KDECore
#include <kcalendarsystem.h>
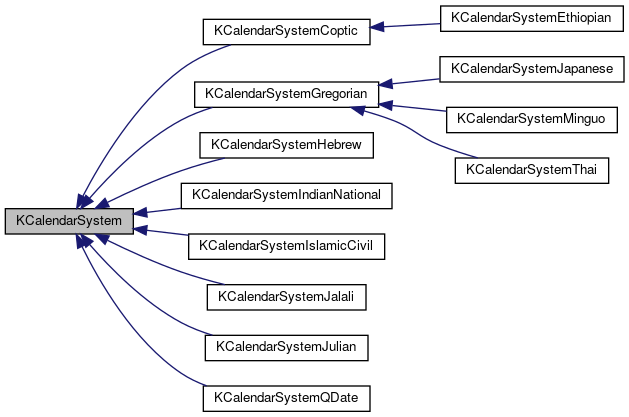
Public Types | |
enum | MonthNameFormat { ShortName, LongName, ShortNamePossessive, LongNamePossessive, NarrowName } |
enum | StringFormat { ShortFormat, LongFormat } |
enum | WeekDayNameFormat { ShortDayName, LongDayName, NarrowDayName } |
Public Member Functions | |
KCalendarSystem (const KLocale *locale=0) | |
KCalendarSystem (const KSharedConfig::Ptr config, const KLocale *locale=0) | |
virtual | ~KCalendarSystem () |
virtual QDate | addDays (const QDate &date, int ndays) const |
virtual QDate | addMonths (const QDate &date, int nmonths) const |
virtual QDate | addYears (const QDate &date, int nyears) const |
int | applyShortYearWindow (int inputYear) const |
QString | calendarLabel () const |
KLocale::CalendarSystem | calendarSystem () const |
virtual QString | calendarType () const =0 |
void | dateDifference (const QDate &fromDate, const QDate &toDate, int *yearsDiff, int *monthsDiff, int *daysDiff, int *direction) const |
virtual int | day (const QDate &date) const |
virtual int | dayOfWeek (const QDate &date) const |
QString | dayOfWeekString (const QDate &pDate) const |
virtual int | dayOfYear (const QDate &date) const |
QString | dayOfYearString (const QDate &pDate, StringFormat format=LongFormat) const |
int | daysDifference (const QDate &fromDate, const QDate &toDate) const |
virtual int | daysInMonth (const QDate &date) const |
int | daysInMonth (int year, int month) const |
QString | daysInMonthString (const QDate &pDate, StringFormat format=LongFormat) const |
virtual int | daysInWeek (const QDate &date) const |
QString | daysInWeekString (const QDate &date) const |
virtual int | daysInYear (const QDate &date) const |
int | daysInYear (int year) const |
QString | daysInYearString (const QDate &pDate, StringFormat format=LongFormat) const |
virtual QString | dayString (const QDate &pDate, StringFormat format=LongFormat) const |
virtual int | dayStringToInteger (const QString &sNum, int &iLength) const |
virtual QDate | earliestValidDate () const |
virtual QDate | epoch () const |
QString | eraName (const QDate &date, StringFormat format=ShortFormat) const |
QString | eraYear (const QDate &date, StringFormat format=ShortFormat) const |
QDate | firstDayOfMonth (int year, int month) const |
QDate | firstDayOfMonth (const QDate &date=QDate::currentDate()) const |
QDate | firstDayOfYear (int year) const |
QDate | firstDayOfYear (const QDate &date=QDate::currentDate()) const |
virtual QString | formatDate (const QDate &fromDate, KLocale::DateFormat toFormat=KLocale::LongDate) const |
QString | formatDate (const QDate &fromDate, const QString &toFormat, KLocale::DateTimeFormatStandard formatStandard=KLocale::KdeFormat) const |
QString | formatDate (const QDate &fromDate, const QString &toFormat, KLocale::DigitSet digitSet, KLocale::DateTimeFormatStandard formatStandard=KLocale::KdeFormat) const |
QString | formatDate (const QDate &date, KLocale::DateTimeComponent component, KLocale::DateTimeComponentFormat format=KLocale::DefaultComponentFormat, KLocale::WeekNumberSystem weekNumberSystem=KLocale::DefaultWeekNumber) const |
void | getDate (const QDate date, int *year, int *month, int *day) const |
virtual bool | isLeapYear (int year) const =0 |
virtual bool | isLeapYear (const QDate &date) const |
virtual bool | isLunar () const =0 |
virtual bool | isLunisolar () const =0 |
virtual bool | isProleptic () const =0 |
virtual bool | isSolar () const =0 |
virtual bool | isValid (int year, int month, int day) const =0 |
bool | isValid (int year, int dayOfYear) const |
bool | isValid (const QString &eraName, int yearInEra, int month, int day) const |
virtual bool | isValid (const QDate &date) const |
bool | isValidIsoWeekDate (int year, int isoWeekNumber, int dayOfIsoWeek) const |
QDate | lastDayOfMonth (int year, int month) const |
QDate | lastDayOfMonth (const QDate &date=QDate::currentDate()) const |
QDate | lastDayOfYear (int year) const |
QDate | lastDayOfYear (const QDate &date=QDate::currentDate()) const |
virtual QDate | latestValidDate () const |
virtual int | month (const QDate &date) const |
virtual QString | monthName (int month, int year, MonthNameFormat format=LongName) const =0 |
virtual QString | monthName (const QDate &date, MonthNameFormat format=LongName) const |
int | monthsDifference (const QDate &fromDate, const QDate &toDate) const |
virtual int | monthsInYear (const QDate &date) const |
int | monthsInYear (int year) const |
QString | monthsInYearString (const QDate &pDate, StringFormat format=LongFormat) const |
virtual QString | monthString (const QDate &pDate, StringFormat format=LongFormat) const |
virtual int | monthStringToInteger (const QString &sNum, int &iLength) const |
virtual QDate | readDate (const QString &str, bool *ok=0) const |
virtual QDate | readDate (const QString &str, KLocale::ReadDateFlags flags, bool *ok=0) const |
virtual QDate | readDate (const QString &dateString, const QString &dateFormat, bool *ok=0) const |
QDate | readDate (const QString &dateString, const QString &dateFormat, bool *ok, KLocale::DateTimeFormatStandard formatStandard) const |
virtual bool | setDate (QDate &date, int year, int month, int day) const |
bool | setDate (QDate &date, int year, int dayOfYear) const |
bool | setDate (QDate &date, QString eraName, int yearInEra, int month, int day) const |
bool | setDateIsoWeek (QDate &date, int year, int isoWeekNumber, int dayOfIsoWeek) const |
virtual bool | setYMD (QDate &date, int y, int m, int d) const |
int | shortYearWindowStartYear () const |
int | week (const QDate &date, int *yearNum=0) const |
int | week (const QDate &date, KLocale::WeekNumberSystem weekNumberSystem, int *yearNum=0) const |
virtual QString | weekDayName (int weekDay, WeekDayNameFormat format=LongDayName) const =0 |
virtual QString | weekDayName (const QDate &date, WeekDayNameFormat format=LongDayName) const |
virtual int | weekDayOfPray () const =0 |
virtual int | weekNumber (const QDate &date, int *yearNum=0) const |
QString | weekNumberString (const QDate &pDate, StringFormat format=LongFormat) const |
virtual int | weeksInYear (const QDate &date) const |
int | weeksInYear (const QDate &date, KLocale::WeekNumberSystem weekNumberSystem) const |
virtual int | weeksInYear (int year) const |
int | weeksInYear (int year, KLocale::WeekNumberSystem weekNumberSystem) const |
QString | weeksInYearString (const QDate &pDate, StringFormat format=LongFormat) const |
virtual int | weekStartDay () const |
virtual int | year (const QDate &date) const |
int | yearInEra (const QDate &date) const |
QString | yearInEraString (const QDate &date, StringFormat format=ShortFormat) const |
int | yearsDifference (const QDate &fromDate, const QDate &toDate) const |
virtual QString | yearString (const QDate &date, StringFormat format=LongFormat) const |
virtual int | yearStringToInteger (const QString &sNum, int &iLength) const |
Static Public Member Functions | |
static QString | calendarLabel (const QString &calendarType) |
static QString | calendarLabel (KLocale::CalendarSystem calendarSystem, const KLocale *locale=KGlobal::locale()) |
static KLocale::CalendarSystem | calendarSystem (const QString &calendarType) |
static KLocale::CalendarSystem | calendarSystemForCalendarType (const QString &calendarType) |
static QStringList | calendarSystems () |
static QList < KLocale::CalendarSystem > | calendarSystemsList () |
static QString | calendarType (KLocale::CalendarSystem calendarSystem) |
static KCalendarSystem * | create (const QString &calType=QLatin1String("gregorian"), const KLocale *locale=0) |
static KCalendarSystem * | create (const QString &calType, KSharedConfig::Ptr config, const KLocale *locale=0) |
static KCalendarSystem * | create (KLocale::CalendarSystem calendarSystem, const KLocale *locale=0) |
static KCalendarSystem * | create (KLocale::CalendarSystem calendarSystem, KSharedConfig::Ptr config, const KLocale *locale=0) |
Protected Member Functions | |
KCalendarSystem (KCalendarSystemPrivate &dd, const KSharedConfig::Ptr config=KSharedConfig::Ptr(), const KLocale *locale=0) | |
virtual bool | dateToJulianDay (int year, int month, int day, int &jd) const =0 |
virtual bool | julianDayToDate (int jd, int &year, int &month, int &day) const =0 |
const KLocale * | locale () const |
void | setHasYear0 (bool hasYear0) |
void | setMaxDaysInWeek (int maxDays) |
void | setMaxMonthsInYear (int maxMonths) |
Detailed Description
KCalendarSystem abstract base class, provides support for local Calendar Systems in KDE.
