KDECore
#include <KPluginFactory>
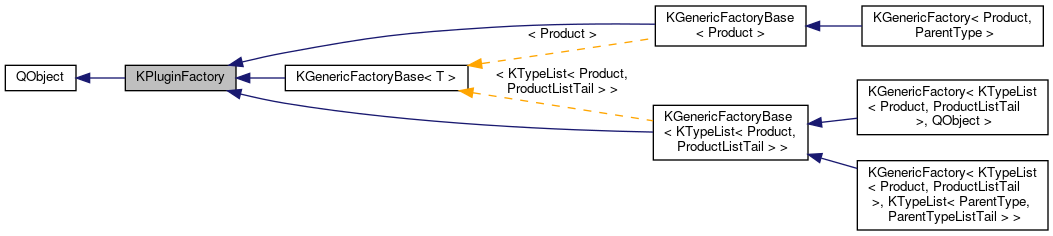
Classes | |
struct | InheritanceChecker |
Signals | |
void | objectCreated (QObject *object) |
Public Member Functions | |
KPluginFactory (const char *componentName=0, const char *catalogName=0, QObject *parent=0) | |
KPluginFactory (const KAboutData &aboutData, QObject *parent=0) | |
KDE_CONSTRUCTOR_DEPRECATED | KPluginFactory (const KAboutData *aboutData, QObject *parent=0) |
KDE_CONSTRUCTOR_DEPRECATED | KPluginFactory (QObject *parent) |
virtual | ~KPluginFactory () |
KComponentData | componentData () const |
template<typename T > | |
T * | create (QObject *parent=0, const QVariantList &args=QVariantList()) |
template<typename T > | |
T * | create (const QString &keyword, QObject *parent=0, const QVariantList &args=QVariantList()) |
template<typename T > | |
T * | create (QWidget *parentWidget, QObject *parent, const QString &keyword=QString(), const QVariantList &args=QVariantList()) |
template<typename T > | |
T * | create (QObject *parent, const QStringList &args) |
QObject * | create (QObject *parent=0, const char *classname="QObject", const QStringList &args=QStringList()) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Protected Types | |
typedef QObject *(* | CreateInstanceFunction )(QWidget *, QObject *, const QVariantList &) |
Protected Member Functions | |
KPluginFactory (KPluginFactoryPrivate &dd, QObject *parent=0) | |
virtual QObject * | create (const char *iface, QWidget *parentWidget, QObject *parent, const QVariantList &args, const QString &keyword) |
virtual QObject * | createObject (QObject *parent, const char *className, const QStringList &args) |
virtual KParts::Part * | createPartObject (QWidget *parentWidget, QObject *parent, const char *classname, const QStringList &args) |
template<class T > | |
void | registerPlugin (const QString &keyword=QString(), CreateInstanceFunction instanceFunction=InheritanceChecker< T >().createInstanceFunction(reinterpret_cast< T * >(0))) |
void | setComponentData (const KComponentData &componentData) |
virtual void | setupTranslations () |
QVariantList | stringListToVariantList (const QStringList &list) |
QStringList | variantListToStringList (const QVariantList &list) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Static Protected Member Functions | |
template<class impl , class ParentType > | |
static QObject * | createInstance (QWidget *parentWidget, QObject *parent, const QVariantList &args) |
template<class impl > | |
static QObject * | createPartInstance (QWidget *parentWidget, QObject *parent, const QVariantList &args) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
Protected Attributes | |
KPluginFactoryPrivate *const | d_ptr |
Related Functions | |
(Note that these are not member functions.) | |
#define | K_PLUGIN_FACTORY(name, pluginRegistrations) K_PLUGIN_FACTORY_WITH_BASEFACTORY(name, KPluginFactory, pluginRegistrations) |
#define | K_PLUGIN_FACTORY_DECLARATION(name) K_PLUGIN_FACTORY_DECLARATION_WITH_BASEFACTORY(name, KPluginFactory) |
#define | K_PLUGIN_FACTORY_DEFINITION(name, pluginRegistrations) K_PLUGIN_FACTORY_DEFINITION_WITH_BASEFACTORY(name, KPluginFactory, pluginRegistrations) |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
objectName | |
Detailed Description
If you develop a library that is to be loaded dynamically at runtime, then you should return a pointer to a KPluginFactory.
