okteta
#include <abstractbytearraycolumnrenderer.h>
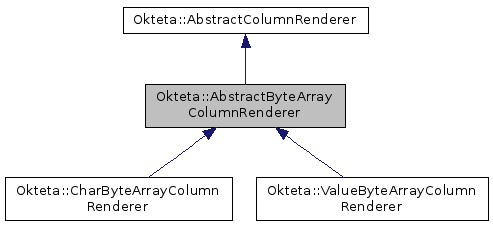
Public Types | |
enum | FrameStyle { Frame, Left, Right } |
Protected Member Functions | |
bool | getNextMarkedAddressRange (AddressRange *markedRange, unsigned int *flag, const AddressRange &range) const |
bool | getNextSelectedAddressRange (AddressRange *selectedRange, unsigned int *flag, const AddressRange &range) const |
virtual void | recalcByteWidth () |
void | recalcX () |
void | renderBookmark (QPainter *painter, const QBrush &brush) |
virtual void | renderByteText (QPainter *painter, Byte byte, Character charByte, const QColor &color) const =0 |
void | renderMarking (QPainter *painter, const LinePositionRange &linePositions, Address byteIndex, int flag) |
void | renderPlain (QPainter *painter, const LinePositionRange &linePositions, Address byteIndex) |
void | renderRange (QPainter *painter, const QBrush &brush, const LinePositionRange &linePositions, int flag) |
void | renderSelection (QPainter *painter, const LinePositionRange &linePositions, Address byteIndex, int flag) |
void | renderSelectionSpaceBehind (QPainter *painter, LinePosition linePosition) |
void | renderSpaceBehind (QPainter *painter, const QBrush &brush, LinePosition linePosition) |
void | setByteWidth (int byteWidth) |
![]() | |
void | renderBlankLine (QPainter *painter) const |
void | restrictToXSpan (PixelXRange *xSpan) const |
void | setWidth (PixelX width) |
Detailed Description
base class of all buffer column displayers holds all information about the vertical layout of a buffer column knows how to paint the data and the editing things (focus, cursor, selection) but does not offer
Definition at line 60 of file abstractbytearraycolumnrenderer.h.
Member Enumeration Documentation
Enumerator | |
---|---|
Frame | |
Left | |
Right |
Definition at line 63 of file abstractbytearraycolumnrenderer.h.
Constructor & Destructor Documentation
Okteta::AbstractByteArrayColumnRenderer::AbstractByteArrayColumnRenderer | ( | AbstractColumnStylist * | stylist, |
AbstractByteArrayModel * | byteArrayModel, | ||
ByteArrayTableLayout * | layout, | ||
ByteArrayTableRanges * | ranges | ||
) |
Definition at line 48 of file abstractbytearraycolumnrenderer.cpp.
|
virtual |
Definition at line 790 of file abstractbytearraycolumnrenderer.cpp.
Member Function Documentation
QRect Okteta::AbstractByteArrayColumnRenderer::byteRect | ( | const Coord & | coord | ) | const |
Definition at line 354 of file abstractbytearraycolumnrenderer.cpp.
|
inline |
Definition at line 251 of file abstractbytearraycolumnrenderer.h.
|
inline |
Definition at line 249 of file abstractbytearraycolumnrenderer.h.
PixelX Okteta::AbstractByteArrayColumnRenderer::columnRightXOfLinePosition | ( | LinePosition | posInLine | ) | const |
returns right relative x-coord of byte at position posInLine
Definition at line 328 of file abstractbytearraycolumnrenderer.cpp.
PixelX Okteta::AbstractByteArrayColumnRenderer::columnXOfLinePosition | ( | LinePosition | posInLine | ) | const |
returns relative x-coord of byte at position posInLine
Definition at line 323 of file abstractbytearraycolumnrenderer.cpp.
PixelXRange Okteta::AbstractByteArrayColumnRenderer::columnXsOfLinePositionsInclSpaces | ( | const LinePositionRange & | linePositions | ) | const |
Definition at line 344 of file abstractbytearraycolumnrenderer.cpp.
|
inline |
Definition at line 250 of file abstractbytearraycolumnrenderer.h.
|
inline |
Definition at line 256 of file abstractbytearraycolumnrenderer.h.
|
protected |
Definition at line 762 of file abstractbytearraycolumnrenderer.cpp.
|
protected |
Definition at line 732 of file abstractbytearraycolumnrenderer.cpp.
|
inline |
Definition at line 252 of file abstractbytearraycolumnrenderer.h.
|
inline |
Definition at line 271 of file abstractbytearraycolumnrenderer.h.
|
inline |
Definition at line 257 of file abstractbytearraycolumnrenderer.h.
|
inline |
Definition at line 260 of file abstractbytearraycolumnrenderer.h.
LinePosition Okteta::AbstractByteArrayColumnRenderer::linePositionOfColumnX | ( | PixelX | x | ) | const |
returns byte pos at pixel with relative x-coord x
Definition at line 282 of file abstractbytearraycolumnrenderer.cpp.
