kig
#include <polygon_imp.h>
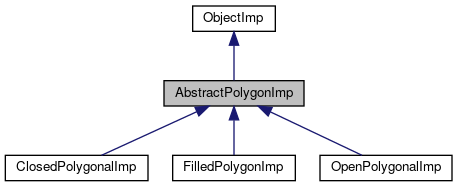
Public Types | |
typedef ObjectImp | Parent |
Public Member Functions | |
AbstractPolygonImp (const std::vector< Coordinate > &points) | |
AbstractPolygonImp (const uint nsides, const std::vector< Coordinate > &points, const Coordinate ¢erofmass) | |
~AbstractPolygonImp () | |
double | area () const |
Coordinate | attachPoint () const |
const Coordinate | centerOfMass () const |
double | cperimeter () const |
bool | equals (const ObjectImp &rhs) const |
const char * | iconForProperty (int which) const |
const ObjectImpType * | impRequirementForProperty (int which) const |
bool | inRect (const Rect &r, int width, const KigWidget &) const |
bool | isConvex () const |
bool | isInPolygon (const Coordinate &p) const |
bool | isMonotoneSteering () const |
bool | isOnCPolygonBorder (const Coordinate &p, double dist, const KigDocument &doc) const |
bool | isOnOPolygonBorder (const Coordinate &p, double dist, const KigDocument &doc) const |
bool | isPropertyDefinedOnOrThroughThisImp (int which) const |
bool | isTwisted () const |
uint | npoints () const |
int | numberOfProperties () const |
double | operimeter () const |
const std::vector< Coordinate > | points () const |
const QByteArrayList | properties () const |
const QByteArrayList | propertiesInternalNames () const |
ObjectImp * | property (int which, const KigDocument &w) const |
std::vector< Coordinate > | ptransform (const Transformation &) const |
Rect | surroundingRect () const |
bool | valid () const |
int | windingNumber () const |
![]() | |
virtual | ~ObjectImp () |
virtual bool | canFillInNextEscape () const |
virtual bool | contains (const Coordinate &p, int width, const KigWidget &si) const =0 |
virtual ObjectImp * | copy () const =0 |
virtual void | draw (KigPainter &p) const =0 |
virtual void | fillInNextEscape (QString &s, const KigDocument &) const |
int | getPropGid (const char *pname) const |
int | getPropLid (int propgid) const |
const char * | getPropName (int propgid) const |
bool | inherits (const ObjectImpType *t) const |
virtual bool | isCache () const |
virtual ObjectImp * | transform (const Transformation &t) const =0 |
virtual const ObjectImpType * | type () const =0 |
bool | valid () const |
virtual void | visit (ObjectImpVisitor *vtor) const =0 |
Static Public Member Functions | |
static const ObjectImpType * | stype () |
![]() | |
static const ObjectImpType * | stype () |
Protected Attributes | |
Coordinate | mcenterofmass |
uint | mnpoints |
std::vector< Coordinate > | mpoints |
Additional Inherited Members | |
![]() | |
ObjectImp () | |
Detailed Description
An ObjectImp representing a polygon.
Definition at line 28 of file polygon_imp.h.
Member Typedef Documentation
typedef ObjectImp AbstractPolygonImp::Parent |
Definition at line 38 of file polygon_imp.h.
Constructor & Destructor Documentation
AbstractPolygonImp::AbstractPolygonImp | ( | const std::vector< Coordinate > & | points | ) |
Definition at line 54 of file polygon_imp.cc.
AbstractPolygonImp::AbstractPolygonImp | ( | const uint | nsides, |
const std::vector< Coordinate > & | points, | ||
const Coordinate & | centerofmass | ||
) |
Definition at line 48 of file polygon_imp.cc.
AbstractPolygonImp::~AbstractPolygonImp | ( | ) |
Definition at line 68 of file polygon_imp.cc.
Member Function Documentation
double AbstractPolygonImp::area | ( | ) | const |
Returns the area of this polygon.
Definition at line 602 of file polygon_imp.cc.
|
virtual |
Returns a reference point where to attach labels; when this returns an invalidCoord then the attachment is either not done at all, or done in a specific way (like for curves, or for points) The treatment of points could also take advantage of this attachment mechanism.
