okteta
#include <abstractbytearrayview.h>
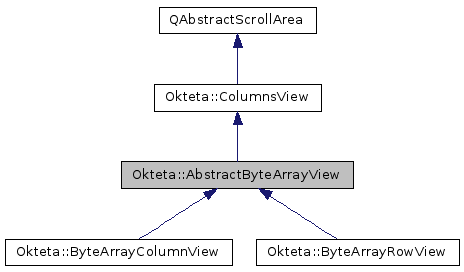
Public Types | |
enum | CharCoding { LocalEncoding =0, ISO8859_1Encoding =1, EBCDIC1047Encoding =2, StartOfOwnEncoding =0x8000, MaxEncodingId =0xFFFF } |
enum | CodingTypeId { NoCodingId =0, ValueCodingId =1, CharCodingId =2 } |
enum | CodingTypes { OnlyValueCoding = ValueCodingId, OnlyCharCoding = CharCodingId, ValueAndCharCodings =ValueCodingId|CharCodingId } |
enum | LayoutStyle { FixedLayoutStyle =0, WrapOnlyByteGroupsLayoutStyle =1, FullSizeLayoutStyle =2, LastUserLayout =0xFF } |
enum | OffsetCoding { HexadecimalOffset =0, DecimalOffset =1, MaxOffsetCodingId =0xFF } |
enum | ValueCoding { HexadecimalCoding =0, DecimalCoding =1, OctalCoding =2, BinaryCoding =3, MaxCodingId =0xFFFF } |
Signals | |
void | charCodecChanged (const QString &codecName) |
void | clicked (Okteta::Address index) |
void | copyAvailable (bool Really) |
void | cursorPositionChanged (Okteta::Address index) |
void | cutAvailable (bool Really) |
void | doubleClicked (Okteta::Address index) |
void | focusChanged (bool hasFocus) |
void | hasSelectedDataChanged (bool hasSelectedData) |
void | layoutStyleChanged (int layoutStyle) |
void | noOfBytesPerLineChanged (int noOfBytesPerLine) |
void | noOfGroupedBytesChanged (int noOfGroupedBytes) |
void | offsetCodingChanged (int offsetCoding) |
void | offsetColumnVisibleChanged (bool visible) |
void | overwriteModeChanged (bool newOverwriteMode) |
void | readOnlyChanged (bool isReadOnly) |
void | selectionChanged (const Okteta::AddressRange &selection) |
void | showsNonprintingChanged (bool showsNonprinting) |
void | substituteCharChanged (QChar substituteChar) |
void | undefinedCharChanged (QChar undefinedChar) |
void | valueCodingChanged (int valueCoding) |
void | visibleByteArrayCodingsChanged (int columns) |
Protected Member Functions | |
AbstractByteArrayView (AbstractByteArrayViewPrivate *d, QWidget *parent) | |
virtual void | changeEvent (QEvent *event) |
const Okteta::CharCodec * | charCodec () const |
void | copyToClipboard (QClipboard::Mode mode) const |
virtual void | dragEnterEvent (QDragEnterEvent *dragEnterEvent) |
virtual void | dragLeaveEvent (QDragLeaveEvent *dragLeaveEvent) |
virtual void | dragMoveEvent (QDragMoveEvent *dragMoveEvent) |
virtual void | dropEvent (QDropEvent *dropEvent) |
void | emitSelectionSignals () |
virtual bool | event (QEvent *event) |
void | finishByteEdit () |
virtual void | focusInEvent (QFocusEvent *focusEvent) |
virtual void | focusOutEvent (QFocusEvent *focusEvent) |
virtual void | keyPressEvent (QKeyEvent *keyEvent) |
ByteArrayTableLayout * | layout () const |
virtual void | mouseDoubleClickEvent (QMouseEvent *mouseDoubleClickEvent) |
virtual void | mouseMoveEvent (QMouseEvent *mouseMoveEvent) |
virtual void | mousePressEvent (QMouseEvent *mousePressEvent) |
virtual void | mouseReleaseEvent (QMouseEvent *mouseReleaseEvent) |
void | pasteFromClipboard (QClipboard::Mode mode) |
