KDECore
#include <KDesktopFile>
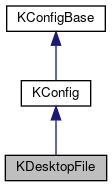
Public Member Functions | |
KDesktopFile (const char *resourceType, const QString &fileName) | |
KDesktopFile (const QString &fileName) | |
virtual | ~KDesktopFile () |
KConfigGroup | actionGroup (const QString &group) |
const KConfigGroup | actionGroup (const QString &group) const |
KDesktopFile * | copyTo (const QString &file) const |
KConfigGroup | desktopGroup () const |
QString | fileName () const |
bool | hasActionGroup (const QString &group) const |
bool | hasApplicationType () const |
bool | hasDeviceType () const |
bool | hasLinkType () const |
bool | hasMimeTypeType () const |
bool | noDisplay () const |
QStringList | readActions () const |
QString | readComment () const |
QString | readDevice () const |
QString | readDocPath () const |
QString | readGenericName () const |
QString | readIcon () const |
QString | readName () const |
QString | readPath () const |
QString | readType () const |
QString | readUrl () const |
const char * | resource () const |
QStringList | sortOrder () const |
bool | tryExec () const |
![]() | |
KConfig (const QString &file=QString(), OpenFlags mode=FullConfig, const char *resourceType="config") | |
KConfig (const KComponentData &componentData, const QString &file=QString(), OpenFlags mode=FullConfig, const char *resourceType="config") | |
KConfig (const QString &file, const QString &backend, const char *resourceType="config") | |
virtual | ~KConfig () |
void | checkUpdate (const QString &id, const QString &updateFile) |
const KComponentData & | componentData () const |
KConfig * | copyTo (const QString &file, KConfig *config=0) const |
QMap< QString, QString > | entryMap (const QString &aGroup=QString()) const |
QStringList | groupList () const |
bool | isDirty () const |
void | markAsClean () |
QString | name () const |
void | reparseConfiguration () |
void | sync () |
AccessMode | accessMode () const |
bool | isConfigWritable (bool warnUser) |
void | addConfigSources (const QStringList &sources) |
QString | locale () const |
bool | setLocale (const QString &aLocale) |
void | setReadDefaults (bool b) |
bool | readDefaults () const |
bool | isImmutable () const |
void | setForceGlobal (bool force) |
bool | forceGlobal () const |
![]() | |
virtual | ~KConfigBase () |
void | deleteGroup (const QByteArray &group, WriteConfigFlags flags=Normal) |
void | deleteGroup (const QString &group, WriteConfigFlags flags=Normal) |
void | deleteGroup (const char *group, WriteConfigFlags flags=Normal) |
KConfigGroup | group (const QByteArray &group) |
KConfigGroup | group (const QString &group) |
KConfigGroup | group (const char *group) |
const KConfigGroup | group (const QByteArray &group) const |
const KConfigGroup | group (const QString &group) const |
const KConfigGroup | group (const char *group) const |
bool | hasGroup (const QString &group) const |
bool | hasGroup (const char *group) const |
bool | hasGroup (const QByteArray &group) const |
bool | isGroupImmutable (const QByteArray &aGroup) const |
bool | isGroupImmutable (const QString &aGroup) const |
bool | isGroupImmutable (const char *aGroup) const |
Static Public Member Functions | |
static bool | isAuthorizedDesktopFile (const QString &path) |
static bool | isDesktopFile (const QString &path) |
static QString | locateLocal (const QString &path) |
Additional Inherited Members | |
![]() | |
enum | OpenFlag { IncludeGlobals = 0x01, CascadeConfig = 0x02, SimpleConfig = 0x00, NoCascade = IncludeGlobals, NoGlobals = CascadeConfig, FullConfig = IncludeGlobals|CascadeConfig } |
![]() | |
enum | AccessMode { NoAccess, ReadOnly, ReadWrite } |
enum | WriteConfigFlag { Persistent = 0x01, Global = 0x02, Localized = 0x04, Normal =Persistent } |
![]() | |
KConfig (KConfigPrivate &d) | |
virtual void | deleteGroupImpl (const QByteArray &group, WriteConfigFlags flags=Normal) |
virtual KConfigGroup | groupImpl (const QByteArray &b) |
virtual const KConfigGroup | groupImpl (const QByteArray &b) const |
virtual bool | hasGroupImpl (const QByteArray &group) const |
virtual bool | isGroupImmutableImpl (const QByteArray &aGroup) const |
virtual void | virtual_hook (int id, void *data) |
![]() | |
KConfigBase () | |
![]() | |
KConfigPrivate *const | d_ptr |
Detailed Description
KDE Desktop File Management.
