libkdegames/libkdegamesprivate/kgame
#include <KGame/KMessageIO>
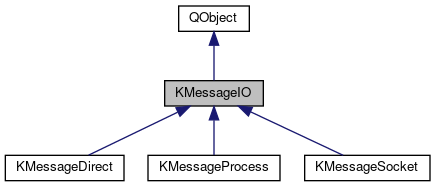
Public Slots | |
virtual void | send (const QByteArray &msg)=0 |
Signals | |
void | connectionBroken () |
void | received (const QByteArray &msg) |
Public Member Functions | |
KMessageIO (QObject *parent=0) | |
~KMessageIO () | |
quint32 | id () |
virtual bool | isConnected () const |
virtual bool | isNetwork () const |
virtual QString | peerName () const |
virtual quint16 | peerPort () const |
virtual int | rtti () const |
void | setId (quint32 id) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Attributes | |
quint32 | m_id |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
This abstract base class represents one end of a message connections between two clients.
Each client has one object of a subclass of KMessageIO. Calling /e send() on one object will emit the signal /e received() on the other object, and vice versa.
For each type of connection (TCP/IP socket, COM port, direct connection within the same class) a subclass of KMessageIO must be defined that implements the pure virtual methods /e send() and /e isConnected(), and sends the signals. (See /e KMessageSocket for an example implementation.)
Two subclasses are already included: /e KMessageSocket (connection using TCP/IP sockets) and /e KMessageDirect (connection using method calls, both sides must be within the same process).
Definition at line 57 of file kmessageio.h.
Constructor & Destructor Documentation
KMessageIO::KMessageIO | ( | QObject * | parent = 0 | ) |
The usual QObject constructor, does nothing else.
Definition at line 32 of file kmessageio.cpp.
KMessageIO::~KMessageIO | ( | ) |
The usual destructor, does nothing special.
Definition at line 36 of file kmessageio.cpp.
Member Function Documentation
|
signal |
This signal is emitted when the connection is closed.
This can be caused by a hardware error (e.g. broken internet connection) or because the other object was killed.
Note: Sometimes a broken connection can be undetected for a long time, or may never be detected at all. So don't rely on this signal!
quint32 KMessageIO::id | ( | ) |
Queries the ID of this object.
Definition at line 44 of file kmessageio.cpp.
|
inlinevirtual |
This method returns the status of the object, whether it is already (or still) connected to another KMessageIO object or not.
This is a pure virtual method, so it has to be implemented in a subclass of KMessageIO.
Reimplemented in KMessageProcess, KMessageDirect, and KMessageSocket.
Definition at line 95 of file kmessageio.h.
|
inlinevirtual |
- Returns
- Whether this KMessageIO is a network IO or not.
Reimplemented in KMessageProcess, KMessageDirect, and KMessageSocket.
Definition at line 81 of file kmessageio.h.
|
inlinevirtual |
- Returns
- "localhost" in the default implementation. Reimplemented in KMessageSocket
Reimplemented in KMessageSocket.
Definition at line 124 of file kmessageio.h.
|
inlinevirtual |
- Returns
- 0 in the default implementation. Reimplemented in KMessageSocket.
Reimplemented in KMessageSocket.
Definition at line 119 of file kmessageio.h.
|
signal |
This signal is emitted when /e send() on the connected KMessageIO object is called.
The parameter contains the same data array in /e msg as was used in /e send().
|
inlinevirtual |
The runtime idendifcation.
Reimplemented in KMessageProcess, KMessageDirect, and KMessageSocket.
Definition at line 75 of file kmessageio.h.
|
pure virtualslot |
This slot sends the data block in /e msg to the connected object, that will emit /e received().
For a concrete class, you have to subclass /e KMessageIO and overwrite this method. In the subclass, implement this method as an ordinary method, not as a slot! (Otherwise another slot would be defined. It would work, but uses more memory and time.) See /e KMessageSocket for an example implementation.
Implemented in KMessageProcess, KMessageDirect, and KMessageSocket.
void KMessageIO::setId | ( | quint32 | id | ) |
Sets the ID number of this object.
This number can for example be used to distinguish several objects in a server.
NOTE: Sometimes it is useful to let two connected KMessageIO objects have the same ID number. You have to do so yourself, KMessageIO doesn't change this value on its own!
Definition at line 39 of file kmessageio.cpp.
Member Data Documentation
|
protected |
Definition at line 159 of file kmessageio.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:18:54 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.