KDECore
#include <kservice.h>
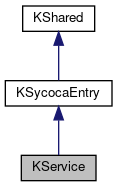
Classes | |
struct | ServiceTypeAndPreference |
Public Types | |
enum | DBusStartupType { DBusNone = 0, DBusUnique, DBusMulti, DBusWait } |
typedef QList< Ptr > | List |
typedef KSharedPtr< KService > | Ptr |
![]() | |
typedef QList< Ptr > | List |
typedef KSharedPtr< KSycocaEntry > | Ptr |
Static Public Member Functions | |
static List | allServices () |
template<class T > | |
static T * | createInstance (const KService::Ptr &service, QObject *parent=0, const QVariantList &args=QVariantList(), QString *error=0) |
template<class T > | |
static T * | createInstance (const KService::Ptr &service, QObject *parent, const QStringList &args, int *error=0) |
template<class T , class ServiceIterator > | |
static T * | createInstance (ServiceIterator begin, ServiceIterator end, QObject *parent=0, const QVariantList &args=QVariantList(), QString *error=0) |
template<class T , class ServiceIterator > | |
static T * | createInstance (ServiceIterator begin, ServiceIterator end, QObject *parent, const QStringList &args, int *error=0) |
static QString | newServicePath (bool showInMenu, const QString &suggestedName, QString *menuId=0, const QStringList *reservedMenuIds=0) |
static Ptr | serviceByDesktopName (const QString &_name) |
static Ptr | serviceByDesktopPath (const QString &_path) |
static Ptr | serviceByMenuId (const QString &_menuId) |
static Ptr | serviceByName (const QString &_name) |
static Ptr | serviceByStorageId (const QString &_storageId) |
![]() | |
static void | read (QDataStream &s, QString &str) |
static void | read (QDataStream &s, QStringList &list) |
Protected Member Functions | |
QVector < ServiceTypeAndPreference > & | _k_accessServiceTypes () |
![]() | |
KSycocaEntry (KSycocaEntryPrivate &d) | |
Friends | |
QDataStream & | operator<< (QDataStream &, const ServiceTypeAndPreference &) |
QDataStream & | operator>> (QDataStream &, ServiceTypeAndPreference &) |
Additional Inherited Members | |
![]() | |
KSycocaEntryPrivate * | d_ptr |
![]() | |
enum | KSycocaType |
Detailed Description
Represent a service, like an application or plugin bound to one or several mimetypes (or servicetypes) as written in its desktop entry file.
The starting point you need is often the static methods, like createInstance(). The types of service a plugin provides is taken from the accompanying desktop file where the 'ServiceTypes=' field is used.
For a tutorial on how to build a plugin-loading mechanism and how to write plugins in general, see http://techbase.kde.org/Development/Tutorials#Services:_Applications_and_Plugins
- See also
- KServiceType
- KServiceGroup
Definition at line 58 of file kservice.h.
Member Typedef Documentation
Definition at line 62 of file kservice.h.
Definition at line 61 of file kservice.h.
Member Enumeration Documentation
Describes the DBUS Startup type of the service.
- None - This service has no DBUS support
- Unique - This service provides a unique DBUS service. The service name is equal to the desktopEntryName.
- Multi - This service provides a DBUS service which can be run with multiple instances in parallel. The service name of an instance is equal to the desktopEntryName + "-" + the PID of the process.
- Wait - This service has no DBUS support, the launcher will wait till it is finished.
Enumerator | |
---|---|
DBusNone | |
DBusUnique | |
DBusMulti | |
DBusWait |
Definition at line 212 of file kservice.h.
Constructor & Destructor Documentation
Construct a temporary service with a given name, exec-line and icon.
- Parameters
-
name the name of the service exec the executable icon the name of the icon
Definition at line 373 of file kservice.cpp.
|
explicit |
Construct a service and take all information from a config file.
- Parameters
-
fullpath Full path to the config file.
Definition at line 387 of file kservice.cpp.
|
explicit |
Construct a service and take all information from a desktop file.
- Parameters
-
config the desktop file to read
Definition at line 396 of file kservice.cpp.
KService::KService | ( | QDataStream & | str, |
int | offset | ||
) |
Construct a service from a stream. The stream must already be positionned at the correct offset.
Definition at line 404 of file kservice.cpp.
|
virtual |
Definition at line 409 of file kservice.cpp.
Member Function Documentation
|
protected |
for KBuildSycoca only
Definition at line 989 of file kservice.cpp.
QList< KServiceAction > KService::actions | ( | ) | const |
Returns the actions defined in this desktop file.
Definition at line 995 of file kservice.cpp.
bool KService::allowAsDefault | ( | ) | const |
Set to true if it is allowed to use this service as the default (main) action for the files it supports (e.g.
