marble
#include <MarbleAbstractPresenter.h>
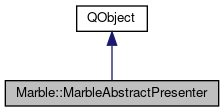
Public Slots | |
void | centerOn (const qreal lon, const qreal lat, bool animated=false) |
void | centerOn (const GeoDataCoordinates &point, bool animated=false) |
void | centerOn (const GeoDataLatLonBox &box, bool animated=false) |
void | centerOn (const GeoDataPlacemark &placemark, bool animated=false) |
void | flyTo (const GeoDataLookAt &newLookAt, FlyToMode mode=Automatic) |
void | goHome (FlyToMode mode=Automatic) |
void | rotateBy (const qreal deltaLon, const qreal deltaLat, FlyToMode mode=Instant) |
void | setAnimationsEnabled (bool enabled) |
void | setCenterLatitude (qreal lat, FlyToMode mode) |
void | setCenterLongitude (qreal lon, FlyToMode mode) |
void | setDistance (qreal newDistance) |
void | setRadius (int radius) |
void | setSelection (const QRect ®ion) |
void | setViewContext (ViewContext viewContext) |
void | setZoom (int newZoom, FlyToMode mode=Instant) |
void | zoomIn (FlyToMode mode=Automatic) |
void | zoomOut (FlyToMode mode=Automatic) |
void | zoomView (int zoom, FlyToMode mode=Instant) |
void | zoomViewBy (int zoomStep, FlyToMode mode=Instant) |
Signals | |
void | distanceChanged (const QString &distanceString) |
void | regionSelected (const QList< double > &) |
void | updateRequired () |
void | zoomChanged (int zoom) |
Public Member Functions | |
MarbleAbstractPresenter () | |
virtual | ~MarbleAbstractPresenter () |
bool | animationsEnabled () const |
qreal | centerLatitude () const |
qreal | centerLongitude () const |
qreal | distance () const |
qreal | distanceFromRadius (qreal radius) const |
qreal | distanceFromZoom (qreal zoom) const |
QString | distanceString () const |
void | initialize (MarbleModel *model, MarbleMap *map) |
int | logzoom () const |
GeoDataLookAt | lookAt () const |
MarbleMap * | map () |
const MarbleMap * | map () const |
int | maximumZoom () const |
int | minimumZoom () const |
MarbleModel * | model () |
const MarbleModel * | model () const |
void | moveByStep (int stepsRight, int stepsDown, FlyToMode mode=Automatic) |
qreal | moveStep () const |
void | moveTo (const QPoint &pos, qreal factor) |
int | polarity () const |
int | radius () const |
qreal | radius (qreal zoom) const |
qreal | radiusFromDistance (qreal distance) const |
void | setLogzoom (int value) |
qreal | viewAngle () const |
ViewContext | viewContext () const |
ViewportParams * | viewport () |
const ViewportParams * | viewport () const |
int | zoom () const |
qreal | zoom (qreal radius) const |
void | zoomAt (const QPoint &pos, qreal newDistance) |
qreal | zoomFromDistance (qreal distance) const |
int | zoomStep () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Definition at line 26 of file MarbleAbstractPresenter.h.
Constructor & Destructor Documentation
Marble::MarbleAbstractPresenter::MarbleAbstractPresenter | ( | ) |
Definition at line 31 of file MarbleAbstractPresenter.cpp.
|
virtual |
Definition at line 43 of file MarbleAbstractPresenter.cpp.
Member Function Documentation
bool Marble::MarbleAbstractPresenter::animationsEnabled | ( | ) | const |
Definition at line 514 of file MarbleAbstractPresenter.cpp.
qreal Marble::MarbleAbstractPresenter::centerLatitude | ( | ) | const |
Definition at line 482 of file MarbleAbstractPresenter.cpp.
qreal Marble::MarbleAbstractPresenter::centerLongitude | ( | ) | const |
Definition at line 487 of file MarbleAbstractPresenter.cpp.
|
slot |
Definition at line 410 of file MarbleAbstractPresenter.cpp.
|
slot |
Definition at line 416 of file MarbleAbstractPresenter.cpp.
|
slot |
Definition at line 423 of file MarbleAbstractPresenter.cpp.
|
slot |
Definition at line 450 of file MarbleAbstractPresenter.cpp.
qreal Marble::MarbleAbstractPresenter::distance | ( | ) | const |
Definition at line 143 of file MarbleAbstractPresenter.cpp.
|
signal |
qreal Marble::MarbleAbstractPresenter::distanceFromRadius | ( | qreal | radius | ) | const |
Definition at line 148 of file MarbleAbstractPresenter.cpp.
qreal Marble::MarbleAbstractPresenter::distanceFromZoom | ( | qreal | zoom | ) | const |
Definition at line 266 of file MarbleAbstractPresenter.cpp.
QString Marble::MarbleAbstractPresenter::distanceString | ( | ) | const |
Definition at line 105 of file MarbleAbstractPresenter.cpp.
|
slot |
Definition at line 77 of file MarbleAbstractPresenter.cpp.
Definition at line 276 of file MarbleAbstractPresenter.cpp.
void Marble::MarbleAbstractPresenter::initialize | ( | MarbleModel * | model, |
MarbleMap * | map | ||
) |
int Marble::MarbleAbstractPresenter::logzoom | ( | ) | const |
Definition at line 524 of file MarbleAbstractPresenter.cpp.
