kiten/lib
#include <entry.h>
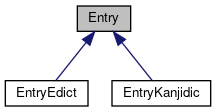
Public Member Functions | |
virtual | ~Entry () |
virtual Entry * | clone () const =0 |
virtual QString | dumpEntry () const =0 |
virtual bool | extendedItemCheck (const QString &key, const QString &value) const |
virtual QString | getDictionaryType () const =0 |
const QString & | getDictName () const |
const QHash< QString, QString > & | getExtendedInfo () const |
QString | getExtendedInfoItem (const QString &x) const |
QString | getMeanings () const |
QStringList | getMeaningsList () const |
QString | getReadings () const |
QStringList | getReadingsList () const |
QString | getWord () const |
virtual bool | loadEntry (const QString &)=0 |
virtual bool | matchesQuery (const DictQuery &) const |
virtual bool | sort (const Entry &that, const QStringList &dictionaryList, const QStringList &fieldList) const |
virtual bool | sortByField (const Entry &that, const QString &field) const |
virtual QString | toHTML () const |
virtual QString | toKVTML () const |
virtual QString | toString () const |
Protected Member Functions | |
Entry (const Entry &) | |
Entry (const QString &sourceDictionary) | |
Entry (const QString &sourceDictionary, const QString &word, const QStringList &readings, const QStringList &meanings) | |
virtual QString | HTMLMeanings () const |
virtual QString | HTMLReadings () const |
virtual QString | HTMLWord () const |
void | init () |
bool | isKanji (const QChar &character) const |
bool | listMatch (const QStringList &list, const QStringList &test, DictQuery::MatchType type) const |
virtual QString | makeLink (const QString &entryString) const |
Protected Attributes | |
QHash< QString, QString > | ExtendedInfo |
QStringList | Meanings |
QString | outputListDelimiter |
QStringList | Readings |
QString | sourceDict |
QString | Word |
Detailed Description
The Entry class is a generic base class for each particular entry in a given dictionary.
It's used as the basic class to ferry information back to the user application. It also handles some of the display aspects.
Constructor & Destructor Documentation
|
protected |
|
protected |
Constructor that includes the dictionary source.
This does not need to be overridded by subclasses, but you might find it to be convenient as the superclass constructor to call from your constructors.
- Parameters
-
sourceDictionary the dictionary name (not fileName) that this entry originated with
|
protected |
A constructor that includes the basic information, nicely separated.
- Parameters
-
sourceDictionary the dictionary name (not fileName) that this entry originated with word the word entry of this dictionary entry (normally kanji/kana) readings a list of possible pronunciations for this result (kana) meanings a list of possible meanings for this word
Member Function Documentation
|
pure virtual |
A clone method, this should just implement "return new EntrySubClass(*this)".
Implemented in EntryEdict, and EntryKanjidic.
|
pure virtual |
Return a QString of an entry, as if it were dumped back into it's source file.
Implemented in EntryEdict, and EntryKanjidic.
Simple accessor.
- Parameters
-
key the key for the extended item that is being verified value the value it is supposed to have
- Returns
- true if the key has that value, false if it is different or does not exist
Reimplemented in EntryKanjidic.
|
pure virtual |
const QString & Entry::getDictName | ( | ) | const |
QString Entry::getMeanings | ( | ) | const |
QStringList Entry::getMeaningsList | ( | ) | const |
QStringList Entry::getReadingsList | ( | ) | const |
QString Entry::getWord | ( | ) | const |
|
inlineprotectedvirtual |
|
inlineprotectedvirtual |
Return and HTML version of a reading list.
Reimplemented in EntryKanjidic.
|
inlineprotectedvirtual |
Return and HTML version of a word.
Prepares Word for output as HTML.
Reimplemented in EntryEdict, and EntryKanjidic.
|
protected |
|
protected |
|
protected |
|
pure virtual |
An entry should be able to parse an in-file representation of an entry as a QString and put it back.
The latter will be useful for writing to dictionaries on disk at some point.
Implemented in EntryEdict, and EntryKanjidic.
|
virtual |
Fairly important method, this tests if this particular entry matches a query.
The EDICT and Kanjidic doSearch methods do an approximate match, load an Entry, and then check more carefully by calling this method. This works nicely for handling searchWithinResults cleanly.
|
virtual |
An overrideable sorting function, similer to operator< in most contexts The default version will sort by dictionary, then by fields.
This version of sort only sorts dictionaries...
- Parameters
-
that the second item we are comparing (this) with dictionaryList the list of dictionaries (in order) to sort If this list is empty, the entries will not be sorted in order fieldList the list of fields to sort in, uses special codes of Reading, Meaning, Word/Kanji for those elements, all others by their extended attribute keys.
This is a replacement for a operator< function... so we return true if "this" should show up first on the list.
Overrideable sorting mechanism for sorting by individual fields.
The sort routine checks if the given field is equal, before calling this virtual function So if this is called, you can assume that this->extendedItem(field) != that.extendedItem(field)
- Parameters
-
that the second item we are comparing (this) with field the specific extended item field that is being compared
|
virtual |
An entry should be able to generate a representation of itself in (valid) HTML.
Main switching function for displaying to the user.
Reimplemented in EntryEdict, and EntryKanjidic.
|
inlinevirtual |
|
virtual |
Member Data Documentation
|
protected |
|
protected |
|
protected |
|
protected |
|
protected |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:16:38 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.