attendeeByMails().
Definition at line 429 of file incidencebase.cpp.
int IncidenceBase::attendeeCount | ( | ) | const |
Returns the number of incidence attendees.
Definition at line 383 of file incidencebase.cpp.
const Attendee::List & IncidenceBase::attendees | ( | ) | const |
Returns a list of incidence attendees.
Definition at line 378 of file incidencebase.cpp.
void IncidenceBase::clearAttendees | ( | ) |
Removes all attendees from the incidence.
Definition at line 388 of file incidencebase.cpp.
void IncidenceBase::clearComments | ( | ) |
Deletes all incidence comments.
Definition at line 352 of file incidencebase.cpp.
QStringList IncidenceBase::comments | ( | ) | const |
Returns all incidence comments as a list of strings.
Definition at line 357 of file incidencebase.cpp.
|
protectedvirtual |
CustomProperties::customPropertyUpdated()
Reimplemented from KCal::CustomProperties.
Definition at line 503 of file incidencebase.cpp.
|
virtual |
Returns an incidence's starting date/time as a KDateTime.
- See also
- setDtStart().
Reimplemented in KCal::Todo.
Definition at line 248 of file incidencebase.cpp.
|
virtual |
Returns an incidence's starting date as a string formatted according to the user's locale settings.
- Parameters
-
shortfmt If set to true, use short date format, if set to false use long format. spec If set, return the date in the given spec, else use the incidence's current spec.
Reimplemented in KCal::Todo.
Definition at line 269 of file incidencebase.cpp.
|
virtual |
Returns an incidence's starting date and time as a string formatted according to the user's locale settings.
- Parameters
-
shortfmt If set to true, use short date format, if set to false use long format. spec If set, return the date and time in the given spec, else use the incidence's current spec.
Reimplemented in KCal::Todo.
Definition at line 287 of file incidencebase.cpp.
|
virtual |
Returns an incidence's starting time as a string formatted according to the user's locale settings.
- Parameters
-
shortfmt If set to true, use short date format, if set to false use long format. spec If set, return the time in the given spec, else use the incidence's current spec.
Reimplemented in KCal::Todo.
Definition at line 253 of file incidencebase.cpp.
Duration IncidenceBase::duration | ( | ) | const |
Returns the length of the incidence duration.
- See also
- setDuration()
Definition at line 448 of file incidencebase.cpp.
void IncidenceBase::endUpdates | ( | ) |
Call this when a group of updates is complete, to notify observers that the instance has changed.
This should be called in conjunction with startUpdates().
Definition at line 493 of file incidencebase.cpp.
bool IncidenceBase::hasDuration | ( | ) | const |
Returns true if the incidence has a duration; false otherwise.
- See also
- setHasDuration()
Definition at line 458 of file incidencebase.cpp.
|
inline |
Returns true the object is read-only; false otherwise.
- See also
- setReadOnly()
Definition at line 318 of file incidencebase.h.
KDateTime IncidenceBase::lastModified | ( | ) | const |
Returns the time the incidence was last modified.
- See also
- setLastModified()
Definition at line 203 of file incidencebase.cpp.
IncidenceBase & IncidenceBase::operator= | ( | const IncidenceBase & | other | ) |
Assignment operator.
- Warning
- Not polymorphic. Use AssignmentVisitor for correct assignment of an instance of type IncidenceBase to another instance of type IncidenceBase.
- Parameters
-
other is the IncidenceBase to assign.
- See also
- AssignmentVisitor
Definition at line 131 of file incidencebase.cpp.
bool IncidenceBase::operator== | ( | const IncidenceBase & | ib | ) | const |
Compares this with IncidenceBase ib
for equality.
- Warning
- Not polymorphic. Use ComparisonVisitor for correct comparison of two instances of type IncidenceBase.
- Parameters
-
ib is the IncidenceBase to compare.
- See also
- ComparisonVisitor
Definition at line 144 of file incidencebase.cpp.
Person IncidenceBase::organizer | ( | ) | const |
Returns the Person associated with this incidence.
Definition at line 230 of file incidencebase.cpp.
void IncidenceBase::registerObserver | ( | IncidenceBase::IncidenceObserver * | observer | ) |
Register observer.
The observer is notified when the observed object changes.
- Parameters
-
observer is a pointer to an IncidenceObserver object that will be watching this incidence.
- See also
- unRegisterObserver()
Definition at line 463 of file incidencebase.cpp.
bool IncidenceBase::removeComment | ( | const QString & | comment | ) |
Removes a comment from the incidence.
Removes the first comment whose string is an exact match for the specified string in comment
.
- Parameters
-
comment is the QString containing the comment to remove.
- Returns
- true if match found, false otherwise.
- See also
- addComment().
