kig
#include <object_imp.h>
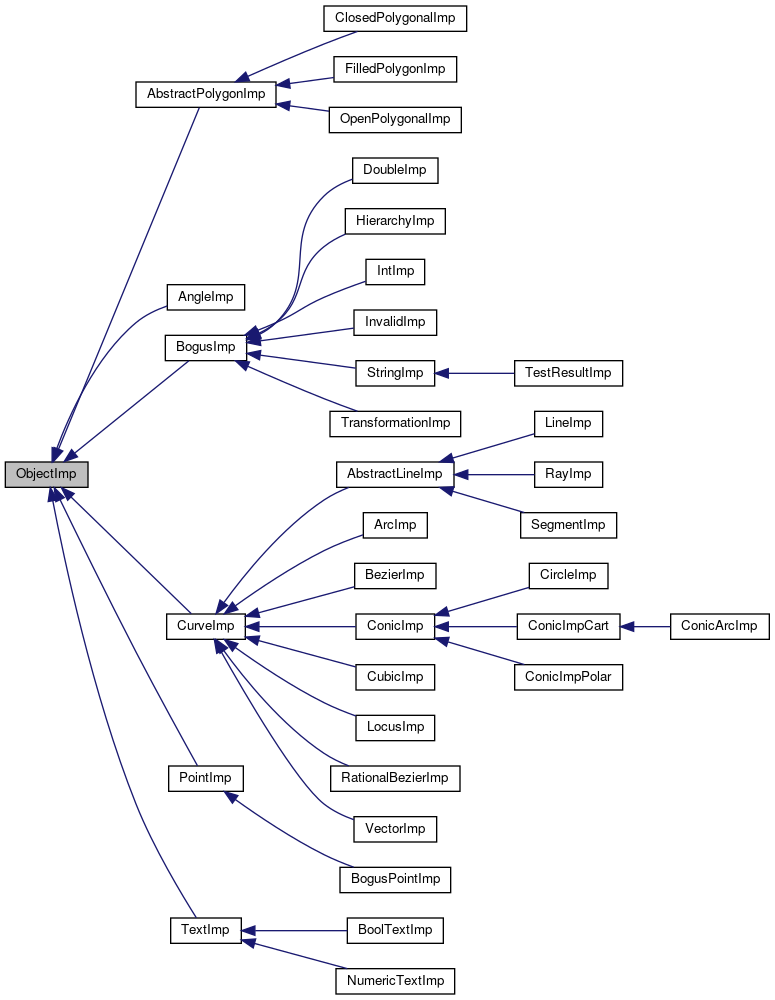
Public Member Functions | |
virtual | ~ObjectImp () |
virtual Coordinate | attachPoint () const =0 |
virtual bool | canFillInNextEscape () const |
virtual bool | contains (const Coordinate &p, int width, const KigWidget &si) const =0 |
virtual ObjectImp * | copy () const =0 |
virtual void | draw (KigPainter &p) const =0 |
virtual bool | equals (const ObjectImp &rhs) const =0 |
virtual void | fillInNextEscape (QString &s, const KigDocument &) const |
int | getPropGid (const char *pname) const |
int | getPropLid (int propgid) const |
const char * | getPropName (int propgid) const |
virtual const char * | iconForProperty (int which) const |
virtual const ObjectImpType * | impRequirementForProperty (int which) const |
bool | inherits (const ObjectImpType *t) const |
virtual bool | inRect (const Rect &r, int width, const KigWidget &si) const =0 |
virtual bool | isCache () const |
virtual bool | isPropertyDefinedOnOrThroughThisImp (int which) const |
virtual int | numberOfProperties () const |
virtual const QByteArrayList | properties () const |
virtual const QByteArrayList | propertiesInternalNames () const |
virtual ObjectImp * | property (int which, const KigDocument &d) const |
virtual Rect | surroundingRect () const =0 |
virtual ObjectImp * | transform (const Transformation &t) const =0 |
virtual const ObjectImpType * | type () const =0 |
bool | valid () const |
virtual void | visit (ObjectImpVisitor *vtor) const =0 |
Static Public Member Functions | |
static const ObjectImpType * | stype () |
Protected Member Functions | |
ObjectImp () | |
Detailed Description
The ObjectImp class represents the behaviour of an object after it is calculated.
