rocs/RocsCore
#include <GraphFilePluginInterface.h>
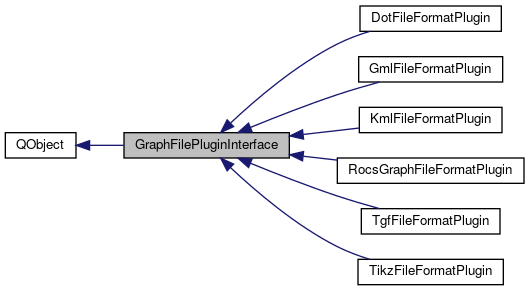
Public Types | |
enum | Error { None, Unknown, FileIsReadOnly, CouldNotOpenFile, NoGraphFound, EncodingProblem, CouldNotRecognizeFileFormat, NotSupportedOperation } |
enum | PluginType { ImportOnly, ExportOnly, ImportAndExport } |
Public Member Functions | |
GraphFilePluginInterface (const KAboutData *aboutData, QObject *parent) | |
virtual | ~GraphFilePluginInterface () |
const KAboutData * | aboutData () const |
Error | error () const |
QString | errorString () const |
virtual const QStringList | extensions () const =0 |
virtual Document * | graphDocument () const |
bool | hasError () const |
virtual bool | isGraphDocument () const |
virtual PluginType | pluginCapability () const |
virtual void | readFile ()=0 |
void | setFile (const KUrl &file) |
virtual void | writeFile (Document &graph)=0 |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
const KUrl & | file () const |
void | setError (Error error, QString message=QString()) |
void | setGraphDocument (Document *document) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
This class provides an interface for graph file format plugins.
A graph file format plugin must provide implementations of
- writeFile(...)
- readFile()
- extensions() and optionally, if it is not a read and write plugin, an implementation of
- pluginCapability() to specify the plugin capabilities.
Definition at line 42 of file GraphFilePluginInterface.h.
Member Enumeration Documentation
Describes the last error of the plugin.
Enumerator | |
---|---|
None | |
Unknown | |
FileIsReadOnly | |
CouldNotOpenFile | |
NoGraphFound | |
EncodingProblem | |
CouldNotRecognizeFileFormat | |
NotSupportedOperation |
Definition at line 50 of file GraphFilePluginInterface.h.
Describes the capability of the plugin, i.e., if the plugin can be used to read and/or write files.
Enumerator | |
---|---|
ImportOnly | |
ExportOnly | |
ImportAndExport |
Definition at line 65 of file GraphFilePluginInterface.h.
Constructor & Destructor Documentation
GraphFilePluginInterface::GraphFilePluginInterface | ( | const KAboutData * | aboutData, |
QObject * | parent | ||
) |
Constructor.
- Parameters
-
aboutData is description of the plugin parent is the object parent
Definition at line 47 of file GraphFilePluginInterface.cpp.
|
virtual |
Definition at line 54 of file GraphFilePluginInterface.cpp.
Member Function Documentation
const KAboutData * GraphFilePluginInterface::aboutData | ( | ) | const |
- Returns
- plugin about data
Definition at line 90 of file GraphFilePluginInterface.cpp.
GraphFilePluginInterface::Error GraphFilePluginInterface::error | ( | ) | const |
Returns last error.
If last operation was successful. Use
- See also
- hasError() to test if error exists.
- Returns
- last Error of the plugin.
Definition at line 71 of file GraphFilePluginInterface.cpp.
QString GraphFilePluginInterface::errorString | ( | ) | const |
- Returns
- last error as human readable text
Definition at line 77 of file GraphFilePluginInterface.cpp.
|
pure virtual |
File extensions that are common for this file type.
- Returns
- extension list
Implemented in RocsGraphFileFormatPlugin, TgfFileFormatPlugin, GmlFileFormatPlugin, TikzFileFormatPlugin, KmlFileFormatPlugin, and DotFileFormatPlugin.
|
protected |
Gives current file.
- Returns
- file that is last used for write/read
Definition at line 120 of file GraphFilePluginInterface.cpp.
|
virtual |
If.
- See also
- isGraphDocument() returns true, this method returns document created by last
- readFile() call.
- Returns
- the graph document
Definition at line 102 of file GraphFilePluginInterface.cpp.
bool GraphFilePluginInterface::hasError | ( | ) | const |
- Returns
- true if last read or write operation caused an error.
Definition at line 65 of file GraphFilePluginInterface.cpp.
|
virtual |
- Returns
- true if a valid graph document was read. Otherwise return false
Definition at line 96 of file GraphFilePluginInterface.cpp.
|
virtual |
Returns PluginType
to indicate whether the plugin only provides import, only export or both capabilities.
This method should be used to determine in which file dialogs the plugin shall be included.
- Returns
- is by default PluginType::ImportAndExport
Reimplemented in TikzFileFormatPlugin.
Definition at line 59 of file GraphFilePluginInterface.cpp.
|
pure virtual |
Open given file and imports it into internal format.
Implemented in RocsGraphFileFormatPlugin, TgfFileFormatPlugin, GmlFileFormatPlugin, TikzFileFormatPlugin, KmlFileFormatPlugin, and DotFileFormatPlugin.
|
protected |
Use this function to set or unset the current error of the plugin. To unset an error use Error::None.
- Parameters
-
error is type of error,
- See also
- GraphFilePluginInterface::Error
- Parameters
-
message is optional description for error
Definition at line 83 of file GraphFilePluginInterface.cpp.
void GraphFilePluginInterface::setFile | ( | const KUrl & | file | ) |
Set file that shall be used for nexte read or write operation.
- Parameters
-
file is KUrl pointing to local file
Definition at line 114 of file GraphFilePluginInterface.cpp.
|
protected |
Use this function for read plugins to set read graph document
- Parameters
-
document that contains the graph
Definition at line 108 of file GraphFilePluginInterface.cpp.
|
pure virtual |
Writes given graph document to formerly specified file.
- See also
- setFile().
- Parameters
-
graph is graph document to be serialized
Implemented in RocsGraphFileFormatPlugin, TgfFileFormatPlugin, GmlFileFormatPlugin, TikzFileFormatPlugin, KmlFileFormatPlugin, and DotFileFormatPlugin.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:16:18 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.