virtual void | resizeEvent (QResizeEvent *resizeEvent) |
virtual void | setNoOfLines (int newNoOfLines) |
virtual void | showEvent (QShowEvent *showEvent) |
ByteArrayTableCursor * | tableCursor () const |
ByteArrayTableRanges * | tableRanges () const |
void | updateChanged () |
const Okteta::ValueCodec * | valueCodec () const |
virtual bool | viewportEvent (QEvent *event) |
virtual void | wheelEvent (QWheelEvent *e) |
![]() | |
void | addColumn (AbstractColumnRenderer *columnRenderer) |
virtual void | paintEvent (QPaintEvent *paintEvent) |
void | removeColumn (AbstractColumnRenderer *columnRenderer) |
virtual void | renderColumns (QPainter *painter, int cx, int cy, int cw, int ch) |
virtual void | renderEmptyArea (QPainter *painter, int cx, int cy, int cw, int ch) |
virtual void | scrollContentsBy (int dx, int dy) |
virtual void | setLineHeight (PixelY lineHeight) |
virtual void | setNoOfLines (LineSize noOfLines) |
void | updateColumn (AbstractColumnRenderer &columnRenderer) |
void | updateColumn (AbstractColumnRenderer &columnRenderer, const LineRange &lines) |
void | updateScrollBars () |
void | updateWidths () |
Protected Attributes | |
AbstractByteArrayViewPrivate *const | d_ptr |
Properties | |
int | BinaryGapWidth |
int | ByteSpacingWidth |
bool | ByteTypeColored |
ValueCoding | Coding |
int | FirstLineOffset |
int | GroupSpacingWidth |
LayoutStyle | LayoutStyle |
bool | Modified |
int | NoOfBytesPerLine |
int | NoOfGroupedBytes |
OffsetCoding | OffsetCoding |
bool | OffsetColumnVisible |
bool | OverwriteMode |
bool | OverwriteOnly |
bool | ReadOnly |
bool | ShowNonprinting |
int | StartOffset |
QChar | SubstituteChar |
bool | TabChangesFocus |
CodingTypes | VisibleCodings |
Detailed Description
Definition at line 55 of file abstractbytearrayview.h.
Member Enumeration Documentation
Enumerator | |
---|---|
LocalEncoding | |
ISO8859_1Encoding | |
EBCDIC1047Encoding | |
StartOfOwnEncoding | |
MaxEncodingId |
Definition at line 99 of file abstractbytearrayview.h.
Enumerator | |
---|---|
NoCodingId | |
ValueCodingId | |
CharCodingId |
Definition at line 103 of file abstractbytearrayview.h.
Enumerator | |
---|---|
OnlyValueCoding | |
OnlyCharCoding | |
ValueAndCharCodings |
Definition at line 104 of file abstractbytearrayview.h.
Enumerator | |
---|---|
FixedLayoutStyle | |
WrapOnlyByteGroupsLayoutStyle | |
FullSizeLayoutStyle | |
LastUserLayout |
Definition at line 101 of file abstractbytearrayview.h.
Enumerator | |
---|---|
HexadecimalOffset | |
DecimalOffset | |
MaxOffsetCodingId |
Definition at line 97 of file abstractbytearrayview.h.
Enumerator | |
---|---|
HexadecimalCoding | |
DecimalCoding | |
OctalCoding | |
BinaryCoding | |
MaxCodingId |
Definition at line 98 of file abstractbytearrayview.h.
Constructor & Destructor Documentation
|
virtual |
Definition at line 633 of file abstractbytearrayview.cpp.
|
protected |
Definition at line 36 of file abstractbytearrayview.cpp.
