KDECore
#include <k3httproxysocketdevice.h>
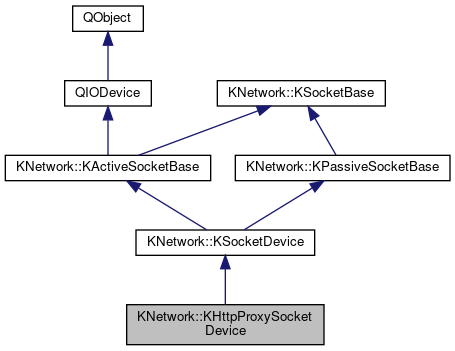
Public Member Functions | |
KHttpProxySocketDevice (const KSocketBase *=0L) | |
KHttpProxySocketDevice (const KResolverEntry &proxy) | |
virtual | ~KHttpProxySocketDevice () |
virtual int | capabilities () const |
virtual void | close () |
virtual bool | connect (const KResolverEntry &address) |
virtual bool | connect (const QString &name, const QString &service) |
virtual KSocketAddress | externalAddress () const |
virtual KSocketAddress | peerAddress () const |
const KResolverEntry & | proxyServer () const |
void | setProxyServer (const KResolverEntry &proxy) |
![]() | |
KSocketDevice (const KSocketBase *=0L, QObject *objparent=0L) | |
KSocketDevice (int fd, OpenMode mode=ReadWrite) | |
KSocketDevice (QObject *parent) | |
virtual | ~KSocketDevice () |
virtual KSocketDevice * | accept () |
virtual bool | bind (const KResolverEntry &address) |
virtual qint64 | bytesAvailable () const |
virtual bool | connect (const KResolverEntry &address, OpenMode mode=ReadWrite) |
virtual bool | create (int family, int type, int protocol) |
bool | create (const KResolverEntry &address) |
virtual bool | disconnect () |
QSocketNotifier * | exceptionNotifier () const |
virtual bool | flush () |
virtual bool | listen (int backlog=5) |
virtual KSocketAddress | localAddress () const |
virtual qint64 | peekData (char *data, qint64 maxlen, KSocketAddress *from=0L) |
virtual bool | poll (bool *input, bool *output, bool *exception=0L, int timeout=-1, bool *timedout=0L) |
bool | poll (int timeout=-1, bool *timedout=0L) |
virtual qint64 | readData (char *data, qint64 maxlen, KSocketAddress *from=0L) |
QSocketNotifier * | readNotifier () const |
virtual bool | setSocketOptions (int opts) |
int | socket () const |
virtual qint64 | waitForMore (int msecs, bool *timeout=0L) |
virtual qint64 | writeData (const char *data, qint64 len, const KSocketAddress *to=0L) |
QSocketNotifier * | writeNotifier () const |
![]() | |
KActiveSocketBase (QObject *parent) | |
virtual | ~KActiveSocketBase () |
virtual bool | atEnd () const |
QString | errorString () const |
virtual bool | isSequential () const |
virtual bool | open (OpenMode mode) |
qint64 | peek (char *data, qint64 maxlen) |
qint64 | peek (char *data, qint64 maxlen, KSocketAddress &from) |
virtual qint64 | pos () const |
qint64 | read (char *data, qint64 maxlen) |
QByteArray | read (qint64 len) |
qint64 | read (char *data, qint64 maxlen, KSocketAddress &from) |
virtual bool | seek (qint64) |
virtual void | setSocketDevice (KSocketDevice *device) |
virtual qint64 | size () const |
void | ungetChar (char) |
qint64 | write (const char *data, qint64 len) |
qint64 | write (const QByteArray &data) |
qint64 | write (const char *data, qint64 len, const KSocketAddress &to) |
![]() | |
QIODevice () | |
QIODevice (QObject *parent) | |
virtual | ~QIODevice () |
void | aboutToClose () |
Offset | at () const |
bool | at (Offset offset) |
virtual qint64 | bytesToWrite () const |
void | bytesWritten (qint64 bytes) |
virtual bool | canReadLine () const |
QString | errorString () const |
int | flags () const |
int | getch () |
bool | getChar (char *c) |
bool | isAsynchronous () const |
bool | isBuffered () const |
bool | isCombinedAccess () const |
bool | isDirectAccess () const |
bool | isInactive () const |
bool | isOpen () const |
bool | isRaw () const |
bool | isReadable () const |
bool | isSequentialAccess () const |
bool | isSynchronous () const |
bool | isTextModeEnabled () const |
bool | isTranslated () const |
bool | isWritable () const |
int | mode () const |
virtual bool | open (QFlags< QIODevice::OpenModeFlag > mode) |
OpenMode | openMode () const |
qint64 | peek (char *data, qint64 maxSize) |
QByteArray | peek (qint64 maxSize) |
int | putch (int ch) |
bool | putChar (char c) |
qint64 | read (char *data, qint64 maxSize) |
QByteArray | read (qint64 maxSize) |
QByteArray | readAll () |
qint64 | readBlock (char *data, quint64 size) |
void | readChannelFinished () |
QByteArray | readLine (qint64 maxSize) |
qint64 | readLine (char *data, qint64 maxSize) |
void | readyRead () |
virtual bool | reset () |
void | resetStatus () |
void | setTextModeEnabled (bool enabled) |
int | state () const |
Status | status () const |
int | ungetch (int ch) |
void | ungetChar (char c) |
virtual bool | waitForBytesWritten (int msecs) |
virtual bool | waitForReadyRead (int msecs) |
qint64 | write (const char *data) |
qint64 | write (const char *data, qint64 maxSize) |
qint64 | write (const QByteArray &byteArray) |
qint64 | writeBlock (const QByteArray &data) |
qint64 | writeBlock (const char *data, quint64 size) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
![]() | |
KSocketBase () | |
virtual | ~KSocketBase () |
bool | addressReuseable () const |
bool | blocking () const |
bool | broadcast () const |
SocketError | error () const |
QString | errorString () const |