Derived classes must be created through the create() static method
Definition at line 40 of file kcalendarsystem.h.
Member Enumeration Documentation
Format for returned month / day name.
Definition at line 55 of file kcalendarsystem.h.
Format for returned year number / month number / day number as string.
Enumerator | |
---|---|
ShortFormat |
Short string format, e.g. 2000 = "00" or 6 = "6" |
LongFormat |
Long string format, e.g. 2000 = "2000" or 6 = "06" |
Definition at line 47 of file kcalendarsystem.h.
Format for returned month / day name.
Enumerator | |
---|---|
ShortDayName |
Short name format, e.g. "Fri" |
LongDayName |
Long name format, e.g. "Friday" |
NarrowDayName |
Narrow name format, e.g. "F".
|
Definition at line 66 of file kcalendarsystem.h.
Constructor & Destructor Documentation
|
explicit |
Constructor of abstract calendar class.
This will be called by derived classes.
- Parameters
-
locale locale to use for translations. The global locale is used if null.
Definition at line 1073 of file kcalendarsystem.cpp.
|
explicit |
Constructor of abstract calendar class.
This will be called by derived classes.
- Parameters
-
config a configuration file with a 'KCalendarSystem calendarName' group detailing locale-related preferences (such as era options). The global config is used if null. locale locale to use for translations. The global locale is used if null.
Definition at line 1080 of file kcalendarsystem.cpp.
|
virtual |
Destructor.
Definition at line 1094 of file kcalendarsystem.cpp.
|
protected |
Constructor of abstract calendar class.
This will be called by derived classes.
- Parameters
-
dd derived private d-pointer. config a configuration file with a 'KCalendarSystem calendarName' group detailing locale-related preferences (such as era options). The global config is used if null. locale locale to use for translations. The global locale is used if null.
Definition at line 1087 of file kcalendarsystem.cpp.
Member Function Documentation
Returns a QDate containing a date ndays
days later.
- Parameters
-
date The old date ndays number of days to add
- Returns
- The new date, null date if any errors
Definition at line 1502 of file kcalendarsystem.cpp.
Returns a QDate containing a date nmonths
months later.
- Parameters
-
date The old date nmonths number of months to add
- Returns
- The new date, null date if any errors
Definition at line 1463 of file kcalendarsystem.cpp.
Returns a QDate containing a date nyears
years later.
- Parameters
-
date The old date nyears The number of years to add
- Returns
- The new date, null date if any errors
Definition at line 1435 of file kcalendarsystem.cpp.
int KCalendarSystem::applyShortYearWindow | ( | int | inputYear | ) | const |
- Since
- 4.6
Returns the Year Number after applying the Year Window.
If the inputYear
is between 0 and 99, then apply the Year Window and return the calculated Year Number.
If the inputYear
is not between 0 and 99, then the original Year Number is returned.
- See also
- KLocale::setYearWindowOffset
- KLocale::yearWindowOffset
- Parameters
-
inputYear the year number to apply the year window to
- Returns
- the year number after applying the year window
Definition at line 2398 of file kcalendarsystem.cpp.
- Deprecated:
- use calendarLabel( KLocale::CalendarSystem ) instead
Returns a typographically correct and translated label to display for the calendar system type. Use with calendarSystems() to neatly format labels to display on combo widget of available calendar systems.
- Parameters
-
calendarType the specific calendar type to return the label for
- Returns
- label for calendar
Definition at line 78 of file kcalendarsystem.cpp.
|
static |
- Since
- 4.6
Returns a localized label to display for the required Calendar System type.
Use with calendarSystemsList() to populate selection lists of available calendar systems.
- Parameters
-
calendarSystem the specific calendar type to return the label for locale the locale to use for the label, defaults to global
- Returns
- label for calendar
Definition at line 146 of file kcalendarsystem.cpp.
QString KCalendarSystem::calendarLabel | ( | ) | const |
- Since
- 4.6
Returns a localized label to display for the current Calendar System type.
- Returns
- localized label for this Calendar System
Definition at line 1108 of file kcalendarsystem.cpp.
|
static |
- Since
- 4.7
Returns the Calendar System enum value for a given Calendar Type, e.g. KLocale::QDateCalendar for "gregorian"
- Parameters
-
calendarType the calendar type to convert
- Returns
- calendar system for calendar type
Definition at line 183 of file kcalendarsystem.cpp.
KLocale::CalendarSystem KCalendarSystem::calendarSystem | ( | ) | const |
- Since
- 4.6
Returns the Calendar System type of the KCalendarSystem object
- Returns
- type of calendar system
Definition at line 1100 of file kcalendarsystem.cpp.
|
static |
- Deprecated:
- use calendarSystem(const QString &calendarType) instead
- Since
- 4.6
Returns the Calendar System enum value for a given Calendar Type, e.g. KLocale::QDateCalendar for "gregorian"
- Parameters
-
calendarType the calendar type to convert
- Returns
- calendar system for calendar type
Definition at line 178 of file kcalendarsystem.cpp.
|
static |
- Deprecated:
- use calendarSystemsList() instead
Gets a list of names of supported calendar systems.
- Returns
- list of names
Definition at line 58 of file kcalendarsystem.cpp.
|
static |
- Since
- 4.6
Returns the list of currently supported Calendar Systems
- Returns
- list of Calendar Systems
Definition at line 126 of file kcalendarsystem.cpp.
|
static |
- Since
- 4.7
Returns the deprecated Calendar Type for a given Calendar System enum value, e.g. "gregorian" for KLocale::QDateCalendar
- Parameters
-
calendarSystem the calendar system to convert
- Returns
- calendar type for calendar system
Definition at line 214 of file kcalendarsystem.cpp.
|
pure virtual |
- Deprecated:
- use calendarSystem() instead
Returns the calendar system type.
- Returns
- type of calendar system
Implemented in KCalendarSystemIslamicCivil, KCalendarSystemGregorian, KCalendarSystemHebrew, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemQDate, KCalendarSystemEthiopian, KCalendarSystemJapanese, KCalendarSystemMinguo, KCalendarSystemThai, KCalendarSystemCoptic, and KCalendarSystemJalali.
|
static |
- Deprecated:
- use create(KLocale::CalendarSystem, KLocale) instead
Creates specific calendar type
- Parameters
-
calType string identification of the specific calendar type to be constructed locale locale to use for translations. The global locale is used if null.
- Returns
- a KCalendarSystem object
Definition at line 47 of file kcalendarsystem.cpp.
|
static |
- Deprecated:
- use create(KLocale::CalendarSystem, KSharedConfig, KLocale) instead
- Since
- 4.5
Creates specific calendar type
- Parameters
-
calType string identification of the specific calendar type to be constructed config a configuration file with a 'KCalendarSystem calendarType' group detailing locale-related preferences (such as era options). The global config is used if null. locale locale to use for translations. The global locale is used if null.
