KCal Library
#include <calendarlocal.h>
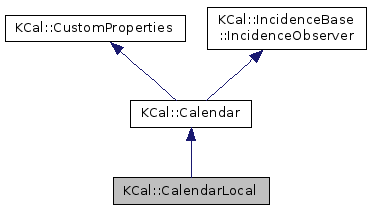
Public Member Functions | |
CalendarLocal (const KDateTime::Spec &timeSpec) | |
CalendarLocal (const QString &timeZoneId) | |
~CalendarLocal () | |
bool | addEvent (Event *event) |
bool | addJournal (Journal *journal) |
bool | addTodo (Todo *todo) |
Alarm::List | alarms (const KDateTime &from, const KDateTime &to) |
Alarm::List | alarmsTo (const KDateTime &to) |
void | close () |
void | deleteAllEvents () |
void | deleteAllJournals () |
void | deleteAllTodos () |
bool | deleteEvent (Event *event) |
bool | deleteJournal (Journal *journal) |
bool | deleteTodo (Todo *todo) |
Event * | event (const QString &uid) |
void | incidenceUpdated (IncidenceBase *incidenceBase) |
Journal * | journal (const QString &uid) |
bool | load (const QString &fileName, CalFormat *format=0) |
Event::List | rawEvents (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Event::List | rawEvents (const QDate &start, const QDate &end, const KDateTime::Spec &timeSpec=KDateTime::Spec(), bool inclusive=false) |
Event::List | rawEventsForDate (const QDate &date, const KDateTime::Spec &timeSpec=KDateTime::Spec(), EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Event::List | rawEventsForDate (const KDateTime &dt) |
Journal::List | rawJournals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Journal::List | rawJournalsForDate (const QDate &date) |
Todo::List | rawTodos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Todo::List | rawTodosForDate (const QDate &date) |
bool | reload () |
bool | save () |
bool | save (const QString &fileName, CalFormat *format=0) |
Todo * | todo (const QString &uid) |
![]() | |
Calendar (const KDateTime::Spec &timeSpec) | |
Calendar (const QString &timeZoneId) | |
virtual | ~Calendar () |
virtual bool | addIncidence (Incidence *incidence) |
void | beginBatchAdding () |
virtual bool | beginChange (Incidence *incidence) |
QStringList | categories () |
virtual bool | deleteIncidence (Incidence *incidence) |
Incidence * | dissociateOccurrence (Incidence *incidence, const QDate &date, const KDateTime::Spec &spec, bool single=true) |
void | endBatchAdding () |
virtual bool | endChange (Incidence *incidence) |
virtual Event::List | events (EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
Event::List | events (const KDateTime &dt) |
Event::List | events (const QDate &start, const QDate &end, const KDateTime::Spec &timeSpec=KDateTime::Spec(), bool inclusive=false) |
Event::List | events (const QDate &date, const KDateTime::Spec &timeSpec=KDateTime::Spec(), EventSortField sortField=EventSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
CalFilter * | filter () |
Incidence * | incidence (const QString &uid) |
Incidence * | incidenceFromSchedulingID (const QString &sid) |
virtual Incidence::List | incidences () |
virtual Incidence::List | incidences (const QDate &date) |
Incidence::List | incidencesFromSchedulingID (const QString &sid) |
bool | isAncestorOf (Incidence *ancestor, Incidence *incidence) |
bool | isModified () const |
virtual bool | isSaving () |
virtual Journal::List | journals (JournalSortField sortField=JournalSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
virtual Journal::List | journals (const QDate &date) |
Person | owner () const |
QString | productId () const |
virtual Incidence::List | rawIncidences () |
void | registerObserver (CalendarObserver *observer) |
virtual void | removeRelations (Incidence *incidence) |
void | setFilter (CalFilter *filter) |
void | setModified (bool modified) |
void | setOwner (const Person &owner) |
void | setProductId (const QString &id) |
void | setTimeSpec (const KDateTime::Spec &timeSpec) |
void | setTimeZoneId (const QString &timeZoneId) |
void | setTimeZones (const ICalTimeZones &zones) |