This class implements KDE's support for the freedesktop.org Desktop Entry Spec.
- See also
- KConfigBase KConfig
- Desktop Entry Spec
Definition at line 38 of file kdesktopfile.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a KDesktopFile object.
See KStandardDirs for more information on resources.
- Parameters
-
resourceType Allows you to change what sort of resource to search for if fileName
is not absolute. For instance, you might want to specify "config".fileName The name or path of the desktop file. If it is not absolute, it will be located using the resource type resType
.
Definition at line 53 of file kdesktopfile.cpp.
|
explicit |
Constructs a KDesktopFile object.
See KStandardDirs for more information on resources.
- Parameters
-
fileName The name or path of the desktop file. If it is not absolute, it will be located using the resource type "apps"
Definition at line 61 of file kdesktopfile.cpp.
|
virtual |
Destructs the KDesktopFile object.
Writes back any dirty configuration entries.
Definition at line 69 of file kdesktopfile.cpp.
Member Function Documentation
KConfigGroup KDesktopFile::actionGroup | ( | const QString & | group | ) |
Sets the desktop action group.
- Parameters
-
group the new action group
Definition at line 247 of file kdesktopfile.cpp.
const KConfigGroup KDesktopFile::actionGroup | ( | const QString & | group | ) | const |
Definition at line 252 of file kdesktopfile.cpp.
KDesktopFile * KDesktopFile::copyTo | ( | const QString & | file | ) | const |
Copies all entries from this config object to a new KDesktopFile object that will save itself to file
.
Actual saving to file
happens when the returned object is destructed or when sync() is called upon it.
- Parameters
-
file the new KDesktopFile object it will save itself to.
Definition at line 372 of file kdesktopfile.cpp.
KConfigGroup KDesktopFile::desktopGroup | ( | ) | const |
Definition at line 73 of file kdesktopfile.cpp.
QString KDesktopFile::fileName | ( | ) | const |
Definition at line 386 of file kdesktopfile.cpp.
Returns true if the action group exists, false otherwise.
- Parameters
-
group the action group to test
- Returns
- true if the action group exists
Definition at line 257 of file kdesktopfile.cpp.
bool KDesktopFile::hasApplicationType | ( | ) | const |
Checks whether there is an entry "Type=Application".
- Returns
- true if there is a "Type=Application" entry
Definition at line 267 of file kdesktopfile.cpp.
bool KDesktopFile::hasDeviceType | ( | ) | const |
Checks whether there is an entry "Type=FSDevice".
- Returns
- true if there is a "Type=FSDevice" entry
Definition at line 277 of file kdesktopfile.cpp.
bool KDesktopFile::hasLinkType | ( | ) | const |
Checks whether there is a "Type=Link" entry.
The link points to the "URL=" entry.
- Returns
- true if there is a "Type=Link" entry
Definition at line 262 of file kdesktopfile.cpp.
bool KDesktopFile::hasMimeTypeType | ( | ) | const |
Checks whether there is an entry "Type=MimeType".
- Returns
- true if there is a "Type=MimeType" entry
Definition at line 272 of file kdesktopfile.cpp.
Checks whether the user is authorized to run this desktop file.