- Returns
- a KCalendarSystem object
Definition at line 52 of file kcalendarsystem.cpp.
|
static |
- Since
- 4.6
Creates a KCalendarSystem object for the required Calendar System
- Parameters
-
calendarSystem the Calendar System to create, defaults to QDate compatible locale locale to use for translations. The global locale is used if null.
- Returns
- a KCalendarSystem object
Definition at line 87 of file kcalendarsystem.cpp.
|
static |
- Since
- 4.6
Creates a KCalendarSystem object for the required Calendar System
- Parameters
-
calendarSystem the Calendar System to create config a configuration file with a 'KCalendarSystem calendarType' group detailing locale-related preferences (such as era options). The global config is used if null. locale locale to use for translations. The global locale is used if null.
- Returns
- a KCalendarSystem object
Definition at line 92 of file kcalendarsystem.cpp.
void KCalendarSystem::dateDifference | ( | const QDate & | fromDate, |
const QDate & | toDate, | ||
int * | yearsDiff, | ||
int * | monthsDiff, | ||
int * | daysDiff, | ||
int * | direction | ||
) | const |
Returns the difference between two dates in years, months and days.
The difference is always caculated from the earlier date to the later date in year, month and day order, with the direction
parameter indicating which direction the difference is applied from the toDate
.
For example, the difference between 2010-06-10 and 2012-09-5 is 2 years, 2 months and 26 days. Note that the difference between two last days of the month is always 1 month, e.g. 2010-01-31 to 2010-02-28 is 1 month not 28 days.
- Parameters
-
fromDate The date to start from toDate The date to end at yearsDiff Returns number of years difference monthsDiff Returns number of months difference daysDiff Returns number of days difference direction Returns direction of difference, 1 if fromDate <= toDate, -1 otherwise
Definition at line 1519 of file kcalendarsystem.cpp.
|
protectedpure virtual |
Internal method to convert YMD values for this calendar system into a Julian Day number.
All calendar system implementations MUST implement julianDayToDate and dateToJulianDay methods as all other methods can be expressed as functions of these. Does no internal validity checking.
- See also
- KCalendarSystem::julianDayToDate
- Parameters
-
year year number month month number day day of month jd Julian day number returned in this variable
- Returns
true
if the date is valid,false
otherwise
Implemented in KCalendarSystemQDate, KCalendarSystemHebrew, KCalendarSystemGregorian, KCalendarSystemIslamicCivil, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemJapanese, KCalendarSystemEthiopian, KCalendarSystemMinguo, KCalendarSystemCoptic, KCalendarSystemJalali, and KCalendarSystemThai.
Definition at line 2448 of file kcalendarsystem.cpp.
|
virtual |
Returns the day portion of a given date in the current calendar system.
- Parameters
-
date date to return day for
- Returns
- day of the month, 0 if input date is invalid
Reimplemented in KCalendarSystemQDate.
Definition at line 1357 of file kcalendarsystem.cpp.
|
virtual |
Returns the weekday number for the given date.
The weekdays are numbered 1..7 for Monday..Sunday.
This value is not affected by the value of weekStartDay()
- Parameters
-
date the date to obtain day from
- Returns
- day of week number, -1 if input date not valid
Reimplemented in KCalendarSystemQDate, and KCalendarSystemHebrew.
Definition at line 1686 of file kcalendarsystem.cpp.
- Deprecated:
- use formatDate(QDate, KLocale::DateTimeComponent, KLocale::DateTimeComponentFormat)
- Since
- 4.4
Converts a date into a day of week literal
- Parameters
-
pDate The date to convert
- Returns
- The day of week literal of the date, empty string if any error
- See also
- dayOfWeek()
Definition at line 1957 of file kcalendarsystem.cpp.
|
virtual |
Returns the day number of year for the given date.
The days are numbered 1..daysInYear()
- Parameters
-
date the date to obtain day from
- Returns
- day of year number, -1 if input date not valid
Reimplemented in KCalendarSystemQDate.
Definition at line 1675 of file kcalendarsystem.cpp.
QString KCalendarSystem::dayOfYearString | ( | const QDate & | pDate, |
StringFormat | format = LongFormat |
||
) | const |
- Deprecated:
- use formatDate(QDate, KLocale::DateTimeComponent, KLocale::DateTimeComponentFormat)
- Since
- 4.4
Converts a date into a day of year literal
- Parameters
-
pDate The date to convert format The format to return, either short or long
- Returns
- The day of year literal of the date, empty string if any error
- See also
- dayOfYear()
Definition at line 1947 of file kcalendarsystem.cpp.
Returns the difference between two dates in days The returned value will be negative if fromDate
> toDate
.
- Parameters
-
fromDate The date to start from toDate The date to end at
- Returns
- The number of days difference
Definition at line 1554 of file kcalendarsystem.cpp.
|
virtual |
Returns the number of days in the given month.
- Parameters
-
date the date to obtain month from
- Returns
- number of days in month, -1 if input date invalid
Reimplemented in KCalendarSystemQDate.
Definition at line 1643 of file kcalendarsystem.cpp.
int KCalendarSystem::daysInMonth | ( | int | year, |
int | month | ||
) | const |
- Since
- 4.5
Returns the number of days in the given month.
- Parameters
-
year the year the month is in month the month
- Returns
- number of days in month, -1 if input date invalid
Definition at line 1657 of file kcalendarsystem.cpp.
QString KCalendarSystem::daysInMonthString | ( | const QDate & | pDate, |
StringFormat | format = LongFormat |
||
) | const |
- Deprecated:
- use formatDate(QDate, KLocale::DateTimeComponent, KLocale::DateTimeComponentFormat)
- Since
- 4.4
Returns the days in month for a date as a numeric string
- Parameters
-
pDate The date to convert format The format to return, either short or long
- Returns
- The days in month literal of the date, empty string if any error
- See also
- daysInMonth()
Definition at line 2003 of file kcalendarsystem.cpp.
|
virtual |
Returns the number of days in the given week.
- Parameters
-
date the date to obtain week from
- Returns
- number of days in week, -1 if input date invalid
Definition at line 1668 of file kcalendarsystem.cpp.
- Deprecated:
- use formatDate(QDate, KLocale::DateTimeComponent, KLocale::DateTimeComponentFormat)
- Since
- 4.4
Returns the days in week for a date as a numeric string
- Parameters
-
date The date to convert
- Returns
- The days in week literal of the date, empty string if any error
- See also
- daysInWeek()
Definition at line 2013 of file kcalendarsystem.cpp.
|
virtual |
Returns the number of days in the given year.
- Parameters
-
date the date to obtain year from
- Returns
- number of days in year, -1 if input date invalid
Reimplemented in KCalendarSystemQDate.
Definition at line 1620 of file kcalendarsystem.cpp.
int KCalendarSystem::daysInYear | ( | int | year | ) | const |
- Since
- 4.5
Returns the number of days in the given year.
- Parameters
-
year the year
- Returns
- number of days in year, -1 if input date invalid
Definition at line 1632 of file kcalendarsystem.cpp.
QString KCalendarSystem::daysInYearString | ( | const QDate & | pDate, |
StringFormat | format = LongFormat |
||
) | const |
- Deprecated:
- use formatDate(QDate, KLocale::DateTimeComponent, KLocale::DateTimeComponentFormat)
- Since
- 4.4
Returns the days in year for a date as a numeric string
- Parameters
-
pDate The date to convert format The format to return, either short or long
- Returns
- The days in year literal of the date, empty string if any error
- See also
- daysInYear()
Definition at line 1993 of file kcalendarsystem.cpp.
|
virtual |
- Deprecated:
- use formatDate(QDate, KLocale::DateTimeComponent, KLocale::DateTimeComponentFormat)
Converts a date into a day literal
- Parameters
-
pDate The date to convert format The format to return, either short or long
- Returns
- The day literal of the date, empty string if any error
- See also
- day()
Definition at line 1927 of file kcalendarsystem.cpp.
|
virtual |
- Deprecated:
- for internal use only
Converts a day literal of a part of a string into a integer starting at the beginning of the string
- Parameters
-
sNum The string to parse iLength The number of QChars used, and 0 if no valid symbols was found in the string
- Returns
- An integer corresponding to the day
Definition at line 2042 of file kcalendarsystem.cpp.
|
virtual |
Returns the earliest date valid in this calendar system implementation.
