kcachegrind
#include <treemap.h>
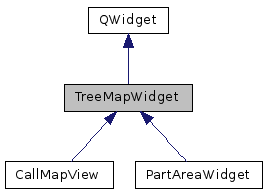
Public Types | |
enum | SelectionMode { Single, Multi, Extended, NoSelection } |
Signals | |
void | clicked (TreeMapItem *) |
void | contextMenuRequested (TreeMapItem *, const QPoint &) |
void | currentChanged (TreeMapItem *, bool keyboard) |
void | doubleClicked (TreeMapItem *) |
void | returnPressed (TreeMapItem *) |
void | rightButtonPressed (TreeMapItem *, const QPoint &) |
void | selectionChanged () |
void | selectionChanged (TreeMapItem *) |
Protected Slots | |
void | splitActivated (QAction *) |
Protected Member Functions | |
void | contextMenuEvent (QContextMenuEvent *) |
bool | event (QEvent *event) |
void | fontChange (const QFont &) |
void | keyPressEvent (QKeyEvent *) |
void | mouseDoubleClickEvent (QMouseEvent *) |
void | mouseMoveEvent (QMouseEvent *) |
void | mousePressEvent (QMouseEvent *) |
void | mouseReleaseEvent (QMouseEvent *) |
void | paintEvent (QPaintEvent *) |
Detailed Description
Class for visualization of a metric of hierarchically nested items as 2D areas.
Member Enumeration Documentation
Constructor & Destructor Documentation
|
explicit |
Definition at line 1153 of file treemap.cpp.
TreeMapWidget::~TreeMapWidget | ( | ) |
Definition at line 1199 of file treemap.cpp.
Member Function Documentation
void TreeMapWidget::addSplitDirectionItems | ( | QMenu * | m | ) |
Populate given menu with option items.
The added items are automatically connected to handlers.
Definition at line 2848 of file treemap.cpp.
|
inline |
Returns the TreeMapItem filling out the widget space.
bool TreeMapWidget::clearSelection | ( | TreeMapItem * | parent = 0 | ) |
Clear selection of all selected items which are children of parent.
When parent == 0, clears whole selection Returns true if selection changed.
Definition at line 1705 of file treemap.cpp.
|
signal |
|
protected |
Definition at line 1820 of file treemap.cpp.
|
signal |
|
inline |
|
signal |
This signal is emitted if the current item changes.
If the change is done because of keyboard navigation, the is set to true
const QFont & TreeMapWidget::currentFont | ( | ) | const |
Returns a reference to the current widget font.
Definition at line 1204 of file treemap.cpp.
bool TreeMapWidget::defaultFieldForced | ( | int | ) | const |
Definition at line 1337 of file treemap.cpp.
DrawParams::Position TreeMapWidget::defaultFieldPosition | ( | int | f | ) | const |
Definition at line 1342 of file treemap.cpp.
QString TreeMapWidget::defaultFieldStop | ( | int | ) | const |
Definition at line 1327 of file treemap.cpp.
QString TreeMapWidget::defaultFieldType | ( | int | f | ) | const |
Definition at line 1322 of file treemap.cpp.
bool TreeMapWidget::defaultFieldVisible | ( | int | f | ) | const |
Definition at line 1332 of file treemap.cpp.
void TreeMapWidget::deletingItem | ( | TreeMapItem * | i | ) |
Definition at line 1501 of file treemap.cpp.
|
signal |
void TreeMapWidget::drawFrame | ( | int | d, |
bool | b | ||
) |
Definition at line 1265 of file treemap.cpp.
void TreeMapWidget::drawTreeMap | ( | ) |
Definition at line 2178 of file treemap.cpp.
|
protected |
Definition at line 2148 of file treemap.cpp.
bool TreeMapWidget::fieldForced | ( | int | f | ) | const |
Definition at line 1434 of file treemap.cpp.
