okteta
#include <abstractbytearraymodel.h>
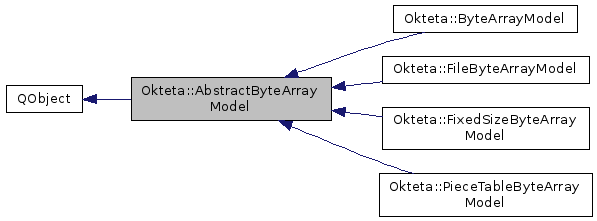
Signals | |
void | contentsChanged (const Okteta::ArrayChangeMetricsList &changeList) |
void | modifiedChanged (bool isModified) |
void | readOnlyChanged (bool isReadOnly) |
void | searchedBytes (Okteta::Size bytes) const |
Public Member Functions | |
virtual | ~AbstractByteArrayModel () |
virtual Byte | byte (Address offset) const =0 |
virtual Size | copyTo (Byte *dest, const AddressRange ©Range) const |
Size | copyTo (Byte *dest, Address offset, Size copyLength) const |
virtual Size | fill (Byte fillByte, Address offset=0, Size fillLength=-1)=0 |
Size | fill (Byte fillChar, const AddressRange &fillRange) |
virtual Address | indexOf (const Byte *pattern, int patternLength, Address fromOffset=0, Address toOffset=-1) const |
Address | indexOf (const QByteArray &pattern, Address fromOffset=0, Address toOffset=-1) const |
Address | indexOfCaseInsensitive (const CharCodec *charCodec, const QByteArray &pattern, Address fromOffset=0, Address toOffset=-1) const |
virtual Size | insert (Address offset, const Byte *insertData, int insertLength) |
Size | insert (Address offset, const QByteArray &insertData) |
virtual bool | isModified () const =0 |
virtual bool | isReadOnly () const |
virtual Address | lastIndexOf (const Byte *pattern, int patternLength, Address fromOffset=-1, Address toOffset=0) const |
Address | lastIndexOf (const QByteArray &pattern, Address fromOffset=-1, Address toOffset=0) const |
Address | lastIndexOfCaseInsensitive (const CharCodec *charCodec, const QByteArray &pattern, Address fromOffset=-1, Address toOffset=0) const |
virtual Size | remove (const AddressRange &removeRange) |
Size | remove (Address offset, Size removeLength) |
virtual Size | replace (const AddressRange &removeRange, const Byte *insertData, int insertLength)=0 |
Size | replace (const AddressRange &removeRange, const QByteArray &insertData) |
Size | replace (Address offset, Size removeLength, const Byte *insertData, Size insertLength) |
virtual void | setByte (Address offset, Byte byte)=0 |
virtual void | setModified (bool modified)=0 |
virtual void | setReadOnly (bool isReadOnly) |
virtual Size | size () const =0 |
virtual bool | swap (Address firstStart, const AddressRange &secondRange)=0 |
Protected Member Functions | |
AbstractByteArrayModel (QObject *parent=0) | |
Detailed Description
could it be useful to hide the data access behind an iterator? * class KDataBufferIterator { public: bool hasNext(); char next(); protected:
protected: char * int Length; }
bool KDataBufferIterator::hasNext() { } this function should be simple as possible char KDataBufferIterator::next() { if next span is empty if( ) return *NextChar++; } base class for all Data buffers that are used to display TODO: think about a way to inform KHexEdit that there has been a change in the buffer outside. what kind of changes are possible?
Operations on the data:
Finding: is implemented stateless. FindNext has to be done by perhaps a FindManager Replacing: not available. Implement within a ReplaceManager
Definition at line 79 of file abstractbytearraymodel.h.
Constructor & Destructor Documentation
|
explicitprotected |
Definition at line 36 of file abstractbytearraymodel.cpp.
|
virtual |
Definition at line 260 of file abstractbytearraymodel.cpp.
Member Function Documentation
locates working range The idea behind is to tell buffer which range will be requested in the following time, so that e.g.
the corresponding pages will be loaded in advance TODO: do we really need this? Where will this lead to real enhancements?
- Parameters
-
Range
- Returns
true
if successfully,false
otherwiseconvenience function, same as above creates an iterator to expects pointer to memory, should be in prepared range it is only expected to be a valid pointer until any further call to this or any modifying function
- Parameters
-
Section
- Returns
- pointer to convenience function, same as above requests a single byte if the offset is not valid the behaviour is undefined
- Parameters
-
offset offset of the datum requested
Implemented in Okteta::PieceTableByteArrayModel, Okteta::ByteArrayModel, Okteta::FixedSizeByteArrayModel, and Okteta::FileByteArrayModel.
|
signal |
|
virtual |
copies the data of the section into a given array Dest.
If the section extends the buffers range the section is limited to the buffer's end. If the section is invalid the behaviour is undefined.
- Parameters
-
dest pointer to a char array large enough to hold the copied section copyRange
- Returns
- number of copied bytes
Definition at line 60 of file abstractbytearraymodel.cpp.
|
inline |
convenience function, behaves as above
Definition at line 283 of file abstractbytearraymodel.h.
|
pure virtual |
fills the buffer with the FillChar.
If the buffer is to small it will be extended as much as possible.
- Parameters
-
fillByte byte to use offset position where the filling starts fillLength number of bytes to fill. If Length is -1, the buffer is filled till the end.
