KCal Library
#include <calendar.h>
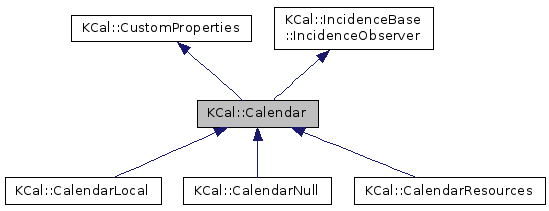
Classes | |
class | CalendarObserver |
Signals | |
void | batchAddingBegins () |
void | batchAddingEnds () |
void | calendarChanged () |
void | calendarLoaded () |
void | calendarSaved () |
Public Member Functions | |
Calendar (const KDateTime::Spec &timeSpec) | |
Calendar (const QString &timeZoneId) | |
virtual | ~Calendar () |
virtual bool | addEvent (Event *event)=0 |
virtual bool | addIncidence (Incidence *incidence) |
virtual bool | addJournal (Journal *journal)=0 |
virtual bool | addTodo (Todo *todo)=0 |
virtual Alarm::List | alarms (const KDateTime &from, const KDateTime &to)=0 |
void | beginBatchAdding () |
virtual bool | beginChange (Incidence *incidence) |
QStringList | categories () |
virtual void | close ()=0 |
virtual void | deleteAllEvents ()=0 |
virtual void | deleteAllJournals ()=0 |
virtual void | deleteAllTodos ()=0 |
virtual bool | deleteEvent (Event *event)=0 |
virtual bool | deleteIncidence (Incidence *incidence) |
virtual bool | deleteJournal (Journal *journal)=0 |
virtual bool | deleteTodo (Todo *todo)=0 |
Incidence * | dissociateOccurrence (Incidence *incidence, const QDate &date, const KDateTime::Spec &spec, bool single=true) |
void | endBatchAdding () |
virtual bool | endChange (Incidence *incidence) |
virtual Event * | event (const QString &uid)=0 |
virtual Event::List | events (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Event::List | events (const KDateTime &dt) |
Event::List | events (const QDate &start, const QDate &end, const KDateTime::Spec &timeSpec=KDateTime::Spec(), bool inclusive=false) |
Event::List | events (const QDate &date, const KDateTime::Spec &timeSpec=KDateTime::Spec(), EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
CalFilter * | filter () |
Incidence * | incidence (const QString &uid) |
Incidence * | incidenceFromSchedulingID (const QString &sid) |
virtual Incidence::List | incidences () |
virtual Incidence::List | incidences (const QDate &date) |
Incidence::List | incidencesFromSchedulingID (const QString &sid) |
bool | isAncestorOf (Incidence *ancestor, Incidence *incidence) |
bool | isModified () const |
virtual bool | isSaving () |
virtual Journal * | journal (const QString &uid)=0 |
virtual Journal::List | journals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
virtual Journal::List | journals (const QDate &date) |
Person | owner () const |
QString | productId () const |
virtual Event::List | rawEvents (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Event::List | rawEvents (const QDate &start, const QDate &end, const KDateTime::Spec &timeSpec=KDateTime::Spec(), bool inclusive=false)=0 |
virtual Event::List | rawEventsForDate (const KDateTime &dt)=0 |
virtual Event::List | rawEventsForDate (const QDate &date, const KDateTime::Spec &timeSpec=KDateTime::Spec(), EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Incidence::List | rawIncidences () |
virtual Journal::List | rawJournals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Journal::List | rawJournalsForDate (const QDate &date)=0 |
virtual Todo::List | rawTodos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending)=0 |
virtual Todo::List | rawTodosForDate (const QDate &date)=0 |
void | registerObserver (CalendarObserver *observer) |
virtual bool | reload ()=0 |
virtual void | removeRelations (Incidence *incidence) |
virtual bool | save ()=0 |
void | setFilter (CalFilter *filter) |
void | setModified (bool modified) |
void | setOwner (const Person &owner) |
void | setProductId (const QString &id) |
void | setTimeSpec (const KDateTime::Spec &timeSpec) |
void | setTimeZoneId (const QString &timeZoneId) |
void | setTimeZones (const ICalTimeZones &zones) |
virtual void | setupRelations (Incidence *incidence) |
