KDE3Support
#include <k3spell.h>
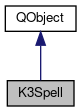
Public Types | |
enum | SpellerType { Text = 0, HTML, TeX, Nroff } |
enum | spellStatus { Starting = 0, Running, Cleaning, Finished, Error, Crashed, FinishedNoMisspellingsEncountered } |
Signals | |
void | addword (const QString &originalword) |
void | corrected (const QString &originalword, const QString &newword, unsigned int pos) |
void | death () |
void | dialog3 () |
void | done (const QString &buffer) |
void | done (bool) |
void | ignoreall (const QString &originalword) |
void | ignoreword (const QString &originalword) |
void | misspelling (const QString &originalword, const QStringList &suggestions, unsigned int pos) |
void | progress (unsigned int i) |
void | ready (K3Spell *) |
void | replaceall (const QString &origword, const QString &replacement) |
Public Member Functions | |
K3Spell (QWidget *parent, const QString &caption, QObject *receiver, const char *slot, K3SpellConfig *kcs=0, bool progressbar=true, bool modal=false) | |
K3Spell (QWidget *parent, const QString &caption, QObject *receiver, const char *slot, K3SpellConfig *kcs, bool progressbar, bool modal, SpellerType type) | |
virtual | ~K3Spell () |
virtual bool | addPersonal (const QString &word) |
virtual bool | check (const QString &_buffer, bool usedialog=true) |
virtual bool | checkList (QStringList *_wordlist, bool usedialog=true) |
virtual bool | checkWord (const QString &_buffer, bool usedialog=false) |
bool | checkWord (const QString &buffer, bool _usedialog, bool suggest) |
virtual void | cleanUp () |
int | dlgResult () const |
int | heightDlg () const |
void | hide () |
virtual bool | ignore (const QString &word) |
QString | intermediateBuffer () const |
K3SpellConfig | ksConfig () const |
int | lastPosition () const |
void | moveDlg (int x, int y) |
void | setAutoDelete (bool _autoDelete) |
void | setIgnoreTitleCase (bool b) |
void | setIgnoreUpperWords (bool b) |
void | setProgressResolution (unsigned int res) |
spellStatus | status () const |
QStringList | suggestions () const |
int | widthDlg () const |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static int | modalCheck (QString &text) |
static int | modalCheck (QString &text, K3SpellConfig *kcs) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Protected Slots | |
void | check2 () |
void | check3 () |
void | checkList2 () |
void | checkList3a () |
void | checkList4 () |
void | checkListReplaceCurrent () |
void | checkNext () |
void | checkWord2 () |
void | checkWord3 () |
void | dialog2 (int dlgresult) |
void | emitDeath () |
void | ispellErrors () |
void | ispellExit () |
void | K3Spell2 () |
void | slotStopCancel (int) |
void | suggestWord () |
Protected Member Functions | |
bool | cleanFputs (const QString &s) |
bool | cleanFputsWord (const QString &s) |
void | dialog (const QString &word, QStringList &sugg, const char *_slot) |
void | emitProgress () |
QString | funnyWord (const QString &word) |
void | initialize (QWidget *_parent, const QString &_caption, QObject *obj, const char *slot, K3SpellConfig *_ksc, bool _progressbar, bool _modal, SpellerType type) |
int | parseOneResponse (const QString &_buffer, QString &word, QStringList &sugg) |
QString | replacement () const |
void | setUpDialog (bool reallyusedialogbox=true) |
void | startIspell () |
bool | writePersonalDictionary () |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
bool | autoDelete |
QString | caption |
unsigned int | curprog |
QString | cwword |
QString | dialog3slot |
bool | dialogsetup |
bool | dialogwillprocess |
bool | dlgon |
QString | dlgorigword |
QString | dlgreplacement |
int | dlgresult |
QStringList | ignorelist |
K3SpellConfig * | ksconfig |
K3SpellDlg * | ksdlg |
unsigned int | lastlastline |
int | lastline |
int | lastpos |
spellStatus | m_status |
int | maxtrystart |
bool | modaldlg |
QString | newbuffer |
unsigned int | offset |
QString | orig |
QString | origbuffer |
QWidget * | parent |
bool | personaldict |
unsigned int | posinline |
KProcess * | proc |
unsigned int | progres |
bool | progressbar |
QStringList | replacelist |
QStringList | sugg |
bool | texmode |
unsigned int | totalpos |
int | trystart |
bool | usedialog |
QStringList::Iterator | wlIt |
QStringList * | wordlist |
Static Protected Attributes | |
static int | modalreturn = 0 |
static QString | modaltext |
static QWidget * | modalWidgetHack = 0 |
Additional Inherited Members | |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
KDE Spellchecker
A KDE programmer's interface to International ISpell 3.1, ASpell, HSpell and ZPSpell.. A static method, modalCheck() is provided for convenient access to the spellchecker.
