okteta
#include <taggeduniondatainformation.h>
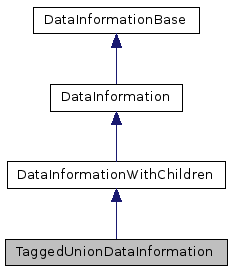
Classes | |
struct | FieldInfo |
Public Member Functions | |
TaggedUnionDataInformation (const QString &name, DataInformation *parent=0) | |
virtual | ~TaggedUnionDataInformation () |
void | appendDefaultField (DataInformation *field, bool emitSignal) |
virtual DataInformation * | childAt (unsigned int index) const |
virtual unsigned int | childCount () const |
virtual BitCount64 | childPosition (const DataInformation *child, Okteta::Address start) const |
virtual int | indexOf (const DataInformation *const data) const |
virtual bool | isTaggedUnion () const |
virtual qint64 | readData (Okteta::AbstractByteArrayModel *input, Okteta::Address address, BitCount64 bitsRemaining, quint8 *bitOffset) |
virtual bool | replaceChildAt (unsigned int index, DataInformation *newChild) |
void | setAlternatives (const QVector< FieldInfo > &alternatives, bool emitSignal) |
virtual BitCount32 | size () const |
![]() | |
DataInformationWithChildren (const QString &name, const QVector< DataInformation * > &children=QVector< DataInformation * >(), DataInformation *parent=0) | |
virtual | ~DataInformationWithChildren () |
void | appendChild (DataInformation *child, bool emitSignal=true) |
void | appendChildren (const QVector< DataInformation * > &newChildren, bool emitSignal=true) |
virtual void | calculateValidationState () |
virtual bool | canHaveChildren () const |
virtual QVariant | childData (int row, int column, int role) const |
virtual QWidget * | createEditWidget (QWidget *parent) const |
virtual QVariant | dataFromWidget (const QWidget *w) const |
virtual void | resetValidationState () |
void | setChildren (const QVector< DataInformation * > &newChildren) |
void | setChildren (QScriptValue newChildren) |
virtual bool | setData (const QVariant &value, Okteta::AbstractByteArrayModel *out, Okteta::Address address, BitCount64 bitsRemaining, quint8 bitOffset) |
virtual void | setWidgetData (QWidget *w) const |
virtual QString | tooltipString () const |
![]() | |
DataInformation (const QString &name, DataInformationBase *parent=NULL) | |
virtual | ~DataInformation () |
void | beginRead () |
DataInformationEndianess | byteOrder () const |
virtual DataInformation * | child (const QString &name) const |
virtual DataInformation * | clone () const =0 |
virtual QVariant | data (int column, int role) const |
QSysInfo::Endian | effectiveByteOrder () const |
virtual Qt::ItemFlags | flags (int column, bool fileLoaded=true) const |
QString | fullObjectPath () const |
bool | hasBeenUpdated () const |
bool | hasBeenValidated () const |
virtual bool | isTopLevel () const |
QDebug | logError () const |
ScriptLogger::LogLevel | loggedData () const |
ScriptLogger * | logger () const |
QDebug | logInfo () const |
QDebug | logWarn () const |
DataInformation * | mainStructure () |
QString | name () const |
DataInformationBase * | parent () const |
virtual BitCount64 | positionInFile (Okteta::Address start) const |
int | row () const |
void | setByteOrder (DataInformationEndianess newEndianess) |
void | setCustomTypeName (const QString &customTypeName) |
void | setLoggedData (ScriptLogger::LogLevel lvl) const |
void | setName (const QString &newName) |
void | setParent (DataInformationBase *newParent) |
void | setToStringFunction (const QScriptValue &value) |
void | setUpdateFunc (const QScriptValue &func) |
void | setValidationFunc (const QScriptValue &func) |
virtual QString | sizeString () const |
TopLevelDataInformation * | topLevelDataInformation () const |
virtual QScriptValue | toScriptValue (QScriptEngine *engine, ScriptHandlerInfo *handlerInfo) |
QScriptValue | toScriptValue (TopLevelDataInformation *top) |
QScriptValue | toStringFunction () const |
QString | typeName () const |
QScriptValue | updateFunc () const |
QString | validationError () const |
QScriptValue | validationFunc () const |
bool | validationSuccessful () const |
QString | valueString () const |
bool | wasAbleToRead () const |
![]() | |
DataInformationBase () | |
virtual | ~DataInformationBase () |
ArrayDataInformation * | asArray () |
const ArrayDataInformation * | asArray () const |