void | setViewTimeSpec (const KDateTime::Spec &timeSpec) const |
void | setViewTimeZoneId (const QString &timeZoneId) const |
void | shiftTimes (const KDateTime::Spec &oldSpec, const KDateTime::Spec &newSpec) |
KDateTime::Spec | timeSpec () const |
QString | timeZoneId () const |
ICalTimeZones * | timeZones () const |
virtual Todo * | todo (const QString &uid)=0 |
virtual Todo::List | todos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
virtual Todo::List | todos (const QDate &date) |
void | unregisterObserver (CalendarObserver *observer) |
KDateTime::Spec | viewTimeSpec () const |
QString | viewTimeZoneId () const |
![]() | |
CustomProperties () | |
CustomProperties (const CustomProperties &other) | |
virtual | ~CustomProperties () |
QMap< QByteArray, QString > | customProperties () const |
QString | customProperty (const QByteArray &app, const QByteArray &key) const |
QString | nonKDECustomProperty (const QByteArray &name) const |
CustomProperties & | operator= (const CustomProperties &other) |
bool | operator== (const CustomProperties &properties) const |
void | removeCustomProperty (const QByteArray &app, const QByteArray &key) |
void | removeNonKDECustomProperty (const QByteArray &name) |
void | setCustomProperties (const QMap< QByteArray, QString > &properties) |
void | setCustomProperty (const QByteArray &app, const QByteArray &key, const QString &value) |
void | setNonKDECustomProperty (const QByteArray &name, const QString &value) |
![]() | |
virtual | ~IncidenceObserver () |
Static Public Member Functions | |
static Incidence::List | mergeIncidenceList (const Event::List &events, const Todo::List &todos, const Journal::List &journals) |
static Event::List | sortEvents (Event::List *eventList, EventSortField sortField, SortDirection sortDirection) |
static Event::List | sortEventsForDate (Event::List *eventList, const QDate &date, const KDateTime::Spec &timeSpec, EventSortField sortField, SortDirection sortDirection) |
static Journal::List | sortJournals (Journal::List *journalList, JournalSortField sortField, SortDirection sortDirection) |
static Todo::List | sortTodos (Todo::List *todoList, TodoSortField sortField, SortDirection sortDirection) |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
Protected Member Functions | |
void | appendAlarms (Alarm::List &alarms, Incidence *incidence, const KDateTime &from, const KDateTime &to) |
void | appendRecurringAlarms (Alarm::List &alarms, Incidence *incidence, const KDateTime &from, const KDateTime &to) |
virtual void | customPropertyUpdated () |
virtual void | doSetTimeSpec (const KDateTime::Spec &timeSpec) |
void | incidenceUpdated (IncidenceBase *incidenceBase) |
void | notifyIncidenceAdded (Incidence *incidence) |
void | notifyIncidenceChanged (Incidence *incidence) |
void | notifyIncidenceDeleted (Incidence *incidence) |
void | setObserversEnabled (bool enabled) |
Detailed Description
Represents the main calendar class.
A calendar contains information like incidences (events, to-dos, journals), alarms, time zones, and other useful information.
This is an abstract base class defining the interface to a calendar. It is implemented by subclasses like CalendarLocal, which use different methods to store and access the data.
Ownership of Incidences:
Incidence ownership is handled by the following policy: as soon as an incidence (or any other subclass of IncidenceBase) is added to the Calendar by an add...() method it is owned by the Calendar object. The Calendar takes care of deleting the incidence using the delete...() methods. All Incidences returned by the query functions are returned as pointers so that changes to the returned Incidences are immediately visible in the Calendar. Do Not attempt to 'delete' any Incidence object you get from Calendar – use the delete...() methods.