DrawParams::Position TreeMapWidget::fieldPosition | ( | int | f | ) | const |
Definition at line 1453 of file treemap.cpp.
QString TreeMapWidget::fieldPositionString | ( | int | f | ) | const |
Definition at line 1479 of file treemap.cpp.
QString TreeMapWidget::fieldStop | ( | int | f | ) | const |
Definition at line 1398 of file treemap.cpp.
QString TreeMapWidget::fieldType | ( | int | f | ) | const |
Definition at line 1382 of file treemap.cpp.
bool TreeMapWidget::fieldVisible | ( | int | f | ) | const |
Definition at line 1415 of file treemap.cpp.
|
protected |
Definition at line 2142 of file treemap.cpp.
bool TreeMapWidget::isSelected | ( | TreeMapItem * | i | ) | const |
Definition at line 1722 of file treemap.cpp.
TreeMapItem * TreeMapWidget::item | ( | int | x, |
int | y | ||
) | const |
Returns the area item at position x/y, independent from any maxSelectDepth setting.
Definition at line 1541 of file treemap.cpp.
|
protected |
Definition at line 2027 of file treemap.cpp.
|
protected |
Definition at line 1980 of file treemap.cpp.
|
protected |
Definition at line 1907 of file treemap.cpp.
|
protected |
Definition at line 1839 of file treemap.cpp.
|
protected |
Definition at line 1950 of file treemap.cpp.
|
protected |
Definition at line 2171 of file treemap.cpp.
TreeMapItem * TreeMapWidget::possibleSelection | ( | TreeMapItem * | i | ) | const |
Returns the item possible for selection.
this returns the given item itself or a parent thereof, depending on setting of maxSelectDepth().
Definition at line 1593 of file treemap.cpp.
void TreeMapWidget::redraw | ( | TreeMapItem * | i | ) |
Redraws an item with all children.
This takes changed values(), sums(), colors() and text() into account.
Definition at line 2231 of file treemap.cpp.
|
inline |
|
signal |
|
signal |
|
inline |
|
signal |
|
signal |
|
inline |
void TreeMapWidget::setAllowRotation | ( | bool | enable | ) |
Do we allow the texts to be rotated by 90 degrees for better fitting?
Definition at line 1281 of file treemap.cpp.
void TreeMapWidget::setBorderWidth | ( | int | w | ) |
Definition at line 1306 of file treemap.cpp.
void TreeMapWidget::setCurrent | ( | TreeMapItem * | i, |
bool | kbd = false |
||
) |
Sets the current item.
The current item is mainly used for keyboard navigation.
Definition at line 1735 of file treemap.cpp.
void TreeMapWidget::setFieldForced | ( | int | f, |
bool | enable | ||
) |
Should the drawing of the name into the rectangle be forced? This enables drawing of the name before drawing subitems, and thus destroys proportional constrains.
Definition at line 1423 of file treemap.cpp.
void TreeMapWidget::setFieldPosition | ( | int | f, |
DrawParams::Position | pos | ||
) |
Set the field position in the area.
Definition at line 1442 of file treemap.cpp.
void TreeMapWidget::setFieldPosition | ( | int | f, |
const QString & | pos | ||
) |
Definition at line 1461 of file treemap.cpp.
void TreeMapWidget::setFieldStop | ( | int | f, |
const QString & | stop | ||
) |
Stop drawing at item with name.
Definition at line 1388 of file treemap.cpp.
void TreeMapWidget::setFieldType | ( | int | f, |
const QString & | type | ||
) |
Set the type name of a field.
This is important for the visualization menu generated with visualizationMenu()
Definition at line 1373 of file treemap.cpp.
void TreeMapWidget::setFieldVisible | ( | int | f, |
bool | enable | ||
) |
Should the text with number textNo be visible? This is only done if remaining space is enough to allow for proportional size constrains.
