KCal Library
#include <freebusy.h>
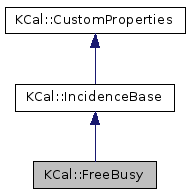
Public Member Functions | |
FreeBusy () | |
FreeBusy (const FreeBusy &other) | |
FreeBusy (const Period::List &busyPeriods) | |
FreeBusy (const FreeBusyPeriod::List &busyPeriods) | |
FreeBusy (const KDateTime &start, const KDateTime &end) | |
FreeBusy (Calendar *calendar, const KDateTime &start, const KDateTime &end) | |
FreeBusy (const Event::List &events, const KDateTime &start, const KDateTime &end) | |
~FreeBusy () | |
void | addPeriod (const KDateTime &start, const KDateTime &end) |
void | addPeriod (const KDateTime &start, const Duration &duration) |
void | addPeriods (const Period::List &list) |
void | addPeriods (const FreeBusyPeriod::List &list) |
Period::List | busyPeriods () const |
virtual KDateTime | dtEnd () const |
FreeBusyPeriod::List | fullBusyPeriods () const |
void | merge (FreeBusy *freebusy) |
FreeBusy & | operator= (const FreeBusy &other) |
bool | operator== (const FreeBusy &freebusy) const |
void | setDtEnd (const KDateTime &end) |
virtual void | setDtStart (const KDateTime &start) |
virtual void | shiftTimes (const KDateTime::Spec &oldSpec, const KDateTime::Spec &newSpec) |
void | sortList () |
QByteArray | type () const |
![]() | |
IncidenceBase () | |
IncidenceBase (const IncidenceBase &ib) | |
virtual | ~IncidenceBase () |
void | addAttendee (Attendee *attendee, bool doUpdate=true) |
void | addComment (const QString &comment) |
bool | allDay () const |
Attendee * | attendeeByMail (const QString &email) const |
Attendee * | attendeeByMails (const QStringList &emails, const QString &email=QString()) const |
Attendee * | attendeeByUid (const QString &uid) const |
int | attendeeCount () const |
const Attendee::List & | attendees () const |
void | clearAttendees () |
void | clearComments () |
QStringList | comments () const |
virtual KDateTime | dtStart () const |
virtual KCAL_DEPRECATED QString | dtStartDateStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
virtual KCAL_DEPRECATED QString | dtStartStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
virtual KCAL_DEPRECATED QString | dtStartTimeStr (bool shortfmt=true, const KDateTime::Spec &spec=KDateTime::Spec()) const |
Duration | duration () const |
void | endUpdates () |
bool | hasDuration () const |
bool | isReadOnly () const |
KDateTime | lastModified () const |
IncidenceBase & | operator= (const IncidenceBase &other) |
bool | operator== (const IncidenceBase &ib) const |
Person | organizer () const |
void | registerObserver (IncidenceObserver *observer) |
bool | removeComment (const QString &comment) |
void | setAllDay (bool allDay) |
virtual void | setDuration (const Duration &duration) |
void | setHasDuration (bool hasDuration) |
void | setLastModified (const KDateTime &lm) |
void | setOrganizer (const Person &organizer) |
void | setOrganizer (const QString &organizer) |
virtual void | setReadOnly (bool readOnly) |
void | setUid (const QString &uid) |
void | startUpdates () |
QString | uid () const |
void | unRegisterObserver (IncidenceObserver *observer) |
void | updated () |
KUrl | uri () const |
![]() | |
CustomProperties () | |
CustomProperties (const CustomProperties &other) | |
virtual | ~CustomProperties () |
QMap< QByteArray, QString > | customProperties () const |
QString | customProperty (const QByteArray &app, const QByteArray &key) const |
QString | nonKDECustomProperty (const QByteArray &name) const |
CustomProperties & | operator= (const CustomProperties &other) |
bool | operator== (const CustomProperties &properties) const |
void | removeCustomProperty (const QByteArray &app, const QByteArray &key) |
void | removeNonKDECustomProperty (const QByteArray &name) |
void | setCustomProperties (const QMap< QByteArray, QString > &properties) |
void | setCustomProperty (const QByteArray &app, const QByteArray &key, const QString &value) |
void | setNonKDECustomProperty (const QByteArray &name, const QString &value) |
Additional Inherited Members | |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
![]() | |
virtual void | customPropertyUpdated () |
![]() | |
bool | mReadOnly |
Detailed Description
Provides information about the free/busy time of a calendar.
A free/busy is a collection of Periods (
- See also
- Period).
Definition at line 50 of file freebusy.h.
Constructor & Destructor Documentation
FreeBusy::FreeBusy | ( | ) |
Constructs an free/busy without any periods.
Definition at line 76 of file freebusy.cpp.
FreeBusy::FreeBusy | ( | const FreeBusy & | other | ) |
Copy constructor.
- Parameters
-
other is the free/busy to copy.
Definition at line 81 of file freebusy.cpp.
|
explicit |
Constructs a free/busy from a list of periods.
