KDECore
#include <ktimezone.h>
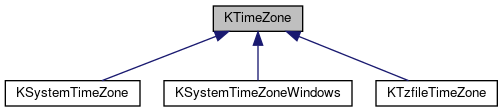
Classes | |
class | LeapSeconds |
class | Phase |
class | Transition |
Public Member Functions | |
KTimeZone () | |
KTimeZone (const QString &name) | |
KTimeZone (const KTimeZone &tz) | |
virtual | ~KTimeZone () |
QByteArray | abbreviation (const QDateTime &utcDateTime) const |
QList< QByteArray > | abbreviations () const |
QString | comment () const |
QDateTime | convert (const KTimeZone &newZone, const QDateTime &zoneDateTime) const |
QString | countryCode () const |
int | currentOffset (Qt::TimeSpec basis=Qt::UTC) const |
const KTimeZoneData * | data (bool create=false) const |
virtual bool | hasTransitions () const |
virtual bool | isDst (time_t t) const |
virtual bool | isDstAtUtc (const QDateTime &utcDateTime) const |
bool | isValid () const |
float | latitude () const |
QList< LeapSeconds > | leapSecondChanges () const |
float | longitude () const |
QString | name () const |
virtual int | offset (time_t t) const |
virtual int | offsetAtUtc (const QDateTime &utcDateTime) const |
virtual int | offsetAtZoneTime (const QDateTime &zoneDateTime, int *secondOffset=0) const |
bool | operator!= (const KTimeZone &rhs) const |
KTimeZone & | operator= (const KTimeZone &tz) |
bool | operator== (const KTimeZone &rhs) const |
bool | parse () const |
QList< Phase > | phases () const |
KTimeZoneSource * | source () const |
QDateTime | toUtc (const QDateTime &zoneDateTime) const |
QDateTime | toZoneTime (const QDateTime &utcDateTime, bool *secondOccurrence=0) const |
const KTimeZone::Transition * | transition (const QDateTime &dt, const Transition **secondTransition=0, bool *validTime=0) const |
int | transitionIndex (const QDateTime &dt, int *secondIndex=0, bool *validTime=0) const |
QList< KTimeZone::Transition > | transitions (const QDateTime &start=QDateTime(), const QDateTime &end=QDateTime()) const |
QList< QDateTime > | transitionTimes (const Phase &phase, const QDateTime &start=QDateTime(), const QDateTime &end=QDateTime()) const |
QByteArray | type () const |
bool | updateBase (const KTimeZone &other) |
QList< int > | utcOffsets () const |
Static Public Member Functions | |
static QDateTime | fromTime_t (time_t t) |
static time_t | toTime_t (const QDateTime &utcDateTime) |
static KTimeZone | utc () |
Static Public Attributes | |
static const int | InvalidOffset = 0x80000000 |
static const time_t | InvalidTime_t = 0x80000000 |
static const float | UNKNOWN = 1000.0 |
Protected Member Functions | |
KTimeZone (KTimeZoneBackend *impl) | |
void | setData (KTimeZoneData *data, KTimeZoneSource *source=0) |
Detailed Description
Base class representing a time zone.
The KTimeZone base class contains general descriptive data about the time zone, and provides an interface for methods to read and parse time zone definitions, and to translate between UTC and local time. Derived classes must implement these methods, and may also hold the actual details of the dates and times of daylight savings changes, offsets from UTC, etc. They should be tailored to deal with the type and format of data held by a particular type of time zone database.
If this base class is instantiated as a valid instance, it always represents the UTC time zone.
KTimeZone is designed to work in partnership with KTimeZoneSource. KTimeZone provides access to individual time zones, while classes derived from KTimeZoneSource read and parse a particular format of time zone definition. Because time zone sources can differ in what information they provide about time zones, the parsed data retured by KTimeZoneSource can vary between different sources, resulting in the need to create different KTimeZone classes to handle the data.
KTimeZone instances are often grouped into KTimeZones collections.
Copying KTimeZone instances is very efficient since the class data is explicitly shared, meaning that only a pointer to the data is actually copied. To achieve this, each class inherited from KTimeZone must have a corresponding backend class derived from KTimeZoneBackend.
- Note
- Classes derived from KTimeZone should not have their own d-pointer. The d-pointer is instead contained in their backend class (derived from KTimeZoneBackend). This allows KTimeZone's reference-counting of private data to take care of the derived class's data as well, ensuring that instance data is not deleted while any references to the class instance remains. All virtual methods which override KTimeZone virtual methods must be defined in the backend class instead.
