kig
#include <conic_imp.h>
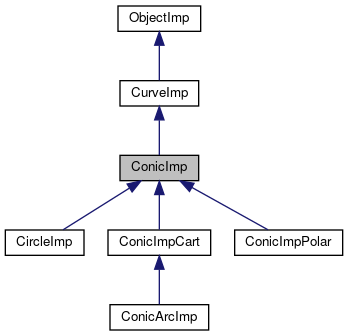
Public Types | |
typedef CurveImp | Parent |
![]() | |
typedef ObjectImp | Parent |
Public Member Functions | |
virtual const ConicCartesianData | cartesianData () const |
virtual QString | cartesianEquationString (const KigDocument &w) const |
virtual Coordinate | coniccenter () const |
virtual int | conicType () const |
virtual QString | conicTypeString () const |
bool | contains (const Coordinate &p, int width, const KigWidget &) const |
bool | containsPoint (const Coordinate &p, const KigDocument &doc) const |
void | draw (KigPainter &p) const |
bool | equals (const ObjectImp &rhs) const |
virtual Coordinate | focus1 () const |
virtual Coordinate | focus2 () const |
double | getParam (const Coordinate &point, const KigDocument &) const |
double | getParam (const Coordinate &point) const |
const Coordinate | getPoint (double param, const KigDocument &) const |
const Coordinate | getPoint (double param) const |
const char * | iconForProperty (int which) const |
const ObjectImpType * | impRequirementForProperty (int which) const |
bool | inRect (const Rect &r, int width, const KigWidget &) const |
bool | internalContainsPoint (const Coordinate &p, double threshold) const |
bool | isPropertyDefinedOnOrThroughThisImp (int which) const |
bool | isVerticalParabola (ConicCartesianData &data) const |
int | numberOfProperties () const |
virtual const ConicPolarData | polarData () const =0 |
virtual QString | polarEquationString (const KigDocument &w) const |
const QByteArrayList | properties () const |
const QByteArrayList | propertiesInternalNames () const |
ObjectImp * | property (int which, const KigDocument &w) const |
Rect | surroundingRect () const |
ObjectImp * | transform (const Transformation &) const |
const ObjectImpType * | type () const |
bool | valid () const |
void | visit (ObjectImpVisitor *vtor) const |
![]() | |
Coordinate | attachPoint () const |
QString | cartesianEquationString (const KigDocument &w) const |
virtual CurveImp * | copy () const =0 |
![]() | |
virtual | ~ObjectImp () |
virtual bool | canFillInNextEscape () const |
virtual void | fillInNextEscape (QString &s, const KigDocument &) const |
int | getPropGid (const char *pname) const |
int | getPropLid (int propgid) const |
const char * | getPropName (int propgid) const |
bool | inherits (const ObjectImpType *t) const |
virtual bool | isCache () const |
bool | valid () const |
Static Public Member Functions | |
static const ObjectImpType * | stype () |
![]() | |
static const ObjectImpType * | stype () |
![]() | |
static const ObjectImpType * | stype () |
Protected Member Functions | |
ConicImp () | |
~ConicImp () | |
![]() | |
double | getDist (double param, const Coordinate &p, const KigDocument &doc) const |
double | getParamofmin (double a, double b, const Coordinate &p, const KigDocument &doc) const |
![]() | |
ObjectImp () | |
Detailed Description
An ObjectImp representing a conic.
A conic is a general second degree curve, and some beautiful theory has been developed about it. See a math book for more information. This class is in fact an abstract base class hiding the fact that a ConicImp can be constructed in two ways. If only its Cartesian equation is known, then you should use ConicImpCart, otherwise, you should use ConicImpPolar. If the other representation is needed, it will be calculated, but a cartesian representation is rarely needed, and not calculating saves some CPU cycles.
Definition at line 39 of file conic_imp.h.
Member Typedef Documentation
typedef CurveImp ConicImp::Parent |
Definition at line 46 of file conic_imp.h.
Constructor & Destructor Documentation
|
protected |
Definition at line 357 of file conic_imp.cc.
|
protected |
Definition at line 361 of file conic_imp.cc.