For most cases it is enough to use the K_PLUGIN_FACTORY macro to create the factory.
Example:
K_PLUGIN_FACTORY is a convenient macro that expands to a class derived from KPluginFactory providing two constructors and a static componentData() function. The second argument to K_PLUGIN_FACTORY is code that is called from the constructors. There you can use registerPlugin to register as many plugins for the factory as you want to.
If you want to write a custom KPluginFactory not using the standard macro(s) you can reimplement the create(const char *iface, QWidget *parentWidget, QObject *parent, const QVariantList &args, const QString &keyword) function.
Example:
If you want to load a library use KPluginLoader. The application that wants to instantiate plugin classes can do the following:
Definition at line 232 of file kpluginfactory.h.
Member Typedef Documentation
|
protected |
Function pointer type to a function that instantiates a plugin.
Definition at line 366 of file kpluginfactory.h.
Constructor & Destructor Documentation
|
explicit |
This constructor creates a factory for a plugin with the given componentName
and catalogName
.
Those values are used to initialize a KComponentData object for the plugin. You can later access it with componentData(). If componentName
is 0, an invalid KComponentData object will be created.
- Parameters
-
componentName the component name of the plugin catalogName the translation catalog to use parent a parent object
Definition at line 33 of file kpluginfactory.cpp.
|
explicit |
This constructor creates a factory for a plugin with the given KAboutData object.
This object is used to initialize a KComponentData object for the plugin. You can later access it with componentData(). KPluginFactory takes ownership of the aboutData
object, so don't delete it yourself!
- Parameters
-
aboutData the KAboutData for the plugin parent a parent object
Definition at line 57 of file kpluginfactory.cpp.
|
explicit |
Definition at line 46 of file kpluginfactory.cpp.
|
explicit |
Definition at line 68 of file kpluginfactory.cpp.
|
virtual |
This destroys the PluginFactory.
It will remove the translation catalog for the plugin, if it was initialized.
Definition at line 83 of file kpluginfactory.cpp.
|
explicitprotected |
Definition at line 77 of file kpluginfactory.cpp.
Member Function Documentation
KComponentData KPluginFactory::componentData | ( | ) | const |
You can use this method to get the component data of the plugin.
It is filled with the information given to the constructor of KPluginFactory. The K_PLUGIN_FACTORY macros provide a static version of this method, this can be used from any place within the plugin.
Only use this method if you specified a componentData name or instance to the factory constructor or to setComponentData. Otherwise you get an invalid KComponentData, which will crash if used.
- Returns
- The KComponentData for the plugin
Definition at line 94 of file kpluginfactory.cpp.
|
inline |
Use this method to create an object.
It will try to create an object which inherits T
. If it has multiple choices, you will get a fatal error (kFatal()), so be careful to request a unique interface or use keywords.
- Template Parameters
-
T The interface for which an object should be created. The object will inherit T
.
- Parameters
-
parent The parent of the object. If parent
is a widget type, it will also passed to the parentWidget argument of the CreateInstanceFunction for the object.args Additional arguments which will be passed to the object.
- Returns
- A pointer to the created object is returned, or 0 if an error occurred.
Definition at line 505 of file kpluginfactory.h.
|
inline |
Use this method to create an object.
It will try to create an object which inherits T
and was registered with keyword
.
- Template Parameters
-
T The interface for which an object should be created. The object will inherit T
.
- Parameters
-
keyword The keyword of the object. parent The parent of the object. If parent
is a widget type, it will also passed to the parentWidget argument of the CreateInstanceFunction for the object.args Additional arguments which will be passed to the object.
- Returns
- A pointer to the created object is returned, or 0 if an error occurred.
Definition at line 517 of file kpluginfactory.h.
|
inline |
Use this method to create an object.
It will try to create an object which inherits T
and was registered with keyword
. This overload has an additional parentWidget
argument, which is used by some plugins (e.g. Parts).
- Template Parameters
-
T The interface for which an object should be created. The object will inherit T
.
- Parameters
-
parentWidget An additional parent widget. parent The parent of the object. If parent
is a widget type, it will also passed to the parentWidget argument of the CreateInstanceFunction for the object.keyword The keyword of the object. args Additional arguments which will be passed to the object.
- Returns
- A pointer to the created object is returned, or 0 if an error occurred.
