KDEUI
#include <kdualaction.h>
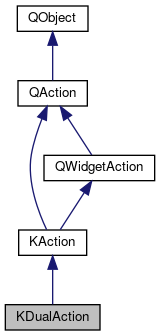
Public Slots | |
void | setActive (bool state) |
Signals | |
void | activeChanged (bool) |
void | activeChangedByUser (bool) |
![]() | |
void | authorized (KAuth::Action *action) |
void | globalShortcutChanged (const QKeySequence &) |
void | triggered (Qt::MouseButtons buttons, Qt::KeyboardModifiers modifiers) |
Additional Inherited Members | |
![]() | |
enum | GlobalShortcutLoading { Autoloading = 0x0, NoAutoloading = 0x4 } |
enum | ShortcutType { ActiveShortcut = 0x1, DefaultShortcut = 0x2 } |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QList< QWidget * > | createdWidgets () const |
virtual QWidget * | createWidget (QWidget *parent) |
virtual void | deleteWidget (QWidget *widget) |
virtual bool | eventFilter (QObject *obj, QEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
KShortcut | globalShortcut |
bool | globalShortcutAllowed |
bool | globalShortcutEnabled |
KShortcut | shortcut |
bool | shortcutConfigurable |
![]() | |
autoRepeat | |
checkable | |
checked | |
enabled | |
font | |
icon | |
iconText | |
iconVisibleInMenu | |
menuRole | |
priority | |
shortcut | |
shortcutContext | |
softKeyRole | |
statusTip | |
text | |
toolTip | |
visible | |
whatsThis | |
![]() | |
objectName | |
Detailed Description
An action which can alternate between two texts/icons when triggered.
KDualAction should be used when you want to create an action which alternate between two states when triggered but which should not be rendered as a checkable widget because it is more appropriate to change the text and icon of the action instead.
You should use KDualAction to implement this kind of actions instead of KToggleAction because KToggleAction is rendered as a checkable widget: this means one of your state will have a checkbox in a menu and will be represented as a sunken button in a toolbar.
Porting from KToggleAction to KDualAction:
- If you used the KToggleAction constructor which accepts the action text, adjust the constructor: KDualAction constructor accepts both inactive and active text.
- Replace connections to the checked(bool) signal with a connection to the activeChanged(bool) (or activeChangedByUser(bool))
- Replace calls to setChecked()/isChecked() with setActive()/isActive()
- Replace calls to setCheckedState(guiItem) with setActiveGuiItem(guiItem)
- Since
- 4.6
Definition at line 58 of file kdualaction.h.
Constructor & Destructor Documentation
|
explicit |
Constructs a KDualAction with the specified parent.
Texts must be set with setTextForState() or setGuiItemForState().
Definition at line 71 of file kdualaction.cpp.
KDualAction::KDualAction | ( | const QString & | inactiveText, |
const QString & | activeText, | ||
QObject * | parent | ||
) |
Constructs a KDualAction with the specified parent and texts.
Definition at line 61 of file kdualaction.cpp.
KDualAction::~KDualAction | ( | ) |
Definition at line 78 of file kdualaction.cpp.
Member Function Documentation
|
signal |
Emitted when the state changes.
This signal is emitted when the user trigger the action and when setActive() is called.
|
signal |
Only emitted when the state changes because the user triggered the action.
KGuiItem KDualAction::activeGuiItem | ( | ) | const |
Gets the KGuiItem for the active state.
Definition at line 84 of file kdualaction.cpp.
QIcon KDualAction::activeIcon | ( | ) | const |
Gets the icon for the active state.
Definition at line 89 of file kdualaction.cpp.
QString KDualAction::activeText | ( | ) | const |
Gets the text for the active state.
Definition at line 94 of file kdualaction.cpp.
QString KDualAction::activeToolTip | ( | ) | const |
Gets the tooltip for the active state.
Definition at line 99 of file kdualaction.cpp.
bool KDualAction::autoToggle | ( | ) | const |
Returns whether the current action will automatically be changed when the user triggers this action.
The default value is true.
Definition at line 114 of file kdualaction.cpp.
KGuiItem KDualAction::inactiveGuiItem | ( | ) | const |
Gets the KGuiItem for the inactive state.
Definition at line 86 of file kdualaction.cpp.
QIcon KDualAction::inactiveIcon | ( | ) | const |
Gets the icon for the inactive state.
Definition at line 91 of file kdualaction.cpp.
QString KDualAction::inactiveText | ( | ) | const |
Gets the text for the inactive state.
Definition at line 96 of file kdualaction.cpp.
QString KDualAction::inactiveToolTip | ( | ) | const |
Gets the tooltip for the inactive state.
Definition at line 101 of file kdualaction.cpp.
bool KDualAction::isActive | ( | ) | const |
Returns the action state.
The action is inactive by default.
Definition at line 129 of file kdualaction.cpp.
|
slot |
Sets the action state.
activeChanged() will be emitted but not activeChangedByUser().
Definition at line 119 of file kdualaction.cpp.
void KDualAction::setActiveGuiItem | ( | const KGuiItem & | item | ) |
Sets the KGuiItem for the active state.
Definition at line 83 of file kdualaction.cpp.
void KDualAction::setActiveIcon | ( | const QIcon & | icon | ) |
Sets the icon for the active state.
Definition at line 88 of file kdualaction.cpp.
void KDualAction::setActiveText | ( | const QString & | text | ) |
Sets the text for the active state.
Definition at line 93 of file kdualaction.cpp.
void KDualAction::setActiveToolTip | ( | const QString & | toolTip | ) |
Sets the tooltip for the active state.
Definition at line 98 of file kdualaction.cpp.
void KDualAction::setAutoToggle | ( | bool | value | ) |
Defines whether the current action should automatically be changed when the user triggers this action.
Definition at line 109 of file kdualaction.cpp.
void KDualAction::setIconForStates | ( | const QIcon & | icon | ) |
Convenience method to set the icon for both active and inactive states.
Definition at line 103 of file kdualaction.cpp.
void KDualAction::setInactiveGuiItem | ( | const KGuiItem & | item | ) |
Sets the KGuiItem for the inactive state.
Definition at line 85 of file kdualaction.cpp.
void KDualAction::setInactiveIcon | ( | const QIcon & | icon | ) |
Sets the icon for the inactive state.
Definition at line 90 of file kdualaction.cpp.
void KDualAction::setInactiveText | ( | const QString & | text | ) |
Sets the text for the inactive state.
Definition at line 95 of file kdualaction.cpp.
void KDualAction::setInactiveToolTip | ( | const QString & | toolTip | ) |
Sets the tooltip for the inactive state.
Definition at line 100 of file kdualaction.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:02 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.