KDECore
#include <ktimezone.h>
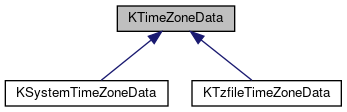
Protected Member Functions | |
void | setLeapSecondChanges (const QList< KTimeZone::LeapSeconds > &adjusts) |
void | setPhases (const QList< KTimeZone::Phase > &phases, const KTimeZone::Phase &previousPhase) |
void | setPhases (const QList< KTimeZone::Phase > &phases, int previousUtcOffset) |
void | setTransitions (const QList< KTimeZone::Transition > &transitions) |
Detailed Description
Base class for the parsed data returned by a KTimeZoneSource class.
It contains all the data available from the KTimeZoneSource class, including, when available, a complete list of daylight savings time changes and leap seconds adjustments.
This base class can be instantiated, but contains no data.
Base class for parsed time zone data
- See also
- KTimeZone, KTimeZoneSource
Definition at line 1302 of file ktimezone.h.
Constructor & Destructor Documentation
KTimeZoneData::KTimeZoneData | ( | ) |
Definition at line 1144 of file ktimezone.cpp.
KTimeZoneData::KTimeZoneData | ( | const KTimeZoneData & | c | ) |
Definition at line 1148 of file ktimezone.cpp.
|
virtual |
Definition at line 1159 of file ktimezone.cpp.
Member Function Documentation
|
virtual |
Returns the time zone abbreviation current at a specified time.
- Parameters
-
utcDateTime UTC date/time. An error occurs if utcDateTime.timeSpec()
is not Qt::UTC.
- Returns
- time zone abbreviation, or empty string if error
- See also
- abbreviations()
Reimplemented in KSystemTimeZoneData.
Definition at line 1197 of file ktimezone.cpp.
|
virtual |
Returns the complete list of time zone abbreviations.
This may include translations.
- Returns
- the list of abbreviations. In this base class, it consists of the single string "UTC".
- See also
- abbreviation()
Reimplemented in KSystemTimeZoneData.
Definition at line 1180 of file ktimezone.cpp.
|
virtual |
Creates a new copy of this object.
The caller is responsible for deleting the copy. Derived classes must reimplement this method to return a copy of the calling instance.
- Returns
- copy of this instance
Reimplemented in KSystemTimeZoneData, and KTzfileTimeZoneData.
Definition at line 1175 of file ktimezone.cpp.
|
virtual |
Return whether daylight saving transitions are available for the time zone.
The base class returns false
.
- Returns
true
if transitions are available,false
if not
- See also
- transitions(), transition()
Reimplemented in KTzfileTimeZoneData.
Definition at line 1244 of file ktimezone.cpp.
KTimeZone::LeapSeconds KTimeZoneData::leapSecondChange | ( | const QDateTime & | utc | ) | const |
Find the leap second adjustment which is applicable at a given UTC time.
- Parameters
-
utc UTC date/time. An error occurs if utc.timeSpec()
is not Qt::UTC.
- Returns
- leap second adjustment, or invalid if
utc
is earlier than the first leap second adjustment orutc
is a local time
Definition at line 1385 of file ktimezone.cpp.
QList< KTimeZone::LeapSeconds > KTimeZoneData::leapSecondChanges | ( | ) | const |
Return all leap second adjustments, in time order.
Note that some time zone data sources (such as system time zones accessed via the system libraries) may not provide information on leap second adjustments. In such cases, this method will return an empty list.
- Returns
- list of adjustments
Definition at line 1375 of file ktimezone.cpp.
KTimeZoneData & KTimeZoneData::operator= | ( | const KTimeZoneData & | c | ) |
Definition at line 1164 of file ktimezone.cpp.
QList< KTimeZone::Phase > KTimeZoneData::phases | ( | ) | const |
Return all daylight savings time phases.
Note that some time zone data sources (such as system time zones accessed via the system libraries) may not allow a list of daylight savings time changes to be compiled easily. In such cases, this method will return an empty list.
- Returns
- list of phases
Definition at line 1227 of file ktimezone.cpp.
int KTimeZoneData::previousUtcOffset | ( | ) | const |
Returns the UTC offset to use before the start of data for the time zone.
- Returns
- UTC offset
Definition at line 1266 of file ktimezone.cpp.
|
protected |
Initialise the leap seconds adjustment list.
- Parameters
-
adjusts list of adjustments
- See also
- leapSecondChanges()
Definition at line 1380 of file ktimezone.cpp.
|
protected |
Initialise the daylight savings time phase list.
- Parameters
-
phases list of phases previousPhase phase to use before the first daylight savings time transition
- See also
- phases()
- Since
- 4.8.3
Definition at line 1232 of file ktimezone.cpp.
|
protected |
Initialise the daylight savings time phase list.
