KDECore
#include <ksystemtimezone.h>
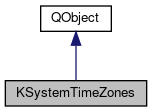
Public Member Functions | |
~KSystemTimeZones () | |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Static Public Member Functions | |
static bool | isSimulated () |
static bool | isTimeZoneDaemonAvailable () |
static KTimeZone | local () |
static KTimeZone | readZone (const QString &name) |
static KTimeZone | realLocalZone () |
static void | setLocalZone (const KTimeZone &tz) |
static KTimeZones * | timeZones () |
static KTimeZone | zone (const QString &name) |
static QString | zoneinfoDir () |
static const KTimeZones::ZoneMap | zones () |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
Additional Inherited Members | |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
The KSystemTimeZones class represents the system time zone database, consisting of a collection of individual system time zone definitions, indexed by name.
Each individual time zone is defined in a KSystemTimeZone or KTzfileTimeZone instance. Additional time zones (of any class derived from KTimeZone) may be added if desired.
At initialisation, KSystemTimeZones on UNIX systems reads the zone.tab file to obtain the list of system time zones, and creates a KTzfileTimeZone instance for each one.
- Note
- KSystemTimeZones gets the system's time zone configuration, including the current local system time zone and the location of zone.tab, from the KDE time zone daemon, ktimezoned. If ktimezoned cannot be started, KSystemTimeZones will only know about the UTC time zone.
Note that KSystemTimeZones is not derived from KTimeZones, but instead contains a KTimeZones instance which holds the system time zone database. Convenience static methods are defined to access its data, or alternatively you can access the KTimeZones instance directly via the timeZones() method.
As an example, find the local time in Oman corresponding to the local system time of 12:15:00 on 13th November 1999:
- Note
- KTzfileTimeZone is used in preference to KSystemTimeZone on UNIX systems since use of the standard system libraries by KSystemTimeZone requires the use of tzset() in several methods. That function reads and parses the local system time zone definition file every time it is called, and this has been observed to make applications hang for many seconds when a large number of KSystemTimeZone calls are made in succession.
- This class provides a facility to simulate the local system time zone. This facility is provided for testing purposes only, and is only available if the library is compiled with debug enabled. In release mode, simulation is inoperative and the real local system time zone is used at all times.
System time zone access
Definition at line 94 of file ksystemtimezone.h.
Constructor & Destructor Documentation
KSystemTimeZones::~KSystemTimeZones | ( | ) |
Definition at line 191 of file ksystemtimezone.cpp.
Member Function Documentation
|
static |
Check whether there is a simulated local system time zone.
- Warning
- This method is provided only for testing purposes, and should not be used in released code. If the library is compiled without debug enabled, isSimulated() always returns false. To avoid confusion, it is recommended that calls to it should be conditionally compiled, e.g.:
- See also
- setLocalZone()
- Since
- 4.3
Definition at line 219 of file ksystemtimezone.cpp.
|
static |
Return whether the KDE time zone daemon, ktimezoned, appears to be available and working.
If not, UTC will be the only recognized time zone.
- Since
- 4.6
Definition at line 234 of file ksystemtimezone.cpp.
|
static |
Returns the current local system time zone.
The idea of this routine is to provide a robust lookup of the local time zone. On Unix systems, there are a variety of mechanisms for setting this information, and no well defined way of getting it. For example, if you set your time zone to "Europe/London", then the tzname[] maintained by tzset() typically returns { "GMT", "BST" }. The function of this routine is to actually return "Europe/London" (or rather, the corresponding KTimeZone).
Note that depending on how the system stores its current time zone, this routine may return a synonym of the expected time zone. For example, "Europe/London", "Europe/Guernsey" and some other time zones are all identical and there may be no way for the routine to distinguish which of these is the correct zone name from the user's point of view.
- Warning
- For testing purposes, if the library is compiled with debug enabled, this method returns any simulated local system time zone set by setLocalZone(). If the library is compiled in release mode, it always returns the real local system time zone.
- Returns
- local system time zone. If necessary, we will use a series of heuristics which end by returning UTC. We will never return NULL. Note that if UTC is returned as a default, it may not belong to the the collection returned by KSystemTimeZones::zones().
Definition at line 195 of file ksystemtimezone.cpp.
Returns the time zone with the given name, containing the full time zone definition read directly from the system time zone database.
This may incur a higher overhead than zone(), but will provide whatever historical data the system holds.
- Parameters
-
name name of time zone
- Returns
- time zone (usually a KTzfileTimeZone instance), or invalid if not found
- See also
- zone()
Definition at line 245 of file ksystemtimezone.cpp.
|
static |
Return the real (not simulated) local system time zone.
- Warning
- This method is provided only for testing purposes, and should not be used in released code. If the library is compiled without debug enabled, local() and realLocalZone() both return the real local system time zone. To avoid confusion, it is recommended that calls to realLocalZone() should be conditionally compiled, e.g.:
- See also
- setLocalZone()
- Since
- 4.3
Definition at line 205 of file ksystemtimezone.cpp.
|
static |
Set or clear the simulated local system time zone.
- Warning
- This method is provided only for testing purposes, and should not be used in released code. If the library is compiled without debug enabled, setLocalZone() has no effect. To avoid confusion, it is recommended that calls to it should be conditionally compiled, e.g.:
- Parameters
-
tz the time zone to simulate, or an invalid KTimeZone instance (i.e. ) to cancel simulationtz.isValid() == false
- Since
- 4.3
Definition at line 211 of file ksystemtimezone.cpp.
|
static |
Returns the unique KTimeZones instance containing the system time zones collection.
It is first created if it does not already exist.
- Returns
- time zones.
Definition at line 240 of file ksystemtimezone.cpp.
Returns the time zone with the given name.
The time zone definition is obtained using system library calls, and may not contain historical data. If you need historical time change data, use the potentially slower method readZone().
- Parameters
-
name name of time zone
- Returns
- time zone (usually a KSystemTimeZone instance), or invalid if not found
- See also
- readZone()
Definition at line 255 of file ksystemtimezone.cpp.
|
static |
Returns the location of the system time zone zoneinfo database.
- Returns
- path of directory containing the zoneinfo database
Definition at line 228 of file ksystemtimezone.cpp.
|
static |
Returns all the time zones defined in this collection.
- Returns
- time zone collection
Definition at line 250 of file ksystemtimezone.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.