kgoldrunner
#include <kgrlevelplayer.h>
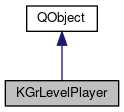
Signals | |
void | animation (bool missed) |
void | deleteSprite (const int spriteId) |
void | endLevel (const int result) |
void | getMousePos (int &i, int &j) |
void | gotGold (const int spriteId, const int i, const int j, const bool hasGold, const bool lost) |
void | interruptDemo () |
int | makeSprite (char spriteType, int i, int j) |
void | paintCell (int i, int j, char tileType) |
void | setMousePos (const int i, const int j) |
void | startAnimation (const int spriteId, const bool repeating, const int i, const int j, const int time, const Direction dirn, const AnimationType type) |
Public Member Functions | |
KGrLevelPlayer (QObject *parent, KRandomSequence *pRandomGen) | |
~KGrLevelPlayer () | |
bool | bumpingFriend (const int spriteId, const Direction dirn, const int gridI, const int gridJ) |
void | dbgControl (int code) |
void | enemyReappear (int &gridI, int &gridJ) |
Direction | getDirection (int heroI, int heroJ) |
Direction | getEnemyDirection (int enemyI, int enemyJ, bool leftRightSearch) |
bool | heroCaught (const int heroX, const int heroY) |
void | init (KGrView *view, KGrRecording *pRecording, const bool pPlayback, const bool gameFrozen) |
void | interruptPlayback () |
void | killHero () |
void | pause (bool stop) |
void | prepareToPlay () |
uchar | randomByte (const uchar limit) |
int | runnerGotGold (const int spriteId, const int i, const int j, const bool hasGold, const bool lost=false) |
void | setControlMode (const int mode) |
void | setDirectionByKey (const Direction dirn, const bool pressed) |
void | setHoldKeyOption (const int option) |
void | setTarget (int pointerI, int pointerJ) |
void | setTimeScale (const int timeScale) |
KGrEnemy * | standOnEnemy (const int spriteId, const int x, const int y) |
void | unstackEnemy (const int spriteId, const int gridI, const int gridJ, const int prevEnemy) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Class to play, record and play back a level of a game.
This class constructs and plays a single level of a KGoldrunner game. A KGrLevelPlayer object is created as each level begins and is destroyed as the level finishes, whether the human player wins the level or loses it. Each level is either recorded as it is played or played back from an earlier recording by emulating the same inputs as were used previously.
The KGrLevelPlayer object in turn creates all the objects needed to play the level, such as a hero, enemies, a play-area grid and a rule-book, which are all derived from data in a KGrLevelData structure. After the level and all its objects are set up, most of the work is done by the private slot tick(), including signals to update the graphics animation. Tick() is activated periodically by time-signals from the KGrTimer object, which also handles the standard Pause action and variations in the overall speed of KGoldrunner, from Beginner to Champion. KGrLevelPlayer is controlled by inputs from the mouse, keyboard or touchpad, which in turn control the movement of the hero and the digging of bricks. The enemies are controlled by algorithms in the polymorphic KGrRuleBook object. Each set of rules has its own distinct algorithm. In playback mode, the inputs are emulated.
KGrLevelPlayer and friends are the internal model and game-engine of KGoldrunner and they communicate with the view, KGrCanvas and friends, solely via signals that indicate what is moving and what has to be painted.
Definition at line 65 of file kgrlevelplayer.h.
Constructor & Destructor Documentation
KGrLevelPlayer::KGrLevelPlayer | ( | QObject * | parent, |
KRandomSequence * | pRandomGen | ||
) |
The constructor of KGrLevelPlayer.
- Parameters
-
parent The object that owns the level-player and will destroy it if the KGoldrunner application is terminated during play. pRandomGen A shared source of random numbers for all enemies.
Definition at line 38 of file kgrlevelplayer.cpp.
KGrLevelPlayer::~KGrLevelPlayer | ( | ) |
Definition at line 70 of file kgrlevelplayer.cpp.
Member Function Documentation
|
signal |
Requests the view to update animated sprites.
Each sprite moves as decided by the parameters of its last startAnimation() signal.
- Parameters
-
missed If true, moves and frame changes occur normally, but they are not displayed on the screen. This is to allow the graphics to catch up if Qt has missed one or more time signals.
bool KGrLevelPlayer::bumpingFriend | ( | const int | spriteId, |
const Direction | dirn, | ||
const int | gridI, | ||
const int | gridJ | ||
) |
Helper function to determine whether an enemy is colliding with another enemy.
This to prevent enemies occupying the same cell, depending on what the rules for this level allow.
- Parameters
-
spriteId The identifier of the enemy. dirn The direction in which the enemy wants to go. gridI The column-position of the enemy. gridJ The row-position of the enemy.
- Returns
- True if the enemy is too close to another enemy.
Definition at line 672 of file kgrlevelplayer.cpp.
void KGrLevelPlayer::dbgControl | ( | int | code | ) |
Implement author's debugging aids, which are activated only if the level is paused and the KConfig file contains group Debugging with setting DebuggingShortcuts=true.
