liblancelot
#include <ExtenderButton.h>
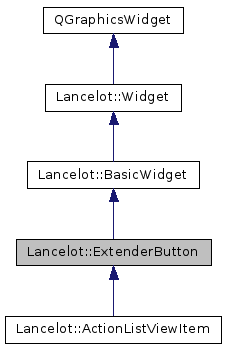
Public Slots | |
void | setChecked (bool checked) |
void | toggle () |
Signals | |
void | activated () |
void | toggled (bool checked) |
![]() | |
void | clicked () |
void | mouseHoverEnter () |
void | mouseHoverLeave () |
void | pressed () |
void | released () |
Public Member Functions | |
ExtenderButton (QGraphicsItem *parent=0) | |
ExtenderButton (QString title, QString description=QString(), QGraphicsItem *parent=0) | |
ExtenderButton (QIcon icon, QString title=QString(), QString description=QString(), QGraphicsItem *parent=0) | |
ExtenderButton (const Plasma::Svg &icon, QString title=QString(), QString description=QString(), QGraphicsItem *parent=0) | |
virtual | ~ExtenderButton () |
int | activationMethod () const |
L_Override QRectF | boundingRect () const |
int | extenderPosition () const |
bool | isCheckable () const |
bool | isChecked () const |
L_Override void | paint (QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget=0) |
void | setActivationMethod (int method) |
void | setCheckable (bool checkable) |
void | setExtenderPosition (int position) |
L_Override void | setGroup (Group *group=NULL) |
L_Override void | setShortcutKey (const QString &key) |
![]() | |
BasicWidget (QIcon icon, QString title=QString(), QString description=QString(), QGraphicsItem *parent=0) | |
BasicWidget (const Plasma::Svg &icon, QString title=QString(), QString description=QString(), QGraphicsItem *parent=0) | |
virtual | ~BasicWidget () |
Qt::Alignment | alignment () const |
QString | description () const |
QIcon | icon () const |
Plasma::Svg & | iconInSvg () const |
QSize | iconSize () const |
Qt::Orientation | innerOrientation () const |
void | setAlignment (Qt::Alignment alignment) |
void | setDescription (const QString &description) |
void | setIcon (QIcon icon) |
void | setIconInSvg (const Plasma::Svg &svg) |
void | setIconSize (QSize size) |
void | setInnerOrientation (Qt::Orientation orientation) |
void | setTitle (const QString &title) |
QString | title () const |
![]() | |
Widget (QGraphicsItem *parent=0) | |
virtual | ~Widget () |
Group * | group () const |
QString | groupName () const |
bool | isDown () const |
bool | isHovered () const |
virtual void | setGroupByName (const QString &groupName) |
Protected Slots | |
void | activate () |
Protected Member Functions | |
L_Override void | hideEvent (QHideEvent *event) |
L_Override void | hoverEnterEvent (QGraphicsSceneHoverEvent *event) |
L_Override void | hoverLeaveEvent (QGraphicsSceneHoverEvent *event) |
L_Override void | resizeEvent (QGraphicsSceneResizeEvent *event) |
![]() | |
void | drawText (QPainter *painter, const QRectF &rectangle, int flags, const QString &text, bool shortcutEnabled) |
L_Override void | paint (QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget=0) |
void | paintForeground (QPainter *painter) |
L_Override QSizeF | sizeHint (Qt::SizeHint which, const QSizeF &constraint=QSizeF()) const |
![]() | |
L_Override void | hideEvent (QHideEvent *event) |
L_Override void | hoverEnterEvent (QGraphicsSceneHoverEvent *event) |
L_Override void | hoverLeaveEvent (QGraphicsSceneHoverEvent *event) |
L_Override void | mousePressEvent (QGraphicsSceneMouseEvent *event) |
L_Override void | mouseReleaseEvent (QGraphicsSceneMouseEvent *event) |
L_Override void | paint (QPainter *painter, const QStyleOptionGraphicsItem *option, QWidget *widget=0) |
void | paintBackground (QPainter *painter) |
void | paintBackground (QPainter *painter, const QString &element) |
void | setDown (bool value) |
void | setHovered (bool value) |
void | setPaintBackwardsWhenRTL (bool value) |
L_Override QSizeF | sizeHint (Qt::SizeHint which, const QSizeF &constraint=QSizeF()) const |
Properties | |
int | activationMethod |
bool | checkable |
bool | checked |
int | extenderPosition |
![]() | |
QIcon | icon |
QSize | iconSize |
QString | title |
![]() | |
bool | down |
QString | group |
Detailed Description
Button widget with special activation options beside clicking - hover and extender activation.