Member Function Documentation
AbstractByteArrayView::CodingTypeId Okteta::AbstractByteArrayView::activeCoding | ( | ) | const |
Definition at line 297 of file abstractbytearrayview.cpp.
|
pure virtual |
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
Okteta::AbstractByteArrayModel * Okteta::AbstractByteArrayView::byteArrayModel | ( | ) | const |
Definition at line 44 of file abstractbytearrayview.cpp.
|
pure virtual |
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
bool Okteta::AbstractByteArrayView::canReadData | ( | const QMimeData * | data | ) | const |
Definition at line 196 of file abstractbytearrayview.cpp.
|
protectedvirtual |
Reimplemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
Definition at line 468 of file abstractbytearrayview.cpp.
|
protected |
Definition at line 100 of file abstractbytearrayview.cpp.
|
signal |
AbstractByteArrayView::CharCoding Okteta::AbstractByteArrayView::charCoding | ( | ) | const |
- Returns
- encoding used for the chars
Definition at line 90 of file abstractbytearrayview.cpp.
const QString & Okteta::AbstractByteArrayView::charCodingName | ( | ) | const |
- Returns
- name of the encoding used for the chars
Definition at line 95 of file abstractbytearrayview.cpp.
|
signal |
Index of the byte that was clicked.
|
virtual |
Definition at line 208 of file abstractbytearrayview.cpp.
|
signal |
there is a copy available or not
|
protected |
Definition at line 511 of file abstractbytearrayview.cpp.
Address Okteta::AbstractByteArrayView::cursorPosition | ( | ) | const |
returns the index of the cursor position
Definition at line 133 of file abstractbytearrayview.cpp.
|
signal |
QRect Okteta::AbstractByteArrayView::cursorRect | ( | ) | const |
Definition at line 523 of file abstractbytearrayview.cpp.
|
virtual |
Definition at line 202 of file abstractbytearrayview.cpp.
|
signal |
there is a cut available or not
|
signal |
Index of the byte that was double clicked.
|
protectedvirtual |
Definition at line 605 of file abstractbytearrayview.cpp.
|
protectedvirtual |
Definition at line 615 of file abstractbytearrayview.cpp.
|
protectedvirtual |
Definition at line 610 of file abstractbytearrayview.cpp.
|
protectedvirtual |
Definition at line 620 of file abstractbytearrayview.cpp.
|
protected |
Definition at line 494 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::ensureCursorVisible | ( | ) |
scrolls the view as much as needed to have the cursor fully visible
Definition at line 390 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::ensureVisible | ( | const AddressRange & | range, |
bool | ensureStartVisible = false |
||
) |
- Parameters
-
ensureStartVisible if true , otherwise the end
Definition at line 383 of file abstractbytearrayview.cpp.
|
protectedvirtual |
reimplemented to catch Tab and BackTab keys, which otherwise gets stolen
Reimplemented from Okteta::ColumnsView.
Definition at line 530 of file abstractbytearrayview.cpp.
|
protected |
Definition at line 487 of file abstractbytearrayview.cpp.
Address Okteta::AbstractByteArrayView::firstLineOffset | ( | ) | const |
Definition at line 122 of file abstractbytearrayview.cpp.
|
signal |
|
protectedvirtual |
Definition at line 549 of file abstractbytearrayview.cpp.
|
protectedvirtual |
Definition at line 556 of file abstractbytearrayview.cpp.
|
pure virtual |
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
bool Okteta::AbstractByteArrayView::hasSelectedData | ( | ) | const |
returns true if there is a selected range in the array
Definition at line 166 of file abstractbytearrayview.cpp.
|
signal |
selection has changed
Address Okteta::AbstractByteArrayView::indexByPoint | ( | const QPoint & | point | ) | const |
detects the index of the byte at the given point
- Parameters
-
point in viewport coordinate system
- Returns
- index of the byte that covers the point
Definition at line 480 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::insert | ( | const QByteArray & | data | ) |
inserts
Definition at line 220 of file abstractbytearrayview.cpp.
|
pure virtual |
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
bool Okteta::AbstractByteArrayView::isCursorBehind | ( | ) | const |
Definition at line 138 of file abstractbytearrayview.cpp.
bool Okteta::AbstractByteArrayView::isModified | ( | ) | const |
Definition at line 64 of file abstractbytearrayview.cpp.