Left Click in a file manager, or KRun in general).
If not, then this service is only available in RMB popups, so it must be selected explicitly by the user in order to be used. Note that servicemenus supersede this functionality though, at least in konqueror.
- Returns
- true if the service may be used as the default (main) handler
Definition at line 955 of file kservice.cpp.
bool KService::allowMultipleFiles | ( | ) | const |
Checks whether this service can handle several files as startup arguments.
- Returns
- true if multiple files may be passed to this service at startup. False if only one file at a time may be passed.
Definition at line 758 of file kservice.cpp.
|
static |
Returns the whole list of services.
Useful for being able to to display them in a list box, for example. More memory consuming than the ones above, don't use unless really necessary.
- Returns
- the list of all services
Definition at line 603 of file kservice.cpp.
QStringList KService::categories | ( | ) | const |
Returns a list of VFolder categories.
- Returns
- the list of VFolder categories
Definition at line 765 of file kservice.cpp.
QString KService::comment | ( | ) | const |
Returns the descriptive comment for the service, if there is one.
- Returns
- the descriptive comment for the service, or QString() if not set
Definition at line 907 of file kservice.cpp.
|
inline |
This template allows to load the library for the specified service and ask the factory to create an instance of the given template type.
- Parameters
-
parent The parent object (see QObject constructor) args A list of arguments, passed to the factory and possibly to the component (see KPluginFactory) error A pointer to QString which should receive the error description or 0
- Returns
- A pointer to the newly created object or a null pointer if the factory was unable to create an object of the given type.
Definition at line 547 of file kservice.h.
|
inline |
This template allows to load the library for the specified service and ask the factory to create an instance of the given template type.
- Parameters
-
parentWidget A parent widget for the service. This is used e. g. for parts parent The parent object (see QObject constructor) args A list of arguments, passed to the factory and possibly to the component (see KPluginFactory) error A pointer to QString which should receive the error description or 0
- Returns
- A pointer to the newly created object or a null pointer if the factory was unable to create an object of the given type.
Definition at line 567 of file kservice.h.
|
inlinestatic |
- Deprecated:
- Use the non-static service->createInstance<T>(parent, args, &error)
Definition at line 591 of file kservice.h.
|
inlinestatic |
- Deprecated:
- Use the non-static service->createInstance<T>(parent, args, &error) where args is a QVariantList rather than a QStringList
Definition at line 604 of file kservice.h.
|
inlinestatic |
This template allows to create a component from a list of services, usually coming from a trader query.
You probably want to use KServiceTypeTrader instead.
- Parameters
-
begin The start iterator to the service describing the library to open end The end iterator to the service describing the library to open parent The parent object (see QObject constructor) args A list of string arguments, passed to the factory and possibly to the component (see KLibFactory) error see KLibLoader
- Returns
- A pointer to the newly created object or a null pointer if the factory was unable to create an object of the given type.
Definition at line 637 of file kservice.h.
|
inlinestatic |
Definition at line 660 of file kservice.h.
KService::DBusStartupType KService::dbusStartupType | ( | ) | const |
Returns the DBUSStartupType supported by this service.
- Returns
- the DBUSStartupType supported by this service
Definition at line 895 of file kservice.cpp.
QString KService::desktopEntryName | ( | ) | const |
Returns the filename of the service desktop entry without any extension.
E.g. "kppp"
- Returns
- the name of the desktop entry without path or extension, or QString() if not set
Definition at line 889 of file kservice.cpp.
QString KService::desktopEntryPath | ( | ) | const |
Returns the path to the location where the service desktop entry is stored.
This is a relative path if the desktop entry was found in any of the locations pointed to by $KDEDIRS (e.g. "Internet/kppp.desktop") It is a full path if the desktop entry originates from another location.
- Deprecated:
- use entryPath() instead
- Returns
- the path of the service's desktop file, or QString() if not set
Definition at line 883 of file kservice.cpp.
QString KService::docPath | ( | ) | const |
The path to the documentation for this service.
- Since
- 4.2
- Returns
- the documentation path, or QString() if not set
Definition at line 744 of file kservice.cpp.
QString KService::exec | ( | ) | const |
Returns the executable.
- Returns
- the command that the service executes, or QString() if not set
Definition at line 846 of file kservice.cpp.
QString KService::genericName | ( | ) | const |
Returns the generic name for the service, if there is one (e.g.
"Mail Client").
- Returns
- the generic name, or QString() if not set
Definition at line 913 of file kservice.cpp.
bool KService::hasMimeType | ( | const KServiceType * | mimeTypePtr | ) | const |
Checks whether the service supports this mime type.
- Parameters
-
mimeTypePtr The name of the mime type you are interested in determining whether this service supports.
