cantor/src/lib
#include <session.h>
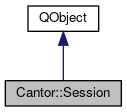
Public Types | |
enum | Status { Running, Done } |
Signals | |
void | error (const QString &msg) |
void | ready () |
void | statusChanged (Cantor::Session::Status newStatus) |
Public Member Functions | |
Session (Backend *backend) | |
~Session () | |
Backend * | backend () |
virtual CompletionObject * | completionFor (const QString &cmd, int index=-1) |
virtual Expression * | evaluateExpression (const QString &command, Expression::FinishingBehavior finishingBehavior)=0 |
Expression * | evaluateExpression (const QString &command) |
virtual void | interrupt ()=0 |
bool | isTypesettingEnabled () |
virtual void | login ()=0 |
virtual void | logout ()=0 |
int | nextExpressionId () |
virtual void | setTypesettingEnabled (bool enable) |
Cantor::Session::Status | status () |
virtual SyntaxHelpObject * | syntaxHelpFor (const QString &cmd) |
virtual QSyntaxHighlighter * | syntaxHighlighter (QObject *parent) |
virtual QAbstractItemModel * | variableModel () |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
void | changeStatus (Cantor::Session::Status newStatus) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
The Session object is the main class used to interact with a Backend.
It is used to evaluate Expressions, get completions, syntax highlighting, etc.
Member Enumeration Documentation
Constructor & Destructor Documentation
Session::Session | ( | Backend * | backend | ) |
Create a new Session.
This should not yet set up the complete session, thats job of the login() function
- See also
- login()
Definition at line 42 of file session.cpp.
Session::~Session | ( | ) |
Destructor.
Definition at line 48 of file session.cpp.
Member Function Documentation
Backend * Session::backend | ( | ) |
|
protected |
Change the status of the Session.
This will cause the stausChanged signal to be emited
- Parameters
-
newStatus the new status of the session
Definition at line 68 of file session.cpp.
|
virtual |
Returns tab-completion, for this command/command-part.
The return type is a CompletionObject. The fetching of the completions works asynchronously, you'll have to listen to the done() Signal of the returned object
- Parameters
-
cmd The partial command that should be completed index The index (cursor position) at which completion was invoked. Defaults to -1, indicating the end of the string.
- Returns
- a Completion object, representing this completion
- See also
- CompletionObject
Definition at line 84 of file session.cpp.
|
signal |
|
pure virtual |
Passes the given command to the backend and returns a Pointer to a new Expression object, which will emit the resultArrived() signal as soon as the computation is done.
The result will then be acessible by Expression::result()
- Parameters
-
command the command that should be run by the backend. finishingBehavior the FinishingBehaviour that should be used for this command.
- See also
- Expression::FinishingBehaviour
- Returns
- an Expression object, representing this command
Expression * Session::evaluateExpression | ( | const QString & | command | ) |
Reimplements evaluateExpression, setting the finishingBehaviour to DoNotDelete.
- See also
- evaluateExpressionconst(QString& command, Expression::FinishingBehavior finishingBehavior)
Definition at line 53 of file session.cpp.
|
pure virtual |
Interrupts all the running calculations in this session.
bool Session::isTypesettingEnabled | ( | ) |
Returns wether typesetting is enabled or not.
- Returns
- wether typesetting is enabled or not
Definition at line 79 of file session.cpp.
|
pure virtual |
|
pure virtual |
int Session::nextExpressionId | ( | ) |
Returns the next available Expression id It is basically a counter, incremented for each new Expression.
- Returns
- next Expression id
Definition at line 119 of file session.cpp.
|
signal |
|
virtual |
Enables/disables Typesetting for this session.
For this setting to make effect, the Backend must support LaTeX typesetting (as indicated by the capabilities() flag.
- Parameters
-
enable true to enable, false to disable typesetting
Definition at line 74 of file session.cpp.
Cantor::Session::Status Session::status | ( | ) |
Returns the status this Session has.
- Returns
- the status this Session has
Definition at line 63 of file session.cpp.
|
signal |
|
virtual |
Returns Syntax help, for this command.
It returns a SyntaxHelpObject, that will fetch the needed information asynchroneousely. You need to listen to the done() Signal of the Object
- Parameters
-
cmd the command, syntax help is requested for
- Returns
- SyntaxHelpObject, representing the help request
- See also
- SyntaxHelpObject
Definition at line 95 of file session.cpp.
|
virtual |
returns a syntax highlighter for this session
- Parameters
-
parent QObject the Highlighter's parent
- Returns
- QSyntaxHighlighter doing the highlighting for this Session
Definition at line 105 of file session.cpp.
|
virtual |
returns a Model to interact with the variables
- Returns
- QAbstractItemModel to interact with the variables
Definition at line 111 of file session.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:16:33 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.