kiten/lib
#include <entryedict.h>
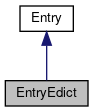
Public Member Functions | |
EntryEdict (const QString &dict) | |
EntryEdict (const QString &dict, const QString &entry) | |
Entry * | clone () const |
virtual QString | dumpEntry () const |
virtual QString | getDictionaryType () const |
QString | getTypes () const |
QStringList | getTypesList () const |
virtual QString | HTMLWord () const |
bool | isAdjective () const |
bool | isAdverb () const |
bool | isCommon () const |
bool | isExpression () const |
bool | isFukisokuVerb () const |
bool | isGodanVerb () const |
bool | isIchidanVerb () const |
bool | isNoun () const |
bool | isParticle () const |
bool | isPrefix () const |
bool | isSuffix () const |
bool | isVerb () const |
virtual bool | loadEntry (const QString &entryLine) |
bool | matchesWordType (const DictQuery &query) const |
virtual QString | toHTML () const |
![]() | |
virtual | ~Entry () |
virtual bool | extendedItemCheck (const QString &key, const QString &value) const |
const QString & | getDictName () const |
const QHash< QString, QString > & | getExtendedInfo () const |
QString | getExtendedInfoItem (const QString &x) const |
QString | getMeanings () const |
QStringList | getMeaningsList () const |
QString | getReadings () const |
QStringList | getReadingsList () const |
QString | getWord () const |
virtual bool | matchesQuery (const DictQuery &) const |
virtual bool | sort (const Entry &that, const QStringList &dictionaryList, const QStringList &fieldList) const |
virtual bool | sortByField (const Entry &that, const QString &field) const |
virtual QString | toKVTML () const |
virtual QString | toString () const |
Protected Member Functions | |
virtual QString | kanjiLinkify (const QString &inString) const |
![]() | |
Entry (const Entry &) | |
Entry (const QString &sourceDictionary) | |
Entry (const QString &sourceDictionary, const QString &word, const QStringList &readings, const QStringList &meanings) | |
virtual QString | HTMLMeanings () const |
virtual QString | HTMLReadings () const |
void | init () |
bool | isKanji (const QChar &character) const |
bool | listMatch (const QStringList &list, const QStringList &test, DictQuery::MatchType type) const |
virtual QString | makeLink (const QString &entryString) const |
Additional Inherited Members | |
![]() | |
QHash< QString, QString > | ExtendedInfo |
QStringList | Meanings |
QString | outputListDelimiter |
QStringList | Readings |
QString | sourceDict |
QString | Word |
Detailed Description
Definition at line 60 of file entryedict.h.
Constructor & Destructor Documentation
EntryEdict::EntryEdict | ( | const QString & | dict | ) |
Definition at line 33 of file entryedict.cpp.
Definition at line 38 of file entryedict.cpp.
Member Function Documentation
|
virtual |
A clone method, this should just implement "return new EntrySubClass(*this)".
Implements Entry.
Definition at line 44 of file entryedict.cpp.
|
virtual |
Regenerate a QString like the one we got in loadEntry()
Implements Entry.
Definition at line 52 of file entryedict.cpp.
|
virtual |
Get the dictionary type (e.g.
edict, kanjidic).
Implements Entry.
Definition at line 61 of file entryedict.cpp.
QString EntryEdict::getTypes | ( | ) | const |
Simple accessor.
Definition at line 66 of file entryedict.cpp.
QStringList EntryEdict::getTypesList | ( | ) | const |
Simple accessor.
Definition at line 71 of file entryedict.cpp.
|
virtual |
Return and HTML version of a word.
Prepares Word for output as HTML.
Reimplemented from Entry.
Definition at line 216 of file entryedict.cpp.
bool EntryEdict::isAdjective | ( | ) | const |
Definition at line 76 of file entryedict.cpp.
bool EntryEdict::isAdverb | ( | ) | const |
Definition at line 89 of file entryedict.cpp.
bool EntryEdict::isCommon | ( | ) | const |
Definition at line 102 of file entryedict.cpp.
bool EntryEdict::isExpression | ( | ) | const |
Definition at line 107 of file entryedict.cpp.
bool EntryEdict::isFukisokuVerb | ( | ) | const |
Definition at line 120 of file entryedict.cpp.
bool EntryEdict::isGodanVerb | ( | ) | const |
Definition at line 133 of file entryedict.cpp.
bool EntryEdict::isIchidanVerb | ( | ) | const |
Definition at line 146 of file entryedict.cpp.
bool EntryEdict::isNoun | ( | ) | const |
Definition at line 159 of file entryedict.cpp.
bool EntryEdict::isParticle | ( | ) | const |
Definition at line 172 of file entryedict.cpp.
bool EntryEdict::isPrefix | ( | ) | const |
Definition at line 177 of file entryedict.cpp.
bool EntryEdict::isSuffix | ( | ) | const |
Definition at line 190 of file entryedict.cpp.
bool EntryEdict::isVerb | ( | ) | const |
Definition at line 203 of file entryedict.cpp.
Makes a link out of each kanji in.
- Parameters
-
inString
Definition at line 225 of file entryedict.cpp.
|
virtual |
Take a QString and load it into the Entry as appropriate The format is basically: KANJI [KANA] /(general information) gloss/gloss/.../ Note that they can rudely place more (general information) in gloss's that are not the first one.
Implements Entry.
Definition at line 250 of file entryedict.cpp.
bool EntryEdict::matchesWordType | ( | const DictQuery & | query | ) | const |
Definition at line 333 of file entryedict.cpp.
|
virtual |
Returns a HTML version of an Entry.
Reimplemented from Entry.
Definition at line 384 of file entryedict.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:16:38 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.