If the calendar system is proleptic then this may be before epoch.
- Returns
- date the earliest valid date
Reimplemented in KCalendarSystemIslamicCivil, KCalendarSystemGregorian, KCalendarSystemHebrew, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemQDate, KCalendarSystemEthiopian, KCalendarSystemJapanese, KCalendarSystemMinguo, KCalendarSystemThai, KCalendarSystemCoptic, and KCalendarSystemJalali.
Definition at line 1120 of file kcalendarsystem.cpp.
|
virtual |
Returns a QDate holding the epoch of the calendar system.
Usually YMD of 1/1/1, access the returned QDates method toJulianDay() if you require the actual Julian day number. Note: a particular calendar system implementation may not include the epoch in its supported range, or the calendar system may be proleptic in which case it supports dates before the epoch.
- See also
- KCalendarSystem::earliestValidDate
- KCalendarSystem::latestValidDate
- KCalendarSystem::isProleptic
- KCalendarSystem::isValid
- Returns
- epoch of calendar system
Reimplemented in KCalendarSystemIslamicCivil, KCalendarSystemGregorian, KCalendarSystemHebrew, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemQDate, KCalendarSystemEthiopian, KCalendarSystemJapanese, KCalendarSystemMinguo, KCalendarSystemThai, KCalendarSystemCoptic, and KCalendarSystemJalali.
Definition at line 1115 of file kcalendarsystem.cpp.
QString KCalendarSystem::eraName | ( | const QDate & | date, |
StringFormat | format = ShortFormat |
||
) | const |
- Since
- 4.5
Returns the Era Name portion of a given date in the current calendar system, for example "AD" or "Anno Domini" for the Gregorian calendar and Christian Era.
- Parameters
-
date date to return Era Name for format format to return, either short or long
- Returns
- era name, empty string if input date is invalid
Definition at line 1371 of file kcalendarsystem.cpp.
QString KCalendarSystem::eraYear | ( | const QDate & | date, |
StringFormat | format = ShortFormat |
||
) | const |
- Since
- 4.5
Returns the Era Year portion of a given date in the current calendar system, for example "2000 AD" or "Heisei 22".
- Parameters
-
date date to return Era Year for format format to return, either short or long
- Returns
- era name, empty string if input date is invalid
Definition at line 1387 of file kcalendarsystem.cpp.
QDate KCalendarSystem::firstDayOfMonth | ( | int | year, |
int | month | ||
) | const |
- Since
- 4.6
Returns a QDate containing the first day of the month
- Parameters
-
year The year to return the date for month The month to return the date for
- Returns
- The first day of the month
Definition at line 1791 of file kcalendarsystem.cpp.
QDate KCalendarSystem::firstDayOfMonth | ( | const QDate & | date = QDate::currentDate() | ) | const |
- Since
- 4.6
Returns a QDate containing the first day of the month
- Parameters
-
date The month to return the date for, defaults to today
- Returns
- The first day of the month
Definition at line 1815 of file kcalendarsystem.cpp.
QDate KCalendarSystem::firstDayOfYear | ( | int | year | ) | const |
- Since
- 4.6
Returns a QDate containing the first day of the year
- Parameters
-
year The year to return the date for
- Returns
- The first day of the year
Definition at line 1743 of file kcalendarsystem.cpp.
QDate KCalendarSystem::firstDayOfYear | ( | const QDate & | date = QDate::currentDate() | ) | const |
- Since
- 4.6
Returns a QDate containing the first day of the year
- Parameters
-
date The year to return the date for, defaults to today
- Returns
- The first day of the year
Definition at line 1767 of file kcalendarsystem.cpp.
|
virtual |
Returns a string formatted to the current locale's conventions regarding dates.
Uses the calendar system's internal locale set when the instance was created, which ensures that the correct calendar system and locale settings are respected, which would not occur in some cases if using the global locale. Defaults to global locale.
- See also
- KLocale::formatDate
- Parameters
-
fromDate the date to be formatted toFormat category of date format to use
- Returns
- The date as a string
Definition at line 2048 of file kcalendarsystem.cpp.
QString KCalendarSystem::formatDate | ( | const QDate & | fromDate, |
const QString & | toFormat, | ||
KLocale::DateTimeFormatStandard | formatStandard = KLocale::KdeFormat |
||
) | const |
- Since
- 4.4
Returns a string formatted to the given format and localised to the correct language and digit set using the requested format standard.
*** WITH GREAT POWER COMES GREAT RESPONSIBILITY ***
Please use with care and only in situations where the DateFormat enum or locale formats or individual string methods do not provide what you need. You should almost always translate your format string as documented. Using the standard DateFormat options instead would take care of the translation for you.
Warning: The n element differs from the GNU/POSIX standard where it is defined as a newline. KDE currently uses this for short day number. It is recommended for compatibility purposes to use %-m instead.
The toFormat parameter is a good candidate to be made translatable, so that translators can adapt it to their language's convention. There should also be a context using the "kdedt-format" keyword (for automatic validation of translations) and stating the format's purpose:
The date format string can be defined using either the KDE or POSIX standards. The KDE standard closely follows the POSIX standard but with some exceptions. Always use the KDE standard within KDE, but where interaction is required with external POSIX compliant systems (e.g. Gnome, glibc, etc) the POSIX standard should be used.
Date format strings are made up of date componants and string literals. Date componants are prefixed by a % escape character and are made up of optional padding and case modifier flags, an optional width value, and a compulsary code for the actual date componant: %[Flags][Width][Componant] e.g. _^5Y No spaces are allowed.
The Flags can modify the padding character and/or case of the Date Componant. The Flags are optional and may be combined and/or repeated in any order, in which case the last Padding Flag and last Case Flag will be the ones used. The Flags must be immediately after the % and before any Width.
The Width can modify how wide the date Componant is padded to. The Width is an optional interger value and must be after any Flags but before the Componant. If the Width is less than the minimum defined for a Componant then the default minimum will be used instead.
By default most numeric Date Componants are right-aligned with leading 0's.
By default all string name fields are capital case and unpadded.
The following Flags may be specified:
- - (hyphen) no padding (e.g. 1 Jan and "%-j" = "1")
- _ (underscore) pad with spaces (e.g. 1 Jan and "%-j" = " 1")
- 0 (zero) pad with 0's (e.g. 1 Jan and "%0j" = "001")
- ^ (caret) make uppercase (e.g. 1 Jan and "%^B" = "JANUARY")
- # (hash) invert case (e.g. 1 Jan and "%#B" = "???")
The following Date Componants can be specified:
- Y the year to 4 digits (e.g. "1984" for 1984, "0584" for 584, "0084" for 84)
- C the 'century' portion of the year to 2 digits (e.g. "19" for 1984, "05" for 584, "00" for 84)
- y the lower 2 digits of the year to 2 digits (e.g. "84" for 1984, "05" for 2005)
- EY the full local era year (e.g. "2000 AD")
- EC the era name short form (e.g. "AD")
- Ey the year in era to 1 digit (e.g. 1 or 2000)
- m the month number to 2 digits (January="01", December="12")
- n the month number to 1 digit (January="1", December="12"), see notes!
- d the day number of the month to 2 digits (e.g. "01" on the first of March)
- e the day number of the month to 1 digit (e.g. "1" on the first of March)
- B the month name long form (e.g. "January")
- b the month name short form (e.g. "Jan" for January)
- h the month name short form (e.g. "Jan" for January)
- A the weekday name long form (e.g. "Wednesday" for Wednesday)
- a the weekday name short form (e.g. "Wed" for Wednesday)
- j the day of the year number to 3 digits (e.g. "001" for 1 Jan)
- V the ISO week of the year number to 2 digits (e.g. "01" for ISO Week 1)
- G the year number in long form of the ISO week of the year to 4 digits (e.g. "2004" for 1 Jan 2005)
- g the year number in short form of the ISO week of the year to 2 digits (e.g. "04" for 1 Jan 2005)
- u the day of the week number to 1 digit (e.g. "1" for Monday)
- D the US short date format (e.g. "%m/%d/%y")
- F the ISO short date format (e.g. "%Y-%m-%d")
- x the KDE locale short date format
- %% the literal "%"
- t a tab character
Everything else in the format string will be taken as literal text.