Base class representing a time zone
- See also
- KTimeZoneBackend, KTimeZoneSource, KTimeZoneData
Definition at line 416 of file ktimezone.h.
Constructor & Destructor Documentation
KTimeZone::KTimeZone | ( | ) |
Constructs a null time zone.
A null time zone is invalid.
- See also
- isValid()
Definition at line 600 of file ktimezone.cpp.
|
explicit |
Constructs a UTC time zone.
- Parameters
-
name name of the UTC time zone
Definition at line 604 of file ktimezone.cpp.
KTimeZone::KTimeZone | ( | const KTimeZone & | tz | ) |
Definition at line 608 of file ktimezone.cpp.
|
virtual |
Definition at line 612 of file ktimezone.cpp.
|
protected |
Definition at line 617 of file ktimezone.cpp.
Member Function Documentation
QByteArray KTimeZone::abbreviation | ( | const QDateTime & | utcDateTime | ) | const |
Returns the time zone abbreviation current at a specified time.
- Parameters
-
utcDateTime UTC date/time. An error occurs if utcDateTime.timeSpec()
is not Qt::UTC.
- Returns
- time zone abbreviation, or empty string if error
- See also
- abbreviations()
Definition at line 681 of file ktimezone.cpp.
QList< QByteArray > KTimeZone::abbreviations | ( | ) | const |
Returns the list of time zone abbreviations used by the time zone.
This may include historical ones which are no longer in use or have been superseded.
- Returns
- list of abbreviations
- See also
- abbreviation()
Definition at line 674 of file ktimezone.cpp.
QString KTimeZone::comment | ( | ) | const |
Returns any comment for the time zone.
- Returns
- comment, may be empty
Definition at line 664 of file ktimezone.cpp.
Converts a date/time, which is interpreted as being local time in this time zone, into local time in another time zone.
- Parameters
-
newZone other time zone which the time is to be converted into zoneDateTime local date/time. An error occurs if zoneDateTime.timeSpec()
is not Qt::LocalTime.
- Returns
- converted date/time, or invalid date/time if error
- See also
- toUtc(), toZoneTime()
Definition at line 854 of file ktimezone.cpp.
QString KTimeZone::countryCode | ( | ) | const |
Returns the two-letter country code of the time zone.
- Returns
- upper case ISO 3166 2-character country code, empty if unknown
Definition at line 649 of file ktimezone.cpp.
int KTimeZone::currentOffset | ( | Qt::TimeSpec | basis = Qt::UTC | ) | const |
Returns the current offset of this time zone to UTC or the local system time zone.
The offset is the number of seconds which you must add to UTC or the local system time to get local time in this time zone.
Take care if you cache the results of this routine; that would break if the result were stored across a daylight savings change.
- Parameters
-
basis Qt::UTC to return the offset to UTC, Qt::LocalTime to return the offset to local system time
- Returns
- offset in seconds
- See also
- offsetAtZoneTime(), offsetAtUtc()
Definition at line 880 of file ktimezone.cpp.
const KTimeZoneData * KTimeZone::data | ( | bool | create = false | ) | const |
Returns the detailed parsed data for the time zone.
This will return null unless either parse() has been called beforehand, or create
is true.
- Parameters
-
create true to parse the zone's data first if not already parsed
- Returns
- pointer to data, or null if data has not been parsed
Definition at line 754 of file ktimezone.cpp.
|
static |
Converts a UTC time, measured in seconds since 00:00:00 UTC 1st January 1970 (as returned by time(2)), to a UTC QDateTime value.
QDateTime::setTime_t() is limited to handling t
>= 0, since its parameter is unsigned. This method takes a parameter of time_t which is signed.
- Returns
- converted time
- See also
- toTime_t()
Definition at line 917 of file ktimezone.cpp.
|
virtual |
Return whether daylight saving transitions are available for the time zone.
The base class returns false
.
- Returns
true
if transitions are available,false
if not
- See also
- transitions(), transition()
Definition at line 702 of file ktimezone.cpp.
|
virtual |
Returns whether daylight savings time is in operation at a specified UTC time.
Note that time_t has a more limited range than QDateTime, so consider using isDstAtUtc() instead.
- Parameters
-
t the UTC time, measured in seconds since 00:00:00 UTC 1st January 1970 (as returned by time(2))
- Returns
true
if daylight savings time is in operation,false
otherwise
- See also
- isDstAtUtc()
Definition at line 906 of file ktimezone.cpp.