This means how to draw() it, whether it claims to contain a certain point etc. It is also the class where the ObjectType's get their information from.
Definition at line 226 of file object_imp.h.
Constructor & Destructor Documentation
|
protected |
Definition at line 33 of file object_imp.cc.
|
virtual |
Definition at line 37 of file object_imp.cc.
Member Function Documentation
|
pure virtual |
Returns a reference point where to attach labels; when this returns an invalidCoord then the attachment is either not done at all, or done in a specific way (like for curves, or for points) The treatment of points could also take advantage of this attachment mechanism.
If this method returns a valid Coordinate, then this is interpreted as a pivot point for the label, which can still be moved relative to that point, but follows the object when the object changes. In practice a new RelativePointType is created (position of the string), this type in turn depends on the object (to get its attachPoint) and two DoubleImp that are interpreted as relative displacement (x and y)
Implemented in RationalBezierImp, AngleImp, BezierImp, AbstractPolygonImp, BogusImp, CurveImp, PointImp, and TextImp.
|
virtual |
Reimplemented in StringImp, IntImp, DoubleImp, InvalidImp, and PointImp.
Definition at line 192 of file object_imp.cc.
|
pure virtual |
Implemented in LineImp, ArcImp, ConicArcImp, OpenPolygonalImp, RayImp, ClosedPolygonalImp, RationalBezierImp, VectorImp, FilledPolygonImp, SegmentImp, LocusImp, BezierImp, PointImp, ConicImp, AngleImp, BogusImp, CircleImp, TextImp, and CubicImp.
|
pure virtual |
Returns a copy of this ObjectImp.
The copy is an exact copy. Changes to the copy don't affect the original.
Implemented in TestResultImp, TransformationImp, HierarchyImp, LineImp, StringImp, ConicArcImp, ArcImp, OpenPolygonalImp, RayImp, ConicImpPolar, IntImp, ClosedPolygonalImp, ConicImpCart, VectorImp, RationalBezierImp, FilledPolygonImp, SegmentImp, DoubleImp, BoolTextImp, NumericTextImp, InvalidImp, LocusImp, PointImp, AngleImp, CurveImp, CubicImp, BezierImp, CircleImp, and TextImp.
|
pure virtual |
Implemented in LineImp, ArcImp, OpenPolygonalImp, RayImp, ClosedPolygonalImp, RationalBezierImp, VectorImp, FilledPolygonImp, SegmentImp, LocusImp, BezierImp, PointImp, ConicImp, AngleImp, BogusImp, CircleImp, TextImp, and CubicImp.
|
pure virtual |
Returns true if this ObjectImp is equal to rhs.
This function checks whether rhs is of the same ObjectImp type, and whether it contains the same data as this ObjectImp.
It is used e.g. by the KigCommand stuff to see what the user has changed during a move.
Implemented in TestResultImp, TransformationImp, ArcImp, HierarchyImp, StringImp, RationalBezierImp, IntImp, VectorImp, ConicImp, DoubleImp, CircleImp, LocusImp, BezierImp, AngleImp, AbstractPolygonImp, InvalidImp, PointImp, AbstractLineImp, CubicImp, and TextImp.
|
virtual |
Reimplemented in StringImp, IntImp, DoubleImp, InvalidImp, and PointImp.