bool Okteta::AbstractByteArrayView::isOverwriteMode | ( | ) | const |
Definition at line 49 of file abstractbytearrayview.cpp.
bool Okteta::AbstractByteArrayView::isOverwriteOnly | ( | ) | const |
Definition at line 54 of file abstractbytearrayview.cpp.
bool Okteta::AbstractByteArrayView::isReadOnly | ( | ) | const |
Definition at line 59 of file abstractbytearrayview.cpp.
|
protectedvirtual |
Definition at line 563 of file abstractbytearrayview.cpp.
|
protected |
Definition at line 75 of file abstractbytearrayview.cpp.
AbstractByteArrayView::LayoutStyle Okteta::AbstractByteArrayView::layoutStyle | ( | ) | const |
Definition at line 143 of file abstractbytearrayview.cpp.
|
signal |
AddressRange Okteta::AbstractByteArrayView::marking | ( | ) | const |
Definition at line 183 of file abstractbytearrayview.cpp.
|
protectedvirtual |
Definition at line 592 of file abstractbytearrayview.cpp.
|
protectedvirtual |
Definition at line 577 of file abstractbytearrayview.cpp.
|
protectedvirtual |
Definition at line 570 of file abstractbytearrayview.cpp.
|
protectedvirtual |
Definition at line 584 of file abstractbytearrayview.cpp.
int Okteta::AbstractByteArrayView::noOfBytesPerLine | ( | ) | const |
Definition at line 117 of file abstractbytearrayview.cpp.
|
signal |
|
pure virtual |
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
|
signal |
AbstractByteArrayView::OffsetCoding Okteta::AbstractByteArrayView::offsetCoding | ( | ) | const |
Definition at line 155 of file abstractbytearrayview.cpp.
|
signal |
bool Okteta::AbstractByteArrayView::offsetColumnVisible | ( | ) | const |
Definition at line 149 of file abstractbytearrayview.cpp.
|
signal |
|
signal |
|
virtual |
Definition at line 214 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::pasteData | ( | const QMimeData * | data | ) |
Definition at line 190 of file abstractbytearrayview.cpp.
|
protected |
Definition at line 517 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::pauseCursor | ( | ) |
simply pauses any blinking, i.e.
ignores any calls to blinkCursor
Definition at line 421 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::placeCursor | ( | const QPoint & | point | ) |
puts the cursor in the column at the pos of Point (in absolute coord), does not handle the drawing
Definition at line 396 of file abstractbytearrayview.cpp.
|
signal |
void Okteta::AbstractByteArrayView::removeSelectedData | ( | ) |
removes the selected data, takes care of the cursor
Definition at line 226 of file abstractbytearrayview.cpp.
|
protectedvirtual |
Reimplemented from Okteta::ColumnsView.
Definition at line 543 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::selectAll | ( | bool | select | ) |
de-/selects all data
Definition at line 333 of file abstractbytearrayview.cpp.
QByteArray Okteta::AbstractByteArrayView::selectedData | ( | ) | const |
- Returns
- deep copy of the selected data
Definition at line 172 of file abstractbytearrayview.cpp.
AddressRange Okteta::AbstractByteArrayView::selection | ( | ) | const |
Definition at line 161 of file abstractbytearrayview.cpp.
QMimeData * Okteta::AbstractByteArrayView::selectionAsMimeData | ( | ) | const |
Definition at line 177 of file abstractbytearrayview.cpp.
|
signal |
bool Okteta::AbstractByteArrayView::selectWord | ( | Address | index | ) |
selects word at index, returns true if there is one
Definition at line 339 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::setActiveCoding | ( | CodingTypeId | codingId | ) |
Definition at line 308 of file abstractbytearrayview.cpp.
|
pure virtual |
sets the spacing in the middle of a binary byte in the value column
- Parameters
-
binaryGapWidth spacing in the middle of a binary in pixels returns true if there was a change
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
|
pure virtual |
sets the spacing in the value column
- Parameters
-
byteSpacingWidth spacing between the bytes in pixels noOfGroupedBytes numbers of grouped bytes, 0 means no grouping groupSpacingWidth spacing between the groups in pixels Default is 4 for NoOfGroupedBytes
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
|
virtual |
Reimplemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
Definition at line 232 of file abstractbytearrayview.cpp.
|
pure virtual |
sets the spacing between the bytes in the value column
- Parameters
-
byteSpacingWidth spacing between the bytes in pixels default is 3
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
|
pure virtual |
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
|
pure virtual |
sets the encoding of the char column.