By default users are authorized to run all desktop files but the KIOSK framework can be used to activate certain restrictions. See README.kiosk for more information.
Note: Since KDE 4.3, there are more restrictions on authorized desktop files to prevent users from inadvertently running trojan desktop files. Your application launchers should have the executable bit set to prevent issues. To see if a restriction is due to KIOSK, see KAuthorized.
- Parameters
-
path the file to check
- Returns
- true if the user is authorized to run the file
Definition at line 137 of file kdesktopfile.cpp.
Checks whether this is really a desktop file.
The check is performed looking at the file extension (the file is not opened). Currently, the only valid extension is ".desktop".
- Parameters
-
path the path of the file to check
- Returns
- true if the file appears to be a desktop file.
Definition at line 131 of file kdesktopfile.cpp.
Returns the location where changes for the .desktop file path
should be written to.
Definition at line 79 of file kdesktopfile.cpp.
bool KDesktopFile::noDisplay | ( | ) | const |
Whether the entry should be suppressed in menus.
This handles the NoDisplay key, but also OnlyShowIn / NotShowIn.
- Returns
- true to suppress this service
- Since
- 4.1
Definition at line 391 of file kdesktopfile.cpp.
QStringList KDesktopFile::readActions | ( | ) | const |
Returns a list of the "Actions=" entries.
- Returns
- the list of actions
Definition at line 241 of file kdesktopfile.cpp.
QString KDesktopFile::readComment | ( | ) | const |
Returns the value of the "Comment=" entry.
- Returns
- the comment or QString() if not specified
Definition at line 198 of file kdesktopfile.cpp.
QString KDesktopFile::readDevice | ( | ) | const |
Returns the value of the "Dev=" entry.
- Returns
- the device or QString() if not specified
Definition at line 218 of file kdesktopfile.cpp.
QString KDesktopFile::readDocPath | ( | ) | const |
Returns the value of the "X-DocPath=" Or "DocPath=" entry.
- Returns
- The value of the "X-DocPath=" Or "DocPath=" entry.
Definition at line 363 of file kdesktopfile.cpp.
QString KDesktopFile::readGenericName | ( | ) | const |
Returns the value of the "GenericName=" entry.
- Returns
- the generic name or QString() if not specified
Definition at line 204 of file kdesktopfile.cpp.
QString KDesktopFile::readIcon | ( | ) | const |
Returns the value of the "Icon=" entry.
- Returns
- the icon or QString() if not specified
Definition at line 186 of file kdesktopfile.cpp.
QString KDesktopFile::readName | ( | ) | const |
Returns the value of the "Name=" entry.
- Returns
- the name or QString() if not specified
Definition at line 192 of file kdesktopfile.cpp.
QString KDesktopFile::readPath | ( | ) | const |
Returns the value of the "Path=" entry.
- Returns
- the path or QString() if not specified
Definition at line 210 of file kdesktopfile.cpp.
QString KDesktopFile::readType | ( | ) | const |
Returns the value of the "Type=" entry.
- Returns
- the type or QString() if not specified
Definition at line 180 of file kdesktopfile.cpp.
QString KDesktopFile::readUrl | ( | ) | const |
Returns the value of the "URL=" entry.
- Returns
- the URL or QString() if not specified
Definition at line 224 of file kdesktopfile.cpp.
const char * KDesktopFile::resource | ( | ) | const |
Definition at line 380 of file kdesktopfile.cpp.
QStringList KDesktopFile::sortOrder | ( | ) | const |
Returns the entry of the "SortOrder=" entry.
- Returns
- the value of the "SortOrder=" entry.
- the filename as passed to the constructor.
- the resource type as passed to the constructor.
Definition at line 354 of file kdesktopfile.cpp.
bool KDesktopFile::tryExec | ( | ) | const |
Checks whether the TryExec field contains a binary which is found on the local system.
- Returns
- true if TryExec contains an existing binary
Definition at line 282 of file kdesktopfile.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.