- Parameters
-
busyPeriods is a QList of periods.
Definition at line 201 of file freebusy.cpp.
|
explicit |
Constructs a free/busy from a list of periods.
- Parameters
-
busyPeriods is a QList of periods.
Definition at line 207 of file freebusy.cpp.
FreeBusy::FreeBusy | ( | const KDateTime & | start, |
const KDateTime & | end | ||
) |
Constructs a free/busy from a single period.
- Parameters
-
start is the start datetime of the period. end is the end datetime of the period.
Definition at line 87 of file freebusy.cpp.
FreeBusy::FreeBusy | ( | Calendar * | calendar, |
const KDateTime & | start, | ||
const KDateTime & | end | ||
) |
Constructs a freebusy for a specified calendar give a single period.
- Parameters
-
calendar is a pointer to a valid Calendar object. start is the start datetime of the period. end is the end datetime of the period.
Definition at line 190 of file freebusy.cpp.
FreeBusy::FreeBusy | ( | const Event::List & | events, |
const KDateTime & | start, | ||
const KDateTime & | end | ||
) |
Constructs a freebusy for a specified list of events given a single period.
- Parameters
-
events list of events. start is the start datetime of the period. end is the end datetime of the period.
- Since
- 4.4
Definition at line 94 of file freebusy.cpp.
FreeBusy::~FreeBusy | ( | ) |
Destroys a free/busy.
Definition at line 212 of file freebusy.cpp.
Member Function Documentation
void FreeBusy::addPeriod | ( | const KDateTime & | start, |
const KDateTime & | end | ||
) |
Adds a period to the freebusy list and sorts the list.
- Parameters
-
start is the start datetime of the period. end is the end datetime of the period.
Definition at line 280 of file freebusy.cpp.
void FreeBusy::addPeriod | ( | const KDateTime & | start, |
const Duration & | duration | ||
) |
Adds a period to the freebusy list and sorts the list.
- Parameters
-
start is the start datetime of the period. duration is the Duration of the period.
Definition at line 286 of file freebusy.cpp.
void FreeBusy::addPeriods | ( | const Period::List & | list | ) |
Adds a list of periods to the freebusy object and then sorts that list.
Use this if you are adding many items, instead of the addPeriod method, to avoid sorting repeatedly.
- Parameters
-
list is a QList of Period objects.
Definition at line 266 of file freebusy.cpp.
void FreeBusy::addPeriods | ( | const FreeBusyPeriod::List & | list | ) |
Adds a list of periods to the freebusy object and then sorts that list.
Use this if you are adding many items, instead of the addPeriod method, to avoid sorting repeatedly.
- Parameters
-
list is a QList of FreeBusyPeriod objects.
Definition at line 274 of file freebusy.cpp.
Period::List FreeBusy::busyPeriods | ( | ) | const |
Returns the list of all periods within the free/busy.
Definition at line 244 of file freebusy.cpp.
|
virtual |
Returns the end datetime for the free/busy.
FIXME: calling addPeriod() does not change mDtEnd. Is that incorrect?
- See also
- setDtEnd().
Definition at line 239 of file freebusy.cpp.
FreeBusyPeriod::List FreeBusy::fullBusyPeriods | ( | ) | const |
Returns the list of all periods within the free/busy.
Definition at line 255 of file freebusy.cpp.
void FreeBusy::merge | ( | FreeBusy * | freebusy | ) |
Merges another free/busy into this free/busy.
- Parameters
-
freebusy is a pointer to a valid FreeBusy object.
Definition at line 292 of file freebusy.cpp.
Assignment operator.
Definition at line 323 of file freebusy.cpp.
bool FreeBusy::operator== | ( | const FreeBusy & | freebusy | ) | const |
Compare this with freebusy
for equality.
- Parameters
-
freebusy is the FreeBusy to compare.
Definition at line 335 of file freebusy.cpp.
void FreeBusy::setDtEnd | ( | const KDateTime & | end | ) |
Sets the end datetime for the free/busy.
Note that this datetime may be later or earlier than all periods within the free/busy.
- Parameters
-
end is a KDateTime specifying an end datetime.
- See also
- dtEnd(), setDtStart().
Definition at line 234 of file freebusy.cpp.
|
virtual |
IncidenceBase::typeStr() Sets the start datetime for the free/busy. Note that this datetime may be later or earlier than all periods within the free/busy.
- Parameters
-
start is a KDateTime specifying an start datetime.
- See also
- IncidenceBase::dtStart(), setDtEnd().
Reimplemented from KCal::IncidenceBase.
Definition at line 228 of file freebusy.cpp.
|
virtual |
Reimplemented from KCal::IncidenceBase.
Definition at line 310 of file freebusy.cpp.
void FreeBusy::sortList | ( | ) |
Sorts the list of free/busy periods into ascending order.
Definition at line 260 of file freebusy.cpp.
|
virtual |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:00:58 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.