kgoldrunner
#include <kgrscene.h>
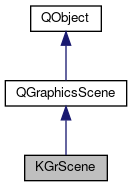
Public Slots | |
void | animate (bool missed) |
void | deleteAllSprites () |
void | deleteSprite (const int id) |
void | getMousePos (int &i, int &j) |
void | gotGold (const int spriteId, const int i, const int j, const bool spriteHasGold, const bool lost=false) |
int | makeSprite (const char type, int i, int j) |
void | paintCell (const int i, const int j, const char type) |
void | preRenderSprites () |
void | setMousePos (const int i, const int j) |
void | showHiddenLadders (const QList< int > &ladders, const int width) |
void | showLives (long lives) |
void | showScore (long score) |
void | startAnimation (const int id, const bool repeating, const int i, const int j, const int time, const Direction dirn, const AnimationType type) |
Signals | |
void | fadeFinished () |
void | redrawEditToolbar () |
Public Member Functions | |
KGrScene (KGrView *view) | |
~KGrScene () | |
void | changeSize () |
void | changeTheme () |
void | fadeIn (bool inOut) |
void | goToBlack () |
KGrRenderer * | renderer () const |
void | setGoldEnemiesRule (bool showIt) |
void | setHasHintText (const QString &msg) |
void | setLevel (unsigned int level) |
void | setPauseResumeText (const QString &msg) |
void | setReplayMessage (const QString &msg) |
void | setTitle (const QString &newTitle) |
void | showReplayMessage (bool onOff) |
QSize | tileSize () const |
![]() | |
QGraphicsScene (QObject *parent) | |
QGraphicsScene (const QRectF &sceneRect, QObject *parent) | |
QGraphicsScene (qreal x, qreal y, qreal width, qreal height, QObject *parent) | |
virtual | ~QGraphicsScene () |
QGraphicsItem * | activePanel () const |
QGraphicsWidget * | activeWindow () const |
QGraphicsEllipseItem * | addEllipse (const QRectF &rect, const QPen &pen, const QBrush &brush) |
QGraphicsEllipseItem * | addEllipse (qreal x, qreal y, qreal w, qreal h, const QPen &pen, const QBrush &brush) |
void | addItem (QGraphicsItem *item) |
QGraphicsLineItem * | addLine (const QLineF &line, const QPen &pen) |
QGraphicsLineItem * | addLine (qreal x1, qreal y1, qreal x2, qreal y2, const QPen &pen) |
QGraphicsPathItem * | addPath (const QPainterPath &path, const QPen &pen, const QBrush &brush) |
QGraphicsPixmapItem * | addPixmap (const QPixmap &pixmap) |
QGraphicsPolygonItem * | addPolygon (const QPolygonF &polygon, const QPen &pen, const QBrush &brush) |
QGraphicsRectItem * | addRect (const QRectF &rect, const QPen &pen, const QBrush &brush) |
QGraphicsRectItem * | addRect (qreal x, qreal y, qreal w, qreal h, const QPen &pen, const QBrush &brush) |
QGraphicsSimpleTextItem * | addSimpleText (const QString &text, const QFont &font) |
QGraphicsTextItem * | addText (const QString &text, const QFont &font) |
QGraphicsProxyWidget * | addWidget (QWidget *widget, QFlags< Qt::WindowType > wFlags) |
void | advance () |
QBrush | backgroundBrush () const |
int | bspTreeDepth () const |
void | changed (const QList< QRectF > ®ion) |
void | clear () |
void | clearFocus () |
void | clearSelection () |
QList< QGraphicsItem * > | collidingItems (const QGraphicsItem *item, Qt::ItemSelectionMode mode) const |
QGraphicsItemGroup * | createItemGroup (const QList< QGraphicsItem * > &items) |
void | destroyItemGroup (QGraphicsItemGroup *group) |
QGraphicsItem * | focusItem () const |
QFont | font () const |
QBrush | foregroundBrush () const |
bool | hasFocus () const |
qreal | height () const |
virtual QVariant | inputMethodQuery (Qt::InputMethodQuery query) const |