Member Function Documentation
|
virtual |
Return the cartesian representation of this conic.
Reimplemented in ConicImpCart, and CircleImp.
Definition at line 285 of file conic_imp.cc.
|
virtual |
A string containing the cartesian equation of the conic.
This will be of the form "a x^2 + b y^2 + c xy + d x + e y + f = 0".
Reimplemented in CircleImp.
Definition at line 221 of file conic_imp.cc.
|
virtual |
Return the center of this conic.
Definition at line 295 of file conic_imp.cc.
|
virtual |
Type of conic.
Return what type of conic this is: -1 for a hyperbola 0 for a parabola 1 for an ellipse
Reimplemented in CircleImp.
Definition at line 191 of file conic_imp.cc.
|
virtual |
A string containing "Hyperbola", "Parabola" or "Ellipse".
Definition at line 205 of file conic_imp.cc.
|
virtual |
|
virtual |
Return whether this Curve contains the given point.
This is implemented as a numerical approximation. Implementations can/should use the value test_threshold in common.h as a threshold value.
Implements CurveImp.
Reimplemented in ConicArcImp.
Definition at line 402 of file conic_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 42 of file conic_imp.cc.
|
virtual |
Returns true if this ObjectImp is equal to rhs.
This function checks whether rhs is of the same ObjectImp type, and whether it contains the same data as this ObjectImp.
It is used e.g. by the KigCommand stuff to see what the user has changed during a move.
Implements ObjectImp.
Definition at line 374 of file conic_imp.cc.
|
virtual |
Return the first focus of this conic.
Reimplemented in CircleImp.
Definition at line 290 of file conic_imp.cc.
|
virtual |
Return the second focus of this conic.
Reimplemented in CircleImp.
Definition at line 306 of file conic_imp.cc.
|
virtual |
Reimplemented from CurveImp.
Reimplemented in ConicArcImp.
Definition at line 144 of file conic_imp.cc.
double ConicImp::getParam | ( | const Coordinate & | point | ) | const |
Definition at line 149 of file conic_imp.cc.
|
virtual |
const Coordinate ConicImp::getPoint | ( | double | param | ) | const |
Definition at line 181 of file conic_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Reimplemented in ConicArcImp.
Definition at line 101 of file conic_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 94 of file conic_imp.cc.
Implements ObjectImp.
Definition at line 57 of file conic_imp.cc.
bool ConicImp::internalContainsPoint | ( | const Coordinate & | p, |
double | threshold | ||
) | const |
Definition at line 410 of file conic_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Reimplemented in ConicArcImp.
Definition at line 439 of file conic_imp.cc.
bool ConicImp::isVerticalParabola | ( | ConicCartesianData & | data | ) | const |
Definition at line 446 of file conic_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Reimplemented in ConicArcImp.
Definition at line 63 of file conic_imp.cc.
|
pure virtual |
Return the polar representation of this conic.
Implemented in ConicImpPolar, ConicImpCart, and CircleImp.
|
virtual |
A string containing the polar equation of the conic.
This will be of the form "rho = pdimen/(1 + ect cos( theta ) + est sin( theta ) )\n [centered at p]"
Reimplemented in CircleImp.
Definition at line 259 of file conic_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Reimplemented in ConicArcImp.
Definition at line 81 of file conic_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Reimplemented in ConicArcImp.
Definition at line 68 of file conic_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Reimplemented in ConicArcImp.
Definition at line 122 of file conic_imp.cc.
|
static |
Returns the ObjectImpType representing the ConicImp type.
Definition at line 380 of file conic_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 454 of file conic_imp.cc.
|
virtual |
Return this ObjectImp, transformed by the transformation t.
Implements ObjectImp.
Reimplemented in ConicArcImp.
Definition at line 34 of file conic_imp.cc.
|
virtual |
Returns the lowermost ObjectImpType that this object is an instantiation of.
E.g. if you want to get a string containing the internal name of the type of an object, you can do:
Implements ObjectImp.
Reimplemented in ConicArcImp.
Definition at line 397 of file conic_imp.cc.
bool ConicImp::valid | ( | ) | const |
Definition at line 47 of file conic_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 369 of file conic_imp.cc.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:12:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.