Definition at line 529 of file kpluginfactory.h.
|
inline |
Definition at line 343 of file kpluginfactory.h.
|
inline |
Definition at line 353 of file kpluginfactory.h.
|
protectedvirtual |
This function is called when the factory asked to create an Object.
You may reimplement it to provide a very flexible factory. This is especially useful to provide generic factories for plugins implemeted using a scripting language.
- Parameters
-
iface The staticMetaObject::className() string identifying the plugin interface that was requested. E.g. for KCModule plugins this string will be "KCModule". parentWidget Only used if the requested plugin is a KPart. parent The parent object for the plugin object. args A plugin specific list of arbitrary arguments. keyword A string that uniquely identifies the plugin. If a KService is used this keyword is read from the X-KDE-PluginKeyword entry in the .desktop file.
Definition at line 161 of file kpluginfactory.cpp.
|
inlinestaticprotected |
Definition at line 469 of file kpluginfactory.h.
|
protectedvirtual |
|
inlinestaticprotected |
Definition at line 481 of file kpluginfactory.h.
|
protectedvirtual |
Definition at line 151 of file kpluginfactory.cpp.
|
signal |
|
inlineprotected |
Registers a plugin with the factory.
Call this function from the constructor of the KPluginFactory subclass to make the create function able to instantiate the plugin when asked for an interface the plugin implements.
You can register as many plugin classes as you want as long as either the plugin interface or the keyword
makes it unique. E.g. it is possible to register a KCModule and a KParts::Part without having to specify keywords since their interfaces differ.
- Template Parameters
-
T the name of the plugin class
- Parameters
-
keyword An optional keyword as unique identifier for the plugin. This allows you to put more than one plugin with the same interface into the same library using the same factory. X-KDE-PluginKeyword is a convenient way to specify the keyword in a desktop file. instanceFunction A function pointer to a function that creates an instance of the plugin. The default function that will be used depends on the type of interface. If the interface inherits from KParts::Part
the function will callQWidget
the function will call- else the function will call
Definition at line 402 of file kpluginfactory.h.
|
protected |
This method sets the component data of the plugin.
You can access the component data object later with componentData(). Normally you don't have to call this, because the factory constructs a component data object from the information given to the constructor. The object is destroyed, when the module containing the plugin is unloaded. Normally this happens only on application shutdown.
- Parameters
-
componentData the new KComponentData object
Definition at line 225 of file kpluginfactory.cpp.
|
protectedvirtual |
Definition at line 215 of file kpluginfactory.cpp.
|
protected |
Converts a QStringList to a QVariantList
Definition at line 241 of file kpluginfactory.cpp.
|
protected |
Converts a QVariantList of strings to a QStringList
Definition at line 232 of file kpluginfactory.cpp.
Friends And Related Function Documentation
|
related |
Defines a KPluginFactory subclass with two constructors and a static componentData function.
The first constructor takes the arguments (const char *componentName, const char *catalogName, QObject *parent). The second constructor takes (const KAboutData *aboutData, QObject *parent).
The static componentData method returns the same KComponentData object as the KPluginFactory::componentData function returns. As you normally don't have a pointer to the factory object in the plugin code the static componentData function is a convenient way to access the KComponentData.
- Parameters
-
name The name of the KPluginFactory derived class. This is the name you'll need for K_EXPORT_PLUGIN pluginRegistrations This is code inserted into the constructors the class. You'll want to call registerPlugin from there.
Example:
Definition at line 127 of file kpluginfactory.h.
|
related |
K_PLUGIN_FACTORY_DECLARATION declares the KPluginFactory subclass. This macro can be used in a header file.
- Parameters
-
name The name of the KPluginFactory derived class. This is the name you'll need for K_EXPORT_PLUGIN
Definition at line 140 of file kpluginfactory.h.
|
related |
K_PLUGIN_FACTORY_DEFINITION defines the KPluginFactory subclass. This macro can not be used in a header file.
- Parameters
-
name The name of the KPluginFactory derived class. This is the name you'll need for K_EXPORT_PLUGIN pluginRegistrations This is code inserted into the constructors the class. You'll want to call registerPlugin from there.
Definition at line 156 of file kpluginfactory.h.
Member Data Documentation
|
protected |
Definition at line 422 of file kpluginfactory.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.