kig
#include <locus_imp.h>
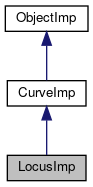
Public Types | |
typedef CurveImp | Parent |
![]() | |
typedef ObjectImp | Parent |
Public Member Functions | |
LocusImp (CurveImp *, const ObjectHierarchy &) | |
~LocusImp () | |
bool | contains (const Coordinate &p, int width, const KigWidget &) const |
bool | containsPoint (const Coordinate &p, const KigDocument &d) const |
LocusImp * | copy () const |
const CurveImp * | curve () const |
void | draw (KigPainter &p) const |
bool | equals (const ObjectImp &rhs) const |
const Coordinate | getPoint (double param, const KigDocument &) const |
const ObjectHierarchy & | hierarchy () const |
const char * | iconForProperty (int which) const |
const ObjectImpType * | impRequirementForProperty (int which) const |
bool | inRect (const Rect &r, int width, const KigWidget &) const |
bool | internalContainsPoint (const Coordinate &p, double threshold, const KigDocument &doc) const |
bool | isPropertyDefinedOnOrThroughThisImp (int which) const |
int | numberOfProperties () const |
const QByteArrayList | properties () const |
const QByteArrayList | propertiesInternalNames () const |
ObjectImp * | property (int which, const KigDocument &w) const |
Rect | surroundingRect () const |
ObjectImp * | transform (const Transformation &) const |
const ObjectImpType * | type () const |
void | visit (ObjectImpVisitor *vtor) const |
![]() | |
Coordinate | attachPoint () const |
QString | cartesianEquationString (const KigDocument &w) const |
virtual double | getParam (const Coordinate &point, const KigDocument &) const |
![]() | |
virtual | ~ObjectImp () |
virtual bool | canFillInNextEscape () const |
virtual void | fillInNextEscape (QString &s, const KigDocument &) const |
int | getPropGid (const char *pname) const |
int | getPropLid (int propgid) const |
const char * | getPropName (int propgid) const |
bool | inherits (const ObjectImpType *t) const |
virtual bool | isCache () const |
bool | valid () const |
Static Public Member Functions | |
static const ObjectImpType * | stype () |
![]() | |
static const ObjectImpType * | stype () |
![]() | |
static const ObjectImpType * | stype () |
Additional Inherited Members | |
![]() | |
double | getDist (double param, const Coordinate &p, const KigDocument &doc) const |
double | getParamofmin (double a, double b, const Coordinate &p, const KigDocument &doc) const |
![]() | |
ObjectImp () | |
Detailed Description
LocusImp is an imp that consists of a copy of the curveimp that the moving point moves over, and an ObjectHierarchy that can calc ( given a point, and optionally some more parent objects the position of the moving point.
The hierarchy should take the moving point as its first argument and all others after that. The others are used to make it possible for Locus to be updated when some of the other objects that appear in the path from the moving point to the dependent point change.
This may seem rather complicated, but I think it is absolutely necessary to support locuses using Kig's object system. It would be very bad for LocusImp to have to keep references to its parents as Objects ( since only the objects know how they are related to their parents ). This is how we used to do it, but the current method is far superior. First and foremost because the separation between ObjectImp and Object is something that Kig depends on very much, and because every ObjectImp should contain all the data it needs itself. ObjectImp's are entirely independent objects. That's also why we don't keep a pointer to the old CurveImp, but a copy of it.
i hope this is a bit clear, if not, feel free to ask for explanation of what you don't understand. NOTE: getParam has been moved by Petr Gajdos at the "curve_imp" level which should be fine, since it makes sense more generally for curves and not only for locuses. In particular it will be useful for the new "bezier" curves, to be added shortly
Definition at line 57 of file locus_imp.h.
Member Typedef Documentation
typedef CurveImp LocusImp::Parent |
Definition at line 65 of file locus_imp.h.
Constructor & Destructor Documentation
LocusImp::LocusImp | ( | CurveImp * | curve, |
const ObjectHierarchy & | hier | ||
) |
Definition at line 86 of file locus_imp.cc.
LocusImp::~LocusImp | ( | ) |
Definition at line 37 of file locus_imp.cc.
Member Function Documentation
|
virtual |
Implements ObjectImp.
Definition at line 52 of file locus_imp.cc.
|
virtual |
Return whether this Curve contains the given point.
This is implemented as a numerical approximation. Implementations can/should use the value test_threshold in common.h as a threshold value.
Implements CurveImp.
Definition at line 222 of file locus_imp.cc.
|
virtual |
Returns a copy of this ObjectImp.
The copy is an exact copy. Changes to the copy don't affect the original.
Implements CurveImp.
Definition at line 142 of file locus_imp.cc.
const CurveImp * LocusImp::curve | ( | ) | const |
Definition at line 147 of file locus_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 47 of file locus_imp.cc.
|
virtual |
Returns true if this ObjectImp is equal to rhs.
This function checks whether rhs is of the same ObjectImp type, and whether it contains the same data as this ObjectImp.
It is used e.g. by the KigCommand stuff to see what the user has changed during a move.
Implements ObjectImp.
Definition at line 193 of file locus_imp.cc.
|
virtual |
Implements CurveImp.
Definition at line 63 of file locus_imp.cc.
const ObjectHierarchy & LocusImp::hierarchy | ( | ) | const |
Definition at line 152 of file locus_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 117 of file locus_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 112 of file locus_imp.cc.
Implements ObjectImp.
Definition at line 57 of file locus_imp.cc.
bool LocusImp::internalContainsPoint | ( | const Coordinate & | p, |
double | threshold, | ||
const KigDocument & | doc | ||
) | const |
Definition at line 227 of file locus_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 234 of file locus_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 91 of file locus_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 104 of file locus_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 96 of file locus_imp.cc.
|
virtual |
Reimplemented from ObjectImp.
Definition at line 129 of file locus_imp.cc.
|
static |
Definition at line 200 of file locus_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 239 of file locus_imp.cc.
|
virtual |
Return this ObjectImp, transformed by the transformation t.
Implements ObjectImp.
Definition at line 42 of file locus_imp.cc.
|
virtual |
Returns the lowermost ObjectImpType that this object is an instantiation of.
E.g. if you want to get a string containing the internal name of the type of an object, you can do:
Implements ObjectImp.
Definition at line 217 of file locus_imp.cc.
|
virtual |
Implements ObjectImp.
Definition at line 188 of file locus_imp.cc.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:12:06 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.