Note that if you only have the name of the mime type, you have to look it up with KMimeType::mimeType( mimetype ) and use .data() on the result (this is because KService doesn't know KMimeType for dependency reasons)
Warning this method will fail to return true if this KService isn't from ksycoca (i.e. it was created with a full path or a KDesktopFile) and the mimetype isn't explicited listed in the .desktop file but a parent mimetype is. For this reason you should generally get KServices with KMimeTypeTrader or one of the KService::serviceBy methods.
- Returns
- true if the mime type you specified is supported, otherwise false.
- Deprecated:
- , use hasMimeType(QString)
Definition at line 446 of file kservice.cpp.
Checks whether the service supports this mime type.
- Parameters
-
mimeType The name of the mime type you are interested in determining whether this service supports.
- Since
- 4.6
Definition at line 454 of file kservice.cpp.
Checks whether the service supports this service type.
- Parameters
-
serviceTypePtr The name of the service type you are interested in determining whether this service supports.
- Returns
- true if the service type you specified is supported, otherwise false.
Definition at line 413 of file kservice.cpp.
QString KService::icon | ( | ) | const |
Returns the name of the icon.
- Returns
- the icon associated with the service, or QString() if not set
Definition at line 863 of file kservice.cpp.
int KService::initialPreference | ( | ) | const |
What preference to associate with this service initially (before the user has had any chance to define a profile for it).
The bigger the value, the most preferred the service is.
- Returns
- the service preference level of the service
Definition at line 961 of file kservice.cpp.
bool KService::isApplication | ( | ) | const |
Services are either applications (executables) or dlopened libraries (plugins).
- Returns
- true if this service is an application, i.e. it has Type=Application in its .desktop file and exec() will not be empty.
Definition at line 832 of file kservice.cpp.
QStringList KService::keywords | ( | ) | const |
Returns a list of descriptive keywords the service, if there are any.
- Returns
- the list of keywords
Definition at line 919 of file kservice.cpp.
QString KService::library | ( | ) | const |
Returns the name of the service's library.
- Returns
- the name of the library that contains the service's implementation, or QString() if not set
Definition at line 857 of file kservice.cpp.
QString KService::locateLocal | ( | ) | const |
Returns a path that can be used for saving changes to this service.
- Returns
- path that can be used for saving changes to this service
Definition at line 789 of file kservice.cpp.
QString KService::menuId | ( | ) | const |
Returns the menu ID of the service desktop entry.
The menu ID is used to add or remove the entry to a menu.
- Returns
- the menu ID
Definition at line 771 of file kservice.cpp.
QStringList KService::mimeTypes | ( | ) | const |
Returns the list of mime types that this service supports.
Note that this doesn't include inherited mimetypes, only the mimetypes types listed in the .desktop file.
- Since
- 4.8.3
Definition at line 942 of file kservice.cpp.
|
static |
Returns a path that can be used to create a new KService based on suggestedName
.
- Parameters
-
showInMenu true, if the service should be shown in the KDE menu false, if the service should be hidden from the menu This argument isn't used anymore, use NoDisplay=true to hide the service. suggestedName name to base the file on, if a service with such name already exists, a prefix will be added to make it unique. menuId If provided, menuId will be set to the menu id to use for the KService reservedMenuIds If provided, the path and menu id will be chosen in such a way that the new menu id does not conflict with any of the reservedMenuIds
- Returns
- The path to use for the new KService.
Definition at line 799 of file kservice.cpp.
bool KService::noDisplay | ( | ) | const |
Whether the entry should be suppressed in the K menu.
- Returns
- true to suppress this service
Such services still appear in trader queries, i.e. in "Open With" popup menus for instance.
Definition at line 704 of file kservice.cpp.
QString KService::parentApp | ( | ) | const |
Name of the application this service belongs to.
(Useful for e.g. plugins)
- Returns
- the parent application, or QString() if not set
Definition at line 722 of file kservice.cpp.
QString KService::path | ( | ) | const |
Returns the working directory to run the program in.
- Returns
- the working directory to run the program in, or QString() if not set
Definition at line 901 of file kservice.cpp.
QString KService::pluginKeyword | ( | ) | const |
The keyword to be used when constructing the plugin using KPluginFactory.
The keyword is defined with X-KDE-PluginKeyword in the .desktop file and with K_REGISTER_PLUGIN_WITH_KEYWORD when implementing the plugin.
Definition at line 733 of file kservice.cpp.
Returns the requested property.
- Parameters
-
_name the name of the property t the assumed type of the property
- Returns
- the property, or invalid if not found
- See also
- KServiceType
Definition at line 498 of file kservice.cpp.
|
static |
Find a service by the name of its desktop file, not depending on its actual location (as long as it's under the applnk or service directories).