- See also
- K3SpellConfig, KSyntaxHighlighter
- Deprecated:
- , use sonnet instead
Member Enumeration Documentation
enum K3Spell::SpellerType |
These are possible types of documents which the spell checker can check.
Text
- The default type, checks every wordHTML
- For HTML/SGML/XML documents, will skip the tags,TeX
- For TeX/LaTeX documents, will skip commands,Nroff
- For nroff/troff documents.
Please note that not every option is supported on every type of checker (e.g. ASpell doesn't support Nroff). In case a type of a document is not supported the default Text option will be used.
Enumerator | |
---|---|
Text | |
HTML | |
TeX | |
Nroff |
enum K3Spell::spellStatus |
Possible states of the spell checker.
Starting
- After creation of K3Spell.Running
- After the ready signal has been emitted.Cleaning
- After cleanUp() has been called.Finished
- After cleanUp() has been completed.
The following error states exist:
Error
- An error occurred in theStarting
state.Crashed
- An error occurred in theRunning
state.
Enumerator | |
---|---|
Starting | |
Running | |
Cleaning | |
Finished | |
Error | |
Crashed | |
FinishedNoMisspellingsEncountered |
Constructor & Destructor Documentation
K3Spell::K3Spell | ( | QWidget * | parent, |
const QString & | caption, | ||
QObject * | receiver, | ||
const char * | slot, | ||
K3SpellConfig * | kcs = 0 , |
||
bool | progressbar = true , |
||
bool | modal = false |
||
) |
Starts the spellchecker.
K3Spell emits ready() when it has verified that ISpell/ASpell is working properly. Pass the name of a slot – do not pass zero! Be sure to call cleanUp() when you are done with K3Spell.
If K3Spell could not be started correctly, death() is emitted.
- Parameters
-
parent Parent of K3SpellConfig dialog.. caption Caption of K3SpellConfig dialog. receiver Receiver object for the ready(K3Spell *) signal. slot Receiver's slot, will be connected to the ready(K3Spell *) signal. kcs Configuration for K3Spell. progressbar Indicates if progress bar should be shown. modal Indicates modal or non-modal dialog.
Definition at line 125 of file k3spell.cpp.
K3Spell::K3Spell | ( | QWidget * | parent, |
const QString & | caption, | ||
QObject * | receiver, | ||
const char * | slot, | ||
K3SpellConfig * | kcs, | ||
bool | progressbar, | ||
bool | modal, | ||
SpellerType | type | ||
) |
Starts the spellchecker.
K3Spell emits ready() when it has verified that ISpell/ASpell is working properly. Pass the name of a slot – do not pass zero! Be sure to call cleanUp() when you are done with K3Spell.
If K3Spell could not be started correctly, death() is emitted.
- Parameters
-
parent Parent of K3SpellConfig dialog.. caption Caption of K3SpellConfig dialog. receiver Receiver object for the ready(K3Spell *) signal. slot Receiver's slot, will be connected to the ready(K3Spell *) signal. kcs Configuration for K3Spell. progressbar Indicates if progress bar should be shown. modal Indicates modal or non-modal dialog. type Type of the document to check
Definition at line 133 of file k3spell.cpp.
|
virtual |
The destructor instructs ISpell/ASpell to write out the personal dictionary and then terminates ISpell/ASpell.
Definition at line 1404 of file k3spell.cpp.
Member Function Documentation
Adds a word to the user's personal dictionary.
- Returns
- false if
word
is not a word or there was an error communicating with ISpell/ASpell.
Definition at line 489 of file k3spell.cpp.
|
signal |
Emitted when the user pressed "Add" in the dialog.
This could be used to make an external user dictionary independent of the ISpell personal dictionary.
Spellchecks a buffer of many words in plain text format.
The _buffer
is not modified. The signal done() will be sent when check() is finished and the argument will be a spell-corrected version of _buffer
.
The spell check may be stopped by the user before the entire buffer has been checked. You can check lastPosition() to see how far in _buffer
check() reached before stopping.