Definition at line 119 of file calendar.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a calendar with a specified time zone timeZoneid
.
Private class that helps to provide binary compatibility between releases.
The time specification is used as the default for creating or modifying incidences in the Calendar. The time specification does not alter existing incidences.
The constructor also calls setViewTimeSpec(timeSpec
).
- Parameters
-
timeSpec time specification
Definition at line 106 of file calendar.cpp.
|
explicit |
Construct Calendar object using a time zone ID.
The time zone ID is used as the default for creating or modifying incidences in the Calendar. The time zone does not alter existing incidences.
The constructor also calls setViewTimeZoneId(timeZoneId
).
- Parameters
-
timeZoneId is a string containing a time zone ID, which is assumed to be valid. If no time zone is found, the viewing time specification is set to local clock time. Example: "Europe/Berlin"
Definition at line 113 of file calendar.cpp.
|
virtual |
Destroys the calendar.
Definition at line 119 of file calendar.cpp.
Member Function Documentation
|
pure virtual |
Inserts an Event into the calendar.
- Parameters
-
event is a pointer to the Event to insert.
- Returns
- true if the Event was successfully inserted; false otherwise.
- See also
- deleteEvent()
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
virtual |
Inserts an Incidence into the calendar.
- Parameters
-
incidence is a pointer to the Incidence to insert.
- Returns
- true if the Incidence was successfully inserted; false otherwise.
- See also
- deleteIncidence()
Reimplemented in KCal::CalendarResources.
Definition at line 573 of file calendar.cpp.
|
pure virtual |
Inserts a Journal into the calendar.
- Parameters
-
journal is a pointer to the Journal to insert.
- Returns
- true if the Journal was successfully inserted; false otherwise.
- See also
- deleteJournal()
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
pure virtual |
Inserts a Todo into the calendar.
- Parameters
-
todo is a pointer to the Todo to insert.
- Returns
- true if the Todo was successfully inserted; false otherwise.
- See also
- deleteTodo()
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
pure virtual |
Returns a list of Alarms within a time range for this Calendar.
- Parameters
-
from is the starting timestamp. to is the ending timestamp.
- Returns
- the list of Alarms for the for the specified time range.
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
protected |
Appends alarms of incidence in interval to list of alarms.
- Parameters
-
alarms is a List of Alarms to be appended onto. incidence is a pointer to an Incidence containing the Alarm to be appended. from is the lower range of the next Alarm repitition. to is the upper range of the next Alarm repitition.
Definition at line 1246 of file calendar.cpp.
|
protected |
Appends alarms of recurring events in interval to list of alarms.
- Parameters
-
alarms is a List of Alarms to be appended onto. incidence is a pointer to an Incidence containing the Alarm to be appended. from is the lower range of the next Alarm repitition. to is the upper range of the next Alarm repitition.
Definition at line 1263 of file calendar.cpp.
|
signal |
- See also
- beginBatchAdding()
- Since
- 4.4
|
signal |
- See also
- endBatchAdding()
- Since
- 4.4
void Calendar::beginBatchAdding | ( | ) |
Emits the beginBatchAdding() signal.
This should be called before adding a batch of incidences with addIncidence( Incidence *), addTodo( Todo *), addEvent( Event *) or addJournal( Journal *). Some Calendars are connected to this signal, e.g: CalendarResources uses it to know a series of incidenceAdds are related so the user isn't prompted multiple times which resource to save the incidence to
- Since
- 4.4
Definition at line 939 of file calendar.cpp.
|
virtual |
Flag that a change to a Calendar Incidence is starting.
- Parameters
-
incidence is a pointer to the Incidence that will be changing.
Reimplemented in KCal::CalendarResources.
Definition at line 1229 of file calendar.cpp.
|
signal |
Signals that the calendar has been modified.
|
signal |
Signals that the calendar has been loaded into memory.
|
signal |
Signals that the calendar has been saved.