The main actions are to do timer steps one at a time, activate/deactivate a bug-fix or new-feature patch dynamically, activate/deactivate logging output from fprintf or kDebug() dynamically, print the status of a cell pointed to by the mouse and print the status of the hero or an enemy. See the code in file kgoldrunner.cpp, at the end of KGoldrunner::setupActions() for details of codes and keystrokes.
To use the BUG_FIX or LOGGING options, first patch in and compile some code to achieve the effect required, with tests of static bool flags KGrGame::bugFix or KGrGame::logging surrounding that code. The relevant keystrokes then toggle those flags, so as to execute or skip the code dynamically as the game runs.
- Parameters
-
code A code to indicate the action required (see enum DebugCodes in file kgrglobals.h).
Definition at line 1198 of file kgrlevelplayer.cpp.
|
signal |
|
signal |
void KGrLevelPlayer::enemyReappear | ( | int & | gridI, |
int & | gridJ | ||
) |
Helper function to determine where an enemy should reappear after being trapped in a brick.
This applies with Traditional and Scavenger rules only. The procedure chooses a random place in row 2 or row 1.
- Parameters
-
gridI A randomly-chosen column (return by reference). gridJ Row 2 Traditional or 1 Scavenger (return by reference).
Definition at line 880 of file kgrlevelplayer.cpp.
Direction KGrLevelPlayer::getDirection | ( | int | heroI, |
int | heroJ | ||
) |
Helper function for the hero to find his next direction when using mouse or touchpad control.
Uses the point from setTarget() as a guide.
- Parameters
-
heroI The column-number where the hero is now (>=1). heroJ The row-number where the hero is now (>=1).
- Returns
- The required direction (values defined by enum Direction in file kgrglobals.h).
Definition at line 480 of file kgrlevelplayer.cpp.
Direction KGrLevelPlayer::getEnemyDirection | ( | int | enemyI, |
int | enemyJ, | ||
bool | leftRightSearch | ||
) |
Helper function for an enemy to find his next direction, based on where the hero is and the search algorithm implemented in the level's rules.
- Parameters
-
enemyI The column-number where the enemy is now (>=1). enemyJ The row-number where the enemy is now (>=1). leftRightSearch The search-direction (for KGoldrunner rules only).
- Returns
- The required direction (values defined by enum Direction in file kgrglobals.h).
Definition at line 621 of file kgrlevelplayer.cpp.
|
signal |
|
signal |
bool KGrLevelPlayer::heroCaught | ( | const int | heroX, |
const int | heroY | ||
) |
Helper function to determine whether the hero has collided with an enemy and must lose a life (unless he is standing on the enemy's head).
- Parameters
-
heroX The X grid-position of the hero (within a cell). heroY The Y grid-position of the hero (within a cell).
- Returns
- True if the hero is touching an enemy.
Definition at line 632 of file kgrlevelplayer.cpp.
void KGrLevelPlayer::init | ( | KGrView * | view, |
KGrRecording * | pRecording, | ||
const bool | pPlayback, | ||
const bool | gameFrozen | ||
) |
The main initialisation of KGrLevelPlayer.
This method establishes the playing rules to be used, creates the internal playing-grid, creates the hero and enemies and connects the various signals and slots together. It also initialises the recording or playback of moves made by the hero and enemies. Note that this is the only place where KGrLevelPlayer uses the view directly. All other references are via signals and slots.
- Parameters
-
view Points to the KGrCanvas object that provides graphics. mode The input-mode used to control the hero: mouse, keyboard or hybrid touchpad and keyboard mode (for laptops). keyOption The option to use when in keyboard mode (i.e. CLICK_KEY or HOLD_KEY). pRecording Points to a data-object that contains all the data for the level, including the layout of the maze and the starting positions of hero, enemies and gold, plus the variant of the rules to be followed: Traditional, KGoldrunner or Scavenger. The level is either recorded as it is played or re-played from an earlier recording. If playing "live", the pRecording object has empty buffers into which the level player will store moves. If re-playing, the buffers contain hero's moves to be played back and random numbers for the enemies to re-use, so that all play can be faithfully reproduced, even if the random-number generator's code changes. pPlayback If false, play "live" and record the play. If true, play back a previously recorded level. gameFrozen If true, go into pause-mode when the level starts.
Definition at line 100 of file kgrlevelplayer.cpp.
|
signal |
void KGrLevelPlayer::interruptPlayback | ( | ) |
Stop playback of a recorded level and adjust the content of the recording so that the user can continue playing and recording from that point, if required, as in the Instant Replay or Replay Last Level actions.
Definition at line 1065 of file kgrlevelplayer.cpp.
void KGrLevelPlayer::killHero | ( | ) |
If not in playback mode, add a code to the recording and kill the hero.
Definition at line 1154 of file kgrlevelplayer.cpp.
|
signal |
|
signal |
Requests the view to display a particular type of tile at a particular cell, or make it empty and show the background (tileType = FREE).
Used when loading level-layouts.