AbstractBitfieldDataInformation * | asBitfield () |
const AbstractBitfieldDataInformation * | asBitfield () const |
DataInformation * | asDataInformation () |
const DataInformation * | asDataInformation () const |
DummyDataInformation * | asDummy () |
const DummyDataInformation * | asDummy () const |
EnumDataInformation * | asEnum () |
const EnumDataInformation * | asEnum () const |
PointerDataInformation * | asPointer () |
const PointerDataInformation * | asPointer () const |
PrimitiveDataInformation * | asPrimitive () |
const PrimitiveDataInformation * | asPrimitive () const |
StringDataInformation * | asString () |
const StringDataInformation * | asString () const |
StructureDataInformation * | asStruct () |
const StructureDataInformation * | asStruct () const |
TaggedUnionDataInformation * | asTaggedUnion () |
const TaggedUnionDataInformation * | asTaggedUnion () const |
TopLevelDataInformation * | asTopLevel () |
const TopLevelDataInformation * | asTopLevel () const |
UnionDataInformation * | asUnion () |
const UnionDataInformation * | asUnion () const |
virtual bool | isArray () const |
virtual bool | isBitfield () const |
virtual bool | isDummy () const |
virtual bool | isEnum () const |
virtual bool | isPointer () const |
virtual bool | isPrimitive () const |
virtual bool | isString () const |
virtual bool | isStruct () const |
virtual bool | isUnion () const |
Detailed Description
A class holding the data of a struct for Okteta.
Definition at line 31 of file taggeduniondatainformation.h.
Constructor & Destructor Documentation
TaggedUnionDataInformation::TaggedUnionDataInformation | ( | const QString & | name, |
DataInformation * | parent = 0 |
||
) |
Definition at line 48 of file taggeduniondatainformation.cpp.
|
virtual |
Definition at line 39 of file taggeduniondatainformation.cpp.
Member Function Documentation
void TaggedUnionDataInformation::appendDefaultField | ( | DataInformation * | field, |
bool | emitSignal | ||
) |
Definition at line 63 of file taggeduniondatainformation.cpp.
|
virtual |
Reimplemented from DataInformationWithChildren.
Definition at line 263 of file taggeduniondatainformation.cpp.
|
virtual |
Reimplemented from DataInformationWithChildren.
Definition at line 275 of file taggeduniondatainformation.cpp.
|
virtual |
- Parameters
-
child the direct child we want to find the address for start the start of the root element
- Returns
- the address of
child
in the file
Implements DataInformation.
Definition at line 187 of file taggeduniondatainformation.cpp.
|
virtual |
Find the index of a DataInformation in this object, needed to calculate the row.
- Returns
- the index of
data
or -1 if not found
Reimplemented from DataInformationWithChildren.
Definition at line 243 of file taggeduniondatainformation.cpp.
|
inlinevirtual |
Reimplemented from DataInformationBase.
Definition at line 85 of file taggeduniondatainformation.h.
|
virtual |
Reads the necessary data from input
and returns the number of bytes read.
- Parameters
-
input the byte array to read from address the starting offset to read from bitsRemaining the number of bits remaining in out
bitOffset the bits that have already been read from the current byte (should be modified in this method)
- Returns
- the number of bits read or
-1
if none were read
Implements DataInformation.
Definition at line 146 of file taggeduniondatainformation.cpp.
|
virtual |
replaces child at index
with newChild
.
- Parameters
-
index the index of the child newChild the new child (ownership is taken if replacing is successful, otherwise it must be deleted)
- Returns
- true if replacing was successful, false otherwise
Reimplemented from DataInformationWithChildren.
Definition at line 235 of file taggeduniondatainformation.cpp.
void TaggedUnionDataInformation::setAlternatives | ( | const QVector< FieldInfo > & | alternatives, |
bool | emitSignal | ||
) |
Definition at line 73 of file taggeduniondatainformation.cpp.
|
virtual |
the size in bits of this element
Reimplemented from DataInformationWithChildren.
Definition at line 224 of file taggeduniondatainformation.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:04:11 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.