bool | isIPv6Only () const |
QMutex * | mutex () const |
bool | noDelay () const |
virtual bool | setAddressReuseable (bool enable) |
virtual bool | setBlocking (bool enable) |
virtual bool | setBroadcast (bool enable) |
virtual bool | setIPv6Only (bool enable) |
virtual bool | setNoDelay (bool enable) |
int | setRequestedCapabilities (int add, int remove=0) |
KSocketDevice * | socketDevice () const |
![]() | |
KPassiveSocketBase () | |
virtual | ~KPassiveSocketBase () |
Static Public Attributes | |
static KResolverEntry | defaultProxy |
Additional Inherited Members | |
![]() | |
enum | Capabilities { CanConnectString = 0x01, CanBindString = 0x02, CanNotBind = 0x04, CanNotListen = 0x08, CanMulticast = 0x10, CanNotUseDatagrams = 0x20 } |
![]() | |
enum | SocketError { NoError = 0, LookupFailure, AddressInUse, AlreadyCreated, AlreadyBound, AlreadyConnected, NotConnected, NotBound, NotCreated, WouldBlock, ConnectionRefused, ConnectionTimedOut, InProgress, NetFailure, NotSupported, Timeout, UnknownError, RemotelyDisconnected } |
enum | SocketOptions { Blocking = 0x01, AddressReuseable = 0x02, IPv6Only = 0x04, Keepalive = 0x08, Broadcast = 0x10, NoDelay = 0x20 } |
![]() | |
static void | addNewImpl (KSocketDeviceFactoryBase *factory, int capabilities) |
static KSocketDevice * | createDefault (KSocketBase *parent) |
static KSocketDevice * | createDefault (KSocketBase *parent, int capabilities) |
static KSocketDeviceFactoryBase * | setDefaultImpl (KSocketDeviceFactoryBase *factory) |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
static QString | errorString (SocketError code) |
static bool | isFatalError (int code) |
![]() | |
typedef | Offset |
typedef | OpenMode |
typedef | Status |
![]() | |
KSocketDevice (bool, const KSocketBase *parent=0L) | |
virtual QSocketNotifier * | createNotifier (QSocketNotifier::Type type) const |
![]() | |
virtual qint64 | readData (char *data, qint64 len) |
void | resetError () |
void | setError (SocketError error) |
virtual qint64 | writeData (const char *data, qint64 len) |
![]() | |
virtual qint64 | readData (char *data, qint64 maxSize)=0 |
virtual qint64 | readLineData (char *data, qint64 maxSize) |
void | setErrorString (const QString &str) |
void | setOpenMode (QFlags< QIODevice::OpenModeFlag > openMode) |
virtual qint64 | writeData (const char *data, qint64 maxSize)=0 |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
bool | hasDevice () const |
void | resetError () |
void | setError (SocketError error) |
virtual int | socketOptions () const |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
int | m_sockfd |
![]() | |
objectName | |
Detailed Description
The low-level backend for HTTP proxying.
This class derives from KSocketDevice and implements the necessary calls to make a connection through an HTTP proxy.
- Deprecated:
- Use KSocketFactory or KLocalSocket instead
Definition at line 44 of file k3httpproxysocketdevice.h.
Constructor & Destructor Documentation
KHttpProxySocketDevice::KHttpProxySocketDevice | ( | const KSocketBase * | parent = 0L | ) |
Constructor.
Definition at line 57 of file k3httpproxysocketdevice.cpp.
|
explicit |
Constructor with proxy server's address.
Definition at line 62 of file k3httpproxysocketdevice.cpp.
|
virtual |
Destructor.
Definition at line 68 of file k3httpproxysocketdevice.cpp.
Member Function Documentation
|
virtual |
Sets our capabilities.
Reimplemented from KNetwork::KSocketDevice.
Definition at line 76 of file k3httpproxysocketdevice.cpp.
|
virtual |
Closes the socket.
Reimplemented from KNetwork::KSocketDevice.
Definition at line 92 of file k3httpproxysocketdevice.cpp.
|
virtual |
Overrides connection.
Definition at line 111 of file k3httpproxysocketdevice.cpp.
Name-based connection.
We can tell the HTTP proxy server the full name.
Definition at line 133 of file k3httpproxysocketdevice.cpp.
|
virtual |
Return the externally visible address.
We can't tell what that address is, so this function always returns an empty object.
Reimplemented from KNetwork::KSocketDevice.
Definition at line 106 of file k3httpproxysocketdevice.cpp.
|
virtual |
Return the peer address.
Reimplemented from KNetwork::KSocketDevice.
Definition at line 99 of file k3httpproxysocketdevice.cpp.
const KResolverEntry & KHttpProxySocketDevice::proxyServer | ( | ) | const |
Retrieves the proxy server address.
Definition at line 82 of file k3httpproxysocketdevice.cpp.
void KHttpProxySocketDevice::setProxyServer | ( | const KResolverEntry & | proxy | ) |
Sets the proxy server address.
Definition at line 87 of file k3httpproxysocketdevice.cpp.
Member Data Documentation
|
static |
This is the default proxy server to be used.
Applications may want to set this value so that calling setProxyServer() is unnecessary.
Definition at line 118 of file k3httpproxysocketdevice.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:14 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.