Definition at line 1082 of file k3spell.cpp.
|
protectedslot |
Definition at line 1147 of file k3spell.cpp.
|
protectedslot |
Definition at line 1266 of file k3spell.cpp.
|
virtual |
Spellchecks a list of words.
checkList() is more flexible than check(). You could parse any type of document (HTML, TeX, etc.) into a list of spell-checkable words and send the list to checkList(). Sending a marked-up document to check() would result in the mark-up tags being spell checked.
Definition at line 886 of file k3spell.cpp.
|
protectedslot |
Definition at line 913 of file k3spell.cpp.
|
protectedslot |
Definition at line 944 of file k3spell.cpp.
|
protectedslot |
Definition at line 1033 of file k3spell.cpp.
|
protectedslot |
Definition at line 1018 of file k3spell.cpp.
|
protectedslot |
Definition at line 703 of file k3spell.cpp.
Spellchecks a single word.
checkWord() is the most flexible function. Some applications might need this flexibility but will sacrifice speed when checking large numbers of words. Consider checkList() for checking many words.
Use this method for implementing "online" spellchecking (i.e., spellcheck as-you-type).
checkWord() returns false
if buffer
is not a single word (e.g. if it contains white space), otherwise it returns true
;
If usedialog
is set to true
, K3Spell will open the standard dialog if the word is not found. The dialog results can be queried by using dlgResult() and replacement().
The signal corrected() is emitted when the check is complete. You can look at suggestions() to see what the suggested replacements were.
set the dialog signal handler
Definition at line 571 of file k3spell.cpp.
set the dialog signal handler
Definition at line 615 of file k3spell.cpp.
|
protectedslot |
Definition at line 661 of file k3spell.cpp.
|
protectedslot |
Definition at line 741 of file k3spell.cpp.
Definition at line 549 of file k3spell.cpp.
Definition at line 522 of file k3spell.cpp.
|
virtual |
Cleans up ISpell.
Write out the personal dictionary and close ISpell's stdin. A death() signal will be emitted when the cleanup is complete, but this method will return immediately.
Definition at line 1421 of file k3spell.cpp.
|
signal |
Emitted after the "Replace" or "Replace All" buttons of the dialog was pressed, or if the word was corrected without calling the dialog (i.e., the user previously chose "Replace All" for this word).
Results from the dialog may be checked with dlgResult() and replacement().
Note, that when using checkList() this signal can occur more than once with same list position, when checking a word with hyphens. In this case originalword
is the last replacement.
- See also
- check()
|
signal |
|
protected |
Definition at line 1322 of file k3spell.cpp.
|
protectedslot |
Definition at line 1356 of file k3spell.cpp.
|
signal |
int K3Spell::dlgResult | ( | ) | const |
Gets the result code of the dialog box.
After calling checkWord, you can use this to get the dialog box's result code. The possible values are (from kspelldlg.h):
- KS_CANCEL
- KS_REPLACE
- KS_REPLACEALL
- KS_IGNORE
- KS_IGNOREALL
- KS_ADD
- KS_STOP
Definition at line 153 of file k3spell.cpp.
|
signal |
|
signal |
Emitted when checkList() is done.
If the argument is true
, then you should update your text from the wordlist, otherwise not.
|
protectedslot |
Definition at line 1467 of file k3spell.cpp.
|
protected |
Definition at line 1480 of file k3spell.cpp.
Definition at line 748 of file k3spell.cpp.
int K3Spell::heightDlg | ( | ) | const |
Returns the height of the dialog box.
Definition at line 158 of file k3spell.cpp.
void K3Spell::hide | ( | ) |
Hides the dialog box.
You'll need to do this when you are done with checkWord();
Definition at line 146 of file k3spell.cpp.
Tells ISpell/ASpell to ignore this word for the life of this K3Spell instance.
- Returns
- false if
word
is not a word or there was an error communicating with ISpell/ASpell.
Definition at line 508 of file k3spell.cpp.
|
signal |
Emitted when the user pressed "Ignore All" in the dialog.
This could be used to make an application or file specific user dictionary.
|
signal |
Emitted when the user pressed "Ignore" in the dialog.
Don't know if this could be useful.
|
protected |
Definition at line 1577 of file k3spell.cpp.
QString K3Spell::intermediateBuffer | ( | ) | const |
Returns the partially spellchecked buffer.