For instance "konqbrowser" or "kcookiejar". Note that the ".desktop" extension is implicit.
This is the recommended method (safe even if the user moves stuff) but note that it assumes that no two entries have the same filename.
- Parameters
-
_name the name of the configuration file
- Returns
- a pointer to the requested service or 0 if the service is unknown. Very important: Don't store the result in a KService* !
Definition at line 620 of file kservice.cpp.
|
static |
Find a service based on its path as returned by entryPath().
It's usually better to use serviceByStorageId() instead.
- Parameters
-
_path the path of the configuration file
- Returns
- a pointer to the requested service or 0 if the service is unknown. Very important: Don't store the result in a KService* !
Definition at line 615 of file kservice.cpp.
|
static |
Find a service by its menu-id.
- Parameters
-
_menuId the menu id of the service
- Returns
- a pointer to the requested service or 0 if the service is unknown. Very important: Don't store the result in a KService* !
Definition at line 633 of file kservice.cpp.
|
static |
Find a service by name, i.e.
the translated Name field. Don't use this. Use serviceByDesktopPath or serviceByStorageId instead.
- Parameters
-
_name the name to search
- Returns
- a pointer to the requested service or 0 if the service is unknown. Very important: Don't store the result in a KService* !
- Deprecated:
- there is never a good reason to use this method.
Definition at line 609 of file kservice.cpp.
|
static |
Find a service by its storage-id or desktop-file path.
This function will try very hard to find a matching service.
- Parameters
-
_storageId the storage id or desktop-file path of the service
- Returns
- a pointer to the requested service or 0 if the service is unknown. Very important: Don't store the result in a KService* !
Definition at line 638 of file kservice.cpp.
QStringList KService::serviceTypes | ( | ) | const |
Returns the service types that this service supports.
- Returns
- the list of service types that are supported Note that this doesn't include inherited servicetypes or mimetypes, only the service types listed in the .desktop file.
Definition at line 936 of file kservice.cpp.
void KService::setExec | ( | const QString & | exec | ) |
Overrides the "Exec=" line of the service.
If exec is not empty, its value will override the one the one set when this service was created.
Please note that entryPath is also cleared so the service will no longer be associated with a specific config file.
- Since
- 4.11
Definition at line 979 of file kservice.cpp.
void KService::setMenuId | ( | const QString & | menuId | ) |
Set the menu id
Definition at line 777 of file kservice.cpp.
void KService::setTerminal | ( | bool | b | ) |
Sets whether to use a terminal or not
Definition at line 967 of file kservice.cpp.
void KService::setTerminalOptions | ( | const QString & | options | ) |
Sets the terminal options to use
Definition at line 973 of file kservice.cpp.
bool KService::showInKDE | ( | ) | const |
Whether the service should be shown in KDE at all (including in context menus).
- Returns
- true if the service should be shown.
KMimeTypeTrader honors this and removes such services from its results.
- Since
- 4.5
Definition at line 682 of file kservice.cpp.
QString KService::storageId | ( | ) | const |
Returns a normalized ID suitable for storing in configuration files.
It will be based on the menu-id when available and otherwise falls back to entryPath()
- Returns
- the storage ID
Definition at line 783 of file kservice.cpp.
bool KService::substituteUid | ( | ) | const |
Checks whether the service runs with a different user id.
- Returns
- true if the service has to be run under a different uid.
- See also
- username()
Definition at line 665 of file kservice.cpp.
bool KService::terminal | ( | ) | const |
Checks whethe the service should be run in a terminal.
- Returns
- true if the service is to be run in a terminal.
Definition at line 875 of file kservice.cpp.
QString KService::terminalOptions | ( | ) | const |
Returns any options associated with the terminal the service runs in, if it requires a terminal.
The service must be a tty-oriented program.
- Returns
- the terminal options, or QString() if not set
Definition at line 869 of file kservice.cpp.
QString KService::type | ( | ) | const |
Returns the type of the service.
- Returns
- the type of the service ("Application" or "Service")
Definition at line 839 of file kservice.cpp.
QString KService::untranslatedGenericName | ( | ) | const |
Returns the untranslated (US English) generic name for the service, if there is one (e.g.
"Mail Client").
- Returns
- the generic name, or QString() if not set
Definition at line 717 of file kservice.cpp.
QString KService::username | ( | ) | const |
Returns the user name, if the service runs with a different user id.
- Returns
- the username under which the service has to be run, or QString() if not set
- See also
- substituteUid()
Definition at line 670 of file kservice.cpp.
Friends And Related Function Documentation
|
friend |
Definition at line 52 of file kservice.cpp.
|
friend |
Definition at line 57 of file kservice.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.