KDEUI
#include <khelpmenu.h>
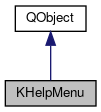
Public Types | |
enum | MenuId { menuHelpContents = 0, menuWhatsThis = 1, menuAboutApp = 2, menuAboutKDE = 3, menuReportBug = 4, menuSwitchLanguage = 5 } |
Public Slots | |
void | aboutApplication () |
void | aboutKDE () |
void | appHelpActivated () |
void | contextHelpActivated () |
void | reportBug () |
void | switchApplicationLanguage () |
Signals | |
void | showAboutApplication () |
Public Member Functions | |
KHelpMenu (QWidget *parent=0, const QString &aboutAppText=QString(), bool showWhatsThis=true) | |
KHelpMenu (QWidget *parent, const KAboutData *aboutData, bool showWhatsThis=true, KActionCollection *actions=0) | |
~KHelpMenu () | |
QAction * | action (MenuId id) const |
KMenu * | menu () |
![]() | |
QObject (QObject *parent) | |
QObject (QObject *parent, const char *name) | |
virtual | ~QObject () |
bool | blockSignals (bool block) |
QObject * | child (const char *objName, const char *inheritsClass, bool recursiveSearch) const |
const QObjectList & | children () const |
const char * | className () const |
bool | connect (const QObject *sender, const char *signal, const char *method, Qt::ConnectionType type) const |
void | deleteLater () |
void | destroyed (QObject *obj) |
bool | disconnect (const QObject *receiver, const char *method) |
bool | disconnect (const char *signal, const QObject *receiver, const char *method) |
void | dumpObjectInfo () |
void | dumpObjectTree () |
QList< QByteArray > | dynamicPropertyNames () const |
virtual bool | event (QEvent *e) |
virtual bool | eventFilter (QObject *watched, QEvent *event) |
T | findChild (const QString &name) const |
QList< T > | findChildren (const QRegExp ®Exp) const |
QList< T > | findChildren (const QString &name) const |
bool | inherits (const char *className) const |
void | insertChild (QObject *object) |
void | installEventFilter (QObject *filterObj) |
bool | isA (const char *className) const |
bool | isWidgetType () const |
void | killTimer (int id) |
virtual const QMetaObject * | metaObject () const |
void | moveToThread (QThread *targetThread) |
const char * | name () const |
const char * | name (const char *defaultName) const |
QString | objectName () const |
QObject * | parent () const |
QVariant | property (const char *name) const |
void | removeChild (QObject *object) |
void | removeEventFilter (QObject *obj) |
void | setName (const char *name) |
void | setObjectName (const QString &name) |
void | setParent (QObject *parent) |
bool | setProperty (const char *name, const QVariant &value) |
bool | signalsBlocked () const |
int | startTimer (int interval) |
QThread * | thread () const |
Additional Inherited Members | |
![]() | |
bool | connect (const QObject *sender, const char *signal, const QObject *receiver, const char *method, Qt::ConnectionType type) |
bool | connect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method, Qt::ConnectionType type) |
bool | disconnect (const QObject *sender, const char *signal, const QObject *receiver, const char *method) |
bool | disconnect (const QObject *sender, const QMetaMethod &signal, const QObject *receiver, const QMetaMethod &method) |
QString | tr (const char *sourceText, const char *disambiguation, int n) |
QString | trUtf8 (const char *sourceText, const char *disambiguation, int n) |
![]() | |
bool | checkConnectArgs (const char *signal, const QObject *object, const char *method) |
virtual void | childEvent (QChildEvent *event) |
virtual void | connectNotify (const char *signal) |
virtual void | customEvent (QEvent *event) |
virtual void | disconnectNotify (const char *signal) |
int | receivers (const char *signal) const |
QObject * | sender () const |
int | senderSignalIndex () const |
virtual void | timerEvent (QTimerEvent *event) |
![]() | |
QByteArray | normalizeSignalSlot (const char *signalSlot) |
![]() | |
objectName | |
Detailed Description
Standard KDE help menu with dialog boxes.
This class provides the standard KDE help menu with the default "about" dialog boxes and help entry.
This class is used in KMainWindow so normally you don't need to use this class yourself. However, if you need the help menu or any of its dialog boxes in your code that is not subclassed from KMainWindow you should use this class.
