superkaramba
#include <Python.h>
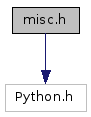
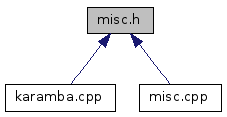
Go to the source code of this file.
Detailed Description
These are global functions that are used to interpret certain Python calls.
Definition in file misc.h.
Function Documentation
Misc/acceptDrops.
SYNOPSIS long acceptDrops(widget) DESCRIPTION Calling this enables your widget to receive Drop events. In other words, the user will be able to drag icons from her desktop and drop them on your widget. The "itemDropped" callback is called as a result with the data about the icon that was dropped on your widget. This allows, for example, icon bars where items are added to the icon bar by Drag and Drop. ARGUMENTS
- long widget – karamba RETURN VALUE 1 if successful
Misc/attachClickArea.
SYNOPSIS long attachClickArea(widget, meter, lB, mB, rB) DESCRIPTION It is possible to attach a clickarea to a meter (image or text field), which is moved and resized correctly if the meter is moved or resized.
There is also a callback meterClicked(widget, meter, button) which is called whenever a meter is clicked (if something is attached to it). Given an Image or a TextLabel, this call makes it clickable. When a mouse click is detected, the callback meterClicked is called.
lB, mB, and rB are strings that specify what command is executed when this meter is clicked with the left mouse button, middle mouse button, and right mouse button respectively. If given, the appropriate command is executed when the mouse click is received.
The keyword arguments are all optional. If command is an empty string nothing is executed.
For now the command given to RightButton has obviosly no effect (because that brings up the SuperKaramba menu).
ARGUMENTS
- long widget – karamba
- long meter – pointer to meter
- string lB – command to left mouse button
- string mB – command to middle mouse button
- string rB – command to right mouse button
RETURN VALUE 1 if successful
Misc/callTheme.
SYNOPSIS long callTheme(widget, theme, info) DESCRIPTION Calls a theme - identified by the pretty name - and passes it a string. This will work, despite superkaramba being multithreaded, because it uses the DCOP interface to contact the other theme. If you need to pass complex arguments (dictionaries, lists etc.) then use the python "repr" and "eval" functions to marshall and unmarshall the data structure. ARGUMENTS
- long widget – karamba
- string theme – path to theme to be called
- string info – a string containing the info to be passed to the theme RETURN VALUE 1 if successful
Misc/createClickArea.
SYNOPSIS long createClickArea(widget, x, y, w, h, cmd_to_run) DESCRIPTION This creates a clickable area at x,y with width and height w,h. When this area is clicked, cmd_to_run will be executed. The mouse will change to the clickable icon when over this area. ARGUMENTS
- long widget – karamba
- long x – x coordinate
- long y – y coordinate
- long w – width
- long h – height
- string cmd_to_run – command to be run RETURN VALUE a handle to the click area
Misc/createServiceClickArea.
SYNOPSIS long createServiceClickArea(widget, x, y, w, h, name_of_command, cmd_to_run, icon_to_display) DESCRIPTION This creates a clickable area at x,y with width and height w,h. When this area is clicked, cmd_to_run will be executed. The mouse will change to the clickable icon when over this area. For more information on the difference between createClickArea and createServiceClickArea, see the KDE documentation about KService, and the difference between KRun::run and KRun::runCommand. ARGUMENTS
- long widget – karamba
- long x – x coordinate
- long y – y coordinate
- long w – width
- long h – height
- string name_of_command – name to be displayed
- string cmd_to_run – command to be run
- string icon_to_display – name of icon to be displayed RETURN VALUE a handle to the click area
Misc/execute.
SYNOPSIS long execute(command) DESCRIPTION This command simply executes a program or command on the system. This is just for convience (IE you could accomplish this directly through python, but sometimes threading problems crop up that way). The only option is a string containing the command to execute. ARGUMENTS
- string command – command to execute RETURN VALUE 1 if successful
Misc/executeInteractive.
SYNOPSIS long executeInteractive(widget, command) DESCRIPTION This command executes a program or command on the system. But it allows you to get any text that the program outputs. Futhermore, it won't freeze up your widget while the command executes.
To use it, call executeInteractive with the reference to your widget and a list of command options. The array is simply a list that contains the command as the first entry, and each option as a separate list entry. Output from the command is returned via the commandOutput callback.
The command returns the process number of the command. This is useful if you want to run more than one program at a time. The number will allow you to figure out which program is outputting in the commandOutput callback.
Example: Run the command "ls -la *.zip"
myCommand = ["ls", "-la", "*.zip"] karamba.executeInteractive(widget, myCommand) ARGUMENTS
- long widget – karamba
- list command – command to execute RETURN VALUE 1 if successful
Misc/getIncomingData.
SYNOPSIS long getIncomingData(widget) DESCRIPTION Obtains the last data received by any other theme that set the "incoming data" of this theme. This isn't particularly sophisticated and could benefit from the data being placed in an FIFO queue instead. ARGUMENTS
- long widget – karamba RETURN VALUE string containing last information received from setIncomingData
Misc/getPrettyName.