- Parameters
-
i The column-number of the cell to paint. i The row-number of the cell to paint. tileType The type of tile to paint (gold, brick, ladder, etc).
void KGrLevelPlayer::pause | ( | bool | stop | ) |
Pause or resume the gameplay in this level.
- Parameters
-
stop If true, pause: if false, resume.
Definition at line 368 of file kgrlevelplayer.cpp.
void KGrLevelPlayer::prepareToPlay | ( | ) |
Indicate that setup is complete and the human player can start playing at any time, by moving the pointer device or pressing a key.
Definition at line 360 of file kgrlevelplayer.cpp.
uchar KGrLevelPlayer::randomByte | ( | const uchar | limit | ) |
Helper function to provide enemies with random numbers for reappearing and deciding whether to pick up or drop gold.
The random bytes generated are stored during recording and re-used during playback, so that play is completely reproducible, even if the library's random number generator behavior should vary across platforms or in future versions.
- Parameters
-
limit The upper limit for the number to be returned.
- Returns
- The random number, value >= 0 and < limit.
Definition at line 925 of file kgrlevelplayer.cpp.
int KGrLevelPlayer::runnerGotGold | ( | const int | spriteId, |
const int | i, | ||
const int | j, | ||
const bool | hasGold, | ||
const bool | lost = false |
||
) |
Helper function for an enemy to pick up or drop gold or the hero to collect gold.
Records the presence or absence of the gold on the internal grid and on the screen. Also pops up the hidden ladders (if any) when there is no gold left.
- Parameters
-
spriteId The identifier of the hero or enemy. i The column-number where the gold is (>=1). j The row-number where the gold is (>=1). hasGold True if gold was picked up: false if it was dropped.
- Returns
- The number of pieces of gold remaining in this level.
Definition at line 820 of file kgrlevelplayer.cpp.
void KGrLevelPlayer::setControlMode | ( | const int | mode | ) |
Change the input-mode during play.
- Parameters
-
mode The new input-mode to use to control the hero: mouse, keyboard or hybrid touchpad and keyboard mode.
Definition at line 1165 of file kgrlevelplayer.cpp.
void KGrLevelPlayer::setDirectionByKey | ( | const Direction | dirn, |
const bool | pressed | ||
) |
Set a direction for the hero to move or dig when using keyboard control.
- Parameters
-
dirn The required direction (values defined by enum Direction in file kgrglobals.h). pressed Tells whether the direction-key was pressed or released.
Definition at line 440 of file kgrlevelplayer.cpp.
void KGrLevelPlayer::setHoldKeyOption | ( | const int | option | ) |
Change the keyboard click/hold option during play.
- Parameters
-
option The new option for keyboard operation: either CLICK_KEY to start running non-stop when a direction key is clicked or HOLD_KEY to start when a key is pressed and stop when it is released, with simultaneous key-holding allowed.
Definition at line 1175 of file kgrlevelplayer.cpp.
|
signal |
void KGrLevelPlayer::setTarget | ( | int | pointerI, |
int | pointerJ | ||
) |
Set a point for the hero to aim at when using mouse or touchpad control.
- Parameters
-
pointerI The required column-number on the playing-grid (>=1). pointerJ The required row-number on the playing-grid (>=1).
Definition at line 378 of file kgrlevelplayer.cpp.
void KGrLevelPlayer::setTimeScale | ( | const int | timeScale | ) |
Set the overall speed of gameplay.
- Parameters
-
timeScale Value 10 is for normal speed. Range is 2 to 20. 5 is for beginner speed: 15 for champion speed.
Definition at line 1185 of file kgrlevelplayer.cpp.
KGrEnemy * KGrLevelPlayer::standOnEnemy | ( | const int | spriteId, |
const int | x, | ||
const int | y | ||
) |
Helper function to determine whether the hero or an enemy is standing on an enemy's head.
- Parameters
-
spriteId The identifier of the hero or enemy. x The X grid-position of the sprite (within a cell). y The Y grid-position of the sprite (within a cell).
- Returns
- Pointer to the enemy the sprite is standing on - or 0.
Definition at line 652 of file kgrlevelplayer.cpp.
|
signal |
Requests the view to display an animation of a dug brick at a particular cell, cancelling and superseding any current animation.
- Parameters
-
spriteId The ID of the sprite (dug brick). repeating If true, repeat the animation (false for dug brick). i The column-number of the cell to dig. j The row-number of the cell to dig. time The time in which to traverse one cell. dirn The direction of motion, always STAND. type The type of animation (open or close the brick).
void KGrLevelPlayer::unstackEnemy | ( | const int | spriteId, |
const int | gridI, | ||
const int | gridJ, | ||
const int | prevEnemy | ||
) |
Helper function to remove an enemy from among several stacked in a cell.
- Parameters
-
spriteId The identifier of the enemy. gridI The column-position of the enemy. gridJ The row-position of the enemy. prevEnemy The previously stacked enemy in the same cell (or -1).
Definition at line 728 of file kgrlevelplayer.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:18:24 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.