The usage is simple:
or if you just want to open a dialog box:
IMPORTANT: The first time you use KHelpMenu::menu(), a KMenu object is allocated. Only one object is created by the class so if you call KHelpMenu::menu() twice or more, the same pointer is returned. The class will destroy the popupmenu in the destructor so do not delete this pointer yourself.
The KHelpMenu object will be deleted when its parent is destroyed but you can delete it yourself if you want. The code below will always work.
Using your own "about application" dialog box:
The standard "about application" dialog box is quite simple. If you need a dialog box with more functionality you must design that one yourself. When you want to display the dialog, you simply need to connect the help menu signal showAboutApplication() to your slot.
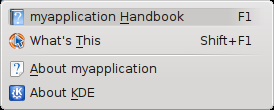
Definition at line 110 of file khelpmenu.h.
Member Enumeration Documentation
enum KHelpMenu::MenuId |
Enumerator | |
---|---|
menuHelpContents | |
menuWhatsThis | |
menuAboutApp | |
menuAboutKDE | |
menuReportBug | |
menuSwitchLanguage |
Definition at line 168 of file khelpmenu.h.
Constructor & Destructor Documentation
|
explicit |
Constructor.
- Parameters
-
parent The parent of the dialog boxes. The boxes are modeless and will be centered with respect to the parent. aboutAppText User definable string that is used in the default application dialog box. showWhatsThis Decides whether a "Whats this" entry will be added to the dialog.
Definition at line 107 of file khelpmenu.cpp.
KHelpMenu::KHelpMenu | ( | QWidget * | parent, |
const KAboutData * | aboutData, | ||
bool | showWhatsThis = true , |
||
KActionCollection * | actions = 0 |
||
) |
Constructor.
This alternative constructor is mainly useful if you want to overide the standard actions (aboutApplication(), aboutKDE(), helpContents(), reportBug, and optionally whatsThis).
- Parameters
-
parent The parent of the dialog boxes. The boxes are modeless and will be centered with respect to the parent. aboutData User and app data used in the About app dialog showWhatsThis Decides whether a "Whats this" entry will be added to the dialog. actions KActionCollection that is used instead of the standard actions.
Definition at line 117 of file khelpmenu.cpp.
KHelpMenu::~KHelpMenu | ( | ) |
Destructor.
Destroys dialogs and the menu pointer retuned by menu
Definition at line 142 of file khelpmenu.cpp.
Member Function Documentation
|
slot |
Opens an application specific dialog box.
The method will try to open the about box using the following steps:
- If the showAboutApplication() signal is connected, then it will be called. This means there is an application defined aboutBox.
- If the aboutData was set in the constructor a KAboutApplicationDialog will be created.
- Else a default about box using the aboutAppText from the constructor will be created.
Definition at line 270 of file khelpmenu.cpp.
|
slot |
Opens the standard "About KDE" dialog box.
Definition at line 315 of file khelpmenu.cpp.
Returns the QAction * associated with the given parameter Will return NULL pointers if menu() has not been called.
- Parameters
-
id The id of the action of which you want to get QAction *
Definition at line 232 of file khelpmenu.cpp.
|
slot |
Opens the help page for the application.
The application name is used as a key to determine what to display and the system will attempt to open <appName>/index.html.
Definition at line 264 of file khelpmenu.cpp.
|
slot |
Activates What's This help for the application.
Definition at line 384 of file khelpmenu.cpp.
KMenu * KHelpMenu::menu | ( | ) |
Returns a popup menu you can use in the menu bar or where you need it.
The returned menu is configured with an icon, a title and menu entries. Therefore adding the returned pointer to your menu is enougth to have access to the help menu.
Note: This method will only create one instance of the menu. If you call this method twice or more the same pointer is returned.
Definition at line 181 of file khelpmenu.cpp.
|
slot |
Opens the standard "Report Bugs" dialog box.
Definition at line 326 of file khelpmenu.cpp.
|
signal |
This signal is emitted from aboutApplication() if no "about application" string has been defined.
The standard application specific dialog box that is normally activated in aboutApplication() will not be displayed when this signal is emitted.
|
slot |
Opens the changing default application language dialog box.
Definition at line 337 of file khelpmenu.cpp.
The documentation for this class was generated from the following files:
Documentation copyright © 1996-2020 The KDE developers.
Generated on Mon Jun 22 2020 13:24:02 by doxygen 1.8.7 written by Dimitri van Heesch, © 1997-2006
KDE's Doxygen guidelines are available online.