kiten/lib
#include <entrylist.h>
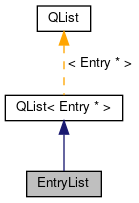
Public Types | |
typedef QListIterator< Entry * > | EntryIterator |
Public Member Functions | |
EntryList () | |
EntryList (const EntryList &old) | |
virtual | ~EntryList () |
void | appendList (const EntryList *other) |
void | deleteAll () |
DictQuery | getQuery () const |
const EntryList & | operator+= (const EntryList &other) |
const EntryList & | operator= (const EntryList &other) |
int | scrollValue () const |
void | setQuery (const DictQuery &newQuery) |
void | setScrollValue (int val) |
void | sort (QStringList &sortOrder, QStringList &dictionaryOrder) |
QString | toHTML () const |
QString | toHTML (unsigned int start, unsigned int length) const |
QString | toKVTML (unsigned int start, unsigned int length) const |
QString | toString () const |
QString | toString (unsigned int start, unsigned int length) const |
![]() | |
QList () | |
QList (const QList< T > &other) | |
QList (std::initializer_list< T > args) | |
~QList () | |
void | append (const T &value) |
void | append (const QList< T > &value) |
const T & | at (int i) const |
T & | back () |
const T & | back () const |
iterator | begin () |
const_iterator | begin () const |
void | clear () |
const_iterator | constBegin () const |
const_iterator | constEnd () const |
bool | contains (const T &value) const |
int | count (const T &value) const |
int | count () const |
bool | empty () const |
iterator | end () |
const_iterator | end () const |
bool | endsWith (const T &value) const |
iterator | erase (iterator pos) |
iterator | erase (iterator begin, iterator end) |
iterator | find (iterator from, const T &t) |
iterator | find (const T &t) |
const_iterator | find (const T &t) const |
const_iterator | find (const_iterator from, const T &t) const |
int | findIndex (const T &t) const |
T & | first () |
const T & | first () const |
T & | front () |
const T & | front () const |
int | indexOf (const T &value, int from) const |
void | insert (int i, const T &value) |
iterator | insert (iterator before, const T &value) |
bool | isEmpty () const |
T & | last () |
const T & | last () const |
int | lastIndexOf (const T &value, int from) const |
int | length () const |
QList< T > | mid (int pos, int length) const |
void | move (int from, int to) |
bool | operator!= (const QList< T > &other) const |
QList< T > | operator+ (const QList< T > &other) const |
QList< T > & | operator+= (const QList< T > &other) |
QList< T > & | operator+= (const T &value) |
QList< T > & | operator<< (const T &value) |
QList< T > & | operator<< (const QList< T > &other) |
QList< T > & | operator= (const QList< T > &other) |
bool | operator== (const QList< T > &other) const |
T & | operator[] (int i) |
const T & | operator[] (int i) const |
void | pop_back () |
void | pop_front () |
void | prepend (const T &value) |
void | push_back (const T &value) |
void | push_front (const T &value) |
iterator | remove (iterator pos) |
int | remove (const T &t) |
int | removeAll (const T &value) |
void | removeAt (int i) |
void | removeFirst () |
void | removeLast () |
bool | removeOne (const T &value) |
void | replace (int i, const T &value) |
void | reserve (int alloc) |
int | size () const |
bool | startsWith (const T &value) const |
void | swap (int i, int j) |
void | swap (QList< T > &other) |
T | takeAt (int i) |
T | takeFirst () |
T | takeLast () |
QSet< T > | toSet () const |
std::list< T > | toStdList () const |
QVector< T > | toVector () const |
T | value (int i, const T &defaultValue) const |
T | value (int i) const |
Additional Inherited Members | |
![]() | |
QList< T > | fromSet (const QSet< T > &set) |
QList< T > | fromStdList (const std::list< T > &list) |
QList< T > | fromVector (const QVector< T > &vector) |
![]() | |
typedef | const_pointer |
typedef | const_reference |
typedef | ConstIterator |
typedef | difference_type |
typedef | Iterator |
typedef | pointer |
typedef | reference |
typedef | size_type |
typedef | value_type |
Detailed Description
EntryList is a simple container for Entry objects, and is-a QList<Entry*> A few simple overrides allow you to deal with sorting and translating.
