okteta
#include <bytearraymodel.h>
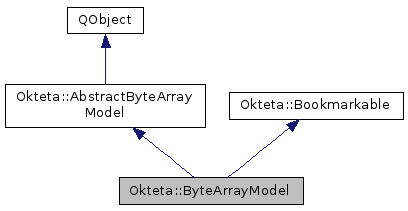
Signals | |
void | bookmarksAdded (const QList< Okteta::Bookmark > &bookmarks) |
void | bookmarksModified (bool modified) |
void | bookmarksModified (const QList< int > &indizes) |
void | bookmarksRemoved (const QList< Okteta::Bookmark > &bookmarks) |
![]() | |
void | contentsChanged (const Okteta::ArrayChangeMetricsList &changeList) |
void | modifiedChanged (bool isModified) |
void | readOnlyChanged (bool isReadOnly) |
void | searchedBytes (Okteta::Size bytes) const |
Public Member Functions | |
ByteArrayModel (Byte *data, int size, int rawSize=-1, bool keepsMemory=true, QObject *parent=0) | |
ByteArrayModel (const Byte *data, int size, QObject *parent=0) | |
ByteArrayModel (int size=0, int maxSize=-1, QObject *parent=0) | |
virtual | ~ByteArrayModel () |
virtual void | addBookmarks (const QList< Okteta::Bookmark > &bookmarks) |
bool | autoDelete () const |
virtual const Okteta::Bookmark & | bookmarkAt (unsigned int index) const |
virtual const Okteta::Bookmark & | bookmarkFor (int offset) const |
virtual unsigned int | bookmarksCount () const |
virtual Byte | byte (Address offset) const |
virtual bool | containsBookmarkFor (int offset) const |
virtual Okteta::BookmarksConstIterator | createBookmarksConstIterator () const |
Byte * | data () const |
virtual Size | fill (Byte fillByte, Address offset=0, Size fillLength=-1) |
virtual Size | insert (Address offset, const Byte *insertData, int insertLength) |
virtual bool | isModified () const |
virtual bool | isReadOnly () const |
bool | keepsMemory () const |
int | maxSize () const |
virtual Size | remove (const AddressRange &removeRange) |
virtual void | removeAllBookmarks () |
virtual void | removeBookmarks (const QList< Okteta::Bookmark > &bookmarks) |
virtual Size | replace (const AddressRange &removeRange, const Byte *insertData, int insertLength) |
void | setAutoDelete (bool autoDelete=true) |
virtual void | setBookmark (unsigned int index, const Okteta::Bookmark &bookmark) |
virtual void | setByte (Address offset, Byte byte) |
void | setData (Byte *data, int size, int rawSize=-1, bool keepsMemory=true) |
void | setKeepsMemory (bool keepsMemory=true) |
void | setMaxSize (int maxSize) |
virtual void | setModified (bool modified=true) |
virtual void | setReadOnly (bool isReadOnly=true) |
void | signalContentsChanged (int i1, int i2) |
virtual Size | size () const |
virtual bool | swap (Address firstStart, const AddressRange &secondRange) |
![]() | |
virtual | ~AbstractByteArrayModel () |
virtual Size | copyTo (Byte *dest, const AddressRange ©Range) const |
Size | copyTo (Byte *dest, Address offset, Size copyLength) const |
Size | fill (Byte fillChar, const AddressRange &fillRange) |
virtual Address | indexOf (const Byte *pattern, int patternLength, Address fromOffset=0, Address toOffset=-1) const |
Address | indexOf (const QByteArray &pattern, Address fromOffset=0, Address toOffset=-1) const |
Address | indexOfCaseInsensitive (const CharCodec *charCodec, const QByteArray &pattern, Address fromOffset=0, Address toOffset=-1) const |
Size | insert (Address offset, const QByteArray &insertData) |
virtual Address | lastIndexOf (const Byte *pattern, int patternLength, Address fromOffset=-1, Address toOffset=0) const |
Address | lastIndexOf (const QByteArray &pattern, Address fromOffset=-1, Address toOffset=0) const |
Address | lastIndexOfCaseInsensitive (const CharCodec *charCodec, const QByteArray &pattern, Address fromOffset=-1, Address toOffset=0) const |
Size | remove (Address offset, Size removeLength) |
Size | replace (const AddressRange &removeRange, const QByteArray &insertData) |
Size | replace (Address offset, Size removeLength, const Byte *insertData, Size insertLength) |
![]() | |
virtual | ~Bookmarkable () |
virtual void | bookmarksAdded (const QList< Okteta::Bookmark > &bookmarks)=0 |
virtual void | bookmarksModified (const QList< int > &indizes)=0 |
virtual void | bookmarksModified (bool modified)=0 |
virtual void | bookmarksRemoved (const QList< Okteta::Bookmark > &bookmarks)=0 |
Protected Attributes | |
ByteArrayModelPrivate *const | d |
Additional Inherited Members | |
![]() | |
AbstractByteArrayModel (QObject *parent=0) | |
Detailed Description
Definition at line 41 of file bytearraymodel.h.