LinePosition Okteta::AbstractByteArrayColumnRenderer::linePositionOfX | ( | PixelX | x | ) | const |
returns byte pos at pixel with absolute x-coord x
Definition at line 206 of file abstractbytearraycolumnrenderer.cpp.
LinePositionRange Okteta::AbstractByteArrayColumnRenderer::linePositionsOfColumnXs | ( | PixelX | x, |
PixelX | width | ||
) | const |
returns byte linePositions covered by pixels with relative x-coord x
Definition at line 296 of file abstractbytearraycolumnrenderer.cpp.
LinePositionRange Okteta::AbstractByteArrayColumnRenderer::linePositionsOfX | ( | PixelX | x, |
PixelX | width | ||
) | const |
returns byte linePositions covered by pixels with absolute x-coord x
Definition at line 243 of file abstractbytearraycolumnrenderer.cpp.
LinePosition Okteta::AbstractByteArrayColumnRenderer::magneticLinePositionOfX | ( | PixelX | x | ) | const |
returns byte pos at pixel with absolute x-coord x, and sets the flag to true if we are closer to the right
Definition at line 222 of file abstractbytearraycolumnrenderer.cpp.
|
inline |
Definition at line 254 of file abstractbytearraycolumnrenderer.h.
void Okteta::AbstractByteArrayColumnRenderer::prepareRendering | ( | const PixelXRange & | Xs | ) |
Definition at line 368 of file abstractbytearraycolumnrenderer.cpp.
|
protectedvirtual |
default implementation sets byte width to one digit width
Reimplemented in Okteta::ValueByteArrayColumnRenderer.
Definition at line 170 of file abstractbytearraycolumnrenderer.cpp.
|
protected |
Definition at line 176 of file abstractbytearraycolumnrenderer.cpp.
|
protected |
Definition at line 622 of file abstractbytearraycolumnrenderer.cpp.
void Okteta::AbstractByteArrayColumnRenderer::renderByte | ( | QPainter * | painter, |
Address | byteIndex | ||
) |
paints the byte with background.
- Parameters
-
byteIndex Index of the byte to paint. If -1 only the background is painted.
Definition at line 651 of file abstractbytearraycolumnrenderer.cpp.
|
protectedpure virtual |
Implemented in Okteta::CharByteArrayColumnRenderer, and Okteta::ValueByteArrayColumnRenderer.
void Okteta::AbstractByteArrayColumnRenderer::renderCursor | ( | QPainter * | painter, |
Address | byteIndex | ||
) |
paints a cursor based on the type of the byte.
- Parameters
-
byteIndex Index of the byte to paint the cursor for. If -1 a space is used as char.
Definition at line 715 of file abstractbytearraycolumnrenderer.cpp.
|
virtual |
Definition at line 384 of file abstractbytearraycolumnrenderer.cpp.
void Okteta::AbstractByteArrayColumnRenderer::renderFramedByte | ( | QPainter * | painter, |
Address | byteIndex, | ||
FrameStyle | style | ||
) |
paints the byte with background and a frame around.
- Parameters
-
byteIndex Index of the byte to paint the frame for. If -1 a space is used as char. style the style of the framing
Definition at line 689 of file abstractbytearraycolumnrenderer.cpp.
void Okteta::AbstractByteArrayColumnRenderer::renderLinePositions | ( | QPainter * | painter, |
Line | lineIndex, | ||
const LineRange & | linePositions | ||
) |
Definition at line 400 of file abstractbytearraycolumnrenderer.cpp.
|
protected |
Definition at line 599 of file abstractbytearraycolumnrenderer.cpp.
|
virtual |
the actual painting call for a column's line.
The default implementation simply paints the background
Reimplemented from Okteta::AbstractColumnRenderer.
Definition at line 394 of file abstractbytearraycolumnrenderer.cpp.
|
protected |
Definition at line 499 of file abstractbytearraycolumnrenderer.cpp.
|
protected |
Definition at line 629 of file abstractbytearraycolumnrenderer.cpp.
|
protected |
Definition at line 544 of file abstractbytearraycolumnrenderer.cpp.
|
protected |
Definition at line 590 of file abstractbytearraycolumnrenderer.cpp.
|
protected |
Definition at line 642 of file abstractbytearraycolumnrenderer.cpp.
void Okteta::AbstractByteArrayColumnRenderer::resetXBuffer | ( | ) |
creates new buffer for x-values; to be called on any change of NoOfBytesPerLine or metrics
Definition at line 77 of file abstractbytearraycolumnrenderer.cpp.
PixelX Okteta::AbstractByteArrayColumnRenderer::rightXOfLinePosition | ( | LinePosition | posInLine | ) | const |
returns right absolute x-coord of byte at position posInLine
Definition at line 276 of file abstractbytearraycolumnrenderer.cpp.
void Okteta::AbstractByteArrayColumnRenderer::set | ( | AbstractByteArrayModel * | byteArrayModel | ) |
Definition at line 70 of file abstractbytearraycolumnrenderer.cpp.
bool Okteta::AbstractByteArrayColumnRenderer::setByteSpacingWidth | ( | PixelX | byteSpacingWidth | ) |
sets the spacing between the bytes in the hex column
- Parameters
-
byteSpacingWidth spacing between the bytes in pixels returns true if there was a change
Definition at line 124 of file abstractbytearraycolumnrenderer.cpp.
|
inline |
Definition at line 267 of file abstractbytearraycolumnrenderer.h.
|
inlineprotected |
Definition at line 273 of file abstractbytearraycolumnrenderer.h.
|
inline |
sets the codec to be used by the char column.