- Returns
- number of filled bytes
Implemented in Okteta::PieceTableByteArrayModel, Okteta::ByteArrayModel, Okteta::FixedSizeByteArrayModel, and Okteta::FileByteArrayModel.
|
inline |
Definition at line 280 of file abstractbytearraymodel.h.
|
virtual |
searches beginning with byte at Pos.
- Parameters
-
pattern patternLength length of search string fromOffset the position to start the search
- Returns
- index of the first or -1
Definition at line 73 of file abstractbytearraymodel.cpp.
|
inline |
Definition at line 286 of file abstractbytearraymodel.h.
Address Okteta::AbstractByteArrayModel::indexOfCaseInsensitive | ( | const CharCodec * | charCodec, |
const QByteArray & | pattern, | ||
Address | fromOffset = 0 , |
||
Address | toOffset = -1 |
||
) | const |
Definition at line 162 of file abstractbytearraymodel.cpp.
|
virtual |
inserts bytes copied from the given source at Position.
The original data beginnung at the position is moved with the buffer enlarged as needed If the buffer is readOnly this is a noop and returns 0.
- Parameters
-
offset insertData data source insertLength number of bytes to copy
- Returns
- length of inserted data
Reimplemented in Okteta::PieceTableByteArrayModel, Okteta::ByteArrayModel, Okteta::FixedSizeByteArrayModel, and Okteta::FileByteArrayModel.
Definition at line 47 of file abstractbytearraymodel.cpp.
|
inline |
Definition at line 267 of file abstractbytearraymodel.h.
|
pure virtual |
- Returns
true
if the buffer has been modified,false
otherwise
Implemented in Okteta::PieceTableByteArrayModel, Okteta::ByteArrayModel, Okteta::FixedSizeByteArrayModel, and Okteta::FileByteArrayModel.
|
virtual |
Default returns true
.
- Returns
true
if the buffer can only be read,false
if writing is allowed
Reimplemented in Okteta::PieceTableByteArrayModel, Okteta::ByteArrayModel, Okteta::FixedSizeByteArrayModel, and Okteta::FileByteArrayModel.
Definition at line 40 of file abstractbytearraymodel.cpp.
|
virtual |
searches for a given data string The section limits the data within which the key has to be found If the end of the section is lower than the start the search continues at the start???
- Parameters
-
param Length length of search string Section section within the keydata is to be found
- Returns
- index of the first occurrence or -1searches backward beginning with byte at Pos.
- Parameters
-
pattern patternLength length of search string fromOffset the position to start the search. If -1 the search starts at the end.
- Returns
- index of the first or -1
Definition at line 104 of file abstractbytearraymodel.cpp.
|
inline |
Definition at line 289 of file abstractbytearraymodel.h.
Address Okteta::AbstractByteArrayModel::lastIndexOfCaseInsensitive | ( | const CharCodec * | charCodec, |
const QByteArray & | pattern, | ||
Address | fromOffset = -1 , |
||
Address | toOffset = 0 |
||
) | const |
Definition at line 207 of file abstractbytearraymodel.cpp.
|
signal |
|
signal |
|
virtual |
removes beginning with position as much as possible
- Parameters
-
removeRange
- Returns
- length of removed data
Reimplemented in Okteta::PieceTableByteArrayModel, Okteta::ByteArrayModel, Okteta::FixedSizeByteArrayModel, and Okteta::FileByteArrayModel.
Definition at line 53 of file abstractbytearraymodel.cpp.
convenience function, behaves as above
Definition at line 270 of file abstractbytearraymodel.h.
|
pure virtual |
replaces as much as possible
- Parameters
-
removeRange insertData insertLength
- Returns
- length of inserted data
Implemented in Okteta::PieceTableByteArrayModel, Okteta::ByteArrayModel, Okteta::FixedSizeByteArrayModel, and Okteta::FileByteArrayModel.
|
inline |
convenience function, behaves as above
Definition at line 273 of file abstractbytearraymodel.h.
|
inline |
convenience function, behaves as above
Definition at line 276 of file abstractbytearraymodel.h.
|
signal |
sets a single byte if the offset is not valid the behaviour is undefined
- Parameters
-
offset offset of the datum requested byte new byte value
Implemented in Okteta::PieceTableByteArrayModel, Okteta::ByteArrayModel, Okteta::FixedSizeByteArrayModel, and Okteta::FileByteArrayModel.
|
pure virtual |
sets the modified flag for the buffer
- Parameters
-
modified
Implemented in Okteta::PieceTableByteArrayModel, Okteta::ByteArrayModel, Okteta::FixedSizeByteArrayModel, and Okteta::FileByteArrayModel.
|
virtual |
sets the readonly flag for the byte array if this is possible.
Default implementation does not do anything.
- Parameters
-
isReadOnly new state
Reimplemented in Okteta::PieceTableByteArrayModel, Okteta::ByteArrayModel, Okteta::FileByteArrayModel, and Okteta::FixedSizeByteArrayModel.
Definition at line 42 of file abstractbytearraymodel.cpp.
|
pure virtual |
- Returns
- the size of the data
Implemented in Okteta::PieceTableByteArrayModel, Okteta::ByteArrayModel, Okteta::FixedSizeByteArrayModel, and Okteta::FileByteArrayModel.
|
pure virtual |
moves the second section before the start of the first which is the same as moving the first behind the second.
- Parameters
-
firstStart position of the data where the section should be moved behind secondRange data range to be moved
- Returns
- if operation was successful, otherwise
Implemented in Okteta::PieceTableByteArrayModel, Okteta::ByteArrayModel, Okteta::FixedSizeByteArrayModel, and Okteta::FileByteArrayModel.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:04:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.