QStringList Calendar::categories | ( | ) |
Returns a list of all categories used by Incidences in this Calendar.
- Returns
- a QStringList containing all the categories.
Definition at line 258 of file calendar.cpp.
|
pure virtual |
Clears out the current calendar, freeing all used memory etc.
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
protectedvirtual |
CustomProperties::customPropertyUpdated()
Reimplemented from KCal::CustomProperties.
Definition at line 1192 of file calendar.cpp.
|
pure virtual |
Removes all Events from the calendar.
- See also
- deleteEvent()
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
pure virtual |
Removes all Journals from the calendar.
- See also
- deleteJournal()
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
pure virtual |
Removes all To-dos from the calendar.
- See also
- deleteTodo()
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
pure virtual |
Removes an Event from the calendar.
- Parameters
-
event is a pointer to the Event to remove.
- Returns
- true if the Event was successfully remove; false otherwise.
- See also
- addEvent(), deleteAllEvents()
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
virtual |
Removes an Incidence from the calendar.
- Parameters
-
incidence is a pointer to the Incidence to remove.
- Returns
- true if the Incidence was successfully removed; false otherwise.
- See also
- addIncidence()
Definition at line 580 of file calendar.cpp.
|
pure virtual |
Removes a Journal from the calendar.
- Parameters
-
journal is a pointer to the Journal to remove.
- Returns
- true if the Journal was successfully removed; false otherwise.
- See also
- addJournal(), deleteAllJournals()
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
pure virtual |
Removes a Todo from the calendar.
- Parameters
-
todo is a pointer to the Todo to remove.
- Returns
- true if the Todo was successfully removed; false otherwise.
- See also
- addTodo(), deleteAllTodos()
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
Incidence * Calendar::dissociateOccurrence | ( | Incidence * | incidence, |
const QDate & | date, | ||
const KDateTime::Spec & | spec, | ||
bool | single = true |
||
) |
Dissociate an Incidence from a recurring Incidence.
By default, only one single Incidence for the specified date will be dissociated and returned. If single is false, then the recurrence will be split at date, the old Incidence will have its recurrence ending at date and the new Incidence will have all recurrences past the date.
- Parameters
-
incidence is a pointer to a recurring Incidence. date is the QDate within the recurring Incidence on which the dissociation will be performed. spec is the spec in which the date is formulated. single is a flag meaning that a new Incidence should be created from the recurring Incidences after date.
- Returns
- a pointer to a new recurring Incidence if single is false.
Definition at line 595 of file calendar.cpp.
|
protectedvirtual |
Let Calendar subclasses set the time specification.
- Parameters
-
timeSpec is the time specification (time zone, etc.) for viewing Incidence dates.
Reimplemented in KCal::CalendarResources.
Definition at line 1154 of file calendar.cpp.
void Calendar::endBatchAdding | ( | ) |
Emits the endBatchAdding() signal.
Used with beginBatchAdding(). Should be called after adding all incidences.
- Since
- 4.4
Definition at line 944 of file calendar.cpp.
|
virtual |
Flag that a change to a Calendar Incidence has completed.
- Parameters
-
incidence is a pointer to the Incidence that was changed.
Reimplemented in KCal::CalendarResources.
Definition at line 1235 of file calendar.cpp.
|
pure virtual |
Returns the Event associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string.
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
virtual |
Returns a sorted, filtered list of all Events for this Calendar.
- Parameters
-
sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all filtered Events sorted as specified.
Definition at line 565 of file calendar.cpp.
Event::List Calendar::events | ( | const KDateTime & | dt | ) |
Returns a filtered list of all Events which occur on the given timestamp.
- Parameters
-
dt request filtered Event list for this KDateTime only.
- Returns
- the list of filtered Events occurring on the specified timestamp.
Definition at line 549 of file calendar.cpp.