Definition at line 46 of file object_imp.cc.
int ObjectImp::getPropGid | ( | const char * | pname | ) | const |
Definition at line 333 of file object_imp.cc.
int ObjectImp::getPropLid | ( | int | propgid | ) | const |
mp: The following three methods (getPropLid, getPropGid, getPropName) deal with the properties of ObjectImp(s).
the properties architecture (vectors of properties associated to each Imp) didn't work at all in situation when a node in the object hierarchy of which a properties is used changes it's Imp dynamically. An example of this is a python script that constructs a "hyperbolic segment" in the half-plane model of hyperbolic geometry, that results in a circular arc or a segment depending on the relative position of the two end-points. A workaround for this problem is to maintain two numberings for a property: the "local" id (Lid) is the index in the property vector associated to an ObjectImp, it makes only sense relatively to a specific ObjectImp; the "global" id (Gid) is a global numbering generated runtime and distinguishing properties only based on their internal name. A property node must only use the global numbering, so that when the parent changes it's Imp, the correct property (if it exists) of the new Imp will be used. the association of Gid to properties is constructed runtime whenever a new property is first used by populating a static vector (see object_imp.cc). The conversion Gid->Lid requires a vector search, so that a caching mechanism has been set up in the ObjectPropertyCalcer that stores the Lid and recalculates it when the typeid of the ObjectImp of the parent changes.
getPropLid: returns the local numbering corresponding to a Gid getPropGid: returns the Gid of a property given its internal name getPropName: returns the internal name of a property given its Gid
Note: in object_hierarchy.cc the class "FetchPropertyNode" is quite similar to "ObjectPropertyCalcer", and thus is similarly restructured. However the caching mechanism has not been setup in this case. It seems that "FetchPropertyNode" is only used for the "drawprelim" in constructions that involve a macro that in turn involves a property, so perhaps the caching is not that important.
Definition at line 347 of file object_imp.cc.
const char * ObjectImp::getPropName | ( | int | propgid | ) | const |
Definition at line 356 of file object_imp.cc.
|
virtual |
Reimplemented in TestResultImp, ArcImp, ConicArcImp, OpenPolygonalImp, RayImp, RationalBezierImp, ClosedPolygonalImp, VectorImp, FilledPolygonImp, SegmentImp, BoolTextImp, NumericTextImp, LocusImp, BezierImp, ConicImp, PointImp, AngleImp, AbstractPolygonImp, CircleImp, TextImp, AbstractLineImp, and CubicImp.
Definition at line 187 of file object_imp.cc.
|
virtual |
Reimplemented in TestResultImp, ArcImp, OpenPolygonalImp, RayImp, RationalBezierImp, ClosedPolygonalImp, VectorImp, FilledPolygonImp, SegmentImp, BoolTextImp, NumericTextImp, LocusImp, BezierImp, PointImp, AngleImp, AbstractPolygonImp, CircleImp, ConicImp, TextImp, AbstractLineImp, and CubicImp.
Definition at line 76 of file object_imp.cc.
bool ObjectImp::inherits | ( | const ObjectImpType * | t | ) | const |
|
pure virtual |
Implemented in ArcImp, RationalBezierImp, VectorImp, LocusImp, BezierImp, PointImp, ConicImp, AngleImp, AbstractPolygonImp, BogusImp, CircleImp, AbstractLineImp, TextImp, and CubicImp.
|
virtual |
Return true if this imp is just a cache imp. This means that it will never be considered to be stored in a file or in an ObjectHierarchy. This is useful for objects which cannot (easily and usefully) be (de)serialized, like e.g. PythonCompiledScriptImp. For normal objects, the default implementation returns false, which is fine.
Definition at line 306 of file object_imp.cc.
|
virtual |
Reimplemented in TestResultImp, ArcImp, ConicArcImp, OpenPolygonalImp, RationalBezierImp, ClosedPolygonalImp, VectorImp, FilledPolygonImp, BoolTextImp, NumericTextImp, LocusImp, BezierImp, PointImp, AngleImp, AbstractPolygonImp, CircleImp, ConicImp, TextImp, AbstractLineImp, and CubicImp.