Examples: "%Y-%m-%d" = "2009-01-01" "%Y-%-m-%_4d" = "2009-1- 1"
The following format codes behave differently in the KDE and POSIX standards
- e in GNU/POSIX is space padded to 2 digits, in KDE is not padded
- n in GNU/POSIX is newline, in KDE is short month number
The following POSIX format codes are currently not supported:
- U US week number
- w US day of week
- W US week number
- O locale's alternative numeric symbols, in KDE is not supported
%0 is not supported as the returned result is always in the locale's chosen numeric symbol digit set.
- See also
- KLocale::formatDate
- Parameters
-
fromDate the date to be formatted toFormat the date format to use formatStandard the standard the date format uses, defaults to KDE Standard
- Returns
- The date as a string
Definition at line 2092 of file kcalendarsystem.cpp.
QString KCalendarSystem::formatDate | ( | const QDate & | fromDate, |
const QString & | toFormat, | ||
KLocale::DigitSet | digitSet, | ||
KLocale::DateTimeFormatStandard | formatStandard = KLocale::KdeFormat |
||
) | const |
- Since
- 4.4
Returns a string formatted to the given format string and Digit Set. Only use this version if you need control over the Digit Set and do not want to use the locale Digit Set.
- See also
- formatDate
- Parameters
-
fromDate the date to be formatted toFormat the date format to use digitSet the Digit Set to format the date in formatStandard the standard the date format uses, defaults to KDE Standard
- Returns
- The date as a string
Definition at line 2099 of file kcalendarsystem.cpp.
QString KCalendarSystem::formatDate | ( | const QDate & | date, |
KLocale::DateTimeComponent | component, | ||
KLocale::DateTimeComponentFormat | format = KLocale::DefaultComponentFormat , |
||
KLocale::WeekNumberSystem | weekNumberSystem = KLocale::DefaultWeekNumber |
||
) | const |
- Since
- 4.6
Returns a Date Component as a localized string in the requested format.
For example for 2010-01-01 the KLocale::Month with en_US Locale and Gregorian calendar may return: KLocale::ShortNumber = "1" KLocale::LongNumber = "01" KLocale::NarrowName = "J" KLocale::ShortName = "Jan" KLocale::LongName = "January"
- Parameters
-
date The date to format component The date component to return format The format to return the component
inweekNumberSystem To override the default Week Number System to use
- Returns
- The localized string form of the date component
Definition at line 2111 of file kcalendarsystem.cpp.
void KCalendarSystem::getDate | ( | const QDate | date, |
int * | year, | ||
int * | month, | ||
int * | day | ||
) | const |
- Since
- 4.5
Returns the year, month and day portion of a given date in the current calendar system
- Parameters
-
date date to get year, month and day for year year number returned in this variable month month number returned in this variable day day of month returned in this variable
Definition at line 1307 of file kcalendarsystem.cpp.
|
pure virtual |
Returns whether a given year is a leap year.
Input year must be checked for validity in current Calendar System prior to calling, no validity checking performed in this routine, behaviour is undefined in invalid case.
- Parameters
-
year the year to check
- Returns
true
if the year is a leap year,false
otherwise
Implemented in KCalendarSystemQDate, KCalendarSystemHebrew, KCalendarSystemIslamicCivil, KCalendarSystemGregorian, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemEthiopian, KCalendarSystemJapanese, KCalendarSystemMinguo, KCalendarSystemThai, KCalendarSystemCoptic, and KCalendarSystemJalali.
Definition at line 1720 of file kcalendarsystem.cpp.
Returns whether a given date falls in a leap year.
Input date must be checked for validity in current Calendar System prior to calling, no validity checking performed in this routine, behaviour is undefined in invalid case.
- Parameters
-
date the date to check
- Returns
true
if the date falls in a leap year,false
otherwise
Reimplemented in KCalendarSystemQDate, KCalendarSystemHebrew, KCalendarSystemIslamicCivil, KCalendarSystemGregorian, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemEthiopian, KCalendarSystemJapanese, KCalendarSystemMinguo, KCalendarSystemThai, KCalendarSystemCoptic, and KCalendarSystemJalali.
Definition at line 1731 of file kcalendarsystem.cpp.
|
pure virtual |
Returns whether the calendar is lunar based.
- Returns
true
if the calendar is lunar based,false
if not
Implemented in KCalendarSystemQDate, KCalendarSystemHebrew, KCalendarSystemGregorian, KCalendarSystemIslamicCivil, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemJapanese, KCalendarSystemEthiopian, KCalendarSystemMinguo, KCalendarSystemCoptic, KCalendarSystemJalali, and KCalendarSystemThai.
|
pure virtual |
Returns whether the calendar is lunisolar based.
- Returns
true
if the calendar is lunisolar based,false
if not
Implemented in KCalendarSystemQDate, KCalendarSystemHebrew, KCalendarSystemGregorian, KCalendarSystemIslamicCivil, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemJapanese, KCalendarSystemEthiopian, KCalendarSystemMinguo, KCalendarSystemCoptic, KCalendarSystemJalali, and KCalendarSystemThai.
|
pure virtual |
Returns whether the calendar system is proleptic, i.e.
whether dates before the epoch are supported.
- See also
- KCalendarSystem::epoch
- Returns
true
if the calendar system is proleptic,false
if not
Implemented in KCalendarSystemQDate, KCalendarSystemHebrew, KCalendarSystemGregorian, KCalendarSystemIslamicCivil, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemJapanese, KCalendarSystemEthiopian, KCalendarSystemMinguo, KCalendarSystemCoptic, KCalendarSystemJalali, and KCalendarSystemThai.
|
pure virtual |
Returns whether the calendar is solar based.
- Returns
true
if the calendar is solar based,false
if not
Implemented in KCalendarSystemQDate, KCalendarSystemHebrew, KCalendarSystemGregorian, KCalendarSystemIslamicCivil, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemJapanese, KCalendarSystemEthiopian, KCalendarSystemMinguo, KCalendarSystemCoptic, KCalendarSystemJalali, and KCalendarSystemThai.
|
pure virtual |
Returns whether a given date is valid in this calendar system.
- Parameters
-
year the year portion of the date to check month the month portion of the date to check day the day portion of the date to check
- Returns
true
if the date is valid,false
otherwise
Implemented in KCalendarSystemIslamicCivil, KCalendarSystemGregorian, KCalendarSystemHebrew, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemQDate, KCalendarSystemEthiopian, KCalendarSystemJapanese, KCalendarSystemMinguo, KCalendarSystemThai, KCalendarSystemCoptic, and KCalendarSystemJalali.
Definition at line 1133 of file kcalendarsystem.cpp.
bool KCalendarSystem::isValid | ( | int | year, |
int | dayOfYear | ||
) | const |
- Since
- 4.4
Returns whether a given date is valid in this calendar system.
- Parameters
-
year the year portion of the date to check dayOfYear the day of year portion of the date to check
- Returns
true
if the date is valid,false
otherwise
Definition at line 1154 of file kcalendarsystem.cpp.
- Since
- 4.5
Returns whether a given date is valid in this calendar system.
- Parameters
-
eraName the Era Name portion of the date to check yearInEra the Year In Era portion of the date to check month the Month portion of the date to check day the Day portion of the date to check
- Returns
true
if the date is valid,false
otherwise
Definition at line 1162 of file kcalendarsystem.cpp.
Returns whether a given date is valid in this calendar system.
- Parameters
-
date the date to check
- Returns
true
if the date is valid,false
otherwise
Reimplemented in KCalendarSystemIslamicCivil, KCalendarSystemGregorian, KCalendarSystemHebrew, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemQDate, KCalendarSystemEthiopian, KCalendarSystemJapanese, KCalendarSystemMinguo, KCalendarSystemThai, KCalendarSystemCoptic, and KCalendarSystemJalali.