Constructor & Destructor Documentation
Okteta::ByteArrayModel::ByteArrayModel | ( | Byte * | data, |
int | size, | ||
int | rawSize = -1 , |
||
bool | keepsMemory = true , |
||
QObject * | parent = 0 |
||
) |
Definition at line 33 of file bytearraymodel.cpp.
Definition at line 38 of file bytearraymodel.cpp.
|
explicit |
Definition at line 43 of file bytearraymodel.cpp.
|
virtual |
Definition at line 153 of file bytearraymodel.cpp.
Member Function Documentation
|
virtual |
Implements Okteta::Bookmarkable.
Definition at line 108 of file bytearraymodel.cpp.
bool Okteta::ByteArrayModel::autoDelete | ( | ) | const |
Definition at line 69 of file bytearraymodel.cpp.
|
virtual |
Implements Okteta::Bookmarkable.
Definition at line 138 of file bytearraymodel.cpp.
|
virtual |
Implements Okteta::Bookmarkable.
Definition at line 133 of file bytearraymodel.cpp.
|
signal |
|
virtual |
Implements Okteta::Bookmarkable.
Definition at line 148 of file bytearraymodel.cpp.
|
signal |
|
signal |
|
signal |
locates working range The idea behind is to tell buffer which range will be requested in the following time, so that e.g.
the corresponding pages will be loaded in advance TODO: do we really need this? Where will this lead to real enhancements?
- Parameters
-
Range
- Returns
true
if successfully,false
otherwiseconvenience function, same as above creates an iterator to expects pointer to memory, should be in prepared range it is only expected to be a valid pointer until any further call to this or any modifying function
- Parameters
-
Section
- Returns
- pointer to convenience function, same as above requests a single byte if the offset is not valid the behaviour is undefined
- Parameters
-
offset offset of the datum requested
Implements Okteta::AbstractByteArrayModel.
Definition at line 49 of file bytearraymodel.cpp.
|
virtual |
Implements Okteta::Bookmarkable.
Definition at line 143 of file bytearraymodel.cpp.
|
virtual |
Implements Okteta::Bookmarkable.
Definition at line 128 of file bytearraymodel.cpp.
Byte * Okteta::ByteArrayModel::data | ( | ) | const |
Definition at line 66 of file bytearraymodel.cpp.
|
virtual |
fills the buffer with the FillChar.
If the buffer is to small it will be extended as much as possible.
- Parameters
-
fillByte byte to use offset position where the filling starts fillLength number of bytes to fill. If Length is -1, the buffer is filled till the end.
- Returns
- number of filled bytes
Implements Okteta::AbstractByteArrayModel.
Definition at line 103 of file bytearraymodel.cpp.
|
virtual |
inserts bytes copied from the given source at Position.
The original data beginnung at the position is moved with the buffer enlarged as needed If the buffer is readOnly this is a noop and returns 0.
- Parameters
-
offset insertData data source insertLength number of bytes to copy
- Returns
- length of inserted data
Reimplemented from Okteta::AbstractByteArrayModel.
