kcachegrind
#include <callgraphview.h>
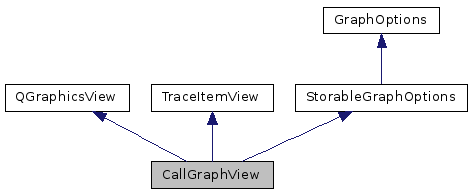
Public Types | |
enum | ZoomPosition { TopLeft, TopRight, BottomLeft, BottomRight, Auto, Hide } |
![]() | |
enum | { nothingChanged = 0, eventTypeChanged = 1, eventType2Changed = 2, groupTypeChanged = 4, partsChanged = 8, activeItemChanged = 16, selectedItemChanged = 32, dataChanged = 64, configChanged = 128 } |
enum | Direction { None, Back, Forward, Up } |
enum | Position { Hidden, Top, Right, Left, Bottom } |
![]() | |
enum | Layout { TopDown, LeftRight, Circular } |
Public Slots | |
void | calleeDepthTriggered (QAction *) |
void | callerDepthTriggered (QAction *) |
void | callLimitTriggered (QAction *) |
void | dotError () |
void | dotExited () |
void | layoutTriggered (QAction *) |
void | nodeLimitTriggered (QAction *) |
void | readDotOutput () |
void | showRenderError (QString) |
void | showRenderWarning () |
void | stopRendering () |
void | zoomPosTriggered (QAction *) |
void | zoomRectMoved (qreal, qreal) |
void | zoomRectMoveFinished () |
Public Member Functions | |
CallGraphView (TraceItemView *parentView, QWidget *parent=0, const char *name=0) | |
~CallGraphView () | |
void | restoreOptions (const QString &prefix, const QString &postfix) |
void | saveOptions (const QString &prefix, const QString &postfix) |
QString | whatsThis () const |
QWidget * | widget () |
ZoomPosition | zoomPos () const |
![]() | |
TraceItemView (TraceItemView *parentView, TopLevelBase *top=0) | |
virtual | ~TraceItemView () |
bool | activate (CostItem *i) |
virtual void | activated (TraceItemView *sender, CostItem *) |
TraceFunction * | activeFunction () |
CostItem * | activeItem () const |
void | addEventTypeMenu (QMenu *, bool withCost2=true) |
void | addGoMenu (QMenu *) |
TraceData * | data () const |
virtual void | directionActivated (TraceItemView *sender, Direction) |
EventType * | eventType () const |
EventType * | eventType2 () const |
ProfileContext::Type | groupType () const |
void | notifyChange (int changeType) |
const TracePartList & | partList () const |
virtual void | partsSelected (TraceItemView *sender, const TracePartList &) |
Position | position () const |
virtual void | restoreLayout (const QString &prefix, const QString &postfix) |
virtual void | saveLayout (const QString &prefix, const QString &postfix) |
void | select (CostItem *i) |
virtual void | selected (TraceItemView *sender, CostItem *) |
virtual void | selectedEventType (TraceItemView *sender, EventType *) |
virtual void | selectedEventType2 (TraceItemView *sender, EventType *) |
virtual void | selectedGroupType (TraceItemView *sender, ProfileContext::Type) |
CostItem * | selectedItem () const |
void | set (ProfileContext::Type g) |
void | set (const TracePartList &l) |
bool | set (int, TraceData *, EventType *, EventType *, ProfileContext::Type, const TracePartList &, CostItem *, CostItem *) |
virtual void | setData (TraceData *d) |
void | setEventType (EventType *t) |
void | setEventType2 (EventType *t) |
void | setMergeUpdates (bool b) |
void | setPosition (Position p) |
void | setTitle (QString t) |
void | setTopLevel (TopLevelBase *t) |
int | status () const |
QString | title () const |
TopLevelBase * | topLevel () const |
void | updateView (bool force=false) |
![]() | |
StorableGraphOptions () | |
virtual | ~StorableGraphOptions () |
virtual double | callLimit () |
virtual bool | clusterGroups () |
virtual int | detailLevel () |
virtual bool | expandCycles () |
virtual double | funcLimit () |
virtual Layout | layout () |
virtual int | maxCalleeDepth () |
virtual int | maxCallerDepth () |
void | setCallLimit (double l) |
void | setClusterGroups (bool b) |
void | setDetailLevel (int l) |
void | setExpandCycles (bool b) |
void | setFuncLimit (double l) |
void | setLayout (Layout l) |
void | setMaxCalleeDepth (int d) |
void | setMaxCallerDepth (int d) |
void | setShowSkipped (bool b) |
virtual bool | showSkipped () |
![]() | |
virtual | ~GraphOptions () |
Static Public Member Functions | |
static ZoomPosition | zoomPos (QString) |
static QString | zoomPosString (ZoomPosition) |
![]() | |
static Layout | layout (QString) |