Returns whether daylight savings time is in operation at the given UTC date/time.
If a derived class needs to work in terms of time_t (as when accessing the system time functions, for example), it should override both this method and isDst() so as to implement its offset calculations in isDst(), and reimplement this method simply as
- Parameters
-
utcDateTime the UTC date/time. An error occurs if utcDateTime.timeSpec()
is not Qt::UTC.
- Returns
true
if daylight savings time is in operation,false
otherwise
- See also
- isDst()
Definition at line 901 of file ktimezone.cpp.
bool KTimeZone::isValid | ( | ) | const |
Checks whether the instance is valid.
- Returns
- true if valid, false if invalid
Definition at line 644 of file ktimezone.cpp.
float KTimeZone::latitude | ( | ) | const |
Returns the latitude of the time zone.
- Returns
- latitude in degrees, UNKNOWN if not known
Definition at line 654 of file ktimezone.cpp.
QList< KTimeZone::LeapSeconds > KTimeZone::leapSecondChanges | ( | ) | const |
Return all leap second adjustments, in time order.
Note that some time zone data sources (such as system time zones accessed via the system libraries) may not provide information on leap second adjustments. In such cases, this method will return an empty list.
- Returns
- list of adjustments
Definition at line 742 of file ktimezone.cpp.
float KTimeZone::longitude | ( | ) | const |
Returns the latitude of the time zone.
- Returns
- latitude in degrees, UNKNOWN if not known
Definition at line 659 of file ktimezone.cpp.
QString KTimeZone::name | ( | ) | const |
Returns the name of the time zone.
If it is held in a KTimeZones container, the name is the time zone's unique identifier within that KTimeZones instance.
- Returns
- name in system-dependent format
Definition at line 669 of file ktimezone.cpp.
|
virtual |
Returns the offset of this time zone to UTC at a specified UTC time.
The offset is the number of seconds which you must add to UTC to get local time in this time zone.
Note that time_t has a more limited range than QDateTime, so consider using offsetAtUtc() instead.
- Parameters
-
t the UTC time at which the offset is to be calculated, measured in seconds since 00:00:00 UTC 1st January 1970 (as returned by time(2))
- Returns
- offset in seconds, or 0 if error
- See also
- offsetAtUtc()
Definition at line 875 of file ktimezone.cpp.
|
virtual |
Returns the offset of this time zone to UTC at the given UTC date/time.
The offset is the number of seconds which you must add to UTC to get local time in this time zone.
If a derived class needs to work in terms of time_t (as when accessing the system time functions, for example), it should override both this method and offset() so as to implement its offset calculations in offset(), and reimplement this method simply as
- Parameters
-
utcDateTime the UTC date/time at which the offset is to be calculated. An error occurs if utcDateTime.timeSpec()
is not Qt::UTC.
- Returns
- offset in seconds, or 0 if error
- See also
- offset(), offsetAtZoneTime(), currentOffset()
Definition at line 870 of file ktimezone.cpp.
|
virtual |
Returns the offset of this time zone to UTC at the given local date/time.
Because of daylight savings time shifts, the date/time may occur twice. Optionally, the offsets at both occurrences of dateTime
are calculated.
The offset is the number of seconds which you must add to UTC to get local time in this time zone.
- Parameters
-
zoneDateTime the date/time at which the offset is to be calculated. This is interpreted as a local time in this time zone. An error occurs if zoneDateTime.timeSpec()
is not Qt::LocalTime.secondOffset if non-null, and the zoneDateTime
occurs twice, receives the UTC offset for the second occurrence. Otherwise, it is set the same as the return value.
- Returns
- offset in seconds. If
zoneDateTime
occurs twice, it is the offset at the first occurrence which is returned. IfzoneDateTime
does not exist because of daylight savings time shifts, InvalidOffset is returned. If any other error occurs, 0 is returned.
- See also
- offsetAtUtc(), currentOffset()
Definition at line 865 of file ktimezone.cpp.
Definition at line 633 of file ktimezone.h.
Definition at line 624 of file ktimezone.cpp.
Checks whether this is the same instance as another one.
Note that only the pointers to the time zone data are compared, not the contents. So it will only return equality if one instance was copied from the other.
- Parameters
-
rhs other instance
- Returns
- true if the same instance, else false
Definition at line 634 of file ktimezone.cpp.
bool KTimeZone::parse | ( | ) | const |
Extracts time zone detail information for this time zone from the source database.