Definition at line 38 of file entrylist.h.
Member Typedef Documentation
A simple overridden iterator for working with the Entries.
Definition at line 44 of file entrylist.h.
Constructor & Destructor Documentation
EntryList::EntryList | ( | ) |
Basic constructor, create an empty EntryList.
Definition at line 71 of file entrylist.cpp.
EntryList::EntryList | ( | const EntryList & | old | ) |
Copy constructor.
Definition at line 77 of file entrylist.cpp.
|
virtual |
Basic Destructor, does not delete Entry* objects.
Please remember to call deleteAll() before deleting an EntryList.
Definition at line 83 of file entrylist.cpp.
Member Function Documentation
void EntryList::appendList | ( | const EntryList * | other | ) |
Append another EntryList onto this one.
Definition at line 306 of file entrylist.cpp.
void EntryList::deleteAll | ( | ) |
Delete all Entry objects in our list.
In the future, we'll switch to a reference counting system, and this will be deprecated.
Definition at line 99 of file entrylist.cpp.
DictQuery EntryList::getQuery | ( | ) | const |
Get the query that generated this list, note that if you have appended EntryLists from two different queries, the resulting DictQuery from this is undefined.
This method retrieves an earlier saved query in the EntryList, this should be the query that generated the list.
Definition at line 323 of file entrylist.cpp.
Append another EntryList onto this one.
Definition at line 284 of file entrylist.cpp.
Copy an entry list.
Definition at line 298 of file entrylist.cpp.
int EntryList::scrollValue | ( | ) | const |
Definition at line 89 of file entrylist.cpp.
void EntryList::setQuery | ( | const DictQuery & | newQuery | ) |
Set the query for this list.
This allows us to save a query in the EntryList for later retrieval.
See getQuery() for a potential problem with this
Definition at line 331 of file entrylist.cpp.
void EntryList::setScrollValue | ( | int | val | ) |
Definition at line 94 of file entrylist.cpp.
void EntryList::sort | ( | QStringList & | sortOrder, |
QStringList & | dictionaryOrder | ||
) |
Sort the list according to the given fields in sortOrder, if dictionaryOrder is blank, don't order the list by dictionary, otherwise items are sorted by dictionary then by sortOrder aspects.
- Parameters
-
sortOrder the keys to sort by, this should be a list of fields to sort by, this should be the same as the fields that are returned from dictFile::listDictDisplayOptions(). "--NewLine--" entries will be ignored, "Word/Kanji", "Meaning", and "Reading" entries will be accepted. An entry which has an extended attribute is considered higher ranking (sorted to a higher position) than an entry which does not have such an attribute. dictionaryOrder the order for the Entry objects to be sorted in, dictionary-wise. This should match the names of the dictionary objects, passed to the DictionaryManager.
Definition at line 271 of file entrylist.cpp.
QString EntryList::toHTML | ( | ) | const |
Convert every element of the EntryList to a QString in HTML form and return it.
Definition at line 230 of file entrylist.cpp.
QString EntryList::toHTML | ( | unsigned int | start, |
unsigned int | length | ||
) | const |
Convert a given range of the EntryList to a QString in HTML form and return it.
- Parameters
-
start the location in the list where we should start length the length of the list we should generate
Definition at line 111 of file entrylist.cpp.
QString EntryList::toKVTML | ( | unsigned int | start, |
unsigned int | length | ||
) | const |
Convert the entire list to KVTML for export to a flashcard app.
- Parameters
-
start the location in the list where we should start length the length of the list we should generate
Definition at line 200 of file entrylist.cpp.
QString EntryList::toString | ( | ) | const |
Convert every element of the EntryList to a QString and return it.
Definition at line 266 of file entrylist.cpp.
QString EntryList::toString | ( | unsigned int | start, |
unsigned int | length | ||
) | const |
Convert a given range of the EntryList to a QString and return it.
- Parameters
-
start the location in the list where we should start length the length of the list we should generate
Definition at line 238 of file entrylist.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:16:38 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.