void | invalidate (qreal x, qreal y, qreal w, qreal h, QFlags< QGraphicsScene::SceneLayer > layers) |
void | invalidate (const QRectF &rect, QFlags< QGraphicsScene::SceneLayer > layers) |
bool | isActive () const |
bool | isSortCacheEnabled () const |
QGraphicsItem * | itemAt (qreal x, qreal y) const |
QGraphicsItem * | itemAt (qreal x, qreal y, const QTransform &deviceTransform) const |
QGraphicsItem * | itemAt (const QPointF &position) const |
QGraphicsItem * | itemAt (const QPointF &position, const QTransform &deviceTransform) const |
ItemIndexMethod | itemIndexMethod () const |
QList< QGraphicsItem * > | items (qreal x, qreal y, qreal w, qreal h, Qt::ItemSelectionMode mode, Qt::SortOrder order, const QTransform &deviceTransform) const |
QList< QGraphicsItem * > | items () const |
QList< QGraphicsItem * > | items (Qt::SortOrder order) const |
QList< QGraphicsItem * > | items (const QPointF &pos, Qt::ItemSelectionMode mode, Qt::SortOrder order, const QTransform &deviceTransform) const |
QList< QGraphicsItem * > | items (const QPolygonF &polygon, Qt::ItemSelectionMode mode, Qt::SortOrder order, const QTransform &deviceTransform) const |
QList< QGraphicsItem * > | items (const QRectF &rect, Qt::ItemSelectionMode mode, Qt::SortOrder order, const QTransform &deviceTransform) const |
QList< QGraphicsItem * > | items (const QPainterPath &path, Qt::ItemSelectionMode mode, Qt::SortOrder order, const QTransform &deviceTransform) const |
QList< QGraphicsItem * > | items (const QPointF &pos) const |
QList< QGraphicsItem * > | items (const QRectF &rectangle, Qt::ItemSelectionMode mode) const |
QList< QGraphicsItem * > | items (const QPolygonF &polygon, Qt::ItemSelectionMode mode) const |
QList< QGraphicsItem * > | items (const QPainterPath &path, Qt::ItemSelectionMode mode) const |
QList< QGraphicsItem * > | items (qreal x, qreal y, qreal w, qreal h, Qt::ItemSelectionMode mode) const |
QRectF | itemsBoundingRect () const |
QGraphicsItem * | mouseGrabberItem () const |
QPalette | palette () const |
void | removeItem (QGraphicsItem *item) |
void | render (QPainter *painter, const QRectF &target, const QRectF &source, Qt::AspectRatioMode aspectRatioMode) |
QRectF | sceneRect () const |
void | sceneRectChanged (const QRectF &rect) |
QList< QGraphicsItem * > | selectedItems () const |
QPainterPath | selectionArea () const |
void | selectionChanged () |
bool | sendEvent (QGraphicsItem *item, QEvent *event) |
void | setActivePanel (QGraphicsItem *item) |
void | setActiveWindow (QGraphicsWidget *widget) |
void | setBackgroundBrush (const QBrush &brush) |
void | setBspTreeDepth (int depth) |
void | setFocus (Qt::FocusReason focusReason) |
void | setFocusItem (QGraphicsItem *item, Qt::FocusReason focusReason) |
void | setFont (const QFont &font) |
void | setForegroundBrush (const QBrush &brush) |
void | setItemIndexMethod (ItemIndexMethod method) |
void | setPalette (const QPalette &palette) |
void | setSceneRect (qreal x, qreal y, qreal w, qreal h) |
void | setSceneRect (const QRectF &rect) |
void | setSelectionArea (const QPainterPath &path, const QTransform &deviceTransform) |
void | setSelectionArea (const QPainterPath &path) |
void | setSelectionArea (const QPainterPath &path, Qt::ItemSelectionMode mode, const QTransform &deviceTransform) |
void | setSelectionArea (const QPainterPath &path, Qt::ItemSelectionMode mode) |
void | setSortCacheEnabled (bool enabled) |
void | setStickyFocus (bool enabled) |
void | setStyle (QStyle *style) |
bool | stickyFocus () const |
QStyle * | style () const |
