liblancelot
#include <ActionListView.h>
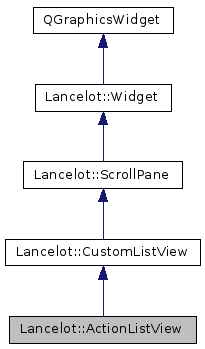
Classes | |
class | Private |
Public Types | |
enum | ItemDisplayMode { Standard = 0, DescriptionFirst = 1, SingleLineNameFirst = 2, SingleLineDescriptionFirst = 3, OnlyName = 4, OnlyDescription = 5 } |
![]() | |
enum | Flag { ClipScrollable = 1, HoverShowScrollbars = 2 } |
Public Slots | |
void | clearSelection () |
void | hide () |
void | initialSelection () |
void | show () |
![]() | |
void | scrollableWidgetSizeUpdateNeeded () |
void | scrollHorizontal (int value) |
void | scrollVertical (int value) |
Signals | |
void | activated (int index) |
![]() | |
void | clicked () |
void | mouseHoverEnter () |
void | mouseHoverLeave () |
void | pressed () |
void | released () |
Public Member Functions | |
ActionListView (QGraphicsItem *parent=0) | |
ActionListView (ActionListModel *model, QGraphicsItem *parent=0) | |
virtual | ~ActionListView () |
bool | areCategoriesActivable () const |
Group * | categoriesGroup () const |
int | categoryHeight (Qt::SizeHint which) const |
QSize | categoryIconSize () const |
ItemDisplayMode | displayMode () const |
int | extenderPosition () const |
int | itemHeight (Qt::SizeHint which) const |
QSize | itemIconSize () const |
Group * | itemsGroup () const |
L_Override void | keyPressEvent (QKeyEvent *event) |
ActionListModel * | model () const |
int | selectedIndex () const |
void | setCategoriesActivable (bool value) |
void | setCategoriesGroup (Group *group=NULL) |
void | setCategoriesGroupByName (const QString &group) |
void | setCategoryHeight (int height, Qt::SizeHint which) |
void | setCategoryIconSize (QSize size) |
void | setDisplayMode (ItemDisplayMode mode) |
void | setExtenderPosition (int position) |
void | setItemHeight (int height, Qt::SizeHint which) |
void | setItemIconSize (QSize size) |
void | setItemsGroup (Group *group=NULL) |
void | setItemsGroupByName (const QString &group) |
void | setModel (ActionListModel *model) |
void | setShowsExtendersOutside (bool value) |
bool | showsExtendersOutside () const |
![]() | |
CustomListView (QGraphicsItem *parent=NULL) | |
CustomListView (CustomListItemFactory *factory, QGraphicsItem *parent=NULL) | |
virtual | ~CustomListView () |
CustomList * | list () const |
![]() | |
ScrollPane (QGraphicsItem *parent=0) | |
virtual | ~ScrollPane () |
void | clearFlag (Flag flag) |
QSizeF | contentsSize () const |
QSizeF | currentViewportSize () const |
Flags | flags () const |
QSizeF | maximumViewportSize () const |
QPointF | scrollPosition () const |
void | scrollTo (QRectF rect) |
void | setFlag (Flag flag) |
void | setFlags (Flags flags) |
void | setFlip (Plasma::Flip flip) |
void | setScrollableWidget (Scrollable *widget) |
void | setScrollPosition (const QPointF &position) |
QRectF | viewportGeometry () const |
![]() | |
Widget (QGraphicsItem *parent=0) | |
virtual | ~Widget () |
Group * | group () const |
QString | groupName () const |
bool | isDown () const |
bool | isHovered () const |
virtual void | setGroup (Group *group=NULL) |
virtual void | setGroupByName (const QString &groupName) |
Protected Member Functions | |
L_Override void | hoverLeaveEvent (QGraphicsSceneHoverEvent *event) |
L_Override void | resizeEvent (QGraphicsSceneResizeEvent *event) |
L_Override bool | sceneEvent (QEvent *event) |
L_Override bool | sceneEventFilter (QGraphicsItem *watched, QEvent *event) |
L_Override QSizeF | sizeHint (Qt::SizeHint which, const QSizeF &constraint=QSizeF()) const |
![]() | |
L_Override QSizeF | sizeHint (Qt::SizeHint which, const QSizeF &constraint=QSizeF()) const |
![]() | |
L_Override void | hoverEnterEvent (QGraphicsSceneHoverEvent *event) |
L_Override void | hoverLeaveEvent (QGraphicsSceneHoverEvent *event) |
L_Override void | resizeEvent (QGraphicsSceneResizeEvent *event) |
![]() | |
L_Override void | hideEvent (QHideEvent *event) |
L_Override void | hoverEnterEvent (QGraphicsSceneHoverEvent *event) |
L_Override void | hoverLeaveEvent (QGraphicsSceneHoverEvent *event) |
L_Override void | mousePressEvent (QGraphicsSceneMouseEvent *event) |
L_Override void | mouseReleaseEvent (QGraphicsSceneMouseEvent *event) |
L_Override void | paint (QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget=0) |
void | paintBackground (QPainter *painter) |
void | paintBackground (QPainter *painter, const QString &element) |
void | setDown (bool value) |
void | setHovered (bool value) |
void | setPaintBackwardsWhenRTL (bool value) |
L_Override QSizeF | sizeHint (Qt::SizeHint which, const QSizeF &constraint=QSizeF()) const |
Properties | |
bool | categoriesActivable |
int | extenderPosition |
int | selectedIndex |
bool | showsExtendersOutside |
![]() | |
QSizeF | contentsSize |
QPointF | scrollPosition |
QRectF | viewportGeometry |
![]() | |
bool | down |
QString | group |
Detailed Description
Definition at line 33 of file ActionListView.h.