virtual void | setupRelations (Incidence *incidence) |
void | setViewTimeSpec (const KDateTime::Spec &timeSpec) const |
void | setViewTimeZoneId (const QString &timeZoneId) const |
void | shiftTimes (const KDateTime::Spec &oldSpec, const KDateTime::Spec &newSpec) |
KDateTime::Spec | timeSpec () const |
QString | timeZoneId () const |
ICalTimeZones * | timeZones () const |
virtual Todo::List | todos (TodoSortField sortField=TodoSortUnsorted, SortDirection sortDirection=SortDirectionAscending) |
virtual Todo::List | todos (const QDate &date) |
void | unregisterObserver (CalendarObserver *observer) |
KDateTime::Spec | viewTimeSpec () const |
QString | viewTimeZoneId () const |
![]() | |
CustomProperties () | |
CustomProperties (const CustomProperties &other) | |
virtual | ~CustomProperties () |
QMap< QByteArray, QString > | customProperties () const |
QString | customProperty (const QByteArray &app, const QByteArray &key) const |
QString | nonKDECustomProperty (const QByteArray &name) const |
CustomProperties & | operator= (const CustomProperties &other) |
bool | operator== (const CustomProperties &properties) const |
void | removeCustomProperty (const QByteArray &app, const QByteArray &key) |
void | removeNonKDECustomProperty (const QByteArray &name) |
void | setCustomProperties (const QMap< QByteArray, QString > &properties) |
void | setCustomProperty (const QByteArray &app, const QByteArray &key, const QString &value) |
void | setNonKDECustomProperty (const QByteArray &name, const QString &value) |
![]() | |
virtual | ~IncidenceObserver () |
Additional Inherited Members | |
![]() | |
void | batchAddingBegins () |
void | batchAddingEnds () |
void | calendarChanged () |
void | calendarLoaded () |
void | calendarSaved () |
![]() | |
static Incidence::List | mergeIncidenceList (const Event::List &events, const Todo::List &todos, const Journal::List &journals) |
static Event::List | sortEvents (Event::List *eventList, EventSortField sortField, SortDirection sortDirection) |
static Event::List | sortEventsForDate (Event::List *eventList, const QDate &date, const KDateTime::Spec &timeSpec, EventSortField sortField, SortDirection sortDirection) |
static Journal::List | sortJournals (Journal::List *journalList, JournalSortField sortField, SortDirection sortDirection) |
static Todo::List | sortTodos (Todo::List *todoList, TodoSortField sortField, SortDirection sortDirection) |
![]() | |
static QByteArray | customPropertyName (const QByteArray &app, const QByteArray &key) |
![]() | |
void | appendAlarms (Alarm::List &alarms, Incidence *incidence, const KDateTime &from, const KDateTime &to) |
void | appendRecurringAlarms (Alarm::List &alarms, Incidence *incidence, const KDateTime &from, const KDateTime &to) |
virtual void | customPropertyUpdated () |
virtual void | doSetTimeSpec (const KDateTime::Spec &timeSpec) |
void | notifyIncidenceAdded (Incidence *incidence) |
void | notifyIncidenceChanged (Incidence *incidence) |
void | notifyIncidenceDeleted (Incidence *incidence) |
void | setObserversEnabled (bool enabled) |
Detailed Description
This class provides a calendar stored as a local file.
Definition at line 43 of file calendarlocal.h.
Constructor & Destructor Documentation
|
explicit |
Private class that helps to provide binary compatibility between releases.
Calendar::Calendar(const KDateTime::Spec &)
Definition at line 97 of file calendarlocal.cpp.
|
explicit |
Calendar::Calendar(const QString &)
Definition at line 103 of file calendarlocal.cpp.
CalendarLocal::~CalendarLocal | ( | ) |
Definition at line 109 of file calendarlocal.cpp.
Member Function Documentation
|
virtual |
|
virtual |
|
virtual |
|
virtual |
Alarm::List CalendarLocal::alarmsTo | ( | const KDateTime & | to | ) |
Return a list of Alarms that occur before the specified timestamp.
- Parameters
-
to is the ending timestamp.