static QString | layoutString (Layout) |
Protected Member Functions | |
void | contextMenuEvent (QContextMenuEvent *) |
void | focusInEvent (QFocusEvent *) |
void | focusOutEvent (QFocusEvent *) |
void | keyPressEvent (QKeyEvent *) |
void | mouseDoubleClickEvent (QMouseEvent *) |
void | mouseMoveEvent (QMouseEvent *) |
void | mousePressEvent (QMouseEvent *) |
void | mouseReleaseEvent (QMouseEvent *) |
void | resizeEvent (QResizeEvent *) |
void | scrollContentsBy (int dx, int dy) |
![]() | |
void | activated (CostItem *) |
void | directionActivated (TraceItemView::Direction) |
virtual bool | isViewVisible () |
void | partsSelected (const TracePartList &) |
void | selected (CostItem *) |
void | selectedEventType (EventType *) |
void | selectedEventType2 (EventType *) |
void | selectedGroupType (ProfileContext::Type) |
Additional Inherited Members | |
![]() | |
CostItem * | _activeItem |
TraceData * | _data |
EventType * | _eventType |
EventType * | _eventType2 |
ProfileContext::Type | _groupType |
TraceItemView * | _parentView |
TracePartList | _partList |
CostItem * | _selectedItem |
TopLevelBase * | _topLevel |
![]() | |
double | _callLimit |
bool | _clusterGroups |
int | _detailLevel |
bool | _expandCycles |
double | _funcLimit |
Layout | _layout |
int | _maxCalleeDepth |
int | _maxCallerDepth |
bool | _showSkipped |
Detailed Description
A QGraphicsView showing a part of the call graph and another zoomed out CanvasView in a border acting as a panner to select to visible part (only if needed)
Definition at line 574 of file callgraphview.h.
Member Enumeration Documentation
Enumerator | |
---|---|
TopLeft | |
TopRight | |
BottomLeft | |
BottomRight | |
Auto | |
Hide |
Definition at line 580 of file callgraphview.h.
Constructor & Destructor Documentation
|
explicit |
Definition at line 1500 of file callgraphview.cpp.
CallGraphView::~CallGraphView | ( | ) |
Definition at line 1540 of file callgraphview.cpp.
Member Function Documentation
|
slot |
Definition at line 2768 of file callgraphview.cpp.
|
slot |
Definition at line 2729 of file callgraphview.cpp.
|
slot |
Definition at line 2848 of file callgraphview.cpp.
|
protected |
Definition at line 2924 of file callgraphview.cpp.
|
slot |
Definition at line 2107 of file callgraphview.cpp.
|
slot |
Definition at line 2127 of file callgraphview.cpp.
|
protected |
Definition at line 1698 of file callgraphview.cpp.
|
protected |
Definition at line 1708 of file callgraphview.cpp.
|
protected |
Definition at line 1714 of file callgraphview.cpp.
|
slot |
Definition at line 2917 of file callgraphview.cpp.
|
protected |
Definition at line 2660 of file callgraphview.cpp.
|
protected |
Definition at line 2639 of file callgraphview.cpp.
|
protected |
Definition at line 2600 of file callgraphview.cpp.
|
protected |
Definition at line 2653 of file callgraphview.cpp.
|
slot |
Definition at line 2811 of file callgraphview.cpp.
|
slot |
Definition at line 2093 of file callgraphview.cpp.
|
protected |
Definition at line 1814 of file callgraphview.cpp.
|
virtual |
Reimplemented from TraceItemView.
Definition at line 3143 of file callgraphview.cpp.
|
virtual |
Reimplemented from TraceItemView.
Definition at line 3163 of file callgraphview.cpp.
|
protected |
Definition at line 2567 of file callgraphview.cpp.
|
slot |
Definition at line 1971 of file callgraphview.cpp.
|
slot |
Definition at line 1955 of file callgraphview.cpp.
|
slot |
Definition at line 1986 of file callgraphview.cpp.
|
virtual |
Reimplemented from TraceItemView.
Definition at line 1546 of file callgraphview.cpp.
|
inlinevirtual |
Implements TraceItemView.
Definition at line 589 of file callgraphview.h.
|
inline |
Definition at line 596 of file callgraphview.h.
|
static |
Definition at line 3107 of file callgraphview.cpp.
|
static |
Definition at line 3125 of file callgraphview.cpp.
|
slot |
Definition at line 2884 of file callgraphview.cpp.
|
slot |
Definition at line 2583 of file callgraphview.cpp.
|
slot |
Definition at line 2594 of file callgraphview.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:03:27 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.