Default is Okteta::LocalEncoding. If the encoding is not available the format will not be changed.
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
|
pure virtual |
sets the encoding of the char column.
Default is Okteta::LocalEncoding. If the encoding is not available the format will not be changed.
- Parameters
-
charCodingName name of the encoding
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
void Okteta::AbstractByteArrayView::setCursorPosition | ( | Address | index, |
bool | isBehind = false |
||
) |
puts the cursor to the position of index, handles all drawing
- Parameters
-
index isBehind
Definition at line 345 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::setFirstLineOffset | ( | Address | firstLineOffset | ) |
sets offset of the char in the upper left corner
Definition at line 275 of file abstractbytearrayview.cpp.
|
pure virtual |
sets the spacing between the groups of bytes in the value column
- Parameters
-
groupSpacingWidth spacing between the groups in pixels default is 9
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
void Okteta::AbstractByteArrayView::setLayoutStyle | ( | LayoutStyle | layoutStyle | ) |
sets the resizestyle for the value column.
Default is Okteta::FixedLayoutStyle
Definition at line 256 of file abstractbytearrayview.cpp.
Definition at line 370 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::setMarking | ( | const AddressRange & | marking | ) |
Definition at line 376 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::setModified | ( | bool | modified | ) |
sets whether the data should be treated modified or not
Definition at line 238 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::setNoOfBytesPerLine | ( | int | noOfBytesPerLine | ) |
sets the number of bytes per line, switching the resize style to Okteta::FixedLayoutStyle
Definition at line 262 of file abstractbytearrayview.cpp.
|
pure virtual |
sets the number of grouped bytes in the value column
- Parameters
-
noOfGroupedBytes numbers of grouped bytes, 0 means no grouping default is 4
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
|
protectedvirtual |
Definition at line 463 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::setOffsetCoding | ( | AbstractByteArrayView::OffsetCoding | offsetCoding | ) |
sets the format of the offset column.
Default is Okteta::HexadecimalCoding
Definition at line 326 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::setOverwriteMode | ( | bool | overwriteMode | ) |
sets whether the widget is in overwrite mode or not.
Default is true.
Definition at line 244 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::setOverwriteOnly | ( | bool | overwriteOnly | ) |
sets whether the widget is overwriteonly or not.
Default is false.
Definition at line 250 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::setReadOnly | ( | bool | readOnly | ) |
sets whether the widget is readonly or not, Default is true.
If the databuffer which is worked on can't be written the widget stays readonly
Definition at line 281 of file abstractbytearrayview.cpp.
Definition at line 357 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::setSelection | ( | const AddressRange & | selection | ) |
Definition at line 363 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::setSelectionCursorPosition | ( | Address | index | ) |
Definition at line 351 of file abstractbytearrayview.cpp.
|
pure virtual |
sets whether control chars or "non-printing" chars should be displayed in the char column with their corresponding character.
Currently this simply means all chars with value <32, as known from the ASCII.
- Parameters
-
showsNonprinting returns true if there was a change
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
void Okteta::AbstractByteArrayView::setStartOffset | ( | Address | startOffset | ) |
sets absolut offset of the data
Definition at line 269 of file abstractbytearrayview.cpp.
|
pure virtual |
sets the substitute character for "non-printing" chars returns true if there was a change
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
void Okteta::AbstractByteArrayView::setTabChangesFocus | ( | bool | tabChangesFocus = true | ) |
sets whether on a tab key there should be switched from the char column back to the value column or be switched to the next focusable widget.