Event::List Calendar::events | ( | const QDate & | start, |
const QDate & | end, | ||
const KDateTime::Spec & | timeSpec = KDateTime::Spec() , |
||
bool | inclusive = false |
||
) |
Returns a filtered list of all Events occurring within a date range.
- Parameters
-
start is the starting date. end is the ending date. timeSpec time zone etc. to interpret start
andend
, or the calendar's default time spec if none is specifiedinclusive if true only Events which are completely included within the date range are returned.
- Returns
- the list of filtered Events occurring within the specified date range.
Definition at line 556 of file calendar.cpp.
Event::List Calendar::events | ( | const QDate & | date, |
const KDateTime::Spec & | timeSpec = KDateTime::Spec() , |
||
EventSortField | sortField = EventSortUnsorted , |
||
SortDirection | sortDirection = SortDirectionAscending |
||
) |
Returns a sorted, filtered list of all Events which occur on the given date.
The Events are sorted according to sortField and sortDirection.
- Parameters
-
date request filtered Event list for this QDate only. timeSpec time zone etc. to interpret start
andend
, or the calendar's default time spec if none is specifiedsortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of sorted, filtered Events occurring on date.
Definition at line 539 of file calendar.cpp.
CalFilter * Calendar::filter | ( | ) |
Incidence * Calendar::incidence | ( | const QString & | uid | ) |
Returns the Incidence associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string.
Definition at line 669 of file calendar.cpp.
Incidence * Calendar::incidenceFromSchedulingID | ( | const QString & | sid | ) |
Returns the Incidence associated with the given scheduling identifier.
- Parameters
-
sid is a unique scheduling identifier string.
Definition at line 698 of file calendar.cpp.
|
virtual |
Returns a filtered list of all Incidences for this Calendar.
- Returns
- the list of all filtered Incidences.
Definition at line 282 of file calendar.cpp.
|
virtual |
Returns a filtered list of all Incidences which occur on the given date.
- Parameters
-
date request filtered Incidence list for this QDate only.
- Returns
- the list of filtered Incidences occurring on the specified date.
Definition at line 277 of file calendar.cpp.
Incidence::List Calendar::incidencesFromSchedulingID | ( | const QString & | sid | ) |
Searches all events and todos for an incidence with this scheduling identifiere.
Returns a list of matching results.
- Parameters
-
sid is a unique scheduling identifier string.
Definition at line 685 of file calendar.cpp.
|
protectedvirtual |
The Observer interface.
So far not implemented.
- Parameters
-
incidenceBase is a pointer an IncidenceBase object.
Implements KCal::IncidenceBase::IncidenceObserver.
Reimplemented in KCal::CalendarLocal, and KCal::CalendarNull.
Definition at line 1141 of file calendar.cpp.
Checks if ancestor
is an ancestor of incidence
.
- Parameters
-
ancestor the incidence we are testing to be an ancestor incidence the incidence we are testing to be descended from ancestor
Definition at line 1075 of file calendar.cpp.
bool Calendar::isModified | ( | ) | const |
Determine the calendar's modification status.
- Returns
- true if the calendar has been modified since open or last save.
- See also
- setModified()
Definition at line 1136 of file calendar.cpp.
|
virtual |
Determine if the calendar is currently being saved.
- Returns
- true if the calendar is currently being saved; false otherwise.
Reimplemented in KCal::CalendarResources.
Definition at line 1120 of file calendar.cpp.
|
pure virtual |
Returns the Journal associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string.
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
virtual |
Returns a sorted, filtered list of all Journals for this Calendar.
- Parameters
-
sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all filtered Journals sorted as specified.
Definition at line 924 of file calendar.cpp.
|
virtual |
Returns a filtered list of all Journals for on the specified date.
- Parameters
-
date request filtered Journals for this QDate only.
- Returns
- the list of filtered Journals for the specified date.
Definition at line 932 of file calendar.cpp.
|
static |
Create a merged list of Events, Todos, and Journals.