- Returns
false
if the parse encountered errors,true
otherwise
Definition at line 784 of file ktimezone.cpp.
QList< KTimeZone::Phase > KTimeZone::phases | ( | ) | const |
Return all daylight savings time phases for the time zone.
Note that some time zone data sources (such as system time zones accessed via the system libraries) may not allow a list of daylight savings time changes to be compiled easily. In such cases, this method will return an empty list.
- Returns
- list of phases
Definition at line 695 of file ktimezone.cpp.
|
protected |
Sets the detailed parsed data for the time zone, and optionally a new time zone source object.
- Parameters
-
data parsed data source if non-null, the new source object for the time zone
- See also
- data()
Definition at line 763 of file ktimezone.cpp.
KTimeZoneSource * KTimeZone::source | ( | ) | const |
Returns the source reader/parser for the time zone's source database.
- Returns
- reader/parser
Definition at line 749 of file ktimezone.cpp.
|
static |
Converts a UTC QDateTime to a UTC time, measured in seconds since 00:00:00 UTC 1st January 1970 (as returned by time(2)).
QDateTime::toTime_t() returns an unsigned value. This method returns a time_t value, which is signed.
- Parameters
-
utcDateTime date/time. An error occurs if utcDateTime.timeSpec()
is not Qt::UTC.
- Returns
- converted time, or -1 if the date is out of range for time_t or
utcDateTime.timeSpec()
is not Qt::UTC
- See also
- fromTime_t()
Definition at line 934 of file ktimezone.cpp.
Converts a date/time, which is interpreted as local time in this time zone, into UTC.
Because of daylight savings time shifts, the date/time may occur twice. In such cases, this method returns the UTC time for the first occurrence. If you need the UTC time of the second occurrence, use offsetAtZoneTime().
- Parameters
-
zoneDateTime local date/time. An error occurs if zoneDateTime.timeSpec()
is not Qt::LocalTime.
- Returns
- UTC date/time, or invalid date/time if error
- See also
- toZoneTime(), convert()
Definition at line 796 of file ktimezone.cpp.
QDateTime KTimeZone::toZoneTime | ( | const QDateTime & | utcDateTime, |
bool * | secondOccurrence = 0 |
||
) | const |
Converts a UTC date/time into local time in this time zone.
Because of daylight savings time shifts, some local date/time values occur twice. The secondOccurrence
parameter may be used to determine whether the time returned is the first or second occurrence of that time.
- Parameters
-
utcDateTime UTC date/time. An error occurs if utcDateTime.timeSpec()
is not Qt::UTC.secondOccurrence if non-null, returns true
if the return value is the second occurrence of that time, elsefalse
- Returns
- local date/time, or invalid date/time if error
Definition at line 808 of file ktimezone.cpp.
const KTimeZone::Transition * KTimeZone::transition | ( | const QDateTime & | dt, |
const Transition ** | secondTransition = 0 , |
||
bool * | validTime = 0 |
||
) | const |
Find the last daylight savings time transition at or before a given UTC or local time.
Because of daylight savings time shifts, a local time may occur twice or may not occur at all. In the former case, the transitions at or before both occurrences of dt
may optionally be calculated and returned in secondTransition
. The latter case may optionally be detected by use of validTime
.
- Parameters
-
dt date/time. dt.timeSpec()
may be set to Qt::UTC or Qt::LocalTime.secondTransition if non-null, and the dt
occurs twice, receives the transition for the second occurrence. Otherwise, it is set the same as the return value.validTime if non-null, is set to false if dt
does not occur, or to true otherwise
- Returns
- time zone transition, or null either if
dt
is either outside the defined range of the transition data or ifdt
does not occur
- See also
- transitionIndex(), hasTransitions(), transitions()
Definition at line 714 of file ktimezone.cpp.
int KTimeZone::transitionIndex | ( | const QDateTime & | dt, |
int * | secondIndex = 0 , |
||
bool * | validTime = 0 |
||
) | const |
Find the index to the last daylight savings time transition at or before a given UTC or local time.
The return value is the index into the transition list returned by transitions().
Because of daylight savings time shifts, a local time may occur twice or may not occur at all. In the former case, the transitions at or before both occurrences of dt
may optionally be calculated and returned in secondIndex
. The latter case may optionally be detected by use of validTime
.