If this method returns a valid Coordinate, then this is interpreted as a pivot point for the label, which can still be moved relative to that point, but follows the object when the object changes. In practice a new RelativePointType is created (position of the string), this type in turn depends on the object (to get its attachPoint) and two DoubleImp that are interpreted as relative displacement (x and y)
Implements ObjectImp.
Definition at line 72 of file polygon_imp.cc.
const Coordinate AbstractPolygonImp::centerOfMass | ( | ) | const |
Returns the center of mass of the polygon.
double AbstractPolygonImp::cperimeter | ( | ) | const |
Definition at line 591 of file polygon_imp.cc.
|
virtual |
Returns true if this ObjectImp is equal to rhs.
This function checks whether rhs is of the same ObjectImp type, and whether it contains the same data as this ObjectImp.
It is used e.g. by the KigCommand stuff to see what the user has changed during a move.
Implements ObjectImp.
Definition at line 645 of file polygon_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Reimplemented in OpenPolygonalImp, ClosedPolygonalImp, and FilledPolygonImp.
Definition at line 371 of file polygon_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Reimplemented in OpenPolygonalImp, ClosedPolygonalImp, and FilledPolygonImp.
Definition at line 343 of file polygon_imp.cc.
Implements ObjectImp.
Definition at line 210 of file polygon_imp.cc.
bool AbstractPolygonImp::isConvex | ( | ) | const |
Definition at line 922 of file polygon_imp.cc.
bool AbstractPolygonImp::isInPolygon | ( | const Coordinate & | p | ) | const |
Definition at line 137 of file polygon_imp.cc.
bool AbstractPolygonImp::isMonotoneSteering | ( | ) | const |
Definition at line 893 of file polygon_imp.cc.
bool AbstractPolygonImp::isOnCPolygonBorder | ( | const Coordinate & | p, |
double | dist, | ||
const KigDocument & | doc | ||
) | const |
Definition at line 188 of file polygon_imp.cc.
bool AbstractPolygonImp::isOnOPolygonBorder | ( | const Coordinate & | p, |
double | dist, | ||
const KigDocument & | doc | ||
) | const |
Definition at line 198 of file polygon_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Reimplemented in OpenPolygonalImp, ClosedPolygonalImp, and FilledPolygonImp.
Definition at line 769 of file polygon_imp.cc.
bool AbstractPolygonImp::isTwisted | ( | ) | const |
Definition at line 845 of file polygon_imp.cc.
uint AbstractPolygonImp::npoints | ( | ) | const |
Returns the number of points of this polygon.
Definition at line 576 of file polygon_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Reimplemented in OpenPolygonalImp, ClosedPolygonalImp, and FilledPolygonImp.
Definition at line 233 of file polygon_imp.cc.
double AbstractPolygonImp::operimeter | ( | ) | const |
Returns the perimeter of this polygon.
Definition at line 581 of file polygon_imp.cc.
const std::vector< Coordinate > AbstractPolygonImp::points | ( | ) | const |
Returns the vector with polygon points.
Definition at line 571 of file polygon_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Reimplemented in OpenPolygonalImp, ClosedPolygonalImp, and FilledPolygonImp.
Definition at line 298 of file polygon_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Reimplemented in OpenPolygonalImp, ClosedPolygonalImp, and FilledPolygonImp.
Definition at line 253 of file polygon_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Reimplemented in OpenPolygonalImp, ClosedPolygonalImp, and FilledPolygonImp.
Definition at line 445 of file polygon_imp.cc.
std::vector< Coordinate > AbstractPolygonImp::ptransform | ( | const Transformation & | t | ) | const |
Definition at line 77 of file polygon_imp.cc.
|
static |
Returns the ObjectImpType representing the PolygonImp type.
Definition at line 651 of file polygon_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 801 of file polygon_imp.cc.
bool AbstractPolygonImp::valid | ( | ) | const |
Definition at line 228 of file polygon_imp.cc.
int AbstractPolygonImp::windingNumber | ( | ) | const |
Definition at line 811 of file polygon_imp.cc.
Member Data Documentation
|
protected |
Definition at line 36 of file polygon_imp.h.
|
protected |
Definition at line 32 of file polygon_imp.h.
|
protected |
Definition at line 33 of file polygon_imp.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:12:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.