SYNOPSIS string getPrettyName(theme) DESCRIPTION When a theme is created (with openNamedTheme), there is an option to give the theme an alternative name. This is useful if you open several widgets from the same theme: you need to give them unique names in order to contact them (for example, with callTheme or with setIncomingData) ARGUMENTS
- string theme – path to new theme RETURN VALUE the pretty name of the theme
Misc/getUpdateTime.
SYNOPSIS long getUpdateTime(widget) DESCRIPTION returns the last stored update time. intended for use so that the next refresh interval can work out how long ago the mouse was last moved over the widget. ARGUMENTS
- long widget – karamba RETURN VALUE last stored update time (from setUpdateTime)
Misc/managementPopup.
SYNOPSIS long managementPopup(widget) DESCRIPTION There's a management menu for SuperKaramba themes which allows themes to be loaded, closed, moved to other screens. If you want this popup menu to appear, call this function. ARGUMENTS
- long widget – karamba RETURN VALUE 1 if successful
Misc/openNamedTheme.
SYNOPSIS long openNamedTheme(theme, pretty_name, is_sub_theme) DESCRIPTION Opens a new theme, giving it a pretty (alternative and by your own choice unique) name. If you do not want the theme to be loaded when superkaramba is first started up (but instead want it to only be opened by this function call) then set is_sub_theme to 1. Themes opened with openNamedTheme will be unique. If a theme with the same pretty name already exists, openNamedTheme will have no effect. If you want duplicate themes (and a bit of a mess), use openTheme, instead. ARGUMENTS
- string theme – path to new theme
- string pretty_name – the name to be associated with the new widget
- long is_sub_theme – sets persistence (save state) of the theme RETURN VALUE a handle to the widget created
Misc/run.
SYNOPSIS long run(name, command, icon, list_of_args) DESCRIPTION This command simply executes a program or command on the system. The difference between run and execute is that run takes arguments, and the name of the icon to be displayed. ARGUMENTS
- string name – name to be displayed
- string command – command to execute
- string icon – name of icon to be displayed
- string list_of_args – arguments to be passed to the command RETURN VALUE 1 if successful
Misc/setIncomingData.
SYNOPSIS long setIncomingData(widget, theme, info) DESCRIPTION Contacts a theme - identified by the pretty name - and stores a string to be associated with the remote theme. The difference between setIncomingData and callTheme is that the theme is not notified by setIncomingData that the data has arrived. Previous information, if any, is overwritten. Use getIncomingData to retrieve the last stored information. setIncomingData is not very sophisticated, and could benefit from having info passed to it put into a queue, instead of being overwritten. ARGUMENTS
- long widget – karamba
- string theme – path to theme to have information passed to it.
- string info – a string containing the info to be passed to the theme RETURN VALUE 1 if successful
Misc/setUpdateTime.
SYNOPSIS long getUpdateTime(widget, updated_time) DESCRIPTION returns the last stored update time. intended for use so that the next refresh interval can work out how long ago the mouse was last moved over the widget. ARGUMENTS
- long widget – karamba
- long updated_time – the update time to be associated with the widget RETURN VALUE 1 if successful
Misc/toggleShowDesktop.
SYNOPSIS long toggleShowDesktop(widget) DESCRIPTION This shows/hides the current desktop just like the Show Desktop button on kicker. Basically, it minimizes all the windows on the current desktop. Call it once to show the desktop and again to hide it. ARGUMENTS
- long widget – karamba RETURN VALUE 1 if successful
Misc/translateAll.
SYNOPSIS long translateAll(widget, relative_x, relative_y) DESCRIPTION Moves all widgets within a theme in a particular direction relative from the previous spot without moving the parent theme widget. ARGUMENTS
- long widget – karamba
- long translate_x – move horizontally
- long translate_y – move vertically RETURN VALUE 1 if successful
Misc/userLanguages.
SYNOPSIS string userLanguages(widget) DESCRIPTION Returns a list (array) containing the language name abbreviations for the preferred interface languages user chose for KDE session in Region & Language settings. Having the whole array of preferred languages available is important for cases when you cannot provide interface translation for the 1st preferred language, but can for consecutive languages. (Implemented in version 0.42.) ARGUMENTS
- long widget – karamba RETURN VALUE list (array) with user languages in the order of preference.
Misc/wantRightButton.
SYNOPSIS long wantRightButton(widget, want_receive_right_button) DESCRIPTION There's a management menu for SuperKaramba themes which allows themes to be loaded, closed, moved to other screens. Not all themes will want this management interface. call karamba.wantRightButton(widget, 1) if you want to receive MouseUpdate button notifications. ARGUMENTS
- long widget – karamba
- long want_receive_right_button – whether the widget will receive right clicks RETURN VALUE 1 if successful
Documentation copyright © 1996-2014 The KDE developers.
Generated on Tue Oct 14 2014 23:07:20 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.