Definition at line 326 of file object_imp.cc.
|
virtual |
Reimplemented in TestResultImp, ArcImp, ConicArcImp, OpenPolygonalImp, RayImp, RationalBezierImp, ClosedPolygonalImp, VectorImp, FilledPolygonImp, SegmentImp, BoolTextImp, NumericTextImp, LocusImp, BezierImp, ConicImp, PointImp, AngleImp, AbstractPolygonImp, CircleImp, TextImp, AbstractLineImp, and CubicImp.
Definition at line 58 of file object_imp.cc.
|
virtual |
Reimplemented in TestResultImp, ArcImp, ConicArcImp, OpenPolygonalImp, RayImp, RationalBezierImp, ClosedPolygonalImp, VectorImp, FilledPolygonImp, SegmentImp, BoolTextImp, NumericTextImp, LocusImp, BezierImp, ConicImp, PointImp, AngleImp, AbstractPolygonImp, CircleImp, TextImp, AbstractLineImp, and CubicImp.
Definition at line 51 of file object_imp.cc.
|
virtual |
Reimplemented in TestResultImp, ArcImp, ConicArcImp, OpenPolygonalImp, RayImp, RationalBezierImp, ClosedPolygonalImp, VectorImp, FilledPolygonImp, SegmentImp, BoolTextImp, NumericTextImp, LocusImp, BezierImp, ConicImp, PointImp, AngleImp, AbstractPolygonImp, CircleImp, TextImp, AbstractLineImp, and CubicImp.
Definition at line 63 of file object_imp.cc.
|
virtual |
Reimplemented in TestResultImp, ArcImp, ConicArcImp, OpenPolygonalImp, RayImp, RationalBezierImp, ClosedPolygonalImp, VectorImp, FilledPolygonImp, SegmentImp, BoolTextImp, NumericTextImp, LocusImp, BezierImp, ConicImp, PointImp, AngleImp, AbstractPolygonImp, CircleImp, TextImp, AbstractLineImp, and CubicImp.
Definition at line 70 of file object_imp.cc.
|
static |
The ObjectImpType representing the base ObjectImp class.
All other ObjectImp's inherit from this type.
Definition at line 284 of file object_imp.cc.
|
pure virtual |
|
pure virtual |
Return this ObjectImp, transformed by the transformation t.
Implemented in LineImp, ConicArcImp, ArcImp, OpenPolygonalImp, RayImp, ClosedPolygonalImp, RationalBezierImp, VectorImp, FilledPolygonImp, SegmentImp, LocusImp, PointImp, BezierImp, BogusImp, ConicImp, AngleImp, CircleImp, TextImp, and CubicImp.
|
pure virtual |
Returns the lowermost ObjectImpType that this object is an instantiation of.
E.g. if you want to get a string containing the internal name of the type of an object, you can do:
Implemented in TestResultImp, TransformationImp, ConicArcImp, HierarchyImp, LineImp, ArcImp, StringImp, OpenPolygonalImp, RayImp, VectorImp, IntImp, RationalBezierImp, ClosedPolygonalImp, ConicImp, SegmentImp, FilledPolygonImp, DoubleImp, BoolTextImp, LocusImp, BogusPointImp, NumericTextImp, BezierImp, InvalidImp, AngleImp, PointImp, CubicImp, CircleImp, and TextImp.
bool ObjectImp::valid | ( | ) | const |
Returns true if this is a valid ObjectImp.
If you want to return an invalid ObjectImp, you should return an InvalidImp instance.
Definition at line 41 of file object_imp.cc.
|
pure virtual |
Implemented in TestResultImp, TransformationImp, HierarchyImp, LineImp, ArcImp, StringImp, OpenPolygonalImp, RayImp, VectorImp, IntImp, RationalBezierImp, ClosedPolygonalImp, ConicImp, SegmentImp, FilledPolygonImp, DoubleImp, LocusImp, BezierImp, InvalidImp, AngleImp, PointImp, CubicImp, CircleImp, and TextImp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:12:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.