You might want the full buffer in its partially-checked state.
Definition at line 161 of file k3spell.cpp.
|
protectedslot |
Definition at line 416 of file k3spell.cpp.
|
protectedslot |
Definition at line 1440 of file k3spell.cpp.
|
protectedslot |
Definition at line 422 of file k3spell.cpp.
K3SpellConfig K3Spell::ksConfig | ( | ) | const |
- Returns
- the K3SpellConfig object being used by this K3Spell instance.
Definition at line 1414 of file k3spell.cpp.
int K3Spell::lastPosition | ( | ) | const |
Returns the position (when using check()) or word number (when using checkList()) of the last word checked.
Definition at line 1141 of file k3spell.cpp.
|
signal |
Emitted whenever a misspelled word is found by check() or by checkWord().
If it is emitted by checkWord(), pos=0
. If it is emitted by check(), then pos
indicates the position of the misspelled word in the (original) _buffer
. (The first position is zero.) If it is emitted by checkList(), pos
is the index to the misspelled word in the QStringList passed to checkList(). Note, that originalword
can be only a word part, if it's a word with hyphens.
These are called before the dialog is opened, so that the calling program's GUI may be updated. (e.g. the misspelled word may be highlighted).
|
static |
- Deprecated:
- Performs a synchronous spellcheck.
This method does not return until spellchecking is done or canceled. Your application's GUI will still be updated, however.
Definition at line 1515 of file k3spell.cpp.
|
static |
Performs a synchronous spellcheck.
This method does not return until spellchecking is done or canceled. Your application's GUI will still be updated, however.
This overloaded method uses the spell-check configuration passed as parameter.
Definition at line 1521 of file k3spell.cpp.
void K3Spell::moveDlg | ( | int | x, |
int | y | ||
) |
Moves the dialog.
If the dialog is not currently visible, it will be placed at this position when it becomes visible. Use this to get the dialog out of the way of a highlighted misspelled word in a document.
Definition at line 1491 of file k3spell.cpp.
|
protected |
Definition at line 783 of file k3spell.cpp.
|
signal |
Emitted during a check().
i
is between 1 and 100.
|
signal |
Emitted after K3Spell has verified that ISpell/ASpell is running and working properly.
Emitted when the user pressed "ReplaceAll" in the dialog.
|
protected |
Definition at line 1351 of file k3spell.cpp.
void K3Spell::setAutoDelete | ( | bool | _autoDelete | ) |
Sets the auto-delete flag.
If this is set, the K3Spell object is automatically deleted after emitting death().
Definition at line 1435 of file k3spell.cpp.
void K3Spell::setIgnoreTitleCase | ( | bool | b | ) |
Call setIgnoreTitleCase(true) to tell the spell-checker to ignore words with a 'title' case, i.e.
starting with an uppercase letter. They are spell-checked by default.
Definition at line 1503 of file k3spell.cpp.
void K3Spell::setIgnoreUpperWords | ( | bool | b | ) |
Call setIgnoreUpperWords(true) to tell the spell-checker to ignore words that are completely uppercase.
They are spell-checked by default.
Definition at line 1498 of file k3spell.cpp.
void K3Spell::setProgressResolution | ( | unsigned int | res | ) |
Sets the resolution (in percent) of the progress() signals.
E.g. setProgressResolution (10) instructs K3Spell to send progress signals (at most) every 10% (10%, 20%, 30%...). The default is 10%.
Definition at line 1475 of file k3spell.cpp.
|
protected |
Definition at line 470 of file k3spell.cpp.
|
protectedslot |
Definition at line 1305 of file k3spell.cpp.
|
protected |
Definition at line 192 of file k3spell.cpp.
K3Spell::spellStatus K3Spell::status | ( | ) | const |
QStringList K3Spell::suggestions | ( | ) | const |
Returns list of suggested word replacements.
After calling checkWord() (an in response to a misspelled() signal you can use this to get the list of suggestions (if any were available).
Definition at line 148 of file k3spell.cpp.
|
protectedslot |
Definition at line 718 of file k3spell.cpp.
int K3Spell::widthDlg | ( | ) | const |
Returns the width of the dialog box.
Definition at line 159 of file k3spell.cpp.
|
protected |
Definition at line 503 of file k3spell.cpp.
Member Data Documentation
|
protected |
|
protected |
|
protected |
|
protected |
|
protected |
|
protected |
|
protected |
|
protected |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:26:48 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.