Definition at line 262 of file abstractbytearraycolumnrenderer.h.
void Okteta::AbstractByteArrayColumnRenderer::setFontMetrics | ( | const QFontMetrics & | fontMetrics | ) |
sets the metrics of the used font
Definition at line 91 of file abstractbytearraycolumnrenderer.cpp.
bool Okteta::AbstractByteArrayColumnRenderer::setGroupSpacingWidth | ( | PixelX | groupSpacingWidth | ) |
sets the spacing between the groups of bytes in the hex column
- Parameters
-
groupSpacingWidth spacing between the groups in pixels returns true if there was a change
Definition at line 154 of file abstractbytearraycolumnrenderer.cpp.
bool Okteta::AbstractByteArrayColumnRenderer::setNoOfGroupedBytes | ( | int | noOfGroupedBytes | ) |
sets the number of grouped bytes in the hex column
- Parameters
-
noOfGroupedBytes numbers of grouped bytes, 0 means no grouping returns true if there was a change
Definition at line 140 of file abstractbytearraycolumnrenderer.cpp.
bool Okteta::AbstractByteArrayColumnRenderer::setSpacing | ( | PixelX | byteSpacingWidth, |
int | noOfGroupedBytes = 0 , |
||
PixelX | groupSpacingWidth = 0 |
||
) |
sets the spacing in the hex column
- Parameters
-
byteSpacingWidth spacing between the bytes in pixels noOfGroupedBytes numbers of grouped bytes, 0 means no grouping groupSpacingWidth spacing between the groups in pixels returns true if there was a change
Definition at line 106 of file abstractbytearraycolumnrenderer.cpp.
LinePositionRange Okteta::AbstractByteArrayColumnRenderer::visibleLinePositions | ( | PixelX | x, |
PixelX | width | ||
) | const |
returns the linePositions that overlap with the x-coords relative to the view
|
inline |
Definition at line 258 of file abstractbytearraycolumnrenderer.h.
PixelX Okteta::AbstractByteArrayColumnRenderer::xOfLinePosition | ( | LinePosition | posInLine | ) | const |
returns absolute x-coord of byte at position posInLine
Definition at line 271 of file abstractbytearraycolumnrenderer.cpp.
PixelXRange Okteta::AbstractByteArrayColumnRenderer::xsOfLinePositionsInclSpaces | ( | const LinePositionRange & | linePositions | ) | const |
returns the
Definition at line 334 of file abstractbytearraycolumnrenderer.cpp.
Member Data Documentation
|
protected |
Definition at line 204 of file abstractbytearraycolumnrenderer.h.
|
protected |
pointer to the buffer
Definition at line 198 of file abstractbytearraycolumnrenderer.h.
|
protected |
width of inserting cursor in pixel
size of the line margin
Definition at line 221 of file abstractbytearraycolumnrenderer.h.
|
protected |
Definition at line 237 of file abstractbytearraycolumnrenderer.h.
|
protected |
total width of byte display in pixel
Definition at line 217 of file abstractbytearraycolumnrenderer.h.
|
protected |
Definition at line 206 of file abstractbytearraycolumnrenderer.h.
|
protected |
Definition at line 211 of file abstractbytearraycolumnrenderer.h.
|
protected |
Definition at line 209 of file abstractbytearraycolumnrenderer.h.
|
protected |
Definition at line 213 of file abstractbytearraycolumnrenderer.h.
|
protected |
width of spacing in pixel
Definition at line 223 of file abstractbytearraycolumnrenderer.h.
|
protected |
index of right position
Definition at line 234 of file abstractbytearraycolumnrenderer.h.
|
protected |
pointer to the layout
Definition at line 200 of file abstractbytearraycolumnrenderer.h.
|
protected |
pointer to array with buffered linePositions (relative to column position) a spacing gets assigned to the left byte -> ...c|c|c |c|c...
Definition at line 231 of file abstractbytearraycolumnrenderer.h.
|
protected |
Definition at line 232 of file abstractbytearraycolumnrenderer.h.
|
protected |
number of grouped bytes
Definition at line 226 of file abstractbytearraycolumnrenderer.h.
|
protected |
pointer to the ranges
Definition at line 202 of file abstractbytearraycolumnrenderer.h.
|
protected |
Definition at line 242 of file abstractbytearraycolumnrenderer.h.
|
protected |
Definition at line 241 of file abstractbytearraycolumnrenderer.h.
|
protected |
Definition at line 244 of file abstractbytearraycolumnrenderer.h.
|
protected |
Definition at line 243 of file abstractbytearraycolumnrenderer.h.
|
protected |
Definition at line 245 of file abstractbytearraycolumnrenderer.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:04:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.