- Parameters
-
dt date/time. dt.timeSpec()
may be set to Qt::UTC or Qt::LocalTime.secondIndex if non-null, and the dt
occurs twice, receives the index to the transition for the second occurrence. Otherwise, it is set the same as the return value.validTime if non-null, is set to false if dt
does not occur, or to true otherwise
- Returns
- index into the time zone transition list, or -1 either if
dt
is either outside the defined range of the transition data or ifdt
does not occur
- See also
- transition(), transitions(), hasTransitions()
Definition at line 725 of file ktimezone.cpp.
QList< KTimeZone::Transition > KTimeZone::transitions | ( | const QDateTime & | start = QDateTime() , |
const QDateTime & | end = QDateTime() |
||
) | const |
Return all daylight saving transitions, in time order.
If desired, the transitions returned may be restricted to a specified time range.
Note that some time zone data sources (such as system time zones accessed via the system libraries) may not allow a list of daylight saving time changes to be compiled easily. In such cases, this method will return an empty list.
- Parameters
-
start start UTC date/time, or invalid date/time to return all transitions up to end
.start.timeSpec()
must be Qt::UTC, elsestart
will be considered invalid.end end UTC date/time, or invalid date/time for no end. end.timeSpec()
must be Qt::UTC, elseend
will be considered invalid.
- Returns
- list of transitions, in time order
- See also
- hasTransitions(), transition(), transitionTimes()
Definition at line 707 of file ktimezone.cpp.
QList< QDateTime > KTimeZone::transitionTimes | ( | const Phase & | phase, |
const QDateTime & | start = QDateTime() , |
||
const QDateTime & | end = QDateTime() |
||
) | const |
Return the times of all daylight saving transitions to a given time zone phase, in time order.
If desired, the times returned may be restricted to a specified time range.
Note that some time zone data sources (such as system time zones accessed via the system libraries) may not allow a list of daylight saving time changes to be compiled easily. In such cases, this method will return an empty list.
- Parameters
-
phase time zone phase start start UTC date/time, or invalid date/time to return all transitions up to end
.start.timeSpec()
must be Qt::UTC, elsestart
will be considered invalid.end end UTC date/time, or invalid date/time for no end. end.timeSpec()
must be Qt::UTC, elseend
will be considered invalid.
- Returns
- ordered list of transition times
- See also
- hasTransitions(), transition(), transitions()
Definition at line 735 of file ktimezone.cpp.
QByteArray KTimeZone::type | ( | ) | const |
Returns the class name of the data represented by this instance.
If a derived class object has been assigned to this instance, this method will return the name of that class.
- Returns
- "KTimeZone" or the class name of a derived class
Definition at line 639 of file ktimezone.cpp.
Update the definition of the time zone to be identical to another KTimeZone instance.
A prerequisite is that the two instances must have the same name.
The main purpose of this method is to allow updates of the time zone definition by derived classes without invalidating pointers to the instance (particularly pointers held by KDateTime objects). Note that the KTimeZoneData object and KTimeZoneSource pointer are not updated: the caller class should do this itself by calling setData().
- Parameters
-
other time zone whose definition is to be used
- Returns
- true if definition was updated (i.e. names are the same)
- See also
- setData()
Definition at line 773 of file ktimezone.cpp.
|
static |
Returns a standard UTC time zone, with name "UTC".
- Note
- The KTimeZone returned by this method does not belong to any KTimeZones collection. Any KTimeZones instance may contain its own UTC KTimeZone defined by its time zone source data, but that will be a different instance than this KTimeZone.
- Returns
- UTC time zone
Definition at line 911 of file ktimezone.cpp.
QList< int > KTimeZone::utcOffsets | ( | ) | const |
Returns the complete list of UTC offsets used by the time zone.
This may include historical ones which are no longer in use or have been superseded.
A UTC offset is the number of seconds which you must add to UTC to get local time in this time zone.
If due to the nature of the source data for the time zone, compiling a complete list would require significant processing, an empty list is returned instead.
- Returns
- sorted list of UTC offsets, or empty list if not readily available.
Definition at line 688 of file ktimezone.cpp.
Member Data Documentation
|
static |
Indicates an invalid UTC offset.
This is returned by offsetAtZoneTime() when the local time does not occur due to a shift to daylight savings time.
Definition at line 1073 of file ktimezone.h.
|
static |
Indicates an invalid time_t value.
Definition at line 1077 of file ktimezone.h.
|
static |
A representation for unknown locations; this is a float that does not represent a real latitude or longitude.
Definition at line 1083 of file ktimezone.h.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.