Definition at line 1404 of file treemap.cpp.
void TreeMapWidget::setMarked | ( | int | markNo = 1 , |
bool | redraw = true |
||
) |
Switches on the marking <markNo>.
Marking 0 switches off marking. This is mutually exclusive to selection, and is automatically switched off when selection is changed (also by the user). Marking is visually the same as selection, and is based on TreeMapItem::isMarked(<markNo>). This enables to programmatically show multiple selected items at once even in single selection mode.
Definition at line 1647 of file treemap.cpp.
void TreeMapWidget::setMaxDrawingDepth | ( | int | d | ) |
Maximal nesting depth.
Definition at line 1314 of file treemap.cpp.
|
inline |
Set the maximal depth a selected item can have.
If you try to select a item with higher depth, the ancestor holding this condition is used.
See also possibleSelection().
void TreeMapWidget::setMinimalArea | ( | int | area | ) |
Minimal area for rectangles to draw.
Definition at line 1492 of file treemap.cpp.
void TreeMapWidget::setRangeSelection | ( | TreeMapItem * | i1, |
TreeMapItem * | i2, | ||
bool | selected | ||
) |
Selects or unselects items in a range.
This is needed internally for Shift-Click in Extended mode. Range means for a hierarchical widget:
- select/unselect i1 and i2 according selected
- search common parent of i1 and i2, and select/unselect the range of direct children between but excluding the child leading to i1 and the child leading to i2.
Definition at line 1764 of file treemap.cpp.
void TreeMapWidget::setSelected | ( | TreeMapItem * | item, |
bool | selected = true |
||
) |
Selects or unselects an item.
In multiselection mode, the constrain that a selected item has no selected children or parents stays true.
Definition at line 1626 of file treemap.cpp.
|
inline |
void TreeMapWidget::setShadingEnabled | ( | bool | s | ) |
Definition at line 1257 of file treemap.cpp.
void TreeMapWidget::setSkipIncorrectBorder | ( | bool | enable = true | ) |
If a children value() is almost the parents sum(), it can happen that the border to be drawn for visibilty of nesting relations takes to much space, and the parent/child size relation can not be mapped to a correct area size relation.
Either (1) Ignore the incorrect drawing, or (2) Skip drawing of the parent level alltogether.
Definition at line 1298 of file treemap.cpp.
void TreeMapWidget::setSplitMode | ( | TreeMapItem::SplitMode | m | ) |
for setting/getting global split direction
Definition at line 1209 of file treemap.cpp.
bool TreeMapWidget::setSplitMode | ( | const QString & | mode | ) |
Definition at line 1222 of file treemap.cpp.
void TreeMapWidget::setTransparent | ( | int | d, |
bool | b | ||
) |
Definition at line 1273 of file treemap.cpp.
void TreeMapWidget::setVisibleWidth | ( | int | width, |
bool | reuseSpace = false |
||
) |
Items usually have a size proportional to their value().
With <width>, you can give the minimum width of the resulting rectangle to still be drawn. For space not used because of to small items, you can specify with <reuseSpace> if the background should shine through or the space will be used to enlarge the next item to be drawn at this level.
Definition at line 1289 of file treemap.cpp.
|
protectedslot |
Definition at line 2835 of file treemap.cpp.
TreeMapItem::SplitMode TreeMapWidget::splitMode | ( | ) | const |
Definition at line 1217 of file treemap.cpp.
QString TreeMapWidget::splitModeString | ( | ) | const |
Definition at line 1238 of file treemap.cpp.
|
virtual |
Return tooltip string to show for a item (can be rich text) Default implementation gives lines with "text0 (text1)" going to root.
Reimplemented in PartAreaWidget, and CallMapView.
Definition at line 1521 of file treemap.cpp.
TreeMapItem * TreeMapWidget::visibleItem | ( | TreeMapItem * | i | ) | const |
Returns the nearest item with a visible area; this can be the given item itself.
Definition at line 1607 of file treemap.cpp.
|
inline |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:03:28 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.