- Parameters
-
events is an Event list to merge. todos is a Todo list to merge. journals is a Journal list to merge.
- Returns
- a list of merged Incidences.
Definition at line 1207 of file calendar.cpp.
|
protected |
Let Calendar subclasses notify that they inserted an Incidence.
- Parameters
-
incidence is a pointer to the Incidence object that was inserted.
Definition at line 1159 of file calendar.cpp.
|
protected |
Let Calendar subclasses notify that they modified an Incidence.
- Parameters
-
incidence is a pointer to the Incidence object that was modified.
Definition at line 1170 of file calendar.cpp.
|
protected |
Let Calendar subclasses notify that they removed an Incidence.
- Parameters
-
incidence is a pointer to the Incidence object that was removed.
Definition at line 1181 of file calendar.cpp.
Person Calendar::owner | ( | ) | const |
Returns the owner of the calendar.
- Returns
- the owner Person object.
- See also
- setOwner()
Definition at line 124 of file calendar.cpp.
QString Calendar::productId | ( | ) | const |
Returns the calendar's Product ID.
- See also
- setProductId()
Definition at line 1202 of file calendar.cpp.
|
pure virtual |
Returns a sorted, unfiltered list of all Events for this Calendar.
- Parameters
-
sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered Events sorted as specified.
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
pure virtual |
Returns an unfiltered list of all Events occurring within a date range.
- Parameters
-
start is the starting date end is the ending date timeSpec time zone etc. to interpret start
andend
, or the calendar's default time spec if none is specifiedinclusive if true only Events which are completely included within the date range are returned.
- Returns
- the list of unfiltered Events occurring within the specified date range.
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
pure virtual |
Returns an unfiltered list of all Events which occur on the given timestamp.
- Parameters
-
dt request unfiltered Event list for this KDateTime only.
- Returns
- the list of unfiltered Events occurring on the specified timestamp.
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
pure virtual |
Returns a sorted, unfiltered list of all Events which occur on the given date.
The Events are sorted according to sortField and sortDirection.
- Parameters
-
date request unfiltered Event list for this QDate only timeSpec time zone etc. to interpret date
, or the calendar's default time spec if none is specifiedsortField specifies the EventSortField sortDirection specifies the SortDirection
- Returns
- the list of sorted, unfiltered Events occurring on
date
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
virtual |
Returns an unfiltered list of all Incidences for this Calendar.
- Returns
- the list of all unfiltered Incidences.
Definition at line 287 of file calendar.cpp.
|
pure virtual |
Returns a sorted, unfiltered list of all Journals for this Calendar.
- Parameters
-
sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered Journals sorted as specified.
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
pure virtual |
Returns an unfiltered list of all Journals for on the specified date.
- Parameters
-
date request unfiltered Journals for this QDate only.
- Returns
- the list of unfiltered Journals for the specified date.
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
pure virtual |
Returns a sorted, unfiltered list of all Todos for this Calendar.
- Parameters
-
sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all unfiltered Todos sorted as specified.
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
pure virtual |
Returns an unfiltered list of all Todos which due on the specified date.
- Parameters
-
date request unfiltered Todos due on this QDate.
- Returns
- the list of unfiltered Todos due on the specified date.
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
void Calendar::registerObserver | ( | CalendarObserver * | observer | ) |
Registers an Observer for this Calendar.
- Parameters
-
observer is a pointer to an Observer object that will be watching this Calendar.
- See also
- unregisterObserver()
Definition at line 1107 of file calendar.cpp.
|
pure virtual |
Loads the calendar contents from storage.
This requires that the calendar has been previously loaded (initialized).
- Returns
- true if the reload was successful; otherwise false.
Implemented in KCal::CalendarResources, KCal::CalendarNull, and KCal::CalendarLocal.
|
virtual |
Removes all Relations from an Incidence.
- Parameters
-
incidence is a pointer to the Incidence to have a Relation removed.
