kig
#include <bezier_imp.h>
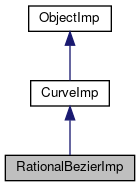
Public Types | |
typedef CurveImp | Parent |
![]() | |
typedef ObjectImp | Parent |
Public Member Functions | |
RationalBezierImp (const std::vector< Coordinate > &points, const std::vector< double > &weights) | |
~RationalBezierImp () | |
Coordinate | attachPoint () const |
const Coordinate | centerOfMass () const |
bool | contains (const Coordinate &p, int width, const KigWidget &) const |
bool | containsPoint (const Coordinate &p, const KigDocument &doc) const |
RationalBezierImp * | copy () const |
void | draw (KigPainter &p) const |
bool | equals (const ObjectImp &rhs) const |
const Coordinate | getPoint (double param, const KigDocument &) const |
const char * | iconForProperty (int which) const |
const ObjectImpType * | impRequirementForProperty (int which) const |
bool | inRect (const Rect &r, int width, const KigWidget &) const |
bool | internalContainsPoint (const Coordinate &p, double threshold, const KigDocument &doc) const |
bool | isPropertyDefinedOnOrThroughThisImp (int which) const |
uint | npoints () const |
int | numberOfProperties () const |
const std::vector< Coordinate > | points () const |
const QByteArrayList | properties () const |
const QByteArrayList | propertiesInternalNames () const |
ObjectImp * | property (int which, const KigDocument &w) const |
Rect | surroundingRect () const |
ObjectImp * | transform (const Transformation &) const |
const ObjectImpType * | type () const |
bool | valid () const |
void | visit (ObjectImpVisitor *vtor) const |
![]() | |
Coordinate | attachPoint () const |
QString | cartesianEquationString (const KigDocument &w) const |
virtual double | getParam (const Coordinate &point, const KigDocument &) const |
![]() | |
virtual | ~ObjectImp () |
virtual bool | canFillInNextEscape () const |
virtual void | fillInNextEscape (QString &s, const KigDocument &) const |
int | getPropGid (const char *pname) const |
int | getPropLid (int propgid) const |
const char * | getPropName (int propgid) const |
bool | inherits (const ObjectImpType *t) const |
virtual bool | isCache () const |
bool | valid () const |
Static Public Member Functions | |
static const ObjectImpType * | stype () |
static const ObjectImpType * | stype2 () |
static const ObjectImpType * | stype3 () |
![]() | |
static const ObjectImpType * | stype () |
![]() | |
static const ObjectImpType * | stype () |
Additional Inherited Members | |
![]() | |
double | getDist (double param, const Coordinate &p, const KigDocument &doc) const |
double | getParamofmin (double a, double b, const Coordinate &p, const KigDocument &doc) const |
![]() | |
ObjectImp () | |
Detailed Description
An ObjectImp representing a rational Bézier curve.
Definition at line 100 of file bezier_imp.h.
Member Typedef Documentation
typedef CurveImp RationalBezierImp::Parent |
Definition at line 112 of file bezier_imp.h.
Constructor & Destructor Documentation
RationalBezierImp::RationalBezierImp | ( | const std::vector< Coordinate > & | points, |
const std::vector< double > & | weights | ||
) |
Constructs a rational Bézier curve.
Definition at line 336 of file bezier_imp.cc.
RationalBezierImp::~RationalBezierImp | ( | ) |
Definition at line 355 of file bezier_imp.cc.
Member Function Documentation
|
virtual |
Returns a reference point where to attach labels; when this returns an invalidCoord then the attachment is either not done at all, or done in a specific way (like for curves, or for points) The treatment of points could also take advantage of this attachment mechanism.
If this method returns a valid Coordinate, then this is interpreted as a pivot point for the label, which can still be moved relative to that point, but follows the object when the object changes. In practice a new RelativePointType is created (position of the string), this type in turn depends on the object (to get its attachPoint) and two DoubleImp that are interpreted as relative displacement (x and y)
Implements ObjectImp.
Definition at line 359 of file bezier_imp.cc.
const Coordinate RationalBezierImp::centerOfMass | ( | ) | const |
Returns the center of mass of the control polygon.
|
virtual |
Implements ObjectImp.
Definition at line 597 of file bezier_imp.cc.
|
virtual |
Return whether this Curve contains the given point.
This is implemented as a numerical approximation. Implementations can/should use the value test_threshold in common.h as a threshold value.
Implements CurveImp.
Definition at line 602 of file bezier_imp.cc.
|
virtual |
Returns a copy of this ObjectImp.
The copy is an exact copy. Changes to the copy don't affect the original.
Implements CurveImp.
Definition at line 497 of file bezier_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 385 of file bezier_imp.cc.
|
virtual |
Returns true if this ObjectImp is equal to rhs.
This function checks whether rhs is of the same ObjectImp type, and whether it contains the same data as this ObjectImp.
It is used e.g. by the KigCommand stuff to see what the user has changed during a move.
Implements ObjectImp.
Definition at line 507 of file bezier_imp.cc.
|
virtual |
Implements CurveImp.
Definition at line 628 of file bezier_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 448 of file bezier_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 441 of file bezier_imp.cc.
Implements ObjectImp.
Definition at line 390 of file bezier_imp.cc.
bool RationalBezierImp::internalContainsPoint | ( | const Coordinate & | p, |
double | threshold, | ||
const KigDocument & | doc | ||
) | const |
Definition at line 607 of file bezier_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 579 of file bezier_imp.cc.
uint RationalBezierImp::npoints | ( | ) | const |
Returns the number of control points.
Definition at line 492 of file bezier_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 416 of file bezier_imp.cc.
const std::vector< Coordinate > RationalBezierImp::points | ( | ) | const |
Returns the vector with control points.
Definition at line 487 of file bezier_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 431 of file bezier_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 421 of file bezier_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 463 of file bezier_imp.cc.
|
static |
Returns the ObjectImpType representing the RationalBezierImp type.
Definition at line 516 of file bezier_imp.cc.
|
static |
Definition at line 534 of file bezier_imp.cc.
|
static |
Definition at line 552 of file bezier_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 587 of file bezier_imp.cc.
|
virtual |
Return this ObjectImp, transformed by the transformation t.
Implements ObjectImp.
Definition at line 364 of file bezier_imp.cc.
|
virtual |
Returns the lowermost ObjectImpType that this object is an instantiation of.
E.g. if you want to get a string containing the internal name of the type of an object, you can do:
Implements ObjectImp.
Definition at line 570 of file bezier_imp.cc.
bool RationalBezierImp::valid | ( | ) | const |
Definition at line 408 of file bezier_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 502 of file bezier_imp.cc.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:12:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.