Default is false
Definition at line 314 of file abstractbytearrayview.cpp.
|
pure virtual |
sets the undefined character for "undefined" chars returns true if there was a change
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
|
pure virtual |
sets the format of the value column.
Default is Okteta::HexadecimalCoding
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
void Okteta::AbstractByteArrayView::setVisibleCodings | ( | int | visibleCodings | ) |
Definition at line 303 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::setZoomLevel | ( | double | level | ) |
Definition at line 457 of file abstractbytearrayview.cpp.
|
protectedvirtual |
Definition at line 536 of file abstractbytearrayview.cpp.
|
pure virtual |
reports if "non-printing" chars are displayed in the char column with their original character.
Default is false
- Returns
true
if original chars are displayed, otherwisefalse
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
|
signal |
|
virtual |
Definition at line 474 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::startCursor | ( | ) |
we have focus again, start the timer
Definition at line 403 of file abstractbytearrayview.cpp.
Address Okteta::AbstractByteArrayView::startOffset | ( | ) | const |
Definition at line 127 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::stopCursor | ( | ) |
we lost focus, stop the timer
Definition at line 415 of file abstractbytearrayview.cpp.
|
pure virtual |
gives the used substitute character for "unprintable" chars, default is '.
'
- Returns
- substitute character
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
|
signal |
bool Okteta::AbstractByteArrayView::tabChangesFocus | ( | ) | const |
Definition at line 292 of file abstractbytearrayview.cpp.
|
protected |
Definition at line 106 of file abstractbytearrayview.cpp.
|
protected |
Definition at line 111 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::toggleOffsetColumn | ( | bool | offsetColumnVisible | ) |
switches the Offset column on/off
Definition at line 320 of file abstractbytearrayview.cpp.
|
pure virtual |
returns the actually used undefined character for "undefined" chars, default is '?'
Implemented in Okteta::ByteArrayColumnView, and Okteta::ByteArrayRowView.
|
signal |
void Okteta::AbstractByteArrayView::unpauseCursor | ( | ) |
undoes pauseCursor
Definition at line 409 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::unZoom | ( | ) |
Definition at line 452 of file abstractbytearrayview.cpp.
|
protected |
Definition at line 505 of file abstractbytearrayview.cpp.
|
protected |
Definition at line 85 of file abstractbytearrayview.cpp.
AbstractByteArrayView::ValueCoding Okteta::AbstractByteArrayView::valueCoding | ( | ) | const |
Definition at line 80 of file abstractbytearrayview.cpp.
|
signal |
|
protectedvirtual |
Definition at line 626 of file abstractbytearrayview.cpp.
|
signal |
AbstractByteArrayView::CodingTypes Okteta::AbstractByteArrayView::visibleCodings | ( | ) | const |
Definition at line 287 of file abstractbytearrayview.cpp.
|
protectedvirtual |
Definition at line 598 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::zoomIn | ( | int | pointInc | ) |
Definition at line 427 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::zoomIn | ( | ) |
Definition at line 432 of file abstractbytearrayview.cpp.
double Okteta::AbstractByteArrayView::zoomLevel | ( | ) | const |
Definition at line 70 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::zoomOut | ( | int | pointDec | ) |
Definition at line 437 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::zoomOut | ( | ) |
Definition at line 442 of file abstractbytearrayview.cpp.
void Okteta::AbstractByteArrayView::zoomTo | ( | int | pointSize | ) |
Definition at line 447 of file abstractbytearrayview.cpp.
Member Data Documentation
|
protected |
Definition at line 433 of file abstractbytearrayview.h.
Property Documentation
|
readwrite |
Definition at line 91 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 88 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 85 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 87 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 79 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 90 of file abstractbytearrayview.h.
Definition at line 76 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 70 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 77 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 89 of file abstractbytearrayview.h.
Definition at line 81 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 80 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 68 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 69 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 71 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 93 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 78 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 94 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 75 of file abstractbytearrayview.h.
|
readwrite |
Definition at line 82 of file abstractbytearrayview.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:04:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.