KDECore
#include <KLibLoader>
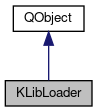
Public Types | |
enum | ComponentLoadingError { ErrNoLibrary = 1, ErrNoFactory, ErrNoComponent, ErrServiceProvidesNoLibrary, ErrNoServiceFound } |
Public Member Functions | |
KPluginFactory * | factory (const QString &libname, QLibrary::LoadHints loadHint=0) |
QString | lastErrorMessage () const |
KLibrary * | library (const QString &libname, QLibrary::LoadHints loadHint=0) |
void | unloadLibrary (const QString &libname) |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
template<typename T > | |
static T * | createInstance (const QString &keyword, const QString &libname, QObject *parent=0, const QVariantList &args=QVariantList(), int *error=0) |
template<typename T > | |
static T * | createInstance (const QString &libname, QObject *parent=0, const QVariantList &args=QVariantList(), int *error=0) |
template<typename T > | |
static T * | createInstance (const QString &libname, QObject *parent, const QStringList &args, int *error=0) |
static QString | errorString (int componentLoadingError) |
static QString | findLibrary (const QString &libname, const KComponentData &cData=KGlobal::mainComponent()) |
static KLibLoader * | self () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
The KLibLoader allows you to load libraries dynamically at runtime.
Dependent libraries are loaded automatically.
KLibLoader follows the singleton pattern. You can not create multiple instances. Use self() to get a pointer to the loader.
- Deprecated:
- You have two other possibilites:
- KPluginLoader or KService::createInstance for plugins
- KLibrary for other libraries
- See also
- KLibrary
- KPluginLoader
Definition at line 55 of file klibloader.h.
Member Enumeration Documentation
This enum type defines the possible error cases that can happen when loading a component.
Use errorString() to convert the error code to a human-readable string
Enumerator | |
---|---|
ErrNoLibrary | |
ErrNoFactory | |
ErrNoComponent | |
ErrServiceProvidesNoLibrary | |
ErrNoServiceFound |
Definition at line 165 of file klibloader.h.
Member Function Documentation
|
inlinestatic |
This template allows to load the specified library and ask the factory to create an instance of the given template type.
- Parameters
-
keyword the keyword for the plugin - see KPluginFactory::registerPlugin() libname the library to open parent the parent object (see QObject constructor) args a list of string arguments, passed to the factory and possibly to the component (see KPluginFactory) error if not null, the int will be set to 0 on success or one of the error codes defined by ComponentLoadingError if there was an error
- Returns
- a pointer to the newly created object or a null pointer if the factory was unable to create an object of the given type
- Deprecated:
- Use KService::createInstance() or KPluginLoader instead
Definition at line 202 of file klibloader.h.
|
inlinestatic |
This template allows to load the specified library and ask the factory to create an instance of the given template type.
- Parameters
-
libname the library to open parent the parent object (see QObject constructor) args a list of string arguments, passed to the factory and possibly to the component (see KPluginFactory) error if not null, the int will be set to 0 on success or one of the error codes defined by ComponentLoadingError if there was an error
- Returns
- a pointer to the newly created object or a null pointer if the factory was unable to create an object of the given type
- Deprecated:
- Use KService::createInstance() or KPluginLoader instead
Definition at line 248 of file klibloader.h.
|
inlinestatic |
- Deprecated:
- Use one of the other createInstance methods or KPluginLoader or KService::createInstance instead
Definition at line 260 of file klibloader.h.
|
static |
Converts a numerical error code into a human-readable error message.
- Parameters
-
componentLoadingError the error code, as set using the error
parameter of createInstance()
- Returns
- the translated error message describing the error represented by
componentLoadingError
- See also
- ComponentLoadingError
Definition at line 142 of file klibloader.cpp.
KPluginFactory * KLibLoader::factory | ( | const QString & | libname, |
QLibrary::LoadHints | loadHint = 0 |
||
) |
Loads and initializes a library.
Loading a library multiple times is handled gracefully.
This is a convenience function that returns the factory immediately
- Parameters
-
libname This is the library name without extension. Usually that is something like "libkspread". The function will then search for a file named "libkspread.la" in the KDE library paths. The *.la files are created by libtool and contain important information especially about the libraries dependencies on other shared libs. Loading a "libfoo.so" could not solve the dependencies problem.
You can, however, give a library name ending in ".so" (or whatever is used on your platform), and the library will be loaded without resolving dependencies. Use with caution.
- Parameters
-
loadHint provides more control over how the library is loaded
- Returns
- the KPluginFactory, or 0 if the library does not exist or it does not have a factory
- See also
- library
Definition at line 127 of file klibloader.cpp.
|
static |
Helper method which looks for a library in the standard paths ("module" and "lib" resources).
Made public for code that doesn't use KLibLoader itself, but still wants to open modules.
- Parameters
-
libname of the library. If it is not a path, the function searches in the "module" and "lib" resources. If there is no extension, ".la" will be appended. cData a KComponentData used to get the standard paths
- Returns
- the name of the library if it was found, an empty string otherwise
Definition at line 84 of file klibloader.cpp.
QString KLibLoader::lastErrorMessage | ( | ) | const |
Returns an error message that can be useful to debug the problem.
Returns QString() if the last call to library() was successful. You can call this function more than once. The error message is only reset by a new call to library().
- Returns
- the last error message, or QString() if there was no error
Definition at line 118 of file klibloader.cpp.
KLibrary * KLibLoader::library | ( | const QString & | libname, |
QLibrary::LoadHints | loadHint = 0 |
||
) |
Loads and initializes a library.
Loading a library multiple times is handled gracefully.
- Parameters
-
libname This is the library name without extension. Usually that is something like "libkspread". The function will then search for a file named "libkspread.la" in the KDE library paths. The *.la files are created by libtool and contain important information especially about the libraries dependencies on other shared libs. Loading a "libfoo.so" could not solve the dependencies problem.
You can, however, give a library name ending in ".so" (or whatever is used on your platform), and the library will be loaded without resolving dependencies. Use with caution.
- Parameters
-
loadHint provides more control over how the library is loaded
- Returns
- KLibrary is invalid (0) when the library couldn't be dlopened. in such a case you can retrieve the error message by calling KLibLoader::lastErrorMessage()
- See also
- factory
Definition at line 89 of file klibloader.cpp.
|
static |
Returns a pointer to the factory.
Use this function to get an instance of KLibLoader.
- Returns
- a pointer to the loader. If no loader exists until now then one is created.
- Deprecated:
- use KPluginLoader instead
Definition at line 46 of file klibloader.cpp.
void KLibLoader::unloadLibrary | ( | const QString & | libname | ) |
Unloads the library with the given name.
- Parameters
-
libname This is the library name without extension. Usually that is something like "libkspread". The function will then search for a file named "libkspread.la" in the KDE library paths. The *.la files are created by libtool and contain important information especially about the libraries dependencies on other shared libs. Loading a "libfoo.so" could not solve the dependencies problem.
You can, however, give a library name ending in ".so" (or whatever is used on your platform), and the library will be loaded without resolving dependencies. Use with caution.
Definition at line 123 of file klibloader.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.