GeoDataLookAt Marble::MarbleAbstractPresenter::lookAt | ( | ) | const |
Definition at line 131 of file MarbleAbstractPresenter.cpp.
MarbleMap * Marble::MarbleAbstractPresenter::map | ( | ) |
Definition at line 544 of file MarbleAbstractPresenter.cpp.
const MarbleMap * Marble::MarbleAbstractPresenter::map | ( | ) | const |
Definition at line 549 of file MarbleAbstractPresenter.cpp.
int Marble::MarbleAbstractPresenter::maximumZoom | ( | ) | const |
Definition at line 185 of file MarbleAbstractPresenter.cpp.
int Marble::MarbleAbstractPresenter::minimumZoom | ( | ) | const |
Definition at line 180 of file MarbleAbstractPresenter.cpp.
MarbleModel * Marble::MarbleAbstractPresenter::model | ( | ) |
Definition at line 554 of file MarbleAbstractPresenter.cpp.
const MarbleModel * Marble::MarbleAbstractPresenter::model | ( | ) | const |
Definition at line 559 of file MarbleAbstractPresenter.cpp.
void Marble::MarbleAbstractPresenter::moveByStep | ( | int | stepsRight, |
int | stepsDown, | ||
FlyToMode | mode = Automatic |
||
) |
Rotate the globe in the given direction in discrete steps.
- Parameters
-
stepsRight Number of steps to go right. Negative values go left. stepsDown Number of steps to go down. Negative values go up. mode Interpolation mode to use when traveling to the target
Definition at line 291 of file MarbleAbstractPresenter.cpp.
qreal Marble::MarbleAbstractPresenter::moveStep | ( | ) | const |
Definition at line 299 of file MarbleAbstractPresenter.cpp.
void Marble::MarbleAbstractPresenter::moveTo | ( | const QPoint & | pos, |
qreal | factor | ||
) |
Definition at line 393 of file MarbleAbstractPresenter.cpp.
int Marble::MarbleAbstractPresenter::polarity | ( | ) | const |
Definition at line 170 of file MarbleAbstractPresenter.cpp.
int Marble::MarbleAbstractPresenter::radius | ( | ) | const |
Definition at line 311 of file MarbleAbstractPresenter.cpp.
qreal Marble::MarbleAbstractPresenter::radius | ( | qreal | zoom | ) | const |
Definition at line 52 of file MarbleAbstractPresenter.cpp.
qreal Marble::MarbleAbstractPresenter::radiusFromDistance | ( | qreal | distance | ) | const |
Definition at line 164 of file MarbleAbstractPresenter.cpp.
|
signal |
This signal is emitted when a new rectangle region is selected over the map The list of double values includes coordinates in degrees using the following: lon1, lat1, lon2, lat2 (or West, North, East, South) as left/top, right/bottom rectangle.
|
slot |
Definition at line 57 of file MarbleAbstractPresenter.cpp.
|
slot |
Definition at line 519 of file MarbleAbstractPresenter.cpp.
|
slot |
Definition at line 472 of file MarbleAbstractPresenter.cpp.
|
slot |
Definition at line 477 of file MarbleAbstractPresenter.cpp.
|
slot |
Definition at line 574 of file MarbleAbstractPresenter.cpp.
void Marble::MarbleAbstractPresenter::setLogzoom | ( | int | value | ) |
Definition at line 529 of file MarbleAbstractPresenter.cpp.
|
slot |
Definition at line 316 of file MarbleAbstractPresenter.cpp.
|
slot |
Definition at line 588 of file MarbleAbstractPresenter.cpp.
|
slot |
Definition at line 497 of file MarbleAbstractPresenter.cpp.
Definition at line 190 of file MarbleAbstractPresenter.cpp.
|
signal |
qreal Marble::MarbleAbstractPresenter::viewAngle | ( | ) | const |
Definition at line 539 of file MarbleAbstractPresenter.cpp.
ViewContext Marble::MarbleAbstractPresenter::viewContext | ( | ) | const |
Definition at line 492 of file MarbleAbstractPresenter.cpp.
ViewportParams * Marble::MarbleAbstractPresenter::viewport | ( | ) |
Definition at line 564 of file MarbleAbstractPresenter.cpp.
const ViewportParams * Marble::MarbleAbstractPresenter::viewport | ( | ) | const |
Definition at line 569 of file MarbleAbstractPresenter.cpp.
int Marble::MarbleAbstractPresenter::zoom | ( | ) | const |
Definition at line 175 of file MarbleAbstractPresenter.cpp.
qreal Marble::MarbleAbstractPresenter::zoom | ( | qreal | radius | ) | const |
Definition at line 47 of file MarbleAbstractPresenter.cpp.
void Marble::MarbleAbstractPresenter::zoomAt | ( | const QPoint & | pos, |
qreal | newDistance | ||
) |
Definition at line 348 of file MarbleAbstractPresenter.cpp.
|
signal |
qreal Marble::MarbleAbstractPresenter::zoomFromDistance | ( | qreal | distance | ) | const |
Definition at line 271 of file MarbleAbstractPresenter.cpp.
Definition at line 230 of file MarbleAbstractPresenter.cpp.
Definition at line 248 of file MarbleAbstractPresenter.cpp.
int Marble::MarbleAbstractPresenter::zoomStep | ( | ) | const |
Definition at line 534 of file MarbleAbstractPresenter.cpp.
Definition at line 220 of file MarbleAbstractPresenter.cpp.
Definition at line 225 of file MarbleAbstractPresenter.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:13:45 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.