liblancelot
#include <StandardActionListModel.h>
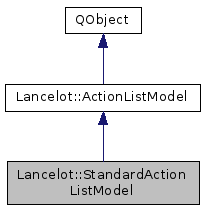
Classes | |
class | Item |
Public Member Functions | |
StandardActionListModel () | |
virtual | ~StandardActionListModel () |
void | add (const Item &item) |
void | add (const QString &title, const QString &description, QIcon icon, const QVariant &data) |
void | clear () |
L_Override QString | description (int index) const |
L_Override QIcon | icon (int index) const |
void | insert (int where, const Item &item) |
void | insert (int where, const QString &title, const QString &description, QIcon icon, const QVariant &data) |
L_Override bool | isCategory (int index) const |
const Item & | itemAt (int index) const |
void | removeAt (int index) |
void | set (int index, const Item &item) |
void | set (int index, const QString &title, const QString &description, QIcon icon, const QVariant &data) |
L_Override int | size () const |
L_Override QString | title (int index) const |
![]() | |
ActionListModel () | |
virtual | ~ActionListModel () |
virtual void | contextActivate (int index, QAction *context) |
virtual void | dataDragFinished (int index, Qt::DropAction action) |
virtual bool | dataDropAvailable (int where, const QMimeData *mimeData) |
virtual void | dataDropped (int where, const QMimeData *mimeData) |
virtual bool | hasContextActions (int index) const |
virtual QMimeData * | mimeData (int index) const |
virtual QIcon | selfIcon () const |
virtual QMimeData * | selfMimeData () const |
virtual QString | selfShortTitle () const |
virtual QString | selfTitle () const |
virtual void | setContextActions (int index, Lancelot::PopupMenu *menu) |
virtual void | setDropActions (int index, Qt::DropActions &actions, Qt::DropAction &defaultAction) |
Protected Member Functions | |
bool | emitInhibited () const |
void | setEmitInhibited (bool value) |
![]() | |
virtual void | activate (int index) |
Additional Inherited Members | |
![]() | |
void | activated (int index) |
![]() | |
void | itemActivated (int index) |
void | itemAltered (int index) |
void | itemDeleted (int index) |
void | itemInserted (int index) |
void | updated () |
Detailed Description
A basic implementation of ActionListModel.
Doesn't provide mimeData for drag and drop.
Definition at line 35 of file StandardActionListModel.h.
Constructor & Destructor Documentation
Lancelot::StandardActionListModel::StandardActionListModel | ( | ) |
Creates a new instance of StandardActionListModel.
Definition at line 30 of file StandardActionListModel.cpp.
|
virtual |
Destroys this StandardActionListModel.
Definition at line 36 of file StandardActionListModel.cpp.
Member Function Documentation
void Lancelot::StandardActionListModel::add | ( | const Item & | item | ) |
Adds a new item into the model.
- Parameters
-
item new item
Definition at line 84 of file StandardActionListModel.cpp.
void Lancelot::StandardActionListModel::add | ( | const QString & | title, |
const QString & | description, | ||
QIcon | icon, | ||
const QVariant & | data | ||
) |
Adds a new item into the model.
- Parameters
-
title the title for the new item description the description of the new item icon the icon for the new item data data for the new item. Not shown to user
Definition at line 92 of file StandardActionListModel.cpp.
void Lancelot::StandardActionListModel::clear | ( | ) |
Clears all items.
Definition at line 132 of file StandardActionListModel.cpp.
|
virtual |
- Parameters
-
index index of the item
- Returns
- description of the specified item
Reimplemented from Lancelot::ActionListModel.
Definition at line 61 of file StandardActionListModel.cpp.
|
protected |
- Returns
- whether emit signals are inhibited
Definition at line 46 of file StandardActionListModel.cpp.
|
virtual |
- Parameters
-
index index of the item
- Returns
- icon for the specified item
Reimplemented from Lancelot::ActionListModel.
Definition at line 67 of file StandardActionListModel.cpp.
void Lancelot::StandardActionListModel::insert | ( | int | where, |
const Item & | item | ||
) |
Adds a new item into the model.
- Parameters
-
where where to insert item new item
Definition at line 97 of file StandardActionListModel.cpp.
void Lancelot::StandardActionListModel::insert | ( | int | where, |
const QString & | title, | ||
const QString & | description, | ||
QIcon | icon, | ||
const QVariant & | data | ||
) |
Adds a new item into the model.
- Parameters
-
where where to insert title the title for the new item description the description of the new item icon the icon for the new item data data for the new item. Not shown to user
Definition at line 105 of file StandardActionListModel.cpp.
|
virtual |
- Parameters
-
index index of the item
- Returns
- whether the item represents a category
Reimplemented from Lancelot::ActionListModel.
Definition at line 73 of file StandardActionListModel.cpp.
const StandardActionListModel::Item & Lancelot::StandardActionListModel::itemAt | ( | int | index | ) | const |
- Returns
- the specified item
- Parameters
-
index index of the item to return
Definition at line 140 of file StandardActionListModel.cpp.
void Lancelot::StandardActionListModel::removeAt | ( | int | index | ) |
Removes an item.
- Parameters
-
index index of the item to remove
Definition at line 124 of file StandardActionListModel.cpp.
void Lancelot::StandardActionListModel::set | ( | int | index, |
const Item & | item | ||
) |
Replaces existing item at specified index with a new one.
- Parameters
-
index index of the item to be replaced item new item
Definition at line 110 of file StandardActionListModel.cpp.
void Lancelot::StandardActionListModel::set | ( | int | index, |
const QString & | title, | ||
const QString & | description, | ||
QIcon | icon, | ||
const QVariant & | data | ||
) |
Replaces existing item at specified index with a new one.
- Parameters
-
index index of the item to be replaced title the title for the new item description the description of the new item icon the icon for the new item data data for the new item. Not shown to user
Definition at line 119 of file StandardActionListModel.cpp.
|
protected |
Sets whether emit signals should be inhibited.
- Parameters
-
value value
Definition at line 41 of file StandardActionListModel.cpp.
|
virtual |
- Returns
- the number of items in model
Implements Lancelot::ActionListModel.
Definition at line 79 of file StandardActionListModel.cpp.
|
virtual |
- Parameters
-
index index of the item
- Returns
- title for the specified item
Implements Lancelot::ActionListModel.
Definition at line 51 of file StandardActionListModel.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Mon Oct 13 2014 22:55:07 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.