This setPhases() variant should be used when the time zone abbreviation used before the start of the first phase is not known.
- Parameters
-
phases list of phases previousUtcOffset UTC offset to use before the start of the first phase
- See also
- phases()
Definition at line 1238 of file ktimezone.cpp.
|
protected |
Initialise the daylight savings time transition list.
- Parameters
-
transitions list of transitions
- See also
- transitions()
Definition at line 1261 of file ktimezone.cpp.
const KTimeZone::Transition * KTimeZoneData::transition | ( | const QDateTime & | dt, |
const KTimeZone::Transition ** | secondTransition = 0 , |
||
bool * | validTime = 0 |
||
) | const |
Find the last daylight savings time transition at or before a given UTC or local time.
Because of daylight savings time shifts, a local time may occur twice or may not occur at all. In the former case, the transitions at or before both occurrences of dt
may optionally be calculated and returned in secondTransition
. The latter case may optionally be detected by use of validTime
.
- Parameters
-
dt date/time. dt.timeSpec()
may be set to Qt::UTC or Qt::LocalTime.secondTransition if non-null, and the dt
occurs twice, receives the transition for the second occurrence. Otherwise, it is set the same as the return value.validTime if non-null, is set to false if dt
does not occur, or to true otherwise
- Returns
- time zone transition, or null either if
dt
is either outside the defined range of the transition data or ifdt
does not occur
- See also
- transitionIndex(), hasTransitions(), transitions()
Definition at line 1271 of file ktimezone.cpp.
int KTimeZoneData::transitionIndex | ( | const QDateTime & | dt, |
int * | secondIndex = 0 , |
||
bool * | validTime = 0 |
||
) | const |
Find the index to the last daylight savings time transition at or before a given UTC or local time.
The return value is the index into the transition list returned by transitions().
Because of daylight savings time shifts, a local time may occur twice or may not occur at all. In the former case, the transitions at or before both occurrences of dt
may optionally be calculated and returned in secondIndex
. The latter case may optionally be detected by use of validTime
.
- Parameters
-
dt date/time. dt.timeSpec()
may be set to Qt::UTC or Qt::LocalTime.secondIndex if non-null, and the dt
occurs twice, receives the index to the transition for the second occurrence. Otherwise, it is set the same as the return value.validTime if non-null, is set to false if dt
does not occur, or to true otherwise
- Returns
- index into the time zone transition list, or -1 either if
dt
is either outside the defined range of the transition data or ifdt
does not occur
- See also
- transition(), transitions(), hasTransitions()
Definition at line 1281 of file ktimezone.cpp.
QList< KTimeZone::Transition > KTimeZoneData::transitions | ( | const QDateTime & | start = QDateTime() , |
const QDateTime & | end = QDateTime() |
||
) | const |
Return all daylight saving transitions, in time order.
If desired, the transitions returned may be restricted to a specified time range.
Note that some time zone data sources (such as system time zones accessed via the system libraries) may not allow a list of daylight saving time changes to be compiled easily. In such cases, this method will return an empty list.
- Parameters
-
start start date/time, or invalid date/time to return all transitions up to end
.start.timeSpec()
must be Qt::UTC, elsestart
will be considered invalid.end end date/time, or invalid date/time for no end. end.timeSpec()
must be Qt::UTC, elseend
will be considered invalid.
- Returns
- list of transitions, in time order
- See also
- hasTransitions(), transition(), transitionTimes()
Definition at line 1249 of file ktimezone.cpp.
QList< QDateTime > KTimeZoneData::transitionTimes | ( | const KTimeZone::Phase & | phase, |
const QDateTime & | start = QDateTime() , |
||
const QDateTime & | end = QDateTime() |
||
) | const |
Return the times of all daylight saving transitions to a given time zone phase, in time order.
If desired, the times returned may be restricted to a specified time range.
Note that some time zone data sources (such as system time zones accessed via the system libraries) may not allow a list of daylight saving time changes to be compiled easily. In such cases, this method will return an empty list.
- Parameters
-
phase time zone phase start start UTC date/time, or invalid date/time to return all transitions up to end
.start.timeSpec()
must be Qt::UTC, elsestart
will be considered invalid.end end UTC date/time, or invalid date/time for no end. end.timeSpec()
must be Qt::UTC, elseend
will be considered invalid.
- Returns
- ordered list of transition times
- See also
- hasTransitions(), transition(), transitions()
Definition at line 1358 of file ktimezone.cpp.
|
virtual |
Returns the complete list of UTC offsets for the time zone, if the time zone's source makes such information readily available.
If compiling a complete list would require significant processing, an empty list is returned instead.
- Returns
- sorted list of UTC offsets, or empty list if not readily available. In this base class, it consists of the single value 0.
Reimplemented in KSystemTimeZoneData.
Definition at line 1209 of file ktimezone.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:22:13 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.