Definition at line 337 of file incidencebase.cpp.
void IncidenceBase::setAllDay | ( | bool | allDay | ) |
Sets whether the incidence is all-day, i.e.
has a date but no time attached to it.
- Parameters
-
allDay sets whether the incidence is all-day.
- See also
- allDay()
Definition at line 315 of file incidencebase.cpp.
|
virtual |
Sets the incidence's starting date/time with a KDateTime.
The incidence's all-day status is set according to whether dtStart
is a date/time (not all-day) or date-only (all-day).
- Parameters
-
dtStart is the incidence start date/time.
- See also
- dtStart().
Reimplemented in KCal::Incidence, KCal::Todo, and KCal::FreeBusy.
Definition at line 240 of file incidencebase.cpp.
|
virtual |
Sets the incidence duration.
- Parameters
-
duration the incidence duration
- See also
- duration()
Reimplemented in KCal::Event.
Definition at line 441 of file incidencebase.cpp.
void IncidenceBase::setHasDuration | ( | bool | hasDuration | ) |
Sets if the incidence has a duration.
- Parameters
-
hasDuration true if the incidence has a duration; false otherwise.
- See also
- hasDuration()
Definition at line 453 of file incidencebase.cpp.
void IncidenceBase::setLastModified | ( | const KDateTime & | lm | ) |
Sets the time the incidence was last modified to lm
.
It is stored as a UTC date/time.
- Parameters
-
lm is the KDateTime when the incidence was last modified.
- See also
- lastModified()
Definition at line 189 of file incidencebase.cpp.
void IncidenceBase::setOrganizer | ( | const Person & | organizer | ) |
Sets the organizer for the incidence.
- See also
- organizer(), setOrganizer(const QString &)
Definition at line 208 of file incidencebase.cpp.
void IncidenceBase::setOrganizer | ( | const QString & | organizer | ) |
Sets the incidence organizer to any string organizer
.
- Parameters
-
organizer is a string to use as the incidence organizer.
- See also
- organizer(), setOrganizer(const Person &)
Definition at line 218 of file incidencebase.cpp.
|
virtual |
Sets readonly status.
- Parameters
-
readOnly if set, the incidence is read-only; else the incidence can be modified.
- See also
- isReadOnly().
Reimplemented in KCal::Incidence.
Definition at line 235 of file incidencebase.cpp.
void IncidenceBase::setUid | ( | const QString & | uid | ) |
Returns the type of Incidence as a translated string.
Sets the unique id for the incidence to uid
.
- Parameters
-
uid is the string containing the incidence uid.
- See also
- uid()
Definition at line 178 of file incidencebase.cpp.
|
virtual |
Shift the times of the incidence so that they appear at the same clock time as before but in a new time zone.
The shift is done from a viewing time zone rather than from the actual incidence time zone.
For example, shifting an incidence whose start time is 09:00 America/New York, using an old viewing time zone (oldSpec
) of Europe/London, to a new time zone (newSpec
) of Europe/Paris, will result in the time being shifted from 14:00 (which is the London time of the incidence start) to 14:00 Paris time.
- Parameters
-
oldSpec the time specification which provides the clock times newSpec the new time specification
Reimplemented in KCal::Todo, KCal::Incidence, KCal::Event, and KCal::FreeBusy.
Definition at line 324 of file incidencebase.cpp.
void IncidenceBase::startUpdates | ( | ) |
Call this when a group of updates is going to be made.
This suppresses change notifications until endUpdates() is called, at which point updated() will automatically be called.
Definition at line 488 of file incidencebase.cpp.
|
pure virtual |
Prints the type of Incidence as a string.
Implemented in KCal::FreeBusy, KCal::Event, KCal::Todo, and KCal::Journal.
QString IncidenceBase::uid | ( | ) | const |
Returns the unique id (uid) for the incidence.
- See also
- setUid()
Definition at line 184 of file incidencebase.cpp.
void IncidenceBase::unRegisterObserver | ( | IncidenceBase::IncidenceObserver * | observer | ) |
Unregister observer.
It isn't notified anymore about changes.
- Parameters
-
observer is a pointer to an IncidenceObserver object that will be watching this incidence.
- See also
- registerObserver().
Definition at line 470 of file incidencebase.cpp.
void IncidenceBase::updated | ( | ) |
Call this to notify the observers after the IncidenceBase object has changed.
Definition at line 475 of file incidencebase.cpp.
KUrl IncidenceBase::uri | ( | ) | const |
Returns the uri for the incidence, of form urn:x-ical:<uid>
Definition at line 508 of file incidencebase.cpp.
Member Data Documentation
|
protected |
Identifies a read-only incidence.
Definition at line 577 of file incidencebase.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:00:58 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.