Definition at line 997 of file calendar.cpp.
|
pure virtual |
Syncs changes in memory to persistent storage.
- Returns
- true if the save was successful; false otherwise.
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
void Calendar::setFilter | ( | CalFilter * | filter | ) |
void Calendar::setModified | ( | bool | modified | ) |
Sets if the calendar has been modified.
- Parameters
-
modified is true if the calendar has been modified since open or last save.
- See also
- isModified()
Definition at line 1125 of file calendar.cpp.
|
protected |
Let Calendar subclasses notify that they enabled an Observer.
- Parameters
-
enabled if true tells the calendar that a subclass has enabled an Observer.
Definition at line 1241 of file calendar.cpp.
void Calendar::setOwner | ( | const Person & | owner | ) |
Sets the owner of the calendar to owner
.
- Parameters
-
owner is a Person object.
- See also
- owner()
Definition at line 129 of file calendar.cpp.
void Calendar::setProductId | ( | const QString & | id | ) |
Sets the calendar Product ID to id
.
- Parameters
-
id is a string containing the Product ID.
- See also
- productId() const
Definition at line 1197 of file calendar.cpp.
void Calendar::setTimeSpec | ( | const KDateTime::Spec & | timeSpec | ) |
Sets the default time specification (time zone, etc.) used for creating or modifying incidences in the Calendar.
The method also calls setViewTimeSpec(timeSpec
).
- Parameters
-
timeSpec time specification
Definition at line 136 of file calendar.cpp.
void Calendar::setTimeZoneId | ( | const QString & | timeZoneId | ) |
Sets the time zone ID used for creating or modifying incidences in the Calendar.
This method has no effect on existing incidences.
The method also calls setViewTimeZoneId(timeZoneId
).
- Parameters
-
timeZoneId is a string containing a time zone ID, which is assumed to be valid. The time zone ID is used to set the time zone for viewing Incidence date/times. If no time zone is found, the viewing time specification is set to local clock time. Example: "Europe/Berlin"
- See also
- setTimeSpec()
Definition at line 150 of file calendar.cpp.
void KCal::Calendar::setTimeZones | ( | const ICalTimeZones & | zones | ) |
Set the time zone collection used by the calendar.
- Parameters
-
zones time zones collection. Important: all time zones references in the calendar must be included in the collection.
|
virtual |
Setup Relations for an Incidence.
- Parameters
-
incidence is a pointer to the Incidence to have a Relation setup.
Definition at line 951 of file calendar.cpp.
void Calendar::setViewTimeSpec | ( | const KDateTime::Spec & | timeSpec | ) | const |
Notes the time specification which the client application intends to use for viewing the incidences in this calendar.
This is simply a convenience method which makes a note of the new time zone so that it can be read back by viewTimeSpec(). The client application must convert date/time values to the desired time zone itself.
The time specification is not used in any way by the Calendar or its incidences; it is solely for use by the client application.
- Parameters
-
timeSpec time specification
- See also
- viewTimeSpec()
Definition at line 195 of file calendar.cpp.
void Calendar::setViewTimeZoneId | ( | const QString & | timeZoneId | ) | const |
Notes the time zone Id which the client application intends to use for viewing the incidences in this calendar.
This is simply a convenience method which makes a note of the new time zone so that it can be read back by viewTimeId(). The client application must convert date/time values to the desired time zone itself.
The Id is not used in any way by the Calendar or its incidences. It is solely for use by the client application.
- Parameters
-
timeZoneId is a string containing a time zone ID, which is assumed to be valid. The time zone ID is used to set the time zone for viewing Incidence date/times. If no time zone is found, the viewing time specification is set to local clock time. Example: "Europe/Berlin"
- See also
- viewTimeZoneId()
Definition at line 201 of file calendar.cpp.
void Calendar::shiftTimes | ( | const KDateTime::Spec & | oldSpec, |
const KDateTime::Spec & | newSpec | ||
) |
Shifts the times of all incidences so that they appear at the same clock time as before but in a new time zone.
