kig
#include <other_imp.h>
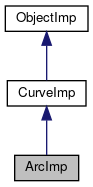
Public Types | |
typedef CurveImp | Parent |
![]() | |
typedef ObjectImp | Parent |
Public Member Functions | |
ArcImp (const Coordinate ¢er, const double radius, const double startangle, const double angle) | |
~ArcImp () | |
double | angle () const |
const Coordinate | center () const |
bool | contains (const Coordinate &p, int width, const KigWidget &w) const |
bool | containsPoint (const Coordinate &p, const KigDocument &doc) const |
ArcImp * | copy () const |
void | draw (KigPainter &p) const |
bool | equals (const ObjectImp &rhs) const |
Coordinate | firstEndPoint () const |
double | getParam (const Coordinate &c, const KigDocument &d) const |
const Coordinate | getPoint (double p, const KigDocument &d) const |
const char * | iconForProperty (int which) const |
const ObjectImpType * | impRequirementForProperty (int which) const |
bool | inRect (const Rect &r, int width, const KigWidget &si) const |
bool | internalContainsPoint (const Coordinate &p, double threshold) const |
bool | isPropertyDefinedOnOrThroughThisImp (int which) const |
int | numberOfProperties () const |
const QByteArrayList | properties () const |
const QByteArrayList | propertiesInternalNames () const |
ObjectImp * | property (int which, const KigDocument &d) const |
double | radius () const |
Coordinate | secondEndPoint () const |
double | sectorSurface () const |
double | startAngle () const |
Rect | surroundingRect () const |
ObjectImp * | transform (const Transformation &t) const |
const ObjectImpType * | type () const |
bool | valid () const |
void | visit (ObjectImpVisitor *vtor) const |
![]() | |
Coordinate | attachPoint () const |
QString | cartesianEquationString (const KigDocument &w) const |
![]() | |
virtual | ~ObjectImp () |
virtual bool | canFillInNextEscape () const |
virtual void | fillInNextEscape (QString &s, const KigDocument &) const |
int | getPropGid (const char *pname) const |
int | getPropLid (int propgid) const |
const char * | getPropName (int propgid) const |
bool | inherits (const ObjectImpType *t) const |
virtual bool | isCache () const |
bool | valid () const |
Static Public Member Functions | |
static const ObjectImpType * | stype () |
![]() | |
static const ObjectImpType * | stype () |
![]() | |
static const ObjectImpType * | stype () |
Additional Inherited Members | |
![]() | |
double | getDist (double param, const Coordinate &p, const KigDocument &doc) const |
double | getParamofmin (double a, double b, const Coordinate &p, const KigDocument &doc) const |
![]() | |
ObjectImp () | |
Detailed Description
An ObjectImp representing an arc.
Definition at line 169 of file other_imp.h.
Member Typedef Documentation
typedef CurveImp ArcImp::Parent |
Definition at line 177 of file other_imp.h.
Constructor & Destructor Documentation
ArcImp::ArcImp | ( | const Coordinate & | center, |
const double | radius, | ||
const double | startangle, | ||
const double | angle | ||
) |
Construct an Arc with a given center, radius, start angle and dimension (both in radians).
Definition at line 320 of file other_imp.cc.
ArcImp::~ArcImp | ( | ) |
Definition at line 333 of file other_imp.cc.
Member Function Documentation
double ArcImp::angle | ( | ) | const |
Return the dimension in radians of this arc.
Definition at line 547 of file other_imp.cc.
const Coordinate ArcImp::center | ( | ) | const |
Return the center of this arc.
Definition at line 532 of file other_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 381 of file other_imp.cc.
|
virtual |
Return whether this Curve contains the given point.
This is implemented as a numerical approximation. Implementations can/should use the value test_threshold in common.h as a threshold value.
Implements CurveImp.
Definition at line 661 of file other_imp.cc.
|
virtual |
Returns a copy of this ObjectImp.
The copy is an exact copy. Changes to the copy don't affect the original.
Implements CurveImp.
Definition at line 337 of file other_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 376 of file other_imp.cc.
|
virtual |
Returns true if this ObjectImp is equal to rhs.
This function checks whether rhs is of the same ObjectImp type, and whether it contains the same data as this ObjectImp.
It is used e.g. by the KigCommand stuff to see what the user has changed during a move.
Implements ObjectImp.
Definition at line 574 of file other_imp.cc.
Coordinate ArcImp::firstEndPoint | ( | ) | const |
Return the start point of this arc.
Definition at line 552 of file other_imp.cc.
|
virtual |
Reimplemented from CurveImp.
Definition at line 511 of file other_imp.cc.
|
virtual |
Implements CurveImp.
Definition at line 525 of file other_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 435 of file other_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 498 of file other_imp.cc.
Implements ObjectImp.
Definition at line 386 of file other_imp.cc.
bool ArcImp::internalContainsPoint | ( | const Coordinate & | p, |
double | threshold | ||
) | const |
Definition at line 666 of file other_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 683 of file other_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 397 of file other_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 402 of file other_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 419 of file other_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 464 of file other_imp.cc.
double ArcImp::radius | ( | ) | const |
Return the radius of this arc.
Definition at line 537 of file other_imp.cc.
Coordinate ArcImp::secondEndPoint | ( | ) | const |
Return the end point of this arc.
Definition at line 558 of file other_imp.cc.
double ArcImp::sectorSurface | ( | ) | const |
Return the size of the sector surface of this arc.
Definition at line 493 of file other_imp.cc.
double ArcImp::startAngle | ( | ) | const |
Return the start angle in radians of this arc.
Definition at line 542 of file other_imp.cc.
|
static |
Returns the ObjectImpType representing the ArcImp type.
Definition at line 629 of file other_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 723 of file other_imp.cc.
|
virtual |
Return this ObjectImp, transformed by the transformation t.
Implements ObjectImp.
Definition at line 342 of file other_imp.cc.
|
virtual |
Returns the lowermost ObjectImpType that this object is an instantiation of.
E.g. if you want to get a string containing the internal name of the type of an object, you can do:
Implements ObjectImp.
Definition at line 656 of file other_imp.cc.
bool ArcImp::valid | ( | ) | const |
Definition at line 392 of file other_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 506 of file other_imp.cc.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:12:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.