- Returns
- the list of Alarms occurring before the specified KDateTime.
Definition at line 324 of file calendarlocal.cpp.
|
virtual |
Clears out the current calendar, freeing all used memory etc.
etc.
Implements KCal::Calendar.
Definition at line 158 of file calendarlocal.cpp.
|
virtual |
Implements KCal::Calendar.
Definition at line 202 of file calendarlocal.cpp.
|
virtual |
Implements KCal::Calendar.
Definition at line 597 of file calendarlocal.cpp.
|
virtual |
Implements KCal::Calendar.
Definition at line 277 of file calendarlocal.cpp.
|
virtual |
Implements KCal::Calendar.
Definition at line 185 of file calendarlocal.cpp.
|
virtual |
Implements KCal::Calendar.
Definition at line 583 of file calendarlocal.cpp.
|
virtual |
|
virtual |
|
virtual |
Notify the IncidenceBase::Observer that the incidence has been updated.
- Parameters
-
incidenceBase is a pointer to the updated IncidenceBase.
Reimplemented from KCal::Calendar.
Definition at line 380 of file calendarlocal.cpp.
|
virtual |
bool CalendarLocal::load | ( | const QString & | fileName, |
CalFormat * | format = 0 |
||
) |
Loads a calendar on disk in vCalendar or iCalendar format into the current calendar.
Incidences already present are preserved. If an incidence of the file to be loaded has the same unique id as an incidence already present the new incidence is ignored.
To load a CalendarLocal object from a file without preserving existing incidences call close() before load().
- Parameters
-
fileName the name of the calendar on disk. format the format to use. If 0, an attempt is made to load iCalendar format, and if that fails tries vCalendar format.
- Returns
- true, if successful, false on error.
Definition at line 115 of file calendarlocal.cpp.
|
virtual |
Calendar::rawEvents(EventSortField, SortDirection)
Implements KCal::Calendar.
Definition at line 541 of file calendarlocal.cpp.
|
virtual |
Calendar::rawEvents(const QDate &, const QDate &, const KDateTime::Spec &, bool)
Implements KCal::Calendar.
Definition at line 476 of file calendarlocal.cpp.
|
virtual |
Returns an unfiltered list of all Events which occur on the given date.
- Parameters
-
date request unfiltered Event list for this QDate only. timeSpec time zone etc. to interpret date
, or the calendar's default time spec if none is specifiedsortField specifies the EventSortField. sortDirection specifies the SortDirection.
- Returns
- the list of unfiltered Events occurring on the specified QDate.
Implements KCal::Calendar.
Definition at line 417 of file calendarlocal.cpp.
|
virtual |
Calendar::rawEventsForDate(const KDateTime &)
Implements KCal::Calendar.
Definition at line 536 of file calendarlocal.cpp.
|
virtual |
Implements KCal::Calendar.
Definition at line 617 of file calendarlocal.cpp.
|
virtual |
Calendar::rawJournalsForDate()
Implements KCal::Calendar.
Definition at line 629 of file calendarlocal.cpp.
|
virtual |
|
virtual |
Implements KCal::Calendar.
Definition at line 309 of file calendarlocal.cpp.
|
virtual |
Reloads the contents of the storage into memory.
The associated file name must be known, in other words a previous load() must have been executed.
- Returns
- true if the reload was successful; false otherwise.
Implements KCal::Calendar.
Definition at line 122 of file calendarlocal.cpp.
|
virtual |
Writes the calendar to disk.
The associated file name and format must be known, in other words a previous load() must have been executed.
- Returns
- true if the save was successful; false otherwise.
Implements KCal::Calendar.
Definition at line 132 of file calendarlocal.cpp.
bool CalendarLocal::save | ( | const QString & | fileName, |
CalFormat * | format = 0 |
||
) |
Writes the calendar to disk in the specified format
.
CalendarLocal takes ownership of the CalFormat object.
- Parameters
-
fileName the name of the file format the format to use. If 0, iCalendar will be used.
- Returns
- true if the save was successful; false otherwise.
Definition at line 146 of file calendarlocal.cpp.
|
virtual |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:00:58 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.