The shift is done from a viewing time zone rather than from the actual incidence time zone.
For example, shifting an incidence whose start time is 09:00 America/New York, using an old viewing time zone (oldSpec
) of Europe/London, to a new time zone (newSpec
) of Europe/Paris, will result in the time being shifted from 14:00 (which is the London time of the incidence start) to 14:00 Paris time.
- Parameters
-
oldSpec the time specification which provides the clock times newSpec the new time specification
- See also
- isLocalTime()
Definition at line 222 of file calendar.cpp.
|
static |
Sort a list of Events.
- Parameters
-
eventList is a pointer to a list of Events. sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- a list of Events sorted as specified.
Definition at line 292 of file calendar.cpp.
|
static |
Sort a list of Events that occur on a specified date.
- Parameters
-
eventList is a pointer to a list of Events occurring on date
.date is the date. timeSpec time specification for date
.sortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- a list of Events sorted as specified.
- Since
- 4.5
Definition at line 395 of file calendar.cpp.
|
static |
Sort a list of Journals.
- Parameters
-
journalList is a pointer to a list of Journals. sortField specifies the JournalSortField. sortDirection specifies the SortDirection.
- Returns
- a list of Journals sorted as specified.
Definition at line 871 of file calendar.cpp.
|
static |
Sort a list of Todos.
- Parameters
-
todoList is a pointer to a list of Todos. sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns
- a list of Todos sorted as specified.
Definition at line 712 of file calendar.cpp.
KDateTime::Spec Calendar::timeSpec | ( | ) | const |
Get the time specification (time zone etc.) used for creating or modifying incidences in the Calendar.
- Returns
- time specification
Definition at line 145 of file calendar.cpp.
QString Calendar::timeZoneId | ( | ) | const |
Returns the time zone ID used for creating or modifying incidences in the calendar.
- Returns
- the string containing the time zone ID, or empty string if the creation/modification time specification is not a time zone.
Definition at line 189 of file calendar.cpp.
ICalTimeZones * Calendar::timeZones | ( | ) | const |
Returns the time zone collection used by the calendar.
- Returns
- the time zones collection.
- See also
- setLocalTime()
Definition at line 217 of file calendar.cpp.
|
pure virtual |
Returns the Todo associated with the given unique identifier.
- Parameters
-
uid is a unique identifier string.
Implemented in KCal::CalendarResources, KCal::CalendarLocal, and KCal::CalendarNull.
|
virtual |
Returns a sorted, filtered list of all Todos for this Calendar.
- Parameters
-
sortField specifies the TodoSortField. sortDirection specifies the SortDirection.
- Returns
- the list of all filtered Todos sorted as specified.
Definition at line 856 of file calendar.cpp.
|
virtual |
Returns a filtered list of all Todos which are due on the specified date.
- Parameters
-
date request filtered Todos due on this QDate.
- Returns
- the list of filtered Todos due on the specified date.
Definition at line 864 of file calendar.cpp.
void Calendar::unregisterObserver | ( | CalendarObserver * | observer | ) |
Unregisters an Observer for this Calendar.
- Parameters
-
observer is a pointer to an Observer object that has been watching this Calendar.
- See also
- registerObserver()
Definition at line 1115 of file calendar.cpp.
KDateTime::Spec Calendar::viewTimeSpec | ( | ) | const |
Returns the time specification used for viewing the incidences in this calendar.
This simply returns the time specification last set by setViewTimeSpec().
- See also
- setViewTimeSpec().
Definition at line 206 of file calendar.cpp.
QString Calendar::viewTimeZoneId | ( | ) | const |
Returns the time zone Id used for viewing the incidences in this calendar.
This simply returns the time specification last set by setViewTimeSpec().
- See also
- setViewTimeZoneId().
Definition at line 211 of file calendar.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:00:58 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.