Definition at line 39 of file ExtenderButton.h.
Constructor & Destructor Documentation
Lancelot::ExtenderButton::ExtenderButton | ( | QGraphicsItem * | parent = 0 | ) |
Creates a new Lancelot::ExtenderButton.
- Parameters
-
parent parent item
Definition at line 299 of file ExtenderButton.cpp.
|
explicit |
Creates a new Lancelot::ExtenderButton.
- Parameters
-
title the title of the widget description the description of the widget parent parent item
Definition at line 307 of file ExtenderButton.cpp.
|
explicit |
Creates a new Lancelot::BasicWidget.
- Parameters
-
icon the icon for the widget title the title of the widget description the description of the widget parent parent item
Definition at line 318 of file ExtenderButton.cpp.
|
explicit |
Creates a new Lancelot::BasicWidget.
- Parameters
-
icon Svg with active, inactive and disabled states title the title of the widget description the description of the widget parent parent item
Definition at line 329 of file ExtenderButton.cpp.
|
virtual |
Destroys Lancelot::ExtenderButton.
Definition at line 345 of file ExtenderButton.cpp.
Member Function Documentation
|
protectedslot |
Definition at line 410 of file ExtenderButton.cpp.
|
signal |
Emitted when the button is activated.
- Parameters
-
checked true if the button is checked
int Lancelot::ExtenderButton::activationMethod | ( | ) | const |
- Returns
- activation method
QRectF Lancelot::ExtenderButton::boundingRect | ( | ) | const |
Definition at line 355 of file ExtenderButton.cpp.
int Lancelot::ExtenderButton::extenderPosition | ( | ) | const |
- Returns
- the extender position
|
protected |
Definition at line 371 of file ExtenderButton.cpp.
|
protected |
Definition at line 360 of file ExtenderButton.cpp.
|
protected |
Definition at line 377 of file ExtenderButton.cpp.
bool Lancelot::ExtenderButton::isCheckable | ( | ) | const |
- Returns
- whether the button is checkable
Definition at line 482 of file ExtenderButton.cpp.
bool Lancelot::ExtenderButton::isChecked | ( | ) | const |
- Returns
- whether the button is checked
Definition at line 459 of file ExtenderButton.cpp.
void Lancelot::ExtenderButton::paint | ( | QPainter * | painter, |
const QStyleOptionGraphicsItem * | option, | ||
QWidget * | widget = 0 |
||
) |
Definition at line 428 of file ExtenderButton.cpp.
|
protected |
Definition at line 422 of file ExtenderButton.cpp.
void Lancelot::ExtenderButton::setActivationMethod | ( | int | method | ) |
Sets the activation method of the ExtenderButton.
- Parameters
-
method new activation method
Definition at line 396 of file ExtenderButton.cpp.
void Lancelot::ExtenderButton::setCheckable | ( | bool | checkable | ) |
Makes the button checkable when set to true.
- Parameters
-
checkable checkable
Definition at line 472 of file ExtenderButton.cpp.
|
slot |
Sets whether the button is checked.
Has no effect if button is not checkable.
- Parameters
-
checked checked
Definition at line 450 of file ExtenderButton.cpp.
void Lancelot::ExtenderButton::setExtenderPosition | ( | int | position | ) |
Sets the position of the extender.
- Parameters
-
position new position
Definition at line 383 of file ExtenderButton.cpp.
|
virtual |
Sets this widget's group.
- Parameters
-
group new group
Reimplemented from Lancelot::Widget.
Definition at line 340 of file ExtenderButton.cpp.
|
virtual |
Sets a shortcut key.
Reimplemented from Lancelot::BasicWidget.
Definition at line 487 of file ExtenderButton.cpp.
|
slot |
Toggles the checked state.
Has no effect if button is not checkable.
Definition at line 464 of file ExtenderButton.cpp.
|
signal |
Emitted when the state of a checkable button is changed.
- Parameters
-
checked the new state
Property Documentation
|
readwrite |
Definition at line 46 of file ExtenderButton.h.
|
readwrite |
Definition at line 47 of file ExtenderButton.h.
|
readwrite |
Definition at line 48 of file ExtenderButton.h.
|
readwrite |
Definition at line 44 of file ExtenderButton.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Mon Oct 13 2014 22:55:07 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.