kgoldrunner
#include <kgrrunner.h>
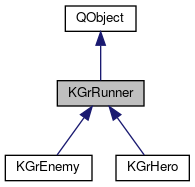
Signals | |
void | incScore (const int n) |
void | startAnimation (const int spriteId, const bool repeating, const int i, const int j, const int time, const Direction dirn, const AnimationType type) |
Public Member Functions | |
KGrRunner (KGrLevelPlayer *pLevelPlayer, KGrLevelGrid *pGrid, int i, int j, const int pSpriteId, KGrRuleBook *pRules, const int startDelay) | |
virtual | ~KGrRunner () |
int | whereAreYou (int &x, int &y) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Member Functions | |
void | getRules () |
char | nextCell () |
bool | setNextMovement (const char spriteType, const char cellType, Direction &dir, AnimationType &anim, int &interval) |
Situation | situation (const int scaledTime) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
AnimationType | currAnimation |
Direction | currDirection |
int | deltaX |
int | deltaY |
int | enemyFallTime |
bool | falling |
int | fallTime |
KGrLevelGrid * | grid |
int | gridI |
int | gridJ |
int | gridX |
int | gridY |
int | interval |
bool | leftRightSearch |
KGrLevelPlayer * | levelPlayer |
int | pointCtr |
int | pointsPerCell |
KGrRuleBook * | rules |
int | runTime |
int | spriteId |
QTime | t |
int | timeLeft |
int | trapTime |
bool | turnAnywhere |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
This class provides the shared features of all runners (hero and enemies).
Definition at line 35 of file kgrrunner.h.
Constructor & Destructor Documentation
KGrRunner::KGrRunner | ( | KGrLevelPlayer * | pLevelPlayer, |
KGrLevelGrid * | pGrid, | ||
int | i, | ||
int | j, | ||
const int | pSpriteId, | ||
KGrRuleBook * | pRules, | ||
const int | startDelay | ||
) |
The constructor of the KGrRunner virtual class.
- Parameters
-
pLevelPlayer The object that owns the runner and will destroy it if the KGoldrunner application is terminated during play. The object also provides helper functions to the runners. pGrid The grid on which the runner is playing. i The starting column-number (>=1). j The starting row-number (>=1). pSpriteId The sprite ID of the runner, as used in animation. pRules The rules that apply to this game and level. startDelay The starting-time advantage enemies give to the hero.
Definition at line 27 of file kgrrunner.cpp.
|
virtual |
Definition at line 68 of file kgrrunner.cpp.
Member Function Documentation
|
protected |
Definition at line 72 of file kgrrunner.cpp.
|
signal |
Requests the KGoldrunner game to add to the human player's score.
- Parameters
-
n The amount to add to the score.
|
protected |
Definition at line 113 of file kgrrunner.cpp.
|
protected |
Definition at line 121 of file kgrrunner.cpp.
|
protected |
Definition at line 82 of file kgrrunner.cpp.
|
signal |
Requests the view-object to display an animation of a runner at a particular cell, cancelling and superseding any current animation.
- Parameters
-
spriteId The ID of the sprite (hero or enemy). repeating If true, repeat the animation until the next signal. i The column-number of the cell to start at. j The row-number of the cell to start at. time The time in which to traverse one cell. dirn The direction of motion, or STAND. type The type of animation (walk, climb. etc.).
|
inline |
Returns the exact position of a runner (in grid-points or cell sub-divisions) and the number of grid-points per cell, from which the cell's column-number and row-number can be calculated if required.
- Parameters
-
x X-coordinate in grid-points (return by reference). y Y-coordinate in grid-points (return by reference).
- Returns
- The number of grid-points per cell.
Definition at line 68 of file kgrrunner.h.
Member Data Documentation
|
protected |
Definition at line 124 of file kgrrunner.h.
|
protected |
Definition at line 123 of file kgrrunner.h.
|
protected |
Definition at line 106 of file kgrrunner.h.
|
protected |
Definition at line 107 of file kgrrunner.h.
|
protected |
Definition at line 128 of file kgrrunner.h.
|
protected |
Definition at line 122 of file kgrrunner.h.
|
protected |
Definition at line 127 of file kgrrunner.h.
|
protected |
Definition at line 97 of file kgrrunner.h.
|
protected |
Definition at line 102 of file kgrrunner.h.
|
protected |
Definition at line 103 of file kgrrunner.h.
|
protected |
Definition at line 104 of file kgrrunner.h.
|
protected |
Definition at line 105 of file kgrrunner.h.
|
protected |
Definition at line 132 of file kgrrunner.h.
|
protected |
Definition at line 135 of file kgrrunner.h.
|
protected |
Definition at line 96 of file kgrrunner.h.
|
protected |
Definition at line 109 of file kgrrunner.h.
|
protected |
Definition at line 110 of file kgrrunner.h.
|
protected |
Definition at line 98 of file kgrrunner.h.
|
protected |
Definition at line 126 of file kgrrunner.h.
|
protected |
Definition at line 100 of file kgrrunner.h.
|
protected |
Definition at line 137 of file kgrrunner.h.
|
protected |
Definition at line 133 of file kgrrunner.h.
|
protected |
Definition at line 129 of file kgrrunner.h.
|
protected |
Definition at line 111 of file kgrrunner.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:18:24 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.