Member Enumeration Documentation
Enumerator | |
---|---|
Standard | |
DescriptionFirst | |
SingleLineNameFirst | |
SingleLineDescriptionFirst | |
OnlyName | |
OnlyDescription |
Definition at line 48 of file ActionListView.h.
Constructor & Destructor Documentation
Lancelot::ActionListView::ActionListView | ( | QGraphicsItem * | parent = 0 | ) |
Definition at line 822 of file ActionListView.cpp.
|
explicit |
Definition at line 828 of file ActionListView.cpp.
|
virtual |
Definition at line 905 of file ActionListView.cpp.
Member Function Documentation
|
signal |
bool Lancelot::ActionListView::areCategoriesActivable | ( | ) | const |
Definition at line 1163 of file ActionListView.cpp.
Group * Lancelot::ActionListView::categoriesGroup | ( | ) | const |
Definition at line 1112 of file ActionListView.cpp.
int Lancelot::ActionListView::categoryHeight | ( | Qt::SizeHint | which | ) | const |
Definition at line 962 of file ActionListView.cpp.
QSize Lancelot::ActionListView::categoryIconSize | ( | ) | const |
Definition at line 994 of file ActionListView.cpp.
|
slot |
Definition at line 1143 of file ActionListView.cpp.
ActionListView::ItemDisplayMode Lancelot::ActionListView::displayMode | ( | ) | const |
Definition at line 1066 of file ActionListView.cpp.
int Lancelot::ActionListView::extenderPosition | ( | ) | const |
|
slot |
Definition at line 1225 of file ActionListView.cpp.
|
protected |
Definition at line 1211 of file ActionListView.cpp.
|
slot |
Definition at line 1148 of file ActionListView.cpp.
int Lancelot::ActionListView::itemHeight | ( | Qt::SizeHint | which | ) | const |
Definition at line 946 of file ActionListView.cpp.
QSize Lancelot::ActionListView::itemIconSize | ( | ) | const |
Definition at line 978 of file ActionListView.cpp.
Group * Lancelot::ActionListView::itemsGroup | ( | ) | const |
Definition at line 1089 of file ActionListView.cpp.
void Lancelot::ActionListView::keyPressEvent | ( | QKeyEvent * | event | ) |
Definition at line 1121 of file ActionListView.cpp.
ActionListModel * Lancelot::ActionListView::model | ( | ) | const |
Definition at line 930 of file ActionListView.cpp.
|
protected |
Definition at line 1202 of file ActionListView.cpp.
|
protected |
Definition at line 845 of file ActionListView.cpp.
|
protected |
Definition at line 839 of file ActionListView.cpp.
int Lancelot::ActionListView::selectedIndex | ( | ) | const |
void Lancelot::ActionListView::setCategoriesActivable | ( | bool | value | ) |
Definition at line 1168 of file ActionListView.cpp.
void Lancelot::ActionListView::setCategoriesGroup | ( | Group * | group = NULL | ) |
Definition at line 1098 of file ActionListView.cpp.
void Lancelot::ActionListView::setCategoriesGroupByName | ( | const QString & | group | ) |
Definition at line 1107 of file ActionListView.cpp.
void Lancelot::ActionListView::setCategoryHeight | ( | int | height, |
Qt::SizeHint | which | ||
) |
Definition at line 954 of file ActionListView.cpp.
void Lancelot::ActionListView::setCategoryIconSize | ( | QSize | size | ) |
Definition at line 986 of file ActionListView.cpp.
void Lancelot::ActionListView::setDisplayMode | ( | ActionListView::ItemDisplayMode | mode | ) |
Definition at line 1057 of file ActionListView.cpp.
void Lancelot::ActionListView::setExtenderPosition | ( | int | position | ) |
Definition at line 1024 of file ActionListView.cpp.
void Lancelot::ActionListView::setItemHeight | ( | int | height, |
Qt::SizeHint | which | ||
) |
Definition at line 938 of file ActionListView.cpp.
void Lancelot::ActionListView::setItemIconSize | ( | QSize | size | ) |
Definition at line 970 of file ActionListView.cpp.
void Lancelot::ActionListView::setItemsGroup | ( | Group * | group = NULL | ) |
Definition at line 1075 of file ActionListView.cpp.
void Lancelot::ActionListView::setItemsGroupByName | ( | const QString & | group | ) |
Definition at line 1084 of file ActionListView.cpp.
void Lancelot::ActionListView::setModel | ( | ActionListModel * | model | ) |
Definition at line 910 of file ActionListView.cpp.
void Lancelot::ActionListView::setShowsExtendersOutside | ( | bool | value | ) |
Definition at line 1002 of file ActionListView.cpp.
|
slot |
Definition at line 1220 of file ActionListView.cpp.
bool Lancelot::ActionListView::showsExtendersOutside | ( | ) | const |
|
protected |
Definition at line 1174 of file ActionListView.cpp.
Property Documentation
|
readwrite |
Definition at line 38 of file ActionListView.h.
|
readwrite |
Definition at line 37 of file ActionListView.h.
|
read |
Definition at line 40 of file ActionListView.h.
|
readwrite |
Definition at line 39 of file ActionListView.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Mon Oct 13 2014 22:55:07 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.