Definition at line 1214 of file kcalendarsystem.cpp.
bool KCalendarSystem::isValidIsoWeekDate | ( | int | year, |
int | isoWeekNumber, | ||
int | dayOfIsoWeek | ||
) | const |
- Since
- 4.4
Returns whether a given date is valid in this calendar system.
- Parameters
-
year the year portion of the date to check isoWeekNumber the ISO week portion of the date to check dayOfIsoWeek the day of week portion of the date to check
- Returns
true
if the date is valid,false
otherwise
Definition at line 1171 of file kcalendarsystem.cpp.
|
protectedpure virtual |
Internal method to convert a Julian Day number into the YMD values for this calendar system.
All calendar system implementations MUST implement julianDayToDate and dateToJulianDay methods as all other methods can be expressed as functions of these. Does no internal validity checking.
- See also
- KCalendarSystem::dateToJulianDay
- Parameters
-
jd Julian day number to convert to date year year number returned in this variable month month number returned in this variable day day of month returned in this variable
- Returns
true
if the date is valid,false
otherwise
Implemented in KCalendarSystemQDate, KCalendarSystemHebrew, KCalendarSystemGregorian, KCalendarSystemIslamicCivil, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemJapanese, KCalendarSystemEthiopian, KCalendarSystemMinguo, KCalendarSystemCoptic, KCalendarSystemJalali, and KCalendarSystemThai.
Definition at line 2416 of file kcalendarsystem.cpp.
QDate KCalendarSystem::lastDayOfMonth | ( | int | year, |
int | month | ||
) | const |
- Since
- 4.6
Returns a QDate containing the last day of the month
- Parameters
-
year The year to return the date for month The month to return the date for
- Returns
- The last day of the month
Definition at line 1803 of file kcalendarsystem.cpp.
QDate KCalendarSystem::lastDayOfMonth | ( | const QDate & | date = QDate::currentDate() | ) | const |
- Since
- 4.6
Returns a QDate containing the last day of the month
- Parameters
-
date The month to return the date for, defaults to today
- Returns
- The last day of the month
Definition at line 1829 of file kcalendarsystem.cpp.
QDate KCalendarSystem::lastDayOfYear | ( | int | year | ) | const |
- Since
- 4.6
Returns a QDate containing the last day of the year
- Parameters
-
year The year to return the date for
- Returns
- The last day of the year
Definition at line 1755 of file kcalendarsystem.cpp.
QDate KCalendarSystem::lastDayOfYear | ( | const QDate & | date = QDate::currentDate() | ) | const |
- Since
- 4.6
Returns a QDate containing the last day of the year
- Parameters
-
date The year to return the date for, defaults to today
- Returns
- The last day of the year
Definition at line 1779 of file kcalendarsystem.cpp.
|
virtual |
Returns the latest date valid in this calendar system implementation.
- Returns
- date the latest valid date
Reimplemented in KCalendarSystemIslamicCivil, KCalendarSystemGregorian, KCalendarSystemHebrew, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemQDate, KCalendarSystemEthiopian, KCalendarSystemJapanese, KCalendarSystemMinguo, KCalendarSystemThai, KCalendarSystemCoptic, and KCalendarSystemJalali.
Definition at line 1127 of file kcalendarsystem.cpp.
|
protected |
Returns the locale used for translations and formats for this calendar system instance.
This allows a calendar system instance to be independent of the global translations and formats if required. All implementations must refer to this locale.
Only for internal calendar system use; if public access is required then provide public methods only for those methods actually required. Any app that creates an instance with its own locale overriding global will have the original handle to the locale and can manipulate it that way if required, e.g. to change default date format. Only expose those methods that library widgets require access to internally.
- See also
- KCalendarSystem::formatDate
- KLocale::formatDate
- KCalendarSystem::weekStartDay
- KLocale::weekStartDay
- KCalendarSystem::readDate
- KLocale::readDate
- Returns
- locale to use
Definition at line 2479 of file kcalendarsystem.cpp.
|
virtual |
Returns the month portion of a given date in the current calendar system.
- Parameters
-
date date to return month for
- Returns
- month of year, 0 if input date is invalid
Reimplemented in KCalendarSystemQDate.
Definition at line 1344 of file kcalendarsystem.cpp.
|
pure virtual |
Gets specific calendar type month name for a given month number If an invalid month is specified, QString() is returned.
- Parameters
-
month the month number year the year the month belongs to format specifies whether the short month name or long month name should be used
- Returns
- name of the month, empty string if any error
Implemented in KCalendarSystemQDate, KCalendarSystemHebrew, KCalendarSystemIslamicCivil, KCalendarSystemGregorian, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemEthiopian, KCalendarSystemJapanese, KCalendarSystemMinguo, KCalendarSystemCoptic, and KCalendarSystemJalali.
Definition at line 1842 of file kcalendarsystem.cpp.
|
virtual |
Gets specific calendar type month name for a given date.
- Parameters
-
date date to obtain month from format specifies whether the short month name or long month name should be used
- Returns
- name of the month, empty string if any error
Reimplemented in KCalendarSystemQDate, KCalendarSystemHebrew, KCalendarSystemIslamicCivil, KCalendarSystemGregorian, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemEthiopian, KCalendarSystemJapanese, KCalendarSystemMinguo, KCalendarSystemCoptic, and KCalendarSystemJalali.
Definition at line 1870 of file kcalendarsystem.cpp.
Returns the difference between two dates in completed calendar months The returned value will be negative if fromDate
> toDate
.
For example, the difference between 2010-06-10 and 2012-09-5 is 26 months. Note that the difference between two last days of the month is always 1 month, e.g. 2010-01-31 to 2010-02-28 is 1 month not 28 days.
- Parameters
-
fromDate The date to start from toDate The date to end at
- Returns
- The number of months difference
Definition at line 1542 of file kcalendarsystem.cpp.
|
virtual |
Returns number of months in the given year.
- Parameters
-
date the date to obtain year from
- Returns
- number of months in the year, -1 if input date invalid
Definition at line 1563 of file kcalendarsystem.cpp.
int KCalendarSystem::monthsInYear | ( | int | year | ) | const |
- Since
- 4.5
Returns number of months in the given year
- Parameters
-
year the required year
- Returns
- number of months in the year, -1 if input date invalid
Definition at line 1575 of file kcalendarsystem.cpp.
QString KCalendarSystem::monthsInYearString | ( | const QDate & | pDate, |
StringFormat | format = LongFormat |
||
) | const |
- Deprecated:
- use formatDate(QDate, KLocale::DateTimeComponent, KLocale::DateTimeComponentFormat)
- Since
- 4.4
Returns the months in year for a date as a numeric string
- Parameters
-
pDate The date to convert format The format to return, either short or long
- Returns
- The months in year literal of the date, empty string if any error
- See also
- monthsInYear()
Definition at line 1973 of file kcalendarsystem.cpp.
|
virtual |
- Deprecated:
- use formatDate(QDate, KLocale::DateTimeComponent, KLocale::DateTimeComponentFormat)
Converts a date into a month literal
- Parameters
-
pDate The date to convert format The format to return, either short or long
- Returns
- The month literal of the date, empty string if any error
- See also
- month()
Definition at line 1918 of file kcalendarsystem.cpp.
|
virtual |
- Deprecated:
- for internal use only
Converts a month literal of a part of a string into a integer starting at the beginning of the string
- Parameters
-
sNum The string to parse iLength The number of QChars used, and 0 if no valid symbols was found in the string
- Returns
- An integer corresponding to the month
Definition at line 2036 of file kcalendarsystem.cpp.
Converts a localized date string to a QDate.
The bool pointed by ok
will be false
if the date entered was invalid.
Uses the calendar system's internal locale set when the instance was created, which ensures that the correct calendar system and locale settings are respected, which would not occur in some cases if using the global locale. Defaults to global locale.
- See also
- KLocale::readDate
- Parameters
-
str the string to convert ok if non-null, will be set to true
if the date is valid,false
if invalid
- Returns
- the string converted to a QDate
Definition at line 2333 of file kcalendarsystem.cpp.
|
virtual |
Converts a localized date string to a QDate.
This method is stricter than readDate(str,&ok): it will either accept a date in full format or a date in short format, depending on flags
.
Uses the calendar system's internal locale set when the instance was created, which ensures that the correct calendar system and locale settings are respected, which would not occur in some cases if using the global locale. Defaults to global locale.
