KDECore
#include <ksycoca.h>
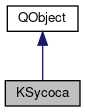
Public Types | |
enum | DatabaseType { LocalDatabase, GlobalDatabase } |
Signals | |
QT_MOC_COMPAT void | databaseChanged () |
void | databaseChanged (const QStringList &changedResources) |
Public Member Functions | |
KSycoca () | |
virtual | ~KSycoca () |
void | addFactory (KSycocaFactory *) |
QStringList | allResourceDirs () |
QDataStream * | findEntry (int offset, KSycocaType &type) |
QDataStream * | findFactory (KSycocaFactoryId id) |
virtual bool | isBuilding () |
QString | kfsstnd_prefixes () |
QString | language () |
quint32 | timeStamp () |
quint32 | updateSignature () |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static QString | absoluteFilePath (DatabaseType type=LocalDatabase) |
static void | disableAutoRebuild () |
static void | flagError () |
static bool | isAvailable () |
static bool | isChanged (const char *type) |
static bool | readError () |
static KSycoca * | self () |
static int | version () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Protected Member Functions | |
KSycoca (bool) | |
KSycocaFactoryList * | factories () |
QDataStream *& | stream () |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
Protected Attributes | |
QDataStream * | m_str_deprecated |
Additional Inherited Members | |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Member Enumeration Documentation
Constructor & Destructor Documentation
|
explicitprotected |
Building database
Definition at line 286 of file ksycoca.cpp.
KSycoca::KSycoca | ( | ) |
Read-only database.
Definition at line 180 of file ksycoca.cpp.
|
virtual |
Definition at line 300 of file ksycoca.cpp.
Member Function Documentation
|
static |
- returns absolute file path of the database
for global database type the database is searched under the 'services' install path. Otherwise, the value from the environment variable KDESYCOCA is returned if set. If not set the path is build based on KStandardDirs cache save location.
Definition at line 529 of file ksycoca.cpp.
void KSycoca::addFactory | ( | KSycocaFactory * | factory | ) |
- add a factory
Definition at line 341 of file ksycoca.cpp.
QStringList KSycoca::allResourceDirs | ( | ) |
- returns all directories with information stored inside sycoca.
Definition at line 552 of file ksycoca.cpp.
|
signal |
Connect to this to get notified when the database changes.
- Deprecated:
- use the databaseChanged(QStringList) signal
|
signal |
Connect to this to get notified when the database changes Example: when mimetype definitions have changed, applications showing files as icons refresh icons to take into account the new mimetypes.
Another example: after creating a .desktop file in KOpenWithDialog, it must wait until kbuildsycoca4 finishes until the KService::Ptr is available.
- Parameters
-
changedResources List of resources where changes were detected. This can include the following resources (as defined in KStandardDirs) : apps, xdgdata-apps, services, servicetypes, xdgdata-mime.
|
static |
- disables launching of kbuildsycoca
Definition at line 588 of file ksycoca.cpp.
|
protected |
Definition at line 383 of file ksycoca.cpp.
QDataStream * KSycoca::findEntry | ( | int | offset, |
KSycocaType & | type | ||
) |
- called by factories in read-only mode This is how factories get a stream to an entry
Definition at line 370 of file ksycoca.cpp.
QDataStream * KSycoca::findFactory | ( | KSycocaFactoryId | id | ) |
- called by factories in read-only mode Returns stream(), but positioned for reading this factory, 0 on error.
Definition at line 459 of file ksycoca.cpp.
|
static |
A read error occurs.
Definition at line 559 of file ksycoca.cpp.
|
static |
- Returns
- true if the ksycoca database is available This is usually the case, except if KDE isn't installed yet, or before kded is started.
Definition at line 309 of file ksycoca.cpp.
|
virtual |
- Returns
- true if building (i.e. if a KBuildSycoca);
Definition at line 583 of file ksycoca.cpp.
|
static |
When you receive a "databaseChanged" signal, you can query here if a change has occurred in a specific resource type.
- See also
- KStandardDirs for the various resource types.
This method is meant to be called from the GUI thread only.
- Deprecated:
- use the signal databaseChanged(QStringList) instead.
Definition at line 347 of file ksycoca.cpp.
QString KSycoca::kfsstnd_prefixes | ( | ) |
- returns kfsstnd stored inside database
Definition at line 487 of file ksycoca.cpp.
QString KSycoca::language | ( | ) |
- returns language stored inside database
Definition at line 545 of file ksycoca.cpp.
|
static |
Definition at line 577 of file ksycoca.cpp.
|
static |
Get or create the only instance of KSycoca (read-only)
Definition at line 293 of file ksycoca.cpp.
|
protected |
Definition at line 593 of file ksycoca.cpp.
quint32 KSycoca::timeStamp | ( | ) |
- returns timestamp of database
The database contains all changes made before this time and might contain changes made after that.
Definition at line 515 of file ksycoca.cpp.
quint32 KSycoca::updateSignature | ( | ) |
- returns update signature of database
Signature that keeps track of changes to $KDEDIR/share/services/update_ksycoca
Touching this file causes the database to be recreated from scratch.
Definition at line 522 of file ksycoca.cpp.
|
static |
- Returns
- the compiled-in version, i.e. the one used when writing a new ksycoca
Definition at line 150 of file ksycoca.cpp.
Member Data Documentation
|
protected |
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.