kgoldrunner
#include <kgreditor.h>
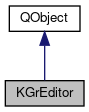
Public Slots | |
void | editNameAndHint () |
Signals | |
void | getMousePos (int &i, int &j) |
Public Member Functions | |
KGrEditor (KGrView *theView, const QString &theSystemDir, const QString &theUserDir, QList< KGrGameData * > &pGameList) | |
~KGrEditor () | |
bool | createLevel (int pGameIndex) |
bool | deleteLevelFile (int pGameIndex, int pLevel) |
bool | editGame (int pGameIndex) |
void | getGameAndLevel (int &game, int &lev) |
bool | moveLevelFile (int pGameIndex, int pLevel) |
bool | saveLevelFile () |
bool | saveOK () |
void | setEditObj (char newEditObj) |
bool | updateLevel (int pGameIndex, int pLevel) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
This class is the game-editor for KGoldrunner.
It loads KGrGameData and KGrLevelData objects from files, operates directly on the data in those objects and saves them back to files. In particular, the layout of a level is edited by selecting objects such as bricks, ladders, etc. from a toolbar and using mouse-clicks and drags to show where those objects are required. As this happens, the corresponding character-codes are stored directly in the QByteArray of layout-data and the corresponding visual objects (tiles) are displayed on the screen by the KGrScene and KGrView objects.
Game-editor class
Definition at line 42 of file kgreditor.h.
Constructor & Destructor Documentation
KGrEditor::KGrEditor | ( | KGrView * | theView, |
const QString & | theSystemDir, | ||
const QString & | theUserDir, | ||
QList< KGrGameData * > & | pGameList | ||
) |
The constructor of KGrEditor.
- Parameters
-
theView The canvas on which the editor paints the layout. Also the object that owns the editor and will destroy it if the KGoldrunner application is terminated. theSystemDir The directory-path where the games and levels released with KGoldrunner are stored. This data is read-only, but can be copied, edited and saved in the user's area. theUserDir The directory-path where the user's composed or edited games and levels are stored. pGameList The current list of system and user game-data. The user can add a game to the list and add levels to that game or any other game in the user's area.
Definition at line 32 of file kgreditor.cpp.
KGrEditor::~KGrEditor | ( | ) |
Definition at line 63 of file kgreditor.cpp.
Member Function Documentation
bool KGrEditor::createLevel | ( | int | pGameIndex | ) |
Set up a blank level-layout, ready for editing.
- Parameters
-
pGameIndex The list-index of the game which will contain the new level: assumed for now, but can change at save time.
- Returns
- If false, the action failed or was cancelled.
Definition at line 72 of file kgreditor.cpp.
bool KGrEditor::deleteLevelFile | ( | int | pGameIndex, |
int | pLevel | ||
) |
Delete a level from a game.
- Parameters
-
pGameIndex The list-index of the game that contains the level to be deleted: verified by a dialog and may change. pLevel The number of the level to be deleted: verified by a dialog and may change.
- Returns
- If false, the action failed or was cancelled.
Definition at line 425 of file kgreditor.cpp.
bool KGrEditor::editGame | ( | int | pGameIndex | ) |
Create a new game (a collection point for levels) or load the details of an existing game, ready for editing.
- Parameters
-
pGameIndex The list-index of the game to be created or edited: 0 = create, >0 = edit (verified by a dialog and may change).
- Returns
- If false, the action failed or was cancelled.
Definition at line 496 of file kgreditor.cpp.
|
slot |
Run a dialog in which the name and hint of a level can be edited.
Definition at line 200 of file kgreditor.cpp.
|
inline |
Definition at line 166 of file kgreditor.h.
|
signal |
Get the next grid-position at which to paint an object in the layout.
- Parameters
-
i The row-number of the cell (return by reference). j The column-number of the cell (return by reference).
bool KGrEditor::moveLevelFile | ( | int | pGameIndex, |
int | pLevel | ||
) |
Move a level to another game or level number.
Can be used to arrange levels in order of difficulty within a game.
- Parameters
-
pGameIndex The list-index of the game that contains the level to be moved: a dialog selects the game to move to. pLevel The number of the level to be moved: a dialog selects the number to move to. Other numbers may be changed, to preserve the sequential numbering of levels.
- Returns
- If false, the action failed or was cancelled.
Definition at line 326 of file kgreditor.cpp.
bool KGrEditor::saveLevelFile | ( | ) |
Save an edited level in a text file (*.grl) in the user's area.
The required game and level number are obtained from a dialog. These are the same as the original game and level number by default, but can be altered so as to get a Save As effect. For example, a system level can be loaded and edited, then saved in one of the user's own games.
- Returns
- If true, the level was successfully saved. If false, the user cancelled the save or the file I/O failed.
Definition at line 214 of file kgreditor.cpp.
bool KGrEditor::saveOK | ( | ) |
Check if there are any unsaved edits and, if so, ask the user what to do.
It will call saveLevelFile() if the user wants to save.
- Returns
- If true, the level was successfully saved or there was nothing to save or the user decided not to save, so it is OK to do something new or close KGoldrunner. If false, the user decided to continue editing or the file I/O failed.
Definition at line 646 of file kgreditor.cpp.
void KGrEditor::setEditObj | ( | char | newEditObj | ) |
Set the next object for the editor to paint, e.g.
brick, enemy, ladder.
- Parameters
-
newEditObj A character-code for the type of object.
Definition at line 67 of file kgreditor.cpp.
bool KGrEditor::updateLevel | ( | int | pGameIndex, |
int | pLevel | ||
) |
Load and display an existing level, ready for editing.
This can be a released level, but the changes must be saved in the user's area.
- Parameters
-
pGameIndex The list-index of the game that contains the level to be edited: verified by a dialog and may change. pLevel The number of the level to be edited: verified by a dialog and may change.
- Returns
- If false, the action failed or was cancelled.
Definition at line 120 of file kgreditor.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:18:24 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.