- See also
- KLocale::readDate
- Parameters
-
str the string to convert flags whether the date string is to be in full format or in short format ok if non-null, will be set to true
if the date is valid,false
if invalid
- Returns
- the string converted to a QDate
Definition at line 2354 of file kcalendarsystem.cpp.
|
virtual |
Converts a localized date string to a QDate, using the specified format
.
You will usually not want to use this method. Uses teh KDE format standard.
- Parameters
-
dateString the string to convert dateFormat the date format to use, in KDE format standard ok if non-null, will be set to true
if the date is valid,false
if invalid
- Returns
- the string converted to a QDate
- See also
- formatDate
- KLocale::readDate
Definition at line 2372 of file kcalendarsystem.cpp.
QDate KCalendarSystem::readDate | ( | const QString & | dateString, |
const QString & | dateFormat, | ||
bool * | ok, | ||
KLocale::DateTimeFormatStandard | formatStandard | ||
) | const |
Converts a localized date string to a QDate, using the specified format
.
You will usually not want to use this method.
You must supply a format and string containing at least one of the following combinations to create a valid date:
- a month and day of month
- a day of year
- a ISO week number and day of week
If a year number is not supplied then the current year will be assumed.
All date componants must be separated by a non-numeric character.
The format is not applied strictly to the input string:
- extra whitespace is ignored
- leading 0's on numbers are ignored
- capitalisation of literals is ignored
The allowed format componants are almost the same as the formatDate() function. The following date componants will be read:
- Y the whole year (e.g. "1984" for 1984)
- y the lower 2 digits of the year (e.g. "84" for 1984)
- EY the full local era year (e.g. "2000 AD")
- EC the era name short form (e.g. "AD")
- Ey the year in era to 1 digit (e.g. 1 or 2000)
- m the month number to two digits (January="01", December="12")
- n the month number (January="1", December="12")
- d the day number of the month to two digits (e.g. "01" on the first of March)
- e the day number of the month (e.g. "1" on the first of March)
- B the month name long form (e.g. "January")
- b the month name short form (e.g. "Jan" for January)
- h the month name short form (e.g. "Jan" for January)
- A the weekday name long form (e.g. "Wednesday" for Wednesday)
- a the weekday name short form (e.g. "Wed" for Wednesday)
- j the day of the year number to three digits (e.g. "001" for 1 Jan)
- V the ISO week of the year number to two digits (e.g. "01" for ISO Week 1)
- u the day of the week number (e.g. "1" for Monday)
The following date componants are NOT supported:
- C the 'century' portion of the year (e.g. "19" for 1984, "5" for 584, "" for 84)
- G the year number in long form of the ISO week of the year (e.g. "2004" for 1 Jan 2005)
- g the year number in short form of the ISO week of the year (e.g. "04" for 1 Jan 2005)
- D the US short date format (e.g. "%m/%d/%y")
- F the ISO short date format (e.g. "%Y-%m-%d")
- x the KDE locale short date format
- %% the literal "%"
- t a tab character
- Parameters
-
dateString the string to convert dateFormat the date format to use ok if non-null, will be set to true
if the date is valid,false
if invalidformatStandard the standard the date format uses
- Returns
- the string converted to a QDate
- See also
- formatDate
- KLocale::readDate
Definition at line 2378 of file kcalendarsystem.cpp.
Changes the date's year, month and day.
The range of the year, month and day depends on which calendar is being used. All years entered are treated literally, i.e. no Y2K translation is applied to years entered in the range 00 to 99. Replaces setYMD.
- Parameters
-
date date to change year year month month number day day of month
- Returns
true
if the date is valid,false
otherwise
Definition at line 1222 of file kcalendarsystem.cpp.
- Since
- 4.4
Set a date using the year number and day of year number only.
- Parameters
-
date date to change year year dayOfYear day of year
- Returns
true
if the date is valid,false
otherwise
Definition at line 1243 of file kcalendarsystem.cpp.
bool KCalendarSystem::setDate | ( | QDate & | date, |
QString | eraName, | ||
int | yearInEra, | ||
int | month, | ||
int | day | ||
) | const |
- Since
- 4.5
Set a date using the era, year in era number, month and day
- Parameters
-
date date to change eraName Era string yearInEra Year In Era number month Month number day Day Of Month number
- Returns
true
if the date is valid,false
otherwise
Definition at line 1263 of file kcalendarsystem.cpp.
bool KCalendarSystem::setDateIsoWeek | ( | QDate & | date, |
int | year, | ||
int | isoWeekNumber, | ||
int | dayOfIsoWeek | ||
) | const |
- Since
- 4.4
Set a date using the year number, ISO week number and day of week number.
- Parameters
-
date date to change year year isoWeekNumber ISO week of year dayOfIsoWeek day of week Mon..Sun (1..7)
- Returns
true
if the date is valid,false
otherwise
Definition at line 1272 of file kcalendarsystem.cpp.
|
protected |
- Deprecated:
- for internal use only
- Since
- 4.4
Sets if Calendar System has Year 0 or not
Only for internal calendar system use
Definition at line 2499 of file kcalendarsystem.cpp.
|
protected |
- Deprecated:
- for internal use only
Sets the maximum number of days in a week
Only for internal calendar system use
Definition at line 2493 of file kcalendarsystem.cpp.
|
protected |
- Deprecated:
- for internal use only
Sets the maximum number of months in a year
Only for internal calendar system use
Definition at line 2487 of file kcalendarsystem.cpp.
- Deprecated:
- Use setDate() instead
Some implementations reject year range 00 to 99, but extended date ranges now require these to be accepted. Equivalent in QDate is obsoleted.
Changes the date's year, month and day. The range of the year, month and day depends on which calendar is being used.
- Parameters
-
date Date to change y Year m Month number d Day of month
- Returns
- true if the date is valid; otherwise returns false.
Definition at line 1301 of file kcalendarsystem.cpp.
int KCalendarSystem::shortYearWindowStartYear | ( | ) | const |
- Since
- 4.6
Returns the Short Year Window Start Year for the current Calendar System.
Use this function to get the Start Year for the Short Year Window to be applied when 2 digit years are entered for a Short Year input format, e.g. if the Short Year Window Start Year is 1930, then the input Short Year value of 40 is interpreted as 1940 and the input Short Year value of 10 is interpreted as 2010.
The Short Year Window is only ever applied when reading the Short Year format and not the Long Year format, i.e. KLocale::ShortFormat or 'y' only and not KLocale::LongFormat or 'Y'.
The Start Year 0 effectively means not to use a Short Year Window
Each Calendar System requires a different Short Year Window as they have different epochs. The Gregorian Short Year Window usually pivots around the year 2000, whereas the Hebrew Short Year Window usually pivots around the year 5000.
This value must always be used when evaluating user input Short Year strings.
- See also
- KLocale::shortYearWindowStartYear
- KLocale::applyShortYearWindow
- Returns
- the short year window start year
Definition at line 2390 of file kcalendarsystem.cpp.
int KCalendarSystem::week | ( | const QDate & | date, |
int * | yearNum = 0 |
||
) | const |
Returns the localized Week Number for the date.
This may be ISO, US, or any other supported week numbering scheme. If you specifically require the ISO Week or any other scheme, you should use the week(KLocale::WeekNumberSystem) form.
If the date falls in the last week of the previous year or the first week of the following year, then the yearNum returned will be set to the appropriate year.
- See also
- weeksInYear()
- formatDate()
- Parameters
-
date the date to obtain week from yearNum returns the year the date belongs to
- Returns
- localized week number, -1 if input date invalid
Definition at line 1703 of file kcalendarsystem.cpp.
int KCalendarSystem::week | ( | const QDate & | date, |
KLocale::WeekNumberSystem | weekNumberSystem, | ||
int * | yearNum = 0 |
||
) | const |
Returns the Week Number for the date in the required Week Number System.
Unless you want a specific Week Number System (e.g. ISO Week), you should use the localized Week Number form of week().
If the date falls in the last week of the previous year or the first week of the following year, then the yearNum returned will be set to the appropriate year.
Technically, the ISO Week Number only applies to the ISO/Gregorian Calendar System, but the same rules will be applied to the current Calendar System.
