kig
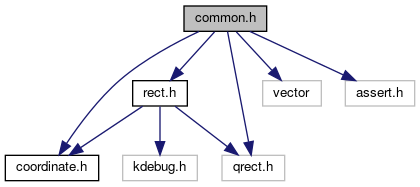

Go to the source code of this file.
Classes | |
class | LineData |
Functions | |
bool | areCollinear (const Coordinate &p1, const Coordinate &p2, const Coordinate &p3) |
const Coordinate | calcArcLineIntersect (const Coordinate &c, const double sqr, const double sa, const double angle, const LineData &l, int side) |
void | calcBorderPoints (Coordinate &p1, Coordinate &p2, const Rect &r) |
void | calcBorderPoints (double &xa, double &xb, double &ya, double &yb, const Rect &r) |
const LineData | calcBorderPoints (const LineData &l, const Rect &r) |
const Coordinate | calcCenter (const Coordinate &a, const Coordinate &b, const Coordinate &c) |
const Coordinate | calcCircleLineIntersect (const Coordinate &c, const double sqr, const LineData &l, int side) |
Coordinate | calcCircleRadicalStartPoint (const Coordinate &ca, const Coordinate &cb, double sqra, double sqrb) |
double | calcDistancePointLine (const Coordinate &p, const LineData &l) |
Coordinate | calcIntersectionPoint (const LineData &l, const LineData &m) |
const Coordinate | calcMirrorPoint (const LineData &l, const Coordinate &p) |
Coordinate | calcPointOnParallel (const LineData &l, const Coordinate &t) |
Coordinate | calcPointOnParallel (const Coordinate &dir, const Coordinate &t) |
Coordinate | calcPointOnPerpend (const LineData &l, const Coordinate &t) |
Coordinate | calcPointOnPerpend (const Coordinate &dir, const Coordinate &t) |
const Coordinate | calcPointProjection (const Coordinate &p, const LineData &l) |
void | calcRayBorderPoints (const Coordinate &a, Coordinate &b, const Rect &r) |
void | calcRayBorderPoints (const double xa, const double xb, double &ya, double &yb, const Rect &r) |
Coordinate | calcRotatedPoint (const Coordinate &a, const Coordinate &c, const double arc) |
double | getDoubleFromUser (const QString &caption, const QString &label, double value, QWidget *parent, bool *ok, double min, double max, int decimals) |
bool | isOnArc (const Coordinate &o, const Coordinate &c, const double r, const double sa, const double a, const double fault) |
bool | isOnLine (const Coordinate &o, const Coordinate &a, const Coordinate &b, const double fault) |
bool | isOnRay (const Coordinate &o, const Coordinate &a, const Coordinate &b, const double fault) |
bool | isOnSegment (const Coordinate &o, const Coordinate &a, const Coordinate &b, const double fault) |
bool | isSingular (const double &a, const double &b, const double &c, const double &d) |
template<typename T > | |
T | kigAbs (const T &a) |
template<typename T > | |
T | kigMax (const T &a, const T &b) |
template<typename T > | |
T | kigMin (const T &a, const T &b) |
template<typename T > | |
int | kigSgn (const T &a) |
bool | lineInRect (const Rect &r, const Coordinate &a, const Coordinate &b, const int width, const ObjectImp *imp, const KigWidget &w) |
bool | operator== (const LineData &l, const LineData &r) |
Variables | |
const double | double_inf |
const double | test_threshold |
Function Documentation
bool areCollinear | ( | const Coordinate & | p1, |
const Coordinate & | p2, | ||
const Coordinate & | p3 | ||
) |
test collinearity of three points
Definition at line 489 of file common.cpp.
const Coordinate calcArcLineIntersect | ( | const Coordinate & | c, |
const double | sqr, | ||
const double | sa, | ||
const double | angle, | ||
const LineData & | l, | ||
int | side | ||
) |
this calcs the intersection points of the arc with center c, radius sqrt( r ), start angle sa and angle angle, and the line l.
As a arc and a line can have max two intersection points, side tells us which one we need... It should be 1 or -1. If the line and the arc have no intersection, valid is set to false, otherwise to true... Note that sqr is the square of the radius. We do this to avoid rounding errors...
Definition at line 285 of file common.cpp.
void calcBorderPoints | ( | Coordinate & | p1, |
Coordinate & | p2, | ||
const Rect & | r | ||
) |
this sets p1 and p2 to p1' and p2' so that p1'p2' is the same line as p1p2, and so that p1' and p2' are on the border of the Rect...
Definition at line 82 of file common.cpp.
void calcBorderPoints | ( | double & | xa, |
double & | xb, | ||
double & | ya, | ||
double & | yb, | ||
const Rect & | r | ||
) |
overload...
Definition at line 94 of file common.cpp.
cleaner overload, intended to replace the above two...
Definition at line 87 of file common.cpp.
const Coordinate calcCenter | ( | const Coordinate & | a, |
const Coordinate & | b, | ||
const Coordinate & | c | ||
) |
This function calculates the center of the circle going through the three given points.
Definition at line 366 of file common.cpp.
const Coordinate calcCircleLineIntersect | ( | const Coordinate & | c, |
const double | sqr, | ||
const LineData & | l, | ||
int | side | ||
) |
this calcs the intersection points of the circle with center c and radius sqrt( r ), and the line l.
As a circle and a line have two intersection points, side tells us which one we need... It should be 1 or -1. If the line and the circle have no intersection, valid is set to false, otherwise to true... Note that sqr is the square of the radius. We do this to avoid rounding errors...
Definition at line 262 of file common.cpp.