Definition at line 83 of file bytearraymodel.cpp.
|
virtual |
- Returns
true
if the buffer has been modified,false
otherwise
Implements Okteta::AbstractByteArrayModel.
Definition at line 53 of file bytearraymodel.cpp.
|
virtual |
Default returns true
.
- Returns
true
if the buffer can only be read,false
if writing is allowed
Reimplemented from Okteta::AbstractByteArrayModel.
Definition at line 52 of file bytearraymodel.cpp.
bool Okteta::ByteArrayModel::keepsMemory | ( | ) | const |
returns whether the memory of the byte array is kept on resize
Definition at line 68 of file bytearraymodel.cpp.
int Okteta::ByteArrayModel::maxSize | ( | ) | const |
Definition at line 67 of file bytearraymodel.cpp.
|
virtual |
removes beginning with position as much as possible
- Parameters
-
removeRange
- Returns
- length of removed data
Reimplemented from Okteta::AbstractByteArrayModel.
Definition at line 88 of file bytearraymodel.cpp.
|
virtual |
Implements Okteta::Bookmarkable.
Definition at line 118 of file bytearraymodel.cpp.
|
virtual |
Implements Okteta::Bookmarkable.
Definition at line 113 of file bytearraymodel.cpp.
|
virtual |
replaces as much as possible
- Parameters
-
removeRange insertData insertLength
- Returns
- length of inserted data
Implements Okteta::AbstractByteArrayModel.
Definition at line 93 of file bytearraymodel.cpp.
void Okteta::ByteArrayModel::setAutoDelete | ( | bool | autoDelete = true | ) |
sets whether the memory given by setData or in the constructor gets deleted in destructor or when new data is set.
The data MUST have been allocated using new[] otherwise behaviour is undefined
Definition at line 59 of file bytearraymodel.cpp.
|
virtual |
Implements Okteta::Bookmarkable.
Definition at line 123 of file bytearraymodel.cpp.
sets a single byte if the offset is not valid the behaviour is undefined
- Parameters
-
offset offset of the datum requested byte new byte value
Implements Okteta::AbstractByteArrayModel.
Definition at line 78 of file bytearraymodel.cpp.
void Okteta::ByteArrayModel::setData | ( | Byte * | data, |
int | size, | ||
int | rawSize = -1 , |
||
bool | keepsMemory = true |
||
) |
Definition at line 61 of file bytearraymodel.cpp.
void Okteta::ByteArrayModel::setKeepsMemory | ( | bool | keepsMemory = true | ) |
sets whether the memory given by setData or in the constructor should be kept on resize
Definition at line 58 of file bytearraymodel.cpp.
void Okteta::ByteArrayModel::setMaxSize | ( | int | maxSize | ) |
Definition at line 57 of file bytearraymodel.cpp.
|
virtual |
sets the modified flag for the buffer
- Parameters
-
modified
Implements Okteta::AbstractByteArrayModel.
Definition at line 56 of file bytearraymodel.cpp.
|
virtual |
sets the readonly flag for the byte array if this is possible.
Default implementation does not do anything.
- Parameters
-
isReadOnly new state
Reimplemented from Okteta::AbstractByteArrayModel.
Definition at line 55 of file bytearraymodel.cpp.
void Okteta::ByteArrayModel::signalContentsChanged | ( | int | i1, |
int | i2 | ||
) |
Definition at line 71 of file bytearraymodel.cpp.
|
virtual |
- Returns
- the size of the data
Implements Okteta::AbstractByteArrayModel.
Definition at line 50 of file bytearraymodel.cpp.
|
virtual |
moves the second section before the start of the first which is the same as moving the first behind the second.
- Parameters
-
firstStart position of the data where the section should be moved behind secondRange data range to be moved
- Returns
- if operation was successful, otherwise
Implements Okteta::AbstractByteArrayModel.
Definition at line 98 of file bytearraymodel.cpp.
Member Data Documentation
|
protected |
Definition at line 112 of file bytearraymodel.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:04:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.