- See also
- weeksInYear()
- formatDate()
- Parameters
-
date the date to obtain week from weekNumberSystem the Week Number System to use yearNum returns the year the date belongs to
- Returns
- week number, -1 if input date invalid
Definition at line 1709 of file kcalendarsystem.cpp.
|
pure virtual |
Gets specific calendar type week day name.
If an invalid week day is specified, QString() is returned.
- Parameters
-
weekDay number of day in week (Monday = 1, ..., Sunday = 7) format specifies whether the short month name or long month name should be used
- Returns
- day name, empty string if any error
Implemented in KCalendarSystemQDate, KCalendarSystemHebrew, KCalendarSystemIslamicCivil, KCalendarSystemGregorian, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemEthiopian, KCalendarSystemJapanese, KCalendarSystemMinguo, KCalendarSystemCoptic, and KCalendarSystemJalali.
Definition at line 1881 of file kcalendarsystem.cpp.
|
virtual |
Gets specific calendar type week day name.
- Parameters
-
date the date format specifies whether the short month name or long month name should be used
- Returns
- day name, empty string if any error
Reimplemented in KCalendarSystemQDate, KCalendarSystemHebrew, KCalendarSystemIslamicCivil, KCalendarSystemGregorian, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemEthiopian, KCalendarSystemJapanese, KCalendarSystemMinguo, KCalendarSystemCoptic, and KCalendarSystemJalali.
Definition at line 1900 of file kcalendarsystem.cpp.
|
pure virtual |
- Deprecated:
- use KLocale::weekDayOfPray() instead
Returns the day of the week traditionally associated with religious observance for this calendar system. Note this may not be accurate for the users locale, e.g. Gregorian calendar used in non-Christian countries, in use cases where this could be an issue it is recommended to use KLocale::weekDayOfPray() instead.
- Returns
- day number (None = 0, Monday = 1, ..., Sunday = 7)
Implemented in KCalendarSystemQDate, KCalendarSystemHebrew, KCalendarSystemGregorian, KCalendarSystemIslamicCivil, KCalendarSystemIndianNational, KCalendarSystemJulian, KCalendarSystemJapanese, KCalendarSystemEthiopian, KCalendarSystemMinguo, KCalendarSystemCoptic, KCalendarSystemJalali, and KCalendarSystemThai.
|
virtual |
- Deprecated:
- use week() instead
Returns the ISO week number for the given date.
ISO 8601 defines the first week of the year as the week containing the first Thursday. See http://en.wikipedia.org/wiki/ISO_8601 and http://en.wikipedia.org/wiki/ISO_week_date
If the date falls in the last week of the previous year or the first week of the following year, then the yearNum returned will be set to the appropriate year.
- Parameters
-
date the date to obtain week from yearNum returns the year the date belongs to
- Returns
- ISO week number, -1 if input date invalid
Definition at line 1697 of file kcalendarsystem.cpp.
QString KCalendarSystem::weekNumberString | ( | const QDate & | pDate, |
StringFormat | format = LongFormat |
||
) | const |
- Deprecated:
- use formatDate(QDate, KLocale::DateTimeComponent, KLocale::DateTimeComponentFormat)
- Since
- 4.4
Converts a date into a week number literal
- Parameters
-
pDate The date to convert format The format to return, either short or long
- Returns
- The day literal of the date, empty string if any error
- See also
- weekNumber()
Definition at line 1963 of file kcalendarsystem.cpp.
|
virtual |
Returns the number of localized weeks in the given year.
- Parameters
-
date the date to obtain year from
- Returns
- number of weeks in the year, -1 if input date invalid
Definition at line 1586 of file kcalendarsystem.cpp.
int KCalendarSystem::weeksInYear | ( | const QDate & | date, |
KLocale::WeekNumberSystem | weekNumberSystem | ||
) | const |
- Since
- 4.7
Returns the number of Weeks in a year using the required Week Number System.
Unless you specifically want a particular Week Number System (e.g. ISO Weeks) you should use the localized number of weeks provided by weeksInYear().
- See also
- week()
- formatDate()
- Parameters
-
date the date to obtain year from weekNumberSystem the week number system to use
- Returns
- number of weeks in the year, -1 if date invalid
Definition at line 1597 of file kcalendarsystem.cpp.
|
virtual |
Returns the number of localized weeks in the given year.
- Parameters
-
year the year
- Returns
- number of weeks in the year, -1 if input date invalid
Definition at line 1591 of file kcalendarsystem.cpp.
int KCalendarSystem::weeksInYear | ( | int | year, |
KLocale::WeekNumberSystem | weekNumberSystem | ||
) | const |
- Since
- 4.7
Returns the number of Weeks in a year using the required Week Number System.
Unless you specifically want a particular Week Number System (e.g. ISO Weeks) you should use the localized number of weeks provided by weeksInYear().
- See also
- week()
- formatDate()
- Parameters
-
year the year weekNumberSystem the week number system to use
- Returns
- number of weeks in the year, -1 if date invalid
Definition at line 1609 of file kcalendarsystem.cpp.
QString KCalendarSystem::weeksInYearString | ( | const QDate & | pDate, |
StringFormat | format = LongFormat |
||
) | const |
- Deprecated:
- use formatDate(QDate, KLocale::DateTimeComponent, KLocale::DateTimeComponentFormat)
- Since
- 4.4
Returns the weeks in year for a date as a numeric string
- Parameters
-
pDate The date to convert format The format to return, either short or long
- Returns
- The weeks in year literal of the date, empty string if any error
- See also
- weeksInYear()
Definition at line 1983 of file kcalendarsystem.cpp.
|
virtual |
Use this to determine which day is the first day of the week.
Uses the calendar system's internal locale set when the instance was created, which ensures that the correct calendar system and locale settings are respected, which would not occur in some cases if using the global locale. Defaults to global locale.
- See also
- KLocale::weekStartDay
- Returns
- an integer (Monday = 1, ..., Sunday = 7)
Definition at line 2405 of file kcalendarsystem.cpp.
|
virtual |
Returns the year portion of a given date in the current calendar system.
- Parameters
-
date date to return year for
- Returns
- year, 0 if input date is invalid
Reimplemented in KCalendarSystemQDate.
Definition at line 1331 of file kcalendarsystem.cpp.
int KCalendarSystem::yearInEra | ( | const QDate & | date | ) | const |
- Since
- 4.5
Returns the Year In Era portion of a given date in the current calendar system, for example 1 for "1 BC".
- Parameters
-
date date to return Year In Era for
- Returns
- Year In Era, -1 if input date is invalid
Definition at line 1400 of file kcalendarsystem.cpp.
QString KCalendarSystem::yearInEraString | ( | const QDate & | date, |
StringFormat | format = ShortFormat |
||
) | const |
- Deprecated:
- use formatDate(QDate, KLocale::DateTimeComponent, KLocale::DateTimeComponentFormat)
- Since
- 4.5
Converts a date into a Year In Era literal
- Parameters
-
date date to return Year In Era for format format to return, either short or long
- Returns
- Year In Era literal of the date, empty string if any error
Definition at line 1937 of file kcalendarsystem.cpp.
Returns the difference between two dates in completed calendar years.
The returned value will be negative if fromDate
> toDate
.
For example, the difference between 2010-06-10 and 2012-09-5 is 2 years.
- Parameters
-
fromDate The date to start from toDate The date to end at
- Returns
- The number of years difference
Definition at line 1530 of file kcalendarsystem.cpp.
|
virtual |
- Deprecated:
- use formatDate(QDate, KLocale::DateTimeComponent, KLocale::DateTimeComponentFormat)
Converts a date into a year literal
- Parameters
-
date date to convert format format to return, either short or long
- Returns
- year literal of the date, empty string if any error
- See also
- year()
Definition at line 1909 of file kcalendarsystem.cpp.
|
virtual |
- Deprecated:
- for internal use only
Converts a year literal of a part of a string into a integer starting at the beginning of the string
- Parameters
-
sNum The string to parse iLength The number of QChars used, and 0 if no valid symbols was found in the string
- Returns
- An integer corresponding to the year
Reimplemented in KCalendarSystemHebrew, KCalendarSystemGregorian, and KCalendarSystemJapanese.
Definition at line 2018 of file kcalendarsystem.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:12 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.