cantor/src/lib
#include <completionobject.h>
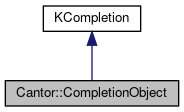
Public Types | |
enum | LineCompletionMode { PreliminaryCompletion, FinalCompletion } |
Signals | |
void | done () |
void | fetchingDone () |
void | fetchingTypeDone (IdentifierType type) |
void | lineDone (QString line, int index) |
Public Member Functions | |
CompletionObject (Session *parent) | |
~CompletionObject () | |
QString | command () const |
void | completeLine (const QString &comp, LineCompletionMode mode) |
QString | completion () const |
QStringList | completions () const |
Session * | session () const |
void | setLine (const QString &line, int index) |
void | updateLine (const QString &line, int index) |
Protected Types | |
enum | IdentifierType { VariableType, FunctionWithArguments, FunctionType = FunctionWithArguments, FunctionWithoutArguments, KeywordType, UnknownType } |
Protected Slots | |
void | completeLineWithType (IdentifierType type) |
virtual void | fetchCompletions ()=0 |
virtual void | fetchIdentifierType () |
void | findCompletion () |
void | handleParenCompletionWithType (IdentifierType type) |
Protected Member Functions | |
void | completeFunctionLine (IdentifierType type=FunctionWithArguments) |
void | completeKeywordLine () |
void | completeUnknownLine () |
void | completeVariableLine () |
QString | identifier () const |
virtual int | locateIdentifier (const QString &cmd, int index) const |
virtual bool | mayIdentifierBeginWith (QChar c) const |
virtual bool | mayIdentifierContain (QChar c) const |
void | setCommand (const QString &cmd) |
void | setCompletions (const QStringList &completions) |
Detailed Description
This Object is used to provide a Tab Completion, in an asynchroneous way.
Each backend, supporting tab completion, needs to provide their own CompletionObject, that reimplements the fetching of the completions and emits done() as soon as the completions are available
Definition at line 41 of file completionobject.h.
Member Enumeration Documentation
|
protected |
Definition at line 107 of file completionobject.h.
Enumerator | |
---|---|
PreliminaryCompletion |
Only insert the completion. |
FinalCompletion |
also add () for functions, etc |
Definition at line 53 of file completionobject.h.
Constructor & Destructor Documentation
CompletionObject::CompletionObject | ( | Session * | parent | ) |
Constructor.
- Parameters
-
parent the session, this object belongs to
Definition at line 43 of file completionobject.cpp.
CompletionObject::~CompletionObject | ( | ) |
Destructor.
Definition at line 56 of file completionobject.cpp.
Member Function Documentation
QString CompletionObject::command | ( | ) | const |
returns the command, this completion is for
- Returns
- the command, this completion is for
Definition at line 61 of file completionobject.cpp.
|
protected |
Completes line with function identifier and emits lineDone with the completed line.
Helper function for completeLine.
- Parameters
-
type whether the function takes arguments, default: FunctionWithArguments
Definition at line 220 of file completionobject.cpp.
|
protected |
Completes line with keyword identifier and emits lineDone with the completed line.
Helper function for completeLine.
Definition at line 266 of file completionobject.cpp.
void CompletionObject::completeLine | ( | const QString & | comp, |
CompletionObject::LineCompletionMode | mode | ||
) |
Takes a completion and a completion mode and triggers and calculates the new line with this completion.
If the completion mode is FinalCompletion some postprocessing is done asynchronously. Emits lineDone when finished.
- Parameters
-
comp the completion that's to be processed type whether the completion is final
Definition at line 121 of file completionobject.cpp.
|
protectedslot |
Calls the appropriate complete*Line based on type.
- Parameters
-
type the identifier type found in line()
Definition at line 201 of file completionobject.cpp.
|
protected |
Completes line with identifier of unknown type and emits lineDone with the completed line.
Helper function for completeLine.
Definition at line 288 of file completionobject.cpp.
|
protected |
Completes line with variable identifier and emits lineDone with the completed line.
Helper function for completeLine.
Definition at line 279 of file completionobject.cpp.
QString CompletionObject::completion | ( | ) | const |
Returns the last completion.
- Returns
- the last completion
Definition at line 81 of file completionobject.cpp.
QStringList CompletionObject::completions | ( | ) | const |
Returns a list of completions.
- Returns
- a list of completions
Definition at line 71 of file completionobject.cpp.
|
signal |
indicates that the possible completions and a common completion string have been found
|
protectedpure virtualslot |
This function should be reimplemented to start the actual fetching of the completions.
It can be asynchroneous. Remember to emit fetchingDone, if the fetching is complete
|
protectedvirtualslot |
Fetch the identifier type of d->identifier; reimplemented in the backends.
Emit fetchingTypeDone when done.
Definition at line 134 of file completionobject.cpp.
|
signal |
indicates that the fetching of completions is done
|
signal |
indicates that the type of identifier() was found and passes the type as an argument
- Parameters
-
type the identifier type
|
protectedslot |
Find the completion.
To be called when fetching is done. Emits done() when done.
Definition at line 175 of file completionobject.cpp.
|
protectedslot |
Handle a completion request after a opening parenthesis.
- Parameters
-
type the type of the identifier before the parenthesis
Definition at line 189 of file completionobject.cpp.
|
protected |
returns the identifier for fetchIdentifierType
- Returns
- the identifier for fetchIdentifierType
Definition at line 76 of file completionobject.cpp.
|
signal |
emitted when the line completion is done, passes the new line and the cursor index
- Parameters
-
line the new line index the new cursor index
|
protectedvirtual |
Find an identifier in cmd that ends at index.
- Parameters
-
cmd the command index the index to look at
Definition at line 151 of file completionobject.cpp.
|
protectedvirtual |
return true if identifier names can begin with c
- Parameters
-
c the character
Definition at line 170 of file completionobject.cpp.
|
protectedvirtual |
return true if c may be used in identifier names
- Parameters
-
c the character
Definition at line 165 of file completionobject.cpp.
Session * CompletionObject::session | ( | ) | const |
returns the session, this object belongs to
- Returns
- the session, this object belongs to
Definition at line 66 of file completionobject.cpp.
|
protected |
sets the command/command-part
- Parameters
-
cmd the command/command-part
Definition at line 146 of file completionobject.cpp.
|
protected |
Sets the completions.
- Parameters
-
completions list of possible completions
Definition at line 140 of file completionobject.cpp.
void CompletionObject::setLine | ( | const QString & | line, |
int | index | ||
) |
Sets the line and cursor index at which a completion should be found This triggers an asynchronous fetching of completions, which emits done() when done.
- Parameters
-
line the line that is to be completed index the cursor postition in line
Definition at line 86 of file completionobject.cpp.
void CompletionObject::updateLine | ( | const QString & | line, |
int | index | ||
) |
Takes the changed line and updates the command accordingly.
This triggers an asynchronous fetching of completions, which emits done() when done.
- Parameters
-
line the line that is to be completed index the cursor position in line
Definition at line 106 of file completionobject.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:16:33 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.