void | update (const QRectF &rect) |
void | update (qreal x, qreal y, qreal w, qreal h) |
QList< QGraphicsView * > | views () const |
qreal | width () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
typedef | SceneLayers |
![]() | |
virtual void | contextMenuEvent (QGraphicsSceneContextMenuEvent *contextMenuEvent) |
virtual void | dragEnterEvent (QGraphicsSceneDragDropEvent *event) |
virtual void | dragLeaveEvent (QGraphicsSceneDragDropEvent *event) |
virtual void | dragMoveEvent (QGraphicsSceneDragDropEvent *event) |
virtual void | drawBackground (QPainter *painter, const QRectF &rect) |
virtual void | drawForeground (QPainter *painter, const QRectF &rect) |
virtual void | drawItems (QPainter *painter, int numItems, QGraphicsItem *[] items, const QStyleOptionGraphicsItem[] options, QWidget *widget) |
virtual void | dropEvent (QGraphicsSceneDragDropEvent *event) |
virtual bool | event (QEvent *event) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
virtual void | focusInEvent (QFocusEvent *focusEvent) |
bool | focusNextPrevChild (bool next) |
virtual void | focusOutEvent (QFocusEvent *focusEvent) |
virtual void | helpEvent (QGraphicsSceneHelpEvent *helpEvent) |
virtual void | inputMethodEvent (QInputMethodEvent *event) |
virtual void | keyPressEvent (QKeyEvent *keyEvent) |
virtual void | keyReleaseEvent (QKeyEvent *keyEvent) |
virtual void | mouseDoubleClickEvent (QGraphicsSceneMouseEvent *mouseEvent) |
virtual void | mouseMoveEvent (QGraphicsSceneMouseEvent *mouseEvent) |
virtual void | mousePressEvent (QGraphicsSceneMouseEvent *mouseEvent) |
virtual void | mouseReleaseEvent (QGraphicsSceneMouseEvent *mouseEvent) |
virtual void | wheelEvent (QGraphicsSceneWheelEvent *wheelEvent) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
backgroundBrush | |
bspTreeDepth | |
font | |
foregroundBrush | |
itemIndexMethod | |
palette | |
sceneRect | |
sortCacheEnabled | |
stickyFocus | |
![]() | |
objectName | |
Detailed Description
The QGraphicsScene that represents KGoldrunner on the screen.
In the KGoldrunner scene, the KGoldrunner level-layouts use tile-coordinates that run from (1, 1) to (28, 20). To simplify programming, these are exactly the same as the cell co-ordinates used in the game-engine (or model).
The central grid has internal coordinates running from (-1, -1) to (30, 22), making 32x24 spaces. The empty space around the level-layout (2 cells wide all around) is designed to absorb over-enthusiastic mouse actions, which could otherwise cause accidents on other windows or the surrounding desktop.
Rows -1, 0, 29 and 30 usually contain titles and scores, which could have fractional co-ordinates. Row 0, row 21, column 0 and column 29 can also contain border-tiles (as in the Egyptian theme).
The hero and enemies (sprites) will have fractional co-ordinates as they move from one cell to another. Other graphics items (tiles) always have whole number co-ordinates (e.g. bricks, ladders and concrete). Empty spaces have no graphic item and the background shows through them.
The width and height of the view will rarely be an exact number of tiles, so there will be unused strips outside the 32x24 tile spaces. The sceneRect() is set larger than 24 tiles by 32 tiles to allow for this and its top left corner has negative fractional co-ordinates that are calculated but never actually used. Their purpose is to ensure that (1, 1) is always the top left corner of the KGoldrunner level layout, whatever the size/shape of the view.
Definition at line 75 of file kgrscene.h.
