kig
#include <other_imp.h>
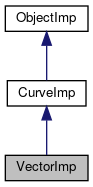
Public Types | |
typedef CurveImp | Parent |
![]() | |
typedef ObjectImp | Parent |
Public Member Functions | |
VectorImp (const Coordinate &a, const Coordinate &b) | |
~VectorImp () | |
const Coordinate | a () const |
const Coordinate | b () const |
bool | contains (const Coordinate &p, int width, const KigWidget &) const |
bool | containsPoint (const Coordinate &p, const KigDocument &doc) const |
VectorImp * | copy () const |
LineData | data () const |
const Coordinate | dir () const |
void | draw (KigPainter &p) const |
bool | equals (const ObjectImp &rhs) const |
double | getParam (const Coordinate &, const KigDocument &) const |
const Coordinate | getPoint (double param, const KigDocument &) const |
const char * | iconForProperty (int which) const |
const ObjectImpType * | impRequirementForProperty (int which) const |
bool | inRect (const Rect &r, int width, const KigWidget &) const |
bool | internalContainsPoint (const Coordinate &p, double threshold) const |
bool | isPropertyDefinedOnOrThroughThisImp (int which) const |
double | length () const |
int | numberOfProperties () const |
const QByteArrayList | properties () const |
const QByteArrayList | propertiesInternalNames () const |
ObjectImp * | property (int which, const KigDocument &w) const |
Rect | surroundingRect () const |
ObjectImp * | transform (const Transformation &) const |
const ObjectImpType * | type () const |
void | visit (ObjectImpVisitor *vtor) const |
![]() | |
Coordinate | attachPoint () const |
QString | cartesianEquationString (const KigDocument &w) const |
![]() | |
virtual | ~ObjectImp () |
virtual bool | canFillInNextEscape () const |
virtual void | fillInNextEscape (QString &s, const KigDocument &) const |
int | getPropGid (const char *pname) const |
int | getPropLid (int propgid) const |
const char * | getPropName (int propgid) const |
bool | inherits (const ObjectImpType *t) const |
virtual bool | isCache () const |
bool | valid () const |
Static Public Member Functions | |
static const ObjectImpType * | stype () |
![]() | |
static const ObjectImpType * | stype () |
![]() | |
static const ObjectImpType * | stype () |
Additional Inherited Members | |
![]() | |
double | getDist (double param, const Coordinate &p, const KigDocument &doc) const |
double | getParamofmin (double a, double b, const Coordinate &p, const KigDocument &doc) const |
![]() | |
ObjectImp () | |
Detailed Description
An ObjectImp representing a vector.
Definition at line 99 of file other_imp.h.
Member Typedef Documentation
typedef CurveImp VectorImp::Parent |
Definition at line 104 of file other_imp.h.
Constructor & Destructor Documentation
VectorImp::VectorImp | ( | const Coordinate & | a, |
const Coordinate & | b | ||
) |
Construct a Vector with a given start point and end point.
Definition at line 191 of file other_imp.cc.
VectorImp::~VectorImp | ( | ) |
Definition at line 196 of file other_imp.cc.
Member Function Documentation
const Coordinate VectorImp::a | ( | ) | const |
Return the start point of this vector.
Definition at line 564 of file other_imp.cc.
const Coordinate VectorImp::b | ( | ) | const |
Return the end point of this vector.
Definition at line 569 of file other_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 213 of file other_imp.cc.
|
virtual |
Return whether this Curve contains the given point.
This is implemented as a numerical approximation. Implementations can/should use the value test_threshold in common.h as a threshold value.
Implements CurveImp.
Definition at line 761 of file other_imp.cc.
|
virtual |
Returns a copy of this ObjectImp.
The copy is an exact copy. Changes to the copy don't affect the original.
Implements CurveImp.
Definition at line 295 of file other_imp.cc.
LineData VectorImp::data | ( | ) | const |
Get the LineData for this vector.
Definition at line 771 of file other_imp.cc.
const Coordinate VectorImp::dir | ( | ) | const |
Return the direction of this vector.
Definition at line 300 of file other_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 208 of file other_imp.cc.
|
virtual |
Returns true if this ObjectImp is equal to rhs.
This function checks whether rhs is of the same ObjectImp type, and whether it contains the same data as this ObjectImp.
It is used e.g. by the KigCommand stuff to see what the user has changed during a move.
Implements ObjectImp.
Definition at line 590 of file other_imp.cc.
|
virtual |
Reimplemented from CurveImp.
Definition at line 747 of file other_imp.cc.
|
virtual |
Implements CurveImp.
Definition at line 742 of file other_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 259 of file other_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 252 of file other_imp.cc.
Implements ObjectImp.
Definition at line 218 of file other_imp.cc.
bool VectorImp::internalContainsPoint | ( | const Coordinate & | p, |
double | threshold | ||
) | const |
Definition at line 766 of file other_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 678 of file other_imp.cc.
double VectorImp::length | ( | ) | const |
Return the length of this vector.
Definition at line 315 of file other_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 223 of file other_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 240 of file other_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 228 of file other_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 277 of file other_imp.cc.
|
static |
Returns the ObjectImpType representing the VectorImp type.
Definition at line 613 of file other_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 718 of file other_imp.cc.
|
virtual |
Return this ObjectImp, transformed by the transformation t.
Implements ObjectImp.
Definition at line 200 of file other_imp.cc.
|
virtual |
Returns the lowermost ObjectImpType that this object is an instantiation of.
E.g. if you want to get a string containing the internal name of the type of an object, you can do:
Implements ObjectImp.
Definition at line 651 of file other_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 310 of file other_imp.cc.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:12:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.