Coordinate calcCircleRadicalStartPoint | ( | const Coordinate & | ca, |
const Coordinate & | cb, | ||
double | sqra, | ||
double | sqrb | ||
) |
Definition at line 335 of file common.cpp.
double calcDistancePointLine | ( | const Coordinate & | p, |
const LineData & | l | ||
) |
calc the distance of point p to the line through a and b...
Definition at line 302 of file common.cpp.
Coordinate calcIntersectionPoint | ( | const LineData & | l, |
const LineData & | m | ||
) |
this calcs the point where the lines l and m intersect...
Definition at line 57 of file common.cpp.
const Coordinate calcMirrorPoint | ( | const LineData & | l, |
const Coordinate & | p | ||
) |
calc the mirror point of p over the line l
Definition at line 250 of file common.cpp.
Coordinate calcPointOnParallel | ( | const LineData & | l, |
const Coordinate & | t | ||
) |
this returns a point, so that the line through point t and the point returned is parallel with the line l
Definition at line 47 of file common.cpp.
Coordinate calcPointOnParallel | ( | const Coordinate & | dir, |
const Coordinate & | t | ||
) |
this returns a point, so that the line through point t and the point returned is parallel with the direction given in dir...
Definition at line 52 of file common.cpp.
Coordinate calcPointOnPerpend | ( | const LineData & | l, |
const Coordinate & | t | ||
) |
this returns a point, so that the line through point t and the point returned is perpendicular to the line l.
this returns a point, so that the line through point t and the point returned is perpendicular to the line l.
Copyright (C) 2002 Dominique Devriese devri ese@ kde.o rg
This program is free software; you can redistribute it and/or modify it under the terms of the GNU General Public License as published by the Free Software Foundation; either version 2 of the License, or (at your option) any later version.
This program is distributed in the hope that it will be useful, but WITHOUT ANY WARRANTY; without even the implied warranty of MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU General Public License for more details.
You should have received a copy of the GNU General Public License along with this program; if not, write to the Free Software Foundation, Inc., 51 Franklin Street, Fifth Floor, Boston, MA 02110-1301 USA
Definition at line 37 of file common.cpp.
Coordinate calcPointOnPerpend | ( | const Coordinate & | dir, |
const Coordinate & | t | ||
) |
this returns a point, so that the line through point t and the point returned is perpendicular to the direction given in dir...
Definition at line 42 of file common.cpp.
const Coordinate calcPointProjection | ( | const Coordinate & | p, |
const LineData & | l | ||
) |
this calculates the perpendicular projection of point p on line ab...
Definition at line 295 of file common.cpp.
void calcRayBorderPoints | ( | const Coordinate & | a, |
Coordinate & | b, | ||
const Rect & | r | ||
) |
this does the same as the above function, but only for b.
Definition at line 131 of file common.cpp.
void calcRayBorderPoints | ( | const double | xa, |
const double | xb, | ||
double & | ya, | ||
double & | yb, | ||
const Rect & | r | ||
) |
overload...
Definition at line 136 of file common.cpp.
Coordinate calcRotatedPoint | ( | const Coordinate & | a, |
const Coordinate & | c, | ||
const double | arc | ||
) |
This calcs the rotation of point a around point c by arc arc.
Arc is in radians, in the range 0 < arc < 2*pi ...
Definition at line 315 of file common.cpp.
double getDoubleFromUser | ( | const QString & | caption, |
const QString & | label, | ||
double | value, | ||
QWidget * | parent, | ||
bool * | ok, | ||
double | min, | ||
double | max, | ||
int | decimals | ||
) |
Here, we define some algorithms which we need in various places...
Definition at line 349 of file common.cpp.
bool isOnArc | ( | const Coordinate & | o, |
const Coordinate & | c, | ||
const double | r, | ||
const double | sa, | ||
const double | a, | ||
const double | fault | ||
) |
Definition at line 238 of file common.cpp.
bool isOnLine | ( | const Coordinate & | o, |
const Coordinate & | a, | ||
const Coordinate & | b, | ||
const double | fault | ||
) |
is o on the line defined by point a and point b ? fault is the allowed difference...
Definition at line 188 of file common.cpp.
bool isOnRay | ( | const Coordinate & | o, |
const Coordinate & | a, | ||
const Coordinate & | b, | ||
const double | fault | ||
) |
Definition at line 226 of file common.cpp.
bool isOnSegment | ( | const Coordinate & | o, |
const Coordinate & | a, | ||
const Coordinate & | b, | ||
const double | fault | ||
) |
is o on the segment defined by point a and point b ? this calls isOnLine(), but also checks if o is "between" a and b...
fault is the allowed difference...
Definition at line 212 of file common.cpp.
bool isSingular | ( | const double & | a, |
const double & | b, | ||
const double & | c, | ||
const double & | d | ||
) |
test if a 2x2 matrix is singular (relatively to the norm of the two row vectors)
Definition at line 495 of file common.cpp.
T kigAbs | ( | const T & | a | ) |
Definition at line 267 of file misc/common.h.
T kigMax | ( | const T & | a, |
const T & | b | ||
) |
Definition at line 261 of file misc/common.h.
T kigMin | ( | const T & | a, |
const T & | b | ||
) |
Definition at line 255 of file misc/common.h.
int kigSgn | ( | const T & | a | ) |
Definition at line 273 of file misc/common.h.
bool lineInRect | ( | const Rect & | r, |
const Coordinate & | a, | ||
const Coordinate & | b, | ||
const int | width, | ||
const ObjectImp * | imp, | ||
const KigWidget & | w | ||
) |
Is the line, segment, ray or vector inside r ? We need the imp to distinguish between rays, lines, segments or whatever.
. ( we use their contains functions actually.. )
Definition at line 401 of file common.cpp.
Variable Documentation
const double double_inf |
Definition at line 509 of file common.cpp.
const double test_threshold |
Definition at line 510 of file common.cpp.
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:12:05 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.