Constructor & Destructor Documentation
KGrScene::KGrScene | ( | KGrView * | view | ) |
Definition at line 37 of file kgrscene.cpp.
KGrScene::~KGrScene | ( | ) |
Definition at line 103 of file kgrscene.cpp.
Member Function Documentation
|
slot |
Definition at line 488 of file kgrscene.cpp.
void KGrScene::changeSize | ( | ) |
Redraw the scene whenever the view widget is resized.
Definition at line 221 of file kgrscene.cpp.
void KGrScene::changeTheme | ( | ) |
Redraw the scene whenever the current theme has changed.
Definition at line 215 of file kgrscene.cpp.
|
slot |
Definition at line 589 of file kgrscene.cpp.
|
slot |
Definition at line 575 of file kgrscene.cpp.
|
signal |
void KGrScene::fadeIn | ( | bool | inOut | ) |
Definition at line 320 of file kgrscene.cpp.
|
slot |
Definition at line 620 of file kgrscene.cpp.
|
slot |
Definition at line 551 of file kgrscene.cpp.
void KGrScene::goToBlack | ( | ) |
Definition at line 315 of file kgrscene.cpp.
|
slot |
Definition at line 442 of file kgrscene.cpp.
|
slot |
Requests the view to display a particular type of tile at a particular cell, or make it empty and show the background (tileType = FREE).
Used when loading level-layouts and also to make gold disappear/appear, hidden ladders appear or cells to be painted by the game editor. If there was something in the cell already, tileType = FREE acts as an erase function.
- Parameters
-
i The column-number of the cell to paint. i The row-number of the cell to paint. tileType The type of tile to paint (gold, brick, ladder, etc).
Definition at line 430 of file kgrscene.cpp.
|
slot |
Just as the game starts, ensure that all frames of the "hero" and "enemy" sprites have been rendered.
This is to avoid hiccups in animation in the first few seconds of play or demo.
Definition at line 595 of file kgrscene.cpp.
|
signal |
|
inline |
Get a pointer to the scene's renderer.
Definition at line 126 of file kgrscene.h.
|
inline |
Definition at line 128 of file kgrscene.h.
void KGrScene::setHasHintText | ( | const QString & | msg | ) |
Definition at line 303 of file kgrscene.cpp.
void KGrScene::setLevel | ( | unsigned int | level | ) |
Set the current level number.
It is used to select a background.
- Parameters
-
level The current level number.
Definition at line 346 of file kgrscene.cpp.
|
slot |
Definition at line 613 of file kgrscene.cpp.
void KGrScene::setPauseResumeText | ( | const QString & | msg | ) |
Definition at line 309 of file kgrscene.cpp.
void KGrScene::setReplayMessage | ( | const QString & | msg | ) |
Definition at line 237 of file kgrscene.cpp.
void KGrScene::setTitle | ( | const QString & | newTitle | ) |
Set the text for the title of the current level.
- Parameters
-
newTitle The title of the current level.
Definition at line 227 of file kgrscene.cpp.
|
slot |
Definition at line 566 of file kgrscene.cpp.
|
slot |
Definition at line 289 of file kgrscene.cpp.
void KGrScene::showReplayMessage | ( | bool | onOff | ) |
Definition at line 242 of file kgrscene.cpp.
|
slot |
Definition at line 296 of file kgrscene.cpp.
|
slot |
Requests the view to display an animation of a runner or dug brick at a particular cell, cancelling and superseding any current animation.
- Parameters
-
spriteId The ID of the sprite (dug brick). repeating If true, repeat the animation (false for dug brick). i The column-number of the cell. j The row-number of the cell. time The time in which to traverse one cell. dirn The direction of motion (always STAND for dug brick). type The type of animation (run, climb, open/close brick).
Definition at line 497 of file kgrscene.cpp.
|
inline |
Get the current size of the squared region occupied by a single visual element (characters, ladders